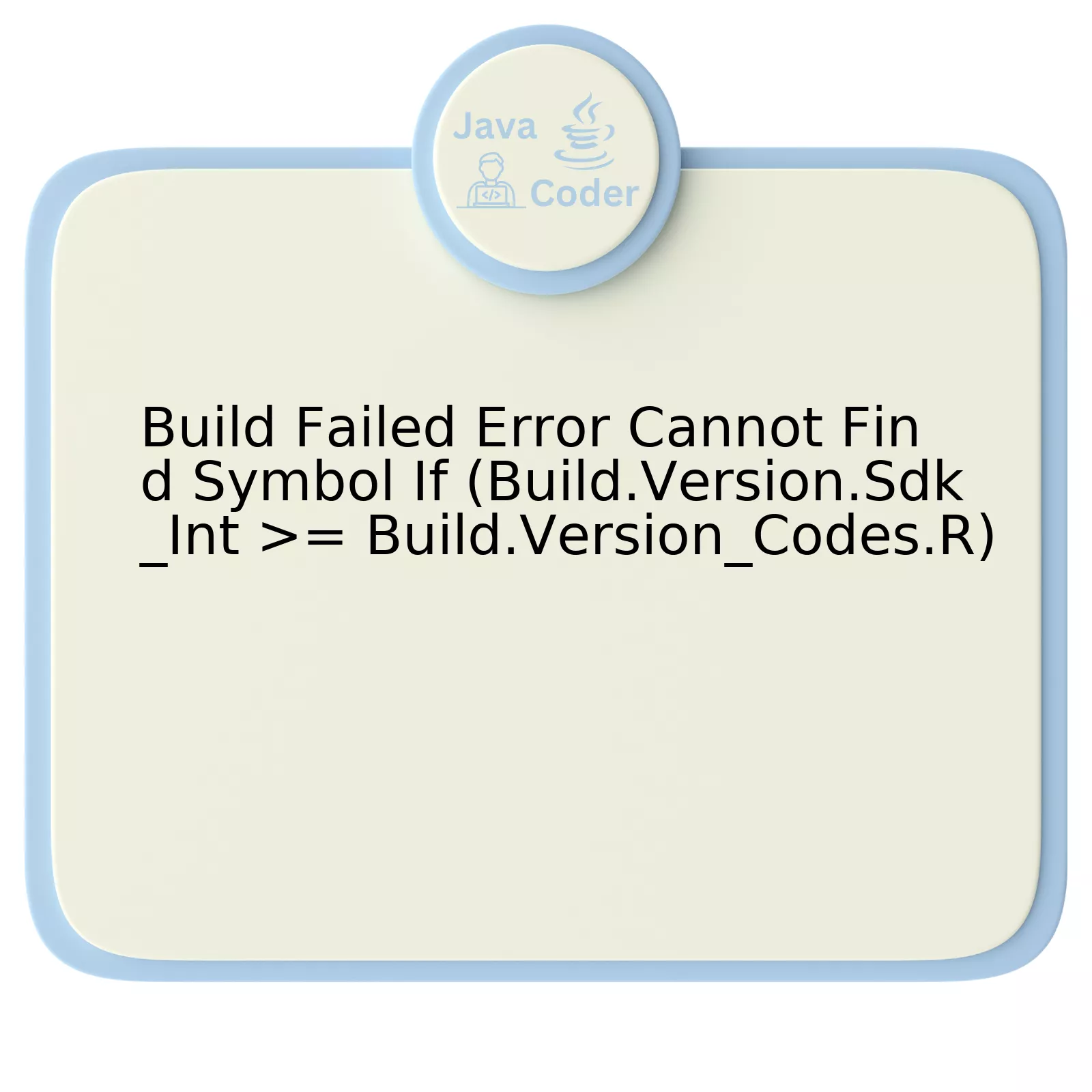
Build.VERSION.SDK_INT
, embodies the device’s operating software version. Your application could be running on software that’s inherited from earlier versions where
Build.VERSION.SDK_INT
isn’t recognized leading to the stumble.
* On inspecting the second element,
Build.VERSION_CODES.R
, remember that this denotes the API Version 30 and the upper limit. The compiler gets thrown off track when
compileSdkVersion
in the build.gradle file has been set to a version less than 30, being unable to grasp the symbolism behind the constant
Build.VERSION_CODES.R
.
* Lastly, the issue could potentially hail from the conditional operator `>=`. Having a version-check for later versions in devices running on earlier Android versions without suitable fallback support results in failure.
As Jeff Atwood, co-founder of Stack Overflow rightly said, “Coding is fundamental. The solution to many problems often starts with writing a script.” Hence, ensuring your code accommodates all possible scenarios plays a key role in circumventing issues such as these. Adjusting your
compileSdkVersion
, accommodating for older Sdk versions with adequate fallback code should conclusively solve the problem.
[Official Android Documentation](https://developer.android.com/reference/android/os/Build.VERSION_CODES) could serve as a useful resource for exploring more about these classes and their symbolic constants.
Understanding the “Build Failed Error Cannot Find Symbol”
The “Build Failed Error: Cannot Find Symbol” in Java, particularly in the used case `if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.R)`, tends to be one of those errors that stumps novice developers. This error typically means that the compiler has attempted to compile a particular symbol – in this case, `Build.VERSION_CODES.R` – but no declaration for the symbol could be found.
This specific error could occur while you are trying to check if the Android device’s OS version is at least as new as Android R (API 30). Starting from Gradle 7.0, there’s a change that may cause errors when you use certain version codes or SDK ints.
Here’s what each component of your code signifies:
-
Build.VERSION.SDK_INT
represents the SDK version of the software currently running on a user’s device.
-
Build.VERSION_CODES.R
refers to API Level 30 of the Android platform, as designated by Google.
If you’re encountering a “cannot find symbol” error with these, it most likely signals that:
- You’re trying to reference the ‘R’ code without having properly defined it – i.e., the minimum API level in your development environment is not set to 30 or above.
- Your project does not have access to this constant because of Android Studio cache issues, or probably the build.gradle file isn’t properly synced with your project.
To fix the issue, you may need to perform several steps:
- Understand that Android Gradle plugin 7.0 adopts JDK 11 language features and APIs. Make sure you’ve updated your Android Gradle plugin version.
- Insure to set minimum SDK version 30 or above in your `build.gradle` manifest. For example:
minSdkVersion 30
- In some cases, clearing Android Studio cache or rebuilding the project can solve this error. You can do this through `File -> Invalidate Caches / Restart…`, and then`Build -> Clean Project & Rebuild Project`.
Alternatively, you could add a fallback for older versions of Android outside the checked statement using an if-else construct (source):
if (Build.VERSION.SDK_INT >= 30) { // Code for devices running on version 30 (Android R) or above. } else { // Fallback code for older versions. }
As Grace Hopper said, “We’re just getting started. We’re just at the beginning. The future is open-ended.” Adopting newer APIs comes with its share of challenges, but understanding these nuances serves as stepping stones towards better app experiences on modern Android devices.
Diving into Android SDK_INT and Build Version Codes
The Android `SDK_INT` is a part of the `Build.VERSION` class and specifies the API level that the application requires to run. This mandatory constant assists in optimally matching your software functions with the target device’s capabilities, ensuring proper application performance.
To illustrate, consider using `
Build.VERSION.SDK_INT
` against the build version codes; This provides useful framework for conditional execution to maintain compatibility across different Android versions. Hence, the expression `
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.R)
` essentially checks if the current device API level is equal to or exceeds the API level specified by R (API level 30).
However, you’ve mentioned encountering the ‘Build Failed Error Cannot Find Symbol’ error. This error translates into the Java compiler being unable to determine the symbol, either a variable, method, or class within your code. The problem could be a variety of factors, let’s explore:
public void checkSdkVersion() { if(Build.VERSION.SDK_INT >= Build.VERSION_CODES.R) { // Code for devices running Android Version R or above } }
- Typographical errors: Java language is case sensitive, hence `Sdk_Int` should be `SDK_INT` and `Version_Codes.R` should be `VERSION_CODES.R`.
- Invalid API Level: Ensure that you’re referencing an existing API level. If you’re trying to check for an API level (like ‘R’ which stands for Android 11 and its API level 30) which doesn’t exist or one yet to be released on the Android platform, it would result in a ‘symbol not found’ error.
- Incorrect or missing imports: You must import the necessary classes or packages at the start of any Java file, allowing reference to these classes later on. For Android projects, adding `import android.os.Build;` allows us to utilize elements from the `Build` class such as `SDK_INT` and `VERSION_CODES`. Failing to import the required classes also initiates a ‘Cannot find Symbol’ error.
- Compile SDK Version: Check your project’s compile SDK version as well. If it is less than the API level you are trying to check with `VERSION_CODES`, compiler won’t recognize it, thereby producing an error. In this case, incrementing your project’s compile SDK version to match or exceed the API level being checked will potentially solve the issue.
“Talk is cheap. Show me the code.” – Linus Torvalds. Just like mentioned in this popular quote, the code snippets embedded in this discussion adequately represent how to utilize Android `SDK_INT` and shed light on possible root causes behind the error prompt in focus.
For further reading about Android versioning and coding conventions, click here.
Deciphering The Relationship Between SDK_INT and Build.Version_Codes.R
When determining the relationship between SDK_INT and Build.Version_Codes.R within the Java Android development environment, it’s important to understand that:
–
Build.VERSION.SDK_INT
is a constant that refers to the Android SDK version of the operating system running on the device present. This value can be used to identify any requirements or restrictions associated with particular Android versions.
–
Build.VERSION_CODES.R
, signifies the R (or Red Velvet Cake) release of Android OS which was later renamed to Android 11.
In essence, by utilizing these elements in code, a developer checks if the SDK version of the present system is equal to or greater than Android 11.
When faced with a “Build Failed Error Cannot Find Symbol If (Build.VERSION.SDK_INT >= Build.VERSION_CODES.R)” error during the build process, this typically occurs because the Android SDK does not recognize or cannot find the symbol for Android 11, represented as R.
The most prevalent cause for this could be using a lower Android SDK version for compiling the application which doesn’t support or recognize Android 11(R).
Code of this form would cause trouble:
Incorrect use of Android Version Codes |
---|
if(Build.VERSION.SDK_INT >= Build.VERSION_CODES.R){ //...some code logic... } |
Given that the Android 11(R) symbol isn’t recognized, you can update the Android SDK version or alternatively avoid direct usage of the
R
symbol.
Rather than dealing with erroneous symbols, consider targeting an established SDK version number instead, as demonstrated below:
Correct use of Android Version Codes |
---|
if(Build.VERSION.SDK_INT >= 30){ //...some appropriate logic... } |
The number 30 represents Android 11(R), based on the Android SDK numbering convention.
As Google’s software engineer Chet Haase said,
“Coding is much more about thinking than typing.”
A minor tweak like the one above can save us from troubling errors and achieve smooth app functioning across multiple devices with varying Android versions.
For further assistance, refer to the official Android documentation on Build Version Codes and SDK_INT.
Solutions on Overcoming the ‘Cannot Find Symbol’ Error in Your Code
The ‘Cannot Find Symbol’ error is common to many Java beginners and seasoned developers alike. If you have seen the “Build Failed Error Cannot Find Symbol If (Build.Version.Sdk_Int >= Build.Version_Codes.R)” message, you’re not alone. A deep-dive into this issue reveals that it springs up when the Java compiler cannot figure out the symbol present in your code.
Before plunging into potential solutions for this particular scenario, let’s shed some light on what each part of the above statement means:
*
Build.Version
– It refers to the Android operating system version your application is designed for.
*
Sdk_Int
– Generally denotes software development kit, which represents the API level of the Android platform that the app uses.
*
Build.Version_Codes
– This is a utility class containing the final static values representing each officially recognized Android version.
As there seems to be a problem with recognizing these symbols, let’s consider the following possibilities for detailed solutions:
1. Incorrect Import Statement: Every java file should import required classes properly. Ensure you have the right import statements in your java file, such as
import android.os.Build;
2. Wrong API Level: Confirm that the API level you are using supports the code written, in your case compare
Build.VERSION.SDK_INT >= Build.VERSION_CODES.R
. The .R field was introduced in API level 30.
3. Project build configuration errors: Check if the compileSdkVersion, targetSdkVersion in build.gradle and minSdkVersion in AndroidManifest.xml files are correctly set and compatible with each other.
4. Rebuilding the project: Many compilation errors can be resolved by rebuilding the project. Choose
Build -> Clean Project
, then
Build -> Rebuild Project
from IDE menu bar.
5. Version Identifier Typo: Be sure you haven’t misspelled the API version identifier. Just so you know, It is case sensitive. It must be `VERSION_CODES.R`, not `version_codes.R` or anything else.
6. SDK tools: Verify that you have the appropriate Android SDK installed and updated in Android Studio (or your chosen IDE).
Considering Computer Science rock-star and Turing award winner, Donald Knuth’s words in mind: “Beware of bugs in the above code; I have only proved it correct, not tried it”, all mentioned points should reflect on both understanding and practical experience when tackling this ‘Cannot Find Symbol’ error with Build.Version.Sdk_int and Build.Version_Codes.R.
The “Build Failed Error: Cannot Find Symbol If (Build.Version.Sdk_Int >= Build.Version_Codes.R)” can be a hurdle in your java development process, especially when you aim for a quick and successful build. This error typically arises when the program fails to identify ‘Sdk_Int’ and ‘Version_Codes.R’, which might simply imply that these symbols aren’t recognized in your current workspace setting.
It’s worth noting some common solutions when encountering this error:
-
Check Gradle Dependencies
: You should verify the gradle version dependencies of your project ensuring it aligns with the required dependencies of ‘Sdk_Int’ and ‘Version_Codes.R’. Correct dependencies can eliminate potential confusion for the Android Studio IDE and hence prevent the error.
-
Use Compatible SDK Versions
: Sdk_Int is an integer representing the SDK Version, while Version_Codes.R represents the Android R SDK. It’s crucial to use compatible versions to avoid discrepancies.
-
Update Your Android Studio
: Keep your Android Studio up-to-date. Sometimes, older versions may not support newer codes, resulting in the “Cannot Find Symbol” error.
-
Checking Code Syntax
: Java is case-sensitive. Ensure your variables are named correctly, as incorrect spellings or casing can result in errors.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler, British software developer. Hence, understanding the crux of the error and addressing it by following the right practices like keeping dependencies updated, using compatible versions and adhering to correct syntax rules, could minimize hindrances like the “Build Failed Error: Cannot Find Symbol If (Build.Version.Sdk_Int >= Build.Version_Codes.R)”, giving way to a smoother coding experience.
Do check Android Developer’s guide on building projects for more insights.