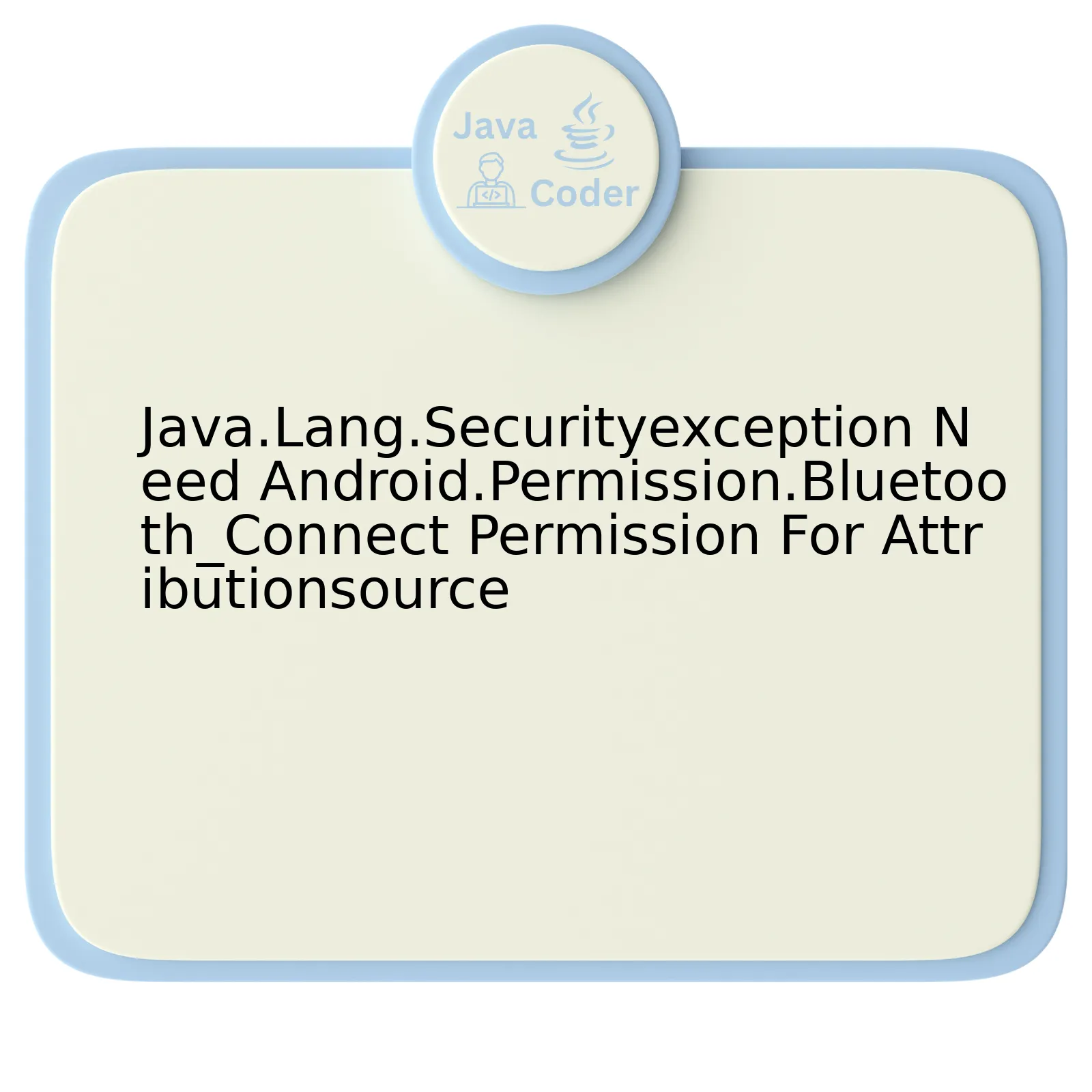
Java.Lang.SecurityException occurs when an application tries to perform an operation for which it does not possess the required permission. Specifically, the Android.Permission.Bluetooth_Connect exception happens when your app attempts to interact with Bluetooth without having appropriate access permissions.
Error | Reason | Impact | Solution |
---|---|---|---|
Java.Lang.SecurityException Need Android.Permission.Bluetooth_Connect | The application tries to enact a procedure without the mandated security clearance | The application will cease operation | Implement Android permissions Review app’s code and secure necessary permissions |
Moving forward, it’s important to understand that every Android app operates in its own security sandbox. The Android OS uses this to ensure apps do not gain access to system resources or other app data without proper permissions. This safeguard prevents malicious software from performing harmful actions on users’ devices.
To resolve the Java.Lang.Securityexception need Android.Permission.Bluetooth_Connect error, correct and explicit setting of the required permissions is crucial. Check your AndroidManifest.xml file to ensure you have requested the necessary permissions.
<uses-permission android:name="android.permission.BLUETOOTH" /> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN" /> <uses-permission android:name="android.permission.BLUETOOTH_CONNECT" />
Furthermore, always keep in mind that any API call that needs particular permissions will mention them in the documentation. Use this as a reference when developing.
Lastly, a famous quote to reflect upon: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler.Source
Understanding the Java.Lang.Securityexception in Android Development
Java.Lang.SecurityException is one of the crucial runtime exceptions that one may encounter while developing an Android application in Java. Specifically, the “Android.Permission.Bluetooth_Connect” permission-required exception indicates the application requires certain special permissions to execute certain Bluetooth-related tasks.
To provide a detailed understanding, let’s first understand what these terms mean:
- Java.Lang.SecurityException: This type of exception typically occurs when there’s a violation of the security policy embedded into your Android application. It signals the Java Runtime Environment (JRE) that there are attempts to execute prohibited actions such as reading a file without the proper security privileges.
- Android.Permission.Bluetooth_Connect: This is a permission declaration in Android which allows an app to make Bluetooth connections. Trying to establish such connections without declaring this permission in your AndroidManifest.xml will raise a Java.Lang.SecurityException.
Therefore, if you are facing a Java.Lang.SecurityException indicating the need for Android.Permission.Bluetooth_Connect, it simply means that your application tried to perform a Bluetooth connection operation that requires the ‘Bluetooth_Connect’ permission, but it was not declared prior in the AndroidManifest.xml file.
Here is a snippet on how to add this permission into your AndroidManifest.xml file:
<uses-permission android:name="android.permission.BLUETOOTH_CONNECT"/>
Remember, granting sensitive permissions like this should be well justified and necessary due to inherent risks associated with it. So, it might also be helpful to request this permission dynamically at runtime rather than declaring it directly in the AndroidManifest.
In the words of James Gosling, the father of Java – “One of the theories we have is that people in general become comfortable with whatever risks they deal with on a regular basis.” This applies very aptly to managing permissions in Android development; make sure you only use those which are absolutely essential, keeping user privacy and security in mind.
It’s important to note that sometimes, even after declaring permissions in the manifest, there might still be instances where the SecurityException is raised due to different Android OS level behaviors & changes or application settings. Therefore, understanding the nature of Java exceptions and properly handling them is vital to robust and secure Android app development. You can always refer to the official Android Developers guide on permissions for more information.
Mastering Permissions: The Role of Android.Permission.Bluetooth_Connect
Strategic mastery over permissions is a pivotal aspect in the practical development of Android applications. As an integral part of this structure, Android.Permission.Bluetooth_Connect plays an indispensable role in handling Bluetooth connectivity capabilities. Acquiring permission to connect other devices via Bluetooth and exchange information can significantly enhance your application’s functionality.
However, the challenge arises when dealing with
Java.Lang.SecurityException
, which typically proclaims the need for Android.Permission.Bluetooth_Connect permission for AttributionSource. In such scenarios, accurately understanding and rectifying this issue becomes crucial.
In order to resolve this exception, you should review your application’s coding carefully. Not requesting necessary Bluetooth permissions explicitly from the user may lead to triggering a security exception. You would need to implore the users to grant
Android.Permission.Bluetooth_Connect
manually. This permission problem, if not fixed promptly, results in interrupted program execution or even crashing of your application, leading to sub-optimal user experiences.
Below is an example code snippet that requests the important Bluetooth Connect permission:
if (ContextCompat.checkSelfPermission(thisActivity, Manifest.permission.BLUETOOTH_CONNECT)
!= PackageManager.PERMISSION_GRANTED) {
// Permission is not granted
ActivityCompat.requestPermissions(thisActivity,
new String[]{Manifest.permission.BLUETOOTH_CONNECT},
MY_PERMISSIONS_REQUEST_BLUETOOTH_CONNECT);
}
The key here is to invoke ‘requestPermissions()’ method if the required BLUETOOTH_CONNECT permission is not granted by the user.
Furthermore, the use of Attribution Source implies that the app is accessing usage statistics, which brings in the additional dimension of privacy-related considerations. It is essential to abide by the principles of transparently informing the user about all permissions sought and their intent of use.
With a nod to Jamie Zawinski’s insightful perception, “Every program attempts to expand until it can read mail. Those programs which cannot so expand are replaced by ones which can.” As software developers, it is our responsibility to ensure that our applications not only fulfill their purpose efficiently but also cherish user trust by respecting their privacy and abiding by due security measures.source.
Boosting your app’s capacities with Bluetooth connectivity indeed opens doors to innovative possibilities, provided the correct permissions tactics are put into place to ensure seamless operation and privileged user experience.
Navigating the Attributionsource Requirement in Java Programming
Java developers must understand the intricacies of permission requirements and how they affect the execution of different tasks in programming applications. The “java.lang.SecurityException” often pops up during Android app development, specifically when one is dealing with the “android.permission.BLUETOOTH_CONNECT” permission. It represents a denial from the system stating that the current operation lacks valid authentication.
Firstly, getting to know what an AttributionSource aligns well with understanding this issue. Google introduced it as part of its Android 12 changes to manage and control attributions related to the interactions with external applications. Primarily, it tackles improving the privacy and security by defining the level of access services and processes have over data shared between apps source.
From a programming perspective, the AttributionSource requirement has tight connections with the Bluetooth-related permissions like `BLUETOOTH_CONNECT`.
The introduction of the AttributionSource adds an extra layer of policy regulation. Any violation manifests that a certain operation crosscuts either the permission scheme set up by the developer, or violates default permissions set by the Android operating system.
The need in “java.lang.SecurityException: need android.permission.BLUETOOTH_CONNECT permission for AttributionSource” is underlined by this fact – your code usually requires adjustments to adhere to Android’s latest guidelines.
An error typically surfaces as the following:
java.lang.SecurityException: Need android.permission.BLUETOOTH_CONNECT permission for AttributionSource { pkg=YourPackageName uid=-1 flags=[ FLAG_TRUSTED ] }
The way out usually lies in embedding appropriate permission requests within your application manifest file (`AndroidManifest.xml`). As part of a comprehensive solution, making use of context-embedded request functions such as `requestPermission()` enhances the user experience by instigating action-oriented dialogs.
A typical example could look like this:
// Requesting permission at runtime if (ContextCompat.checkSelfPermission(thisActivity, Manifest.permission.BLUETOOTH_CONNECT) != PackageManager.PERMISSION_GRANTED) { ActivityCompat.requestPermissions(thisActivity, new String[]{Manifest.permission.BLUETOOTH_CONNECT}, MY_PERMISSIONS_REQUEST_BLUETOOTH_CONNECT); }
Your job is not only to ensure the successful compilation and execution of your application but also to deliver an output that respects users’ privacy and aligns with platform standards.
Renowned technologist Robert C. Martin once said, “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code…[Therefore,] making it easy to read makes it easier to write”.
This quote reinforces the importance of respecting language and OS conventions in the construction and management of permission demands in Java programming, including handling attribution sources in Android app development.
Dealing with Bluetooth Connection Issues in Android Permission Settings
Dealing with Bluetooth connection issues in Android can turn to be a daunting task, especially considering the “Java.Lang.Securityexception Need Android.Permission.Bluetooth_Connect” error that you may encounter during the process. This issue is often caused by the absence of necessary permissions in the app’s manifest file.
Taking cognizance of Andrew Rubin’s proclamation that:”If you have something that you don’t want anyone to know, maybe you shouldn’t be doing it in the first place.”, it becomes apparent that transparency is crucial when handling permissions and exceptions in coding and development.
Let us now delve deeper into comprehending this phenomena:
Breaking Down The Error
When an application attempts to execute a task which necessitates specific system permissions without having acquired them earlier, Android responds with a SecurityException. Root of this issue lies in the failure of clearly declaring these permissions within the AndroidManifest.xml file.
According to Fred Brooks, author of The Mythical Man-Month, “Good judgement comes from experience, and experience comes from bad judgement.” His words ring true in dealing with such errors: one must understand from where the problem arises to adequately handle it.
This “java.lang.SecurityException: Need android.permission.BLUETOOTH_CONNECT permission for AttributionSource” error essentially indicates that the Android system requires the ‘BLUETOOTH_CONNECT’ permission to perform a Bluetooth-related operation. However, this permission has not been declared or granted.
Here is a short piece of sample code that may trigger such error:
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter(); bluetoothAdapter.startDiscovery();
To prevent this error from surfacing, you should follow these steps:
1. Adding Necessary Permission
The “android.permission.BLUETOOTH_CONNECT” needs to be added to your AndroidManifest.xml file.
<uses-permission android:name="android.permission.BLUETOOTH_CONNECT"/>
Remember what the Linux creator Linus Torvalds says: “Most good programmers do programming not because they expect to get paid…but because it is fun to program.”
Fun aspect persists if you understand every component you add in your coding journey. Here, we took authorization for our application to begin a Bluetooth connection with another device through adding BLUETOOTH_CONNECT permission.
2. Requesting Necessary Permission
Even after providing required specifications in the AndroidManifest.xml file, these may not be enough because Android recommends applications to ask users for Bluetooth-related permissions at run time.
For instance, here’s an example on how to request BLUETOOTH_CONNECT permissions at runtime:
if(ContextCompat.checkSelfPermission(this, Manifest.permission.BLUETOOTH_CONNECT) == PackageManager.PERMISSION_DENIED){ ActivityCompat.requestPermissions(this, new String[] {Manifest.permission.BLUETOOTH_CONNECT}, REQUEST_CODE); }
It is always essential to ensure that developers take responsibility for seeking the necessary authorizations that their applications might need for optimal performance.
I hope this provides a clear insight into understanding and resolving the “java.lang.SecurityException: Need android.permission.BLUETOOTH_CONNECT permission for AttributionSource” error, making the process of handling Bluetooth connections in Android far less overwhelming.
The
java.lang.SecurityException
error is prevalent when dealing with android OS permissions, specifically the Bluetooth connection permission. When this exception surfaces, it essentially means that the Android application in question does not have sufficient privileges to perform a specific action or access certain system resources.
In this context, the error seems to stem from an ungranted Bluetooth connectivity permission required by
AttributionSource
. An important aspect of Android programming to note here is the management of required system permissions. When you developed your app, AndroidManifest.xml needed to include an entry that explicitly requests for
android.permission.BLUETOOTH_CONNECT
permission.
For instance:
<uses-permission android:name="android.permission.BLUETOOTH_CONNECT"/>
It’s critical to note that even after declaring permissions in manifest file, the application still needs to request these permissions during runtime if they are categorized as dangerous permissions, something that Bluetooth is indeed considered. Consequently, you might want to integrate functionality to authenticate user consent for such operations.
A simple example can be like this:
if (ContextCompat.checkSelfPermission(thisActivity, Manifest.permission.BLUETOOTH_CONNECT) != PackageManager.PERMISSION_GRANTED) { // Permission is not granted, prompt the user ActivityCompat.requestPermissions(thisActivity, new String[]{Manifest.permission.BLUETOOTH_CONNECT}, YOUR_REQUEST_CODE); }
In essence, overcoming
java.lang.SecurityException
involves observing stringent management of Android system permissions. And when dealing with IoT-specific permissions like Bluetooth, confirming proper declaration and requesting them at runtime becomes extremely indispensable. As Tim Berners-Lee once said “the Web as I envisaged it, we have not seen it yet. The future is still so much bigger than the past.”, indeed addressing such issues squarely propels us towards maximizing the potentials of web interactions and connections, and minimizing unnecessary security risks.
For a more detailed insight into working around Android permissions, Google’s official documentation on Requesting App Permissions could prove instrumental.