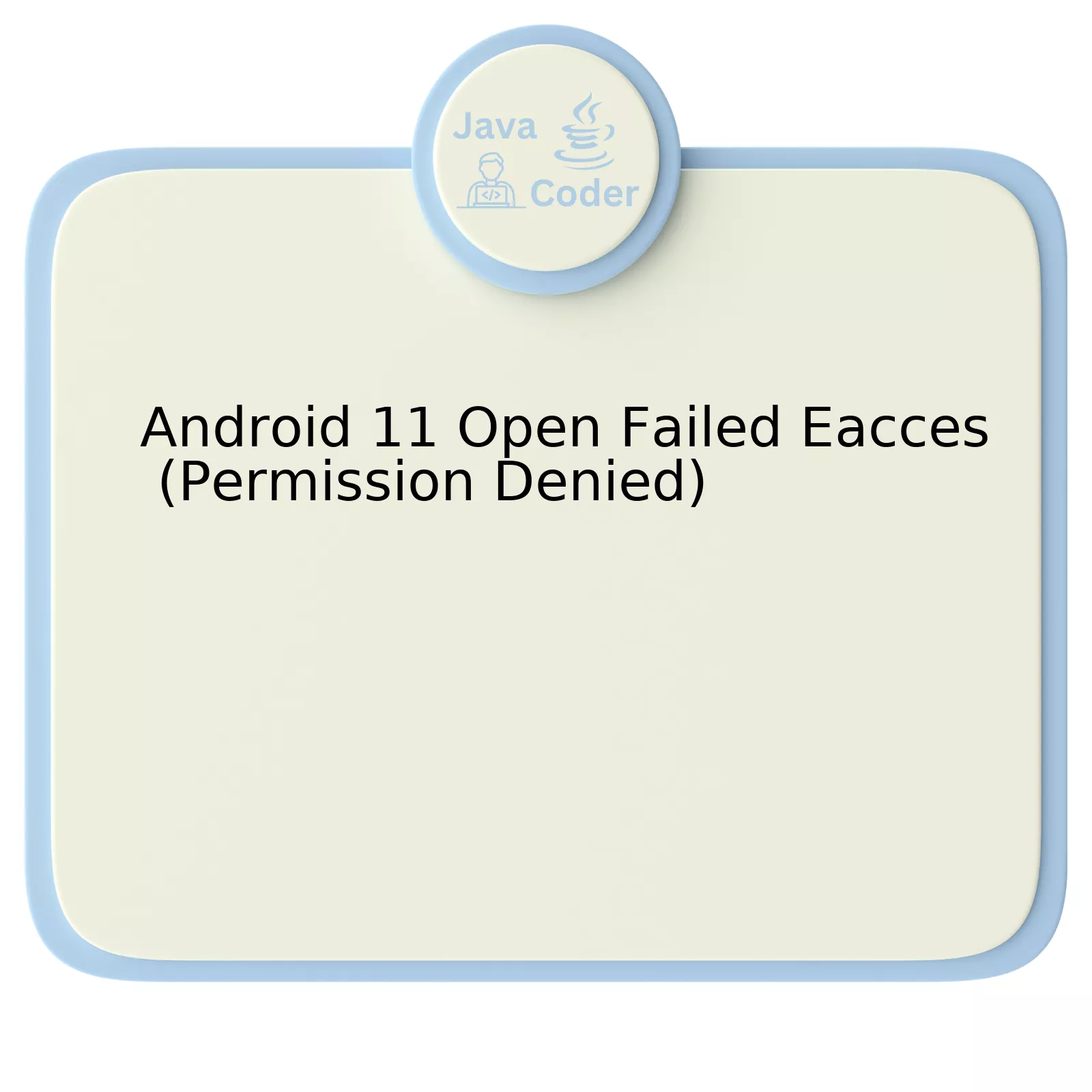
The EACCES (Permission Denied) in Android 11 is a permission issue that is often encountered by developers while trying to access files or directories that are restricted by the Android operating system. Using the following table representation, we can dissect the problem and define solutions:
EACCES Instances | Symptoms | Potential Actions |
---|---|---|
Application attempts to open a file in an external storage directory without necessary permissions. | Receive a “java.io.FileNotFoundException: /storage/emulated/0/myfile.txt: open failed: EACCES (Permission denied)” error message | Ensure all mandatory read/write permissions are included in the AndroidManifest.xml file |
Application tries to access system-restricted files or folders | The application crashes abruptly with no specific indication tracing back to system files | Avoid accessing system-encrypted files/folders if the application doesn’t critically require such actions |
File Uri’s are accessed using the direct file path | The application manages to execute on Android 10 and older versions but fails on Android 11 | Use content:// Uris instead of file:// Uris with intent to align with →scoped storage← rules introduced in Android 11 |
Analyzing this information reveals that Android 11 Open Failed EACCESS (Permission Denied) can be managed by correct planning and mindful coding.
Observing how applications behave while running on Android 11 would help discover places where apps might confront file-access restrictions. As part of these responses, ensure your application requests necessary permissions, especially for files stored on external sources.
For instance, an app manifest may look like:
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
Make sure to avoid any interactions with encrypted system rows. Such action may not only result in an exception but could also raise security issues leading to abrupt termination of the application.
Finally, adhere to the scoped storage requirement as stipulated by Android 11. Abandon the approach of using direct file paths with file:// URI, rather use content:// URIs to share file access between apps or components.
Niklaus Wirth, a renowned computer scientist quoted: “Good programmers do not just write programs. They build an executable model that solves the problems posed by the user.” Keeping that in mind, adapting our code to changes in environment or constraints is an integral part of being a good developer.
Understanding Android 11 Open Failed Eacces: A Deep Dive
An intriguing error that you may have encountered when working with Android 11 is the ‘Open Failed Eacces (Permission Denied)’. This issue arises commonly due to changes to the file access permissions introduced in recent versions of Android, particularly from Android 10 onwards. These updated security policies play a crucial role in preventing unauthorized access to sensitive files and maintaining the sanctity of user privacy.
Under Android’s scoped storage, each app operates within a predefined ‘sandbox’ repository, thereby limiting its ability to freely read or write to the device’s external storage.source
Insights into this problem and potential solutions can be dissected into:
- Requesting Permissions: Prior to accessing either internal or external storage, your application must formally request permission with Manifest.permission.READ_EXTERNAL_STORAGE or Manifest.permission.WRITE_EXTERNAL_STORAGE respectively.
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
- Processing granted permissions: Once relevant permissions are incorporated within your app manifest, you need to handle them from within your code. You could use ActivityCompat APIs to request permissions at runtime:
source
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.READ_EXTERNAL_STORAGE}, MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE);
- Adapting to Scoped Storage principles: From Android 10 (API level 29), apps targeting Android 11 are enforced to utilize ‘Scoped Storage’. Android provides specific directories within app-specific storage where your application can operate without requiring any additional permissions. Using MediaStore API allows your application to add, modify, or delete media files while conforming to Android’s updated access control policies:
source
ContentValues values = new ContentValues(); values.put(MediaStore.Images.Media.DISPLAY_NAME, "MyImage.jpg"); values.put(MediaStore.Images.Media.MIME_TYPE, "image/jpeg"); values.put(MediaStore.Images.Media.RELATIVE_PATH, Environment.DIRECTORY_PICTURES); Uri uri = context.getContentResolver().insert(MediaStore.Images.Media.EXTERNAL_CONTENT_URI, values); OutputStream outputStream = context.getContentResolver().openOutputStream(uri); bitmap.compress(Bitmap.CompressFormat.JPEG, 100, outputStream); outputStream.close();
Admittedly as Ken Thompson, co-creator of UNIX, said: “One of my most productive days was throwing away 1000 lines of code.” Overcoming the Android 11 Open Failed Eacces error may require a radical rethink of how your application interacts with Android’s file system.
Potential Causes of Permission Denied Error in Android 11
The
open failed: EACCES (Permission Denied)
error is an issue that many Android developers face when upgrading to Android 11. The main reason for this issue is due to the new changes implemented in file handling, through a mechanism known as scoped storage.
To understand better, here are crucial factors that bring about this error:
Android 11’s Scoped Storage
Scoped storage was introduced primarily to improve app and user data security by restricting broad access to shared storage. Instead of letting app access files across the whole system, it only allows an application to view files in its own directory or files created explicitly by the same app.
“First rule of coding is: It’s not working because of your assumptions are wrong.” – Thomas Fuchs
Lack of Necessary Permissions
Even when you migrate to scoped storage, you still must ensure that necessary permissions (READ_WRITE_STATIC_FILES) are included and requested from the user correctly within your application’s manifest and runtime.
Incompatible Third-Party Libraries
In some scenarios, certain third-party libraries you are using might interact with the device’s filesystem in ways that they shouldn’t according to Android 11 guidelines.
Incorrect File paths
Another potential cause could be using an incorrect file path in your Java code. Particularly, hardcoded absolute file paths would fail, as they typically don’t conform to Android 11’s new file access regulations.
As a developer, you have different strategies for addressing these issues:
Migrate to Scoped Storage
Consider migrating your application to work with scoped storage early, following Android’s recommended way of manipulating files via MediaStore API. Look into using alternative methods such as openFile() and Uri based solutions instead of using direct file paths.
Sample piece of code:
FileInputStream fis = null; try { fis = getApplicationContext().openFileInput("example.txt"); } catch (FileNotFoundException e) { e.printStackTrace(); }
Handle the necessary permissions important for your app
Ensure to include and request all necessary permissions explicitly, both within your application’s manifest and at runtime. This includes reading and writing static file permissions.
Update to Library Versions that Support Android 11
Be aware that certain third-party libraries might need to be updated, or even replaced if they’re incompatible with Android 11 storage practices. Regularly check for updates for these libraries and apply them promptly.
Always use correct and dynamic file paths
Ensuring file paths used in your Java code are correct and, wherever possible, dynamic instead of hardcoded can help avoid many permission denied errors.
For a more detailed information on handling files on Android 11, refer to Android’s official data storage guide.
Effective Solutions to Address the Open Failed Eacces Issue
Addressing the Open Failed Eaccess (Permission Denied) issue on Android 11 involves troubleshooting techniques that center around granting appropriate permissions and undertaking key code changes. The following section delves into these corrective measures:
1. Code Level Permissions
In Android applications, two types of permissions are utilized: Normal and Dangerous. Open File Eaccess denial is a typical oversight observed when an app tries to perform an action that infringes upon these permissions. As a result, ensuring that your Android application has the necessary permissions in the Manifest file can resolve this issue.
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
Do remember the two permissions above have been made more stringent from Android 6.0 onward due to privacy concerns. Hence, it’s imperative that you verify and demand these permissions at runtime as well.
2. Request Permissions at Runtime
Beginning with Android 6.0, permissions should be requested at runtime, which means permission requires to be granted by the user while using the app, particularly for ‘Dangerous Permissions.’ A conventional way to request these permissions includes a method, as shown in the snippet below:
ActivityCompat.requestPermissions(thisActivity,new String[]{Manifest.permission.READ_EXTERNAL_STORAGE}, MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE);
Subsequently, for each permission that your app requests, add a \
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" android:maxSdkVersion="28" /> tools:ignore="ScopedStorage"> <request-permissions> //permissions list </request-permissions> </uses-permission>
3. Utilize alternative APIs
For Android 11 specifically, apps are encouraged to use alternative APIs for file access including MediaStore (for media files), Storage Access Framework, and the new Scoped Directory Access. These modules provide more targeted ways to access media, documents, and other files. Replace old file operations with the recommended methods.
Remember that Restrictions apply to apps targeting Android 11 (API level 30) and affects different types of files including Images, Videos, and Audio.
As stated by Sir Tim Berners-Lee, “We need diversity of thought in the world to face the new challenges,” the same goes in coding and development, where the innate ability to adapt to changing standards and practices often holds the key to effective problem-solving.
Impacts and Avoidance Strategies for Android 11 Permission Denied Problem
The Android 11 Permission Denied problem, often manifesting as
Open Failed Eacces (Permission Denied)
, presents challenges to both developers and users. The impact of this issue is significant as it can disrupt app functionality, creating barriers in user experience and leading to decreased user satisfaction.
This issue primarily arises from the new scoped storage model launched with Android 11. These changes majorly impact how apps access files outside of their own app-specific directories. Favorably, Android provides explicit user control over files and prevents apps from accessing unnecessary data. However, the adoption of scoped storage resulted in compatibility issues for some apps.
Potential Impacts:
- App Functionality Issues: Without proper permission granted, attempts to read or write an external file could result in the
Open Failed Eacces (Permission Denied)
error.
- Negative User Experience: Frequent appearances of error messages might frustrate users, impacting the overall user experience.
- Safety Concerns: As per Google’s privacy policy[1], if an app violates permissions guidelines or misuses them, its update, or even listing, could be suspended from Google Play Store.
Avoidance Strategies:
To address these challenges, developers can adopt several strategies:
- Request Permissions at Runtime: This could be done using
requestPermissions()
method which allows apps to request necessary permissions when needed.
- Use Scoped Directory Access: You can avoid the need for broad storage permissions by using alternative APIs that provide more specific directory access.
- Test on Android 11: Test your app under different scenarios to ensure it operates effectively within the new permission constraints of Android 11.
- Optimize for Existing Platforms: For older apps targeting API level 29 and lower, you can use the
requestLegacyExternalStorage
flag to maintain broad file access capabilities.
As Martin Fowler once said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” While adapting to changing conditions like Android 11’s permissions may seem challenging, it ultimately helps create cleaner, safer, and more user-friendly apps. Always remember to keep the permission requests minimal and clear to the users, significantly enhancing user trust and app experience.
Analyzing the issue of “Android 11 Open Failed Eacces (Permission Denied)”, it can be deduced that the main problem lies in the enforcement of new security measures introduced with Android 11. Reports suggest that applications, particularly those using the ‘android:requestLegacyExternalStorage’ attribute, are unable to access certain files and directories on the device, resulting in the error message being displayed.
Solving this issue requires a fundamental understanding of how Android 11 treats permissions and storage access. One key area of focus is the introduction of Scoped Storage by Google. This privacy-centric feature gives applications access only to app-specific directories and media, restricting broad access to shared storage.
<!-- AndroidManifest.xml --> <manifest ... > ... <application android:requestLegacyExternalStorage="true" ... > ... </application> </manifest>
However, this approach does not seem to work consistently, as per the developers reporting “Android 11: EACCESS (Permission Denied)” error. A plausible solution is to adapt to the new storage model by opting for the Storage Access Framework (SAF) or use MediaStore API where possible.
Furthermore, data privacy enhancements in Android 11 also impose limitations on foreground service types that an app can start from the background, potentially causing the EACCESS issues.
As famed computer scientist Edsger W. Dijkstra rightly said, “We should do away with the absolutely specious notion that everybody has to earn a living,” developers must strive to address these caveats proactively and adapt their strategies in line with ongoing advancements.
This adaptation includes overhauling permission requests in accordance with the new changes, migrating necessary files into app-specific directories, and careful testing across all target versions when introducing the ‘android:requestLegacyExternalStorage’ attribute.
Referencing the official data storage guide and StorageManager documentation could be immensely beneficial during this process.
New Approach | Old Approach |
MediaStore API / SAF | Broad Storage Access |
Permissions Specific | ‘requestLegacyExternalStorage’ attribute |
To summarize, “Android 11 Open Failed Eacces (Permission Denied)” is bridgeable by adopting the right tools and adapting codebases according to Google’s updated standards. By considering these factors and working towards a more privacy-conscious ethos that respects user rights while delivering services effectively, the road to seamless application experiences in Android 11 becomes clearer.