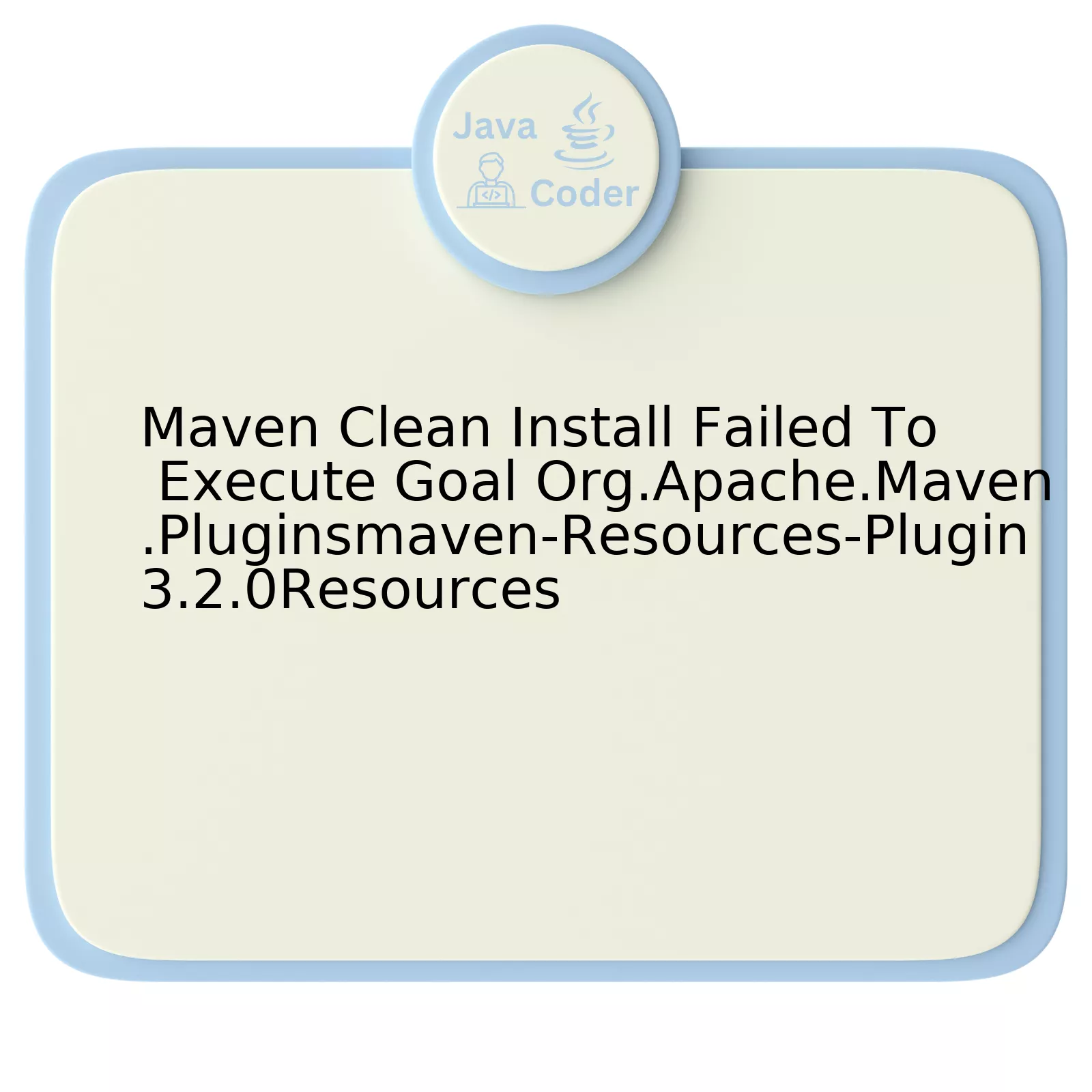
The problem ‘Maven Clean Install Failed To Execute Goal Org.Apache.Maven.Pluginsmaven-Resources-Plugin3.2.0Resources’ is a prevalent one amongst developers using Maven, a powerful tool for managing projects built in Java.
If we want to better understand the scope of this issue, it can be beneficial to lay out the contributing factors within a structured analysis.
Potential Cause | Solution approach |
---|---|
Resource is not available in the path | Ensure that all resource references in the pom.xml file have the accurate and correct location where resources reside. |
Incorrect Plugin version in POM.xml | Match the plugin version in your pom.xml with the exact ones compatible with Maven installed in your development environment. |
Error in the configuration of pom.xml | Check if all the necessary configurations are present and if they are defined correctly. |
Maven compatibility issues | Some plugins might not work correctly with some versions of Maven. Make sure both the version of Maven and plugins used are compatible. |
Issues like “Failed To Execute Goal Org.Apache.Maven.Pluginsmaven-Resources-Plugin3.2.0Resources” often occur due to the missing resources from the specified path or malfunctioning plugins. It’s critical to check whether you’ve declared the correct path in your pom.xml files.
An incorrect Plugin version in your project’s POM.xml file could be a source of the issue. Therefore, you should ensure that the plugin versions declared in your project’s POM.xml match the actual installed versions.
Another possible reason could be a wrong configuration within your project’s pom.xml file. Scan through all the configurations carefully and rectify any errors you encounter. Also, don’t forget to cross-check whether the essential elements such as groupId, artifactId, version, name, URL, etc., are appropriately defined.
Finally, compatibility issues with Maven may cause this type of error. Always double-check the exact Maven and plugins versions your application requires before proceeding with the build process.
As Addy Osmani, a Google engineer, once said, “First, solve the problem. Then, write the code.” By following the structured analysis and solution steps outlined above, you’ll be well on your way to overcome the challenges with the perplexing error “Failed To Execute Goal Org.Apache.Maven.Pluginsmaven-Resources-Plugin3.2.0Resources”.
Understanding the Error: Maven Clean Install Failure
Understanding and diagnosing the error: ‘Maven Clean Install Failure’ in relation to the specific resource failure: “Failed to execute goal org.apache.maven.plugins:maven-resources-plugin:3.2.0:resources” requires an understanding of how Maven functions and the components of its lifecycle.
1. Maven uses a specific sequence of stages when building a project, this is referred to as the build lifecycle. It includes stages such as
compile
,
test
,
package
and
install
. The clean lifecycle is separate from this for cleaning generated project artifacts before starting anew. If this clean function operates unsuccessfully it can result in a Maven Install Failure.
2. Failure during the execution of a specific goal by one of the plugins i.e., maven-resources-plugin:3.2.0:resources, could mean that the plugin may not be accessible, incompatible with your version of Maven or Java, or there may exist some misconfiguration in its usage.
Delving into the issue, further possibilities are:
– Version Incompatibility: The version of the plugin you’re using may not be compatible with your version of Maven. Check the version compatibility matrix to ensure the versions align.
– Settings in POM File: Your Project Object Model (POM) file might not have been set up correctly. Double-check all configurations and paths given in the POM file.
– Missing Files / Dependencies: The error could occur if the resources’ files or dependencies outlined within the plugin configuration couldn’t be located.
To correct the problem, applying remediation steps sequentially is often helpful in diagnostics and resolution.
– Version Compatibility Check: Make sure your versions of Maven, the resources plugin, and Java match their compatible pairs.
Sample Code:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-resources-plugin</artifactId> <version>(compatible version)</version> </plugin>
– Review and Correct POM configurations: Ensure every referenced path, file, and property in your POM.xml exists and is accurate.
– Download Missing Files or Resources: Any reference to missing files or dependencies should be identified and resolved. Update your Maven repository if needed.
– Lastly, rerun
maven clean install
.
As Tim Berners-Lee, the inventor of the World Wide Web, stated, “We need diversity of thought in the world to face new challenges.”
Delve deeper into problems like this, examine what worked and didn’t work, learn so you can anticipate similar issues in the future, diversify your knowledge and continuously improve as a developer. Remember, coding isn’t just about solutions but also understanding the intricacies of problems.
Resolving Issues with Failed Execution of Org.Apache.Maven.Pluginsmaven-Resources-Plugin3.2.0Resources
Resolving issues with ‘Maven Clean Install Failed to Execute Goal Org.Apache.Maven.Pluginsmaven-Resources-Plugin3.2.0Resources’ predominantly pertains to the Maven resources plugin. This plugin helps in copying the project resources to the output directory and is typically used during the process of building a software project.
Understanding the Issue
Maven’s resource plugin issue generally arises when there are missing dependencies, incorrect Project Object Model (POM) settings or environment variables, corrupted repositories, or incompatible Maven versions.
Troubleshooting Steps
The following steps can assist in resolving these issues:
-
<dependencies>
tags in your pom.xml file should be checked rigorously for any errors. Incorrect or missing dependencies can lead to the failure of the Maven execution goal. Correcting these inaccuracies can help avoid this issue.
- Checking and clearing the local repository can resolve many compilation problems. Sometimes the local repository may get corrupted causing the build to fail. You can either manually delete the affected files from the ‘.m2’ directory or use
mvn dependency:purge-local-repository
, allowing Maven to re-download them.
- Running a Maven command with the ‘-X’ flag which gives complete output detail will help. For instance,
mvn clean install -X
. This debug flag assists you in pinpointing specific issues, providing actionable information leading to resolution.
- Checking the compatibility of the Maven version with respect to the ‘Resources Plugin’ is crucial. Make sure that the installed Maven version is compatible with this plugin to prevent any problematic outcomes.
Example Code Snippet
The below sample gives us an insight into how a typical POM configuration might look when using this specific plugin.
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-resources-plugin</artifactId> <version>3.2.0</version> </plugin> </plugins> </build>
As Donald Knuth, known as the “father of the analysis of algorithms,” once said: “If you optimize everything, you will always be unhappy.” Therefore, while trying to fix this issue, it’s essential to view it not as a barrier but as an opportunity to get your hands deeper into Maven, debug issues, and thus grow as a seasoned coder.
Strategies for Successful Maven Resource Plugin Execution
For the successful execution of Maven Resource Plugin, specifically in scenarios where Maven Clean Install fails to execute the goal org.apache.maven.plugins:maven-resources-plugin:3.2.0:resources, follow the below strategies:
1. Ensure Correct Version: Validate that Maven and Java are properly installed and their versions are compatible with each other. You should choose an appropriate version for your Maven Resources plugin. For example, in order to use the maven-resources-plugin:3.2.0, the minimum requirements are Maven 3.0 and JDK 1.7. Check it by
mvn -v
and
java -version
.
2. Build Lifecycle: In Maven, a ‘clean install’ command performs a series of sequential build lifecycle phases including clean, validate, compile, test, package, verify, install. Each phase represents a stage in the life cycle. The Maven Resources Plugin, by default, binds some of its goals to these lifecycle phases.
3. Project Dependencies: Take care of any interdependent projects. Any changes in one project can break another project’s build process if it depends on the first.
4. Plugin Configuration: Use explicit configurations within the pom.xml file to instruct Maven how to handle your resources.
<project> ... <build> <resources> <resource> <directory>[your path]</directory> ... </resource> </resources> </build> ... </project>
5. Checking Paths: Incorrect paths cause resource-related build failures. Always provide the correct path where the resources are located. Typically, Maven follows its standard directory layout, `/src/main/resources` for resources.
6. Update Snapshot Dependencies: Maven takes a snapshot of dependencies at a certain point in time, implying that dynamic changes in dependencies might not be captured. Running mvn clean install -U updates snapshot dependencies, where ‘-U’ forces update snapshots.
Remember Bill Gates’ words, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency”. Similarly, automating your build process using tools like Maven needs following efficient practices, up-to-date knowledge, and understanding of possible issues.
For more details, consult the [Maven Resources Plugin Documentation] and [Introduction to the Build Lifecycle] on Maven’s official site.
Troubleshooting Guide: Overcoming Apache Maven Plugin Failures
Anyone who’s been involved in java development must have faced the troubles related to Apache Maven plugin failures at some point. To be precise, one of the critical issues is when “Maven Clean Install Fails To Execute Goal Org.Apache.Maven.Plugins:maven-resources-plugin:3.2.0:resources.” When such errors occur, the entire development process may halt, leading to project delays.
Let’s visualize how to overcome such issues with a practical step-by-step guide:
- Resolve Java Path:
- Check if the JAVA_HOME path is correctly set in your system environment variables and it points to the correct JDK installation directory. If not, correct it. Use this command to confirm that path is pointing rightly:
echo %JAVA_HOME%
.
- Ensure Maven Version Compatibility:
- Some versions of plugins may not be compatible with the used version of Maven. Check which version of Maven you are using with the command
mvn -version
and ensure that the maven-resources-plugin version is compatible.
- Check POM.xml:
- Inspect your project’s POM.xml file for any errors or discrepancies. Be sure to check whether all necessary dependencies and plugins are included accurately. Also, confirm if maven-resources-plugin is defined properly.
- Maven Repository Issue:
- Sometimes, the Maven repository could have incorrect or corrupted files which cause such errors. Try deleting the entire .m2/repository folder from your user directory and rerun the Maven build (
mvn clean install
) command. This will download all the required resources again and might rectify the failed execution error.
- Network Problem:
- If your network has firewalls or proxies that block access to certain sites, they could impede normal functioning of Maven as well. You’d need to configure them properly in settings.xml file presented in .m2 directory.
Sometimes an older or outdated IDE version may also lead to such issues. In that case, upgrading to the latest stable version might help. Also try executing Maven commands from different command line interfaces (like Git Bash, CMD, etc.) for debugging purposes.
Irving Wladawsky-Berger, a technology thought leader, once quoted, “New technologies often become encrusted with elements of the past.” This implies that even though new tools and technologies evolve, they continue to bear traces of issues or imperfections carried from their earlier versions. So, resolving software glitches like these is a part of the overall development process that one must adeptly handle.
For more detailed understanding of Maven and its pitfalls, refer to the official Apache Maven documentation.
Attempts to run Maven Clean Install can sometimes result in failure due to the inability of executing Org.Apache.Maven.Plugins resources. This particular error message signifies that Maven could not perform its task regarding the resources plugin3.2.0Resources. Errors like these often arise due to a mix-up in dependencies, plugin settings or Java version compatibility issues.
Potential Cause: Dependency Conflicts
The first possibility is related to conflicting dependencies in your pom.xml file. Dependencies from different sources may compete for overriding similar attributes which eventually leads to this error.
Disclosing all the dependencies present on your project can help to pinpoint any conflicts, alleviating the problem.
Possible Solution: Plugin Configuration
Another approach would be to look into the configuration of your plugins in the pom.xml file. The resources plugin should be set up correctly with the right version and required parameters. If not done right, it might trigger this error. Carefully scrutinize the setup of your
resources
plugin, ensuring it’s well-configured:
Third Scenario: Java Version Compatibility
Incompatibility with your Java version could also cause differences in your Maven clean install. Maven has specific compatible versions for executions which need to be upheld. A solution to this would involve:
– Checking your currently used Java version with
java -version
– Comparing this version with the one required by Maven
– If incompatible, you can switch to the matching Java version to troubleshoot the issue
Maven Resources Plugin Documentation
To effectively troubleshoot these issues, I recommend a visit to the official Apache Maven Resource Plugin documentation. This includes complete details about the usage, goals, features, and parameters of the resource plugin.
“Maven is to broadcasting what the Dewey decimal system was to libraries.” – James Governor, RedMonk co-founder