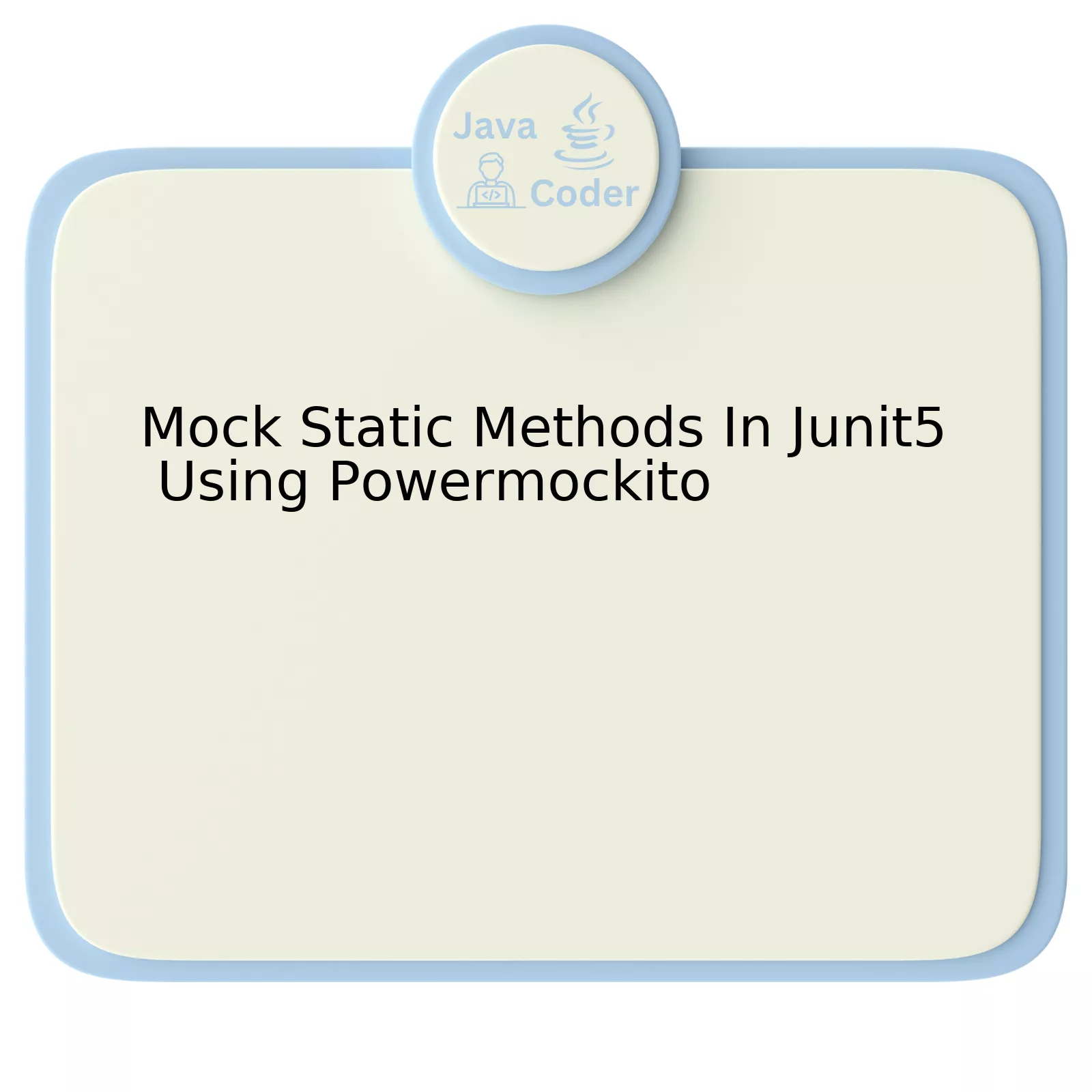
Feature | Description |
---|---|
Mocking Java code | Simulates the behavior of real objects complex enough to be impossible (or infeasible) to directly incorporate into tests. |
PowerMockito | A PowerMock’s sub-project that provides additional capabilities to Mockito, such as mocking static and private methods. |
Junit5 | Latest version of the JUnit testing framework for Java. Supports modern Java language features and integrates with various tool ecosystems. |
Mockito API | Helps in creating, verifying, stubbing, and coping with mocks and spies. |
Static methods | Belong to the class, not an instance of it, and thus can’t be overridden like instance methods, challenging standard mocking techniques. |
Mocking is a crucial aspect of unit testing in Java. When writing unit tests, our objective is to focus on the functionality of the unit. However, many times this unit has dependencies which make testing difficult. This is where the concept of Mocking comes in and allows us to replicate these dependencies and their behaviour for the purpose of unit testing.
PowerMockito, a powerful, flexible Java mocking framework, extends the popular Mockito to add even more functionalities, one of them being the ability to mock static methods. Given that static methods belong to the class rather than an instance, they are usually considered unmockable as they cannot be overridden. Thus, PowerMockito’s support for this feature is impressive.
JUnit5, the latest iteration of the widely-used JUnit testing framework, offers several new features, including Modularity, Flexibility, and extensibility. Combining PowerMockito with JUnit5 thus provides comprehensive support mask behaviours during test scenarios.
Consider a scenario where there’s a utility class with static methods. When unit testing a class that uses this utility class, we might want to control the output of these static methods. Suppose our utility class looks something like this:
public class UtilityClass { public static int performComplexCalculation(int input){ //complex operation here. } }
Without PowerMockito, we would have no control over the “performComplexCalculation” method’s output. But, using PowerMockito, we could mock it like so:
@PrepareForTest(UtilityClass.class) public class OurTestClass{ @Test public void ourTestMethod(){ PowerMockito.mockStatic(UtilityClass.class); when(UtilityClass.performComplexCalculation(anyInt())).thenReturn(5); ... } }
As Bill Gates, Co-founder of Microsoft, once said: “The first rule of any technology used in business is that automation applied to an efficient operation will magnify the efficiency”. The fact that we can automate the process of replacing complex dependencies with simplified, controllable versions encapsulates this statement. Instead of having to manually create complicated constructs to simulate our dependencies’ behavior, a powerful tool like PowerMockito can do the job for us — making the whole testing process that much more efficient.
“Understanding the Role of Static Methods in Junit5”
Static methods in Java have often been considered a roadblock when it comes to unit testing, due to their global state; this is precisely where JUnit5 and PowerMockito brings the solution on the table.
Role of Static Methods in JUnit5
In JUnit5, the role of static methods is pivotal. Static methods aid in writing and organizing test cases by providing assertions (
Assertions.assertXyz()
) and assumptions (
Assumptions.assumeTrue()
). They’re also valuable for utility functions or constant variables that can be used across various test cases without having to re-instantiate objects each time.
Assert Method | Description |
---|---|
assertEquals(expected, actual) |
This asserts that two values are equal. |
assertNotNull(actual) |
This asserts that a given object is not null. |
assertTrue(condition) |
This asserts that a given condition holds true. |
Mocking Static Methods with PowerMockito
This is where JUnit5 connects with PowerMockito. PowerMockito allows you to cope with daunting test scenarios such as mocking static methods.
For example, if you had a Utility class with a static method:
public class Utility { public static String uniqueName(String name) { // Generating a unique name logic } }
In your Unit test, you’d use PowerMockito to mock this in the following manner:
@RunWith(PowerMockRunner.class) @PrepareForTest(Utility.class) public class UtilityTest { @Test public void testUniqueName() { PowerMockito.mockStatic(Utility.class); Mockito.when(Utility.uniqueName("Testing")).thenReturn("MockedName"); // ... } }
Here, we’ve mocked the static method `uniqueName` to return a fixed string “MockedName” whenever it’s called within this test case, thereby eliminating dependencies on the real method implementation.
Irrespective of the complexities brought about by static methods in Java, JUnit5 together with PowerMockito offer potent solutions to effectively tame and test these beasts. As Robert C. Martin once said, “The only way to go fast is to go well!“, and efficiently testing your code using such tools ensures just that.
Find more about PowerMockito and its capabilities at PowerMock Documentation. Keep refining your knowledge and skills, for that’s how you become an extraordinary developer amidst the ordinary.
“Diving Deeper into Powermockito Features for Testing”
Testing in Java is an essential part of software development and using tools like PowerMockito makes it easier, especially when dealing with static methods. Traditionally, static methods are considered unmockable and are difficult to handle in a testing environment. However, PowerMockito offers methods for mocking these incalcitrant methods.
In the recent version of JUnit5, Mockito 3.4.0 has introduced the new `mockStatic()` method which can be used for mocking static methods in the classes under test. Unfortunately, native support of Powermock and Mockito is not yet available for JUnit5. In such situations, PowerMockito would become very handy, using its own copy of the bytecode manipulation library.
To illustrate this usage, let’s consider a utility class
UtilityClass
containing a static method
performAction()
.
public class UtilityClass { public static String performAction(String input) { return "Result " + input; } }
To mock this
performAction()
method using PowerMockito in a JUnit5 environment, you will have to:
1. Annotate your test class with
@ExtendWith(PowerMockExtension.class)
, to enable PowerMock support for JUnit5.
2. Use
@PrepareForTest(UtilityClass.class)
to prepare the static method class for testing.
3. Inside your test method, use
PowerMockito.mockStatic(UtilityClass.class)
to initiate static method mocking.
4. Utilize
PowerMockito.when()
or
PowerMockito.doReturn()
to provide desired behavior to the mocked static method.
@ExtendWith(PowerMockExtension.class) @PrepareForTest(ConverterUtils.class) public class YourTest { @Test public void testMethod() { PowerMockito.mockStatic(UtilityClass.class); PowerMockito.when(UtilityClass.performAction("input")).thenReturn("Mocked Result"); // ... rest of your test logic } }
In this example, “Mocked Result” will be returned when calling
UtilityClass.performAction("input")
. Here, PowerMockito provides all the features you need to effectively execute unit tests on your Java code containing unmockable codes, such as static methods.
“The act of describing a program in unambiguous and ordered detail.” – Bill Gates. Even though Bill Gates, Microsoft Co-Founder is talking about programming in general, his quote indirectly emphasizes on the importance of unit testing. The idea of ordered and detailed description aligns with the essence of unit testing, which assures the expected behaviors are occurring at atomic level of the software.
“Incorporating Mock Static Methods with Junit5 and Powermockito”
Mock static methods are integral parts of unit testing in Java. These methods allow developers to isolate units of code for more robust testing. JUnit5, a widely-used testing framework in Java, however, lacks the ability to mock static methods by default. This is where PowerMockito comes into play. As a powerful framework, PowerMockito extends Mockito’s capabilities and allows for the mocking of static methods.
To incorporate the use of PowerMockito with JUnit5 for mocking static methods, you need to follow these steps:
Step 1: Add the necessary dependencies
Import necessary dependencies in your Maven’s pom.xml file. Including PowerMockito and Junit5 in your test dependency could look something like below:
<dependency> <groupId>org.powermock</groupId> <artifactId>powermock-module-junit4-rule-agent</artifactId> <version>{latest.version}</version> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-engine</artifactId> <version>{latest.version}</version> <scope>test</scope> </dependency>
Remember to replace `{latest.version}` with the most recent stable versions of these dependencies available at the time of writing the code.
Step 2: Mock the Static Method
Now let’s see how to use PowerMockito to mock a static method while using Junit5.
import org.junit.jupiter.api.Test; import org.powermock.reflect.Whitebox; public class MyTestClass { @Test public void testStaticMethod() { // Mock static method PowerMockito.mockStatic(MyClass.class); // Specify behavior Mockito.when(MyClass.myStaticMethod()).thenReturn("mock return value"); // In Test Assert.assertEquals("mock return value", MyClass.myStaticMethod()); } }
The above written test mimics the behavior of the static method `myStaticMethod` from class `MyClass`.
Step 3: Run Your Tests
You can now run your tests as you would do normally. The JUnit5 runner will take care of executing your test class.
It’s important to note that static methods should be used sparingly as they make code harder to test. They create hidden dependencies and mutating global state can cause race conditions in multi-threaded contexts. Martin Fowler once said, “static methods are death to testability”.
“Tips and Techniques: Ensuring Effective Use of Powermockito in Junit5”
PowerMockito allows us to extend Mockito functionality, most importantly giving the ability to mock static methods, which is crucial when unit testing some Java applications. With JUnit 5, it’s crucial to ensure that PowerMockito is used effectively for optimal testing. The main focus here will be on mocking static methods in JUnit5 using PowerMockito.
Why Mock Static Methods?
Static methods are known for being challenging to test primarily because they belong exclusively to class type rather than an instance of a class. In scenarios where these methods have side-effects or interact with external systems, isolating them for unit testing can be difficult. That’s where PowerMockito comes into play, allowing us to mock these static methods and increase our code’s testability.
Using PowerMockito to Mock Static Methods
Consider that your project has a Utility class with a static method, `fetchData()`, which you would like to mock. Here’s how you do it:
@PrepareForTest({Utility.class}) public class ExampleTest { @Test public void testFetchData() throws Exception { PowerMockito.mockStatic(Utility.class); PowerMockito.when(Utility.fetchData()).thenReturn("Mock data"); String responseData = Utility.fetchData(); assertEquals("Mock data", responseData); } }
In this example, we import `@PrepareForTest` annotation from PowerMockito, specifying the class containing the static method. Then, in the `testFetchData()` method, we mock the Utility class, specifying the behavior using `when(…).thenReturn(…)` syntax and then confirm that it works as expected.
It’s important here to note that, “Code is written only once but read many times” -Anonymous, thus readability of tests is crucially important.
Ensure Effective Use of PowerMockito
Here are a few pointers to get the most out of PowerMockito:
– Isolate Static Methods: If feasible, restructure your application so that static methods are isolated into their utility classes. This makes the static methods easier to manage and allows you to leverage PowerMockito to its maximum potential.
– Favor Real Instances Over Mocks: While PowerMockito is powerful, avoid overusing it. If you find yourself having to use PowerMockito frequently, it could be a sign that your design is not very test-friendly. Favor real instances over mocks where possible to increase the strength of your tests.
– Limit Mocking Static Methods: Remember, every time you’re mocking a static method, you potentially introduce a point of failure in your test. Therefore, it should be limited to where it’s absolutely necessary.
For more detailed insights into PowerMockito functionalities, please refer to this guide: PowerMockito Wiki Mockito.
Using PowerMockito in line with these guidelines within JUnit 5 setting will ensure effective unit testing while handling static methods.
As a conclusion, we delve into the vast world of automated testing in Java with a focus on leveraging PowerMockito for mocking static methods in JUnit 5. To ameliorate the dangling complexities tied to unit testing, the feature provided by PowerMockito for mocking static methods stands as an essential tool that exponentially simplifies the task.
Java | Junit5 | Powermockito | Static Methods |
Object-Oriented Programming language | A powerful testing framework | A Mockito-based API | Methods belonging to a class, not an instance |
To illustrate a practical approach, suppose we have a utility class comprising various static methods. To create unit tests and isolate functionalities, we need to mock these static methods. PowerMockito fulfills this requirement in a very effective way.
import org.junit.jupiter.api.Test; import org.powermock.api.mockito.PowerMockito; import java.util.Calendar; public class TimeUtilsTest { @Test public void testGetCurrentTime() { PowerMockito.mockStatic(Calendar.class); //Define what should be the response of mocked method Calendar mockedCurrentTime = new Calendar.Builder().build(); PowerMockito.when(Calendar.getInstance()).thenReturn(mockedCurrentTime); //Rest of the test case } }
The above code gives a glimpse of handling static methods for scenarios where outcomes of other static methods influence results under scrutiny. Considering Martin Fowler’s words on unit testing, “Whenever you are tempted to type something into a print statement during testing or debugging, write it as a test case instead,” one can appreciate the value of testing; particularly tasks performed with frameworks like Junit5 and APIs like PowerMockito.
The implementation of mocking static methods using PowerMockito with Junit5 amplifies two significant aspects: It augments the capability of Junit5 and makes testing easier and robust while also adding onto Powermockito’s reputation as a powerful unit testing tool.
For further insight on the topic, consider visiting Junit official page or PowerMockito Wiki Page. These resources offer in-depth knowledge and numerous examples for beginners and experienced developers alike.