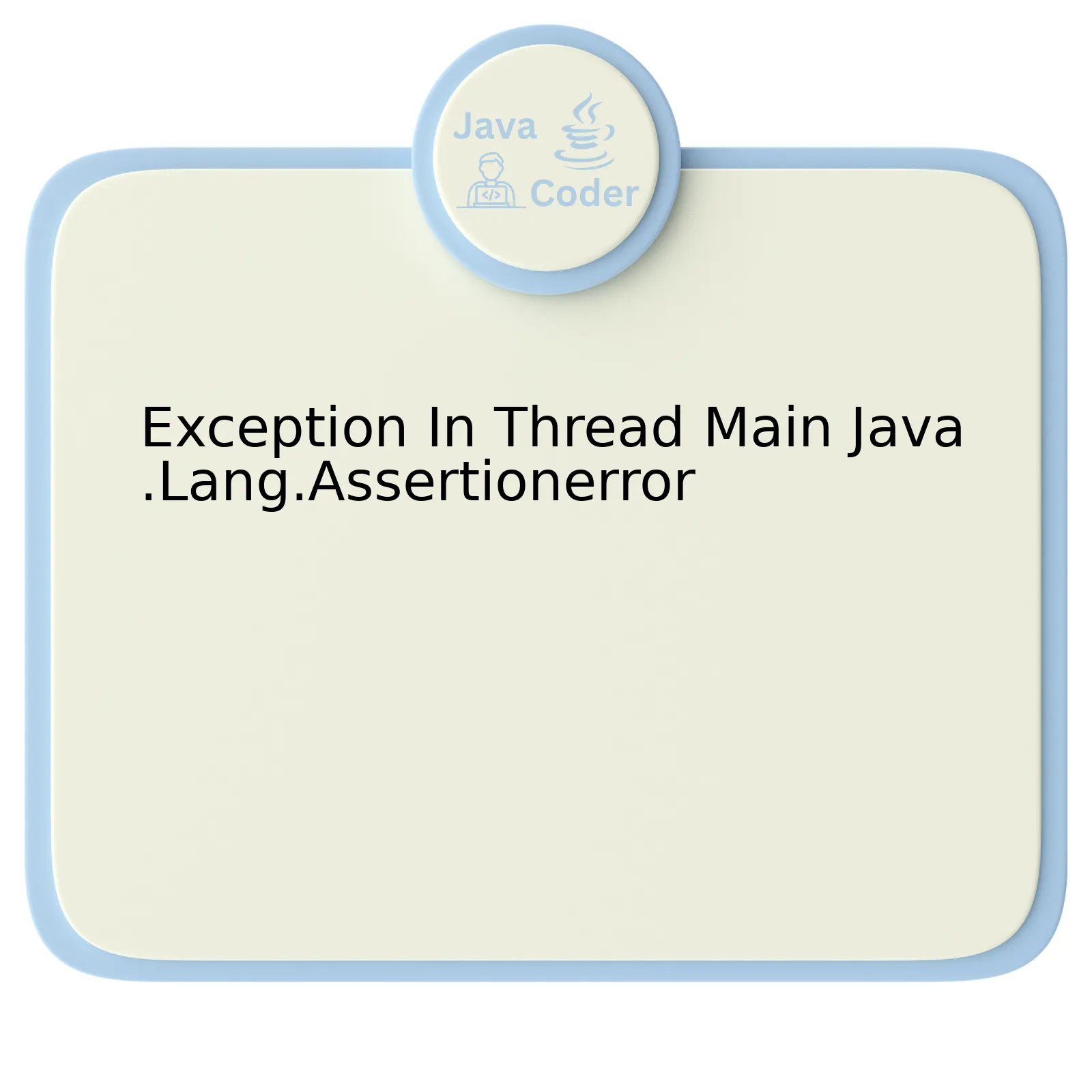
Understanding the occurrence of “Exception In Thread Main Java.Lang.Assertionerror” in Java requires an understanding of some key constructs involved, namely Exception Handling, Thread, and Assertions.
Java Construct | Description |
---|---|
Exception | An Exception is an event that disrupts the normal flow of program instructions. They are conditions that occur during the execution of a program and disrupt its normal flow. Java offers robust exception handling structures like try, catch and finally blocks to handle these abnormal situations. |
Thread | A Thread specifies the independent path of execution within a program. The part where we encounter “main” refers to the main thread, which initiates the sequence of method calls that begins the whole execution cycle. |
Assertion | Assertions are statements used to test assumptions made by programmers. They throw AssertionError if the condition stated in the assertion statement does not hold true. AssertionError extends the Error class, which defines serious errors that should not be captured and handled within the application. Therefore, when it comes to Java.Lang.AssertionError, this means that an assertion statement has failed, meaning a false statement was encountered during program execution. |
According to Oracle’s Java official documentation, “An application should throw instances of this class to indicate that other classes will forever violate class invariants.” Consequently, receiving “Exception In Thread Main Java.Lang.Assertionerror” signifies that the programmer’s hypothesis about their code isn’t correct or isn’t being followed as expected when running the program.
Enable assertions using `-ea` option at runtime, allows your program to test assertion statements. Here’s an example:
java
public class Main {
public static void main(String[] args) {
int value = 15;
assert value >= 20 : ” Underweight”;
System.out.println(“Value is “+value);
}
}
If you run this code snippet without enabling assertions, your program will ignore the `assert` statement. However, if you enable assertions and the condition in `assert` statement fails, this results in an AssertionError, throwing “Exception In Thread: Main java.lang.AssertionError: Underweight”.
As James Gosling (creator of Java programming language) once remarked: “A good programmer builds a tight and sleek ship with zero bugs, ready for anything. Other times you just have to adapt and make it work.” This underscores the importance of leveraging Java’s robust exception handling capabilities and error signaling via assertions to build resilient and efficient applications.
Understanding the Exception in Thread Main Java.Lang.Assertionerror
An
Exception in Thread "main" java.lang.AssertionError
is a significant occurrence for any Java developer. It generally arises when a particular assertion fails – in other words, when an assert statement evaluates to false. Assertions are typically used as a debugging tool. Their role is to verify the correctness of any invariant in the code.
Let’s dive further into understanding this exception and how we can handle it effectively in our Java programs. But first, let’s get a more definitive understanding of what an AssertionError is within the context of Java.
AssertionError in Java
In Java, AssertionError is derived from the Error class, which is facilitated by the Java Virtual Machine (JVM). Primarily, programmers use assertions in their code to verify assumptions they’ve made. In Java’s official documentation, it has been suggested that developers should not use AssertionError for catching purposes as it indicates serious programming issues.
A simple demonstration of assertions in Java:
// A simple example of assert keyword in Java class AssertDemo { public static void main(String args[]) { int value = 15; assert(value >= 20) : " Underweight"; System.out.println("The value is "+value); } }
In this instance, if the condition evaluates to be false, AssertionError will be thrown with a message “Underweight.”
Understanding Exception in Thread Main java.lang.AssertionError
This specific error “Exception in Thread Main java.lang.AssertionError” tells us that an assertion error is thrown within the main thread of execution. The JVM handles the error because your program violates an asserted condition set within the code.
It essentially communicates two primary but interconnected elements:
1. The assertion has failed or, in simpler terms, a boolean condition presented in an assertion statement turned out to be false.
2. This condition of failure within the primary or main thread executing the Java application commands the appearance of the AssertionError.
Validating your codes’ assumptions via the assert keyword is a substantial component in reducing software bugs. However, it raises the likelihood of facing the “Exception in Thread Main java.lang.AssertionError.” Nonetheless, it effectively pinpoints areas in your code where your assumption related to a particular condition was false.
In light of “Grace Hopper”, who is well-known for her contributions in shaping the early years of computer programming, she once had said, “To me programming is more than an important practical art. It is also a gigantic undertaking in the foundations of knowledge.”
Conclusively, extending accurate and efficient debugging practices are embedded in knowing and rectifying such exceptions.
Decoding Causes of Exception in Thread Main Java.Lang.Assertionerror
The exceptional condition denoted by
Exception in thread main java.lang.Assertionerror
is a somewhat common issue that arises during Java programming, especially when unit testing with JUnit. It primarily signifies that a certain statement’s truth value which was assumed to be ‘true’ ended up being ‘false’. Despite its intricacy, the causes behind this kind of exception can be decoded efficiently when given ample attention.
Let’s explore the major underpinning factors:
Error in Assertion Statement
An assertion is a statement that you staunchly believe to be true at the time it is executed. In Java, you use the keyword
assert
followed by a Boolean expression to state an assertion. If the system evaluates this expression as false, an AssertionError is triggered. Ensuring the accuracy of assertion statements allows developers to prevent this exception.
Example:
int x = 10; assert (x > 11): "the value of x is less than or equal to 11";
In the above scenario, since x is not greater than 11, the assertion will fail, causing an AssertionError.
JUnit Test Failure
It’s common to encounter
java.lang.AssertionError
during unit testing using JUnit, especially when a test fails. JUnit uses assertions to signify that the tested code didn’t exhibit the anticipated behavior.
Example:
@Test public void testAddition() { MyClass tester = new MyClass(); assertEqual("Result", 15, tester.add(10, 5)); }
If the method doesn’t return 15 when adding 10 and 5, the assertEqual assertion would fail, triggering an AssertionError.
Negligence of Assertion Activation
One thing worth mentioning is that assertions in Java are inactive by default. This implies that you must activate them using
-ea
in the JVM command line option. Failing this, even if an assertion is false, it won’t cause an AssertionError, leading to confusion and potential misinterpretation of the code flow.
To illustrate it succinctly, Bill Gates’s famous assertion about coding psychology is apt here: “Measuring programming progress by lines of code is like measuring aircraft building progress by weight”. In the same vein, understanding the occurrence of
Exception in thread main java.lang.Assertionerror
relies on awareness and comprehension of the responsible factors rather than a reflexive response to errors. Take time to understand your assertions, align them properly with program essentials and conduct thorough unit testing for smooth execution and fewer surprises. Here is a helpful resource on Java Assertions.
Preventive Measures to Avoid Exception in Thread Main Java.Lang.Assertionerror
The
Exception In Thread Main Java.Lang.Assertionerror
is an error often encountered in Java application development, typically indicative of a situation where an assertion statement evaluates to false. To counter this issue in your programming environment it is essential to employ preventive measures aimed at preserving the logical correctness and ensuring efficient execution of your code.
These tactics encompass the following key facets:
1. Thorough Code Review:
Core aspect of prevention lies on the accuracy of reviewing your own code. This involves analyzing each section of the program to ensure that all Java assertions hold true throughout the span of code execution.
Example:
public static void main(String[] args) { int value = 10; assert value > 10 : "Assertion Error: Value is not greater than 10"; }
This code snippet is example of an assertion that would fail, as value is not greater than 10. Externalizing the assertions to a separate method, or wrapping them inside conditional if-else statements can provide clearer control over how they are handled.
2. Utilization of Exception Handling Mechanisms:
Leveraging the power of exception handling mechanisms provided within the Java language can offer significant preventive capabilities against errors like java.lang.AssertionError. Consider encapsulating risky code parts within try-catch blocks to handle potential exceptions directly.
*Regular Software Testing:*
Regular execution of both unit tests and integration tests can provide insights into bugs and potential AssertionError triggers in your code. Adequate provision of testing ensures that your code doesn’t ship with situations leading to assertion failures, capturing such issues before deployment and during coding process.
3. Incorporating Third-Party Bug Detection Tools:
Tools such as PMD, FindBugs or Checkstyle can scrutinize your codebase for potential risks and errors that might lead to failed assertions.
4. Continuous Integration/Continuous Deployment (CI/CD):
A CI/CD pipeline promotes regular testing and instant feedback on errors through automated tools. This helps developers to rapidly identify and rectify any issues that could lead to AssertionError.
A balanced integration of these strategies provides a bulwark against `java.lang.AssertionError`, protecting your code from potential logical inconsistencies and assuring its robustness against runtime exceptions.
As the esteemed software engineer Kent Beck once said, “First make the change easy (warning: this might be hard), then make the easy change”. Implementing preventive measures might seem cumbersome initially, but their benefits for code quality and robustness prove worthwhile in the long haul.
Rectifying Strategies for Exception in Thread Main Java.Lang.Assertionerror
Exception In Thread Main Java.Lang.Assertionerror occurs in a Java program when there is an assertion exception that hasn’t been handled in the main thread. This implies an error during runtime and it can significantly hinder the functionality of your application. We will discuss some rectifying strategies, each strategy will be substantiated with a coding example to make comprehension effortless.
Assertion Error Description:
This error represents a failed
assert
statement. Assertions are boolean expressions which devs employ during the development process to verify unchanging conditions. If the condition doesn’t hold, a java.lang.AssertionError is automatically thrown.
For instance, consider this block of code:
// Assuming variable x should always be less than 10 assert x<10 : "x should be less than 10";
If the value of x is say, 15, this would lead to Assertion Error as the condition was not met.
Rectifying Strategies:
1. Utilizing Try-Catch:
One method to handle exceptions is wrapping the assert code block within a try-catch clause. By doing so, you’ll ensure that when an AssertionError is thrown, it is caught immediately, allowing the program to continue functioning despite the error.
The corresponding code snippet:
try { assert x<10: "Error Assertion"; } catch (AssertionError e) { e.printStackTrace(); }
2. JVM Arguments:
The other approach involves using JVM arguments. You could potentially disable assertions on a total or partial scope, depending on necessity.
You could use `-ea` or `-enableassertions` to enable assertions at runtime. For disabling, `-da` or `-disableassertions` is used instead. For instance:
java -ea com.example.ExampleClass
3. Fulfilling Assertion Conditions:
Generally, it’s best to see what condition is being checked for within the assert statement and then ensure that said condition is met while executing the program.
Consider the previous code snippet, where x is 15. To avoid an AssertionError, you should see that the condition x<10 is satisfied by ensuring the value of x truly is less than 10.
As Bill Gates astutely mentioned “I choose a lazy person to do a hard job because a lazy person will find an easy way to do it.” instead of suppressing the errors or catching them we could simply work towards fulfilling the necessary conditions.
By judiciously implementing these rectifying strategies, you can efficiently deal with Exception In Thread Main Java.Lang.Assertionerror, resulting in a robust and successfully running Java program.
While addressing the issue of “Exception In Thread Main Java.Lang.Assertionerror,” it’s highly necessary to understand the underlying cause – typically this error appears when an assertion, a statement in Java that enables you to test your assumptions about your program, is found to be false.
During the dynamic execution of a Java program, if an assertion evaluates to false, an instance of AssertionError is automatically thrown. However, an important detail to remember is that assertions are not usually enabled by default; instead, they are activated by the “-ea” command-line option. As such, the non-visibility of the ‘Exception in Thread Main’ could potentially be due to disabled assertions.
public class Main { public static void main(String[] args) { int value = 15; assert value >= 20 : " Underweight"; System.out.println("value is "+value); } }
In the code snippet above, it will show ‘Exception in Thread Main Java.Lang.Assertionerror: Underweight’. If your received value isn’t more or equals to 20 then AssertionError will be thrown and along with the ‘Underweight’ message.
To handle such errors:
– Frequently validate the conditions for all assertions in your code.
– Ensure assertions are enabled during code runs meant for testing. Orrin Hatch once said, “There is a good reason they call these ceremonies ‘commencement exercises’. Graduation is not the end; it’s the beginning.” The same applies to assertive programming. Errors shouldn’t end your debugging process; rather, they provide starting points for digging deeper into your code.
An effective handling of the Exception in Thread Main Java.lang.AssertionError entails understanding the working principles of Assertions and the conditions that activate them.
You can learn more at Oracle’s official Java documentation here.