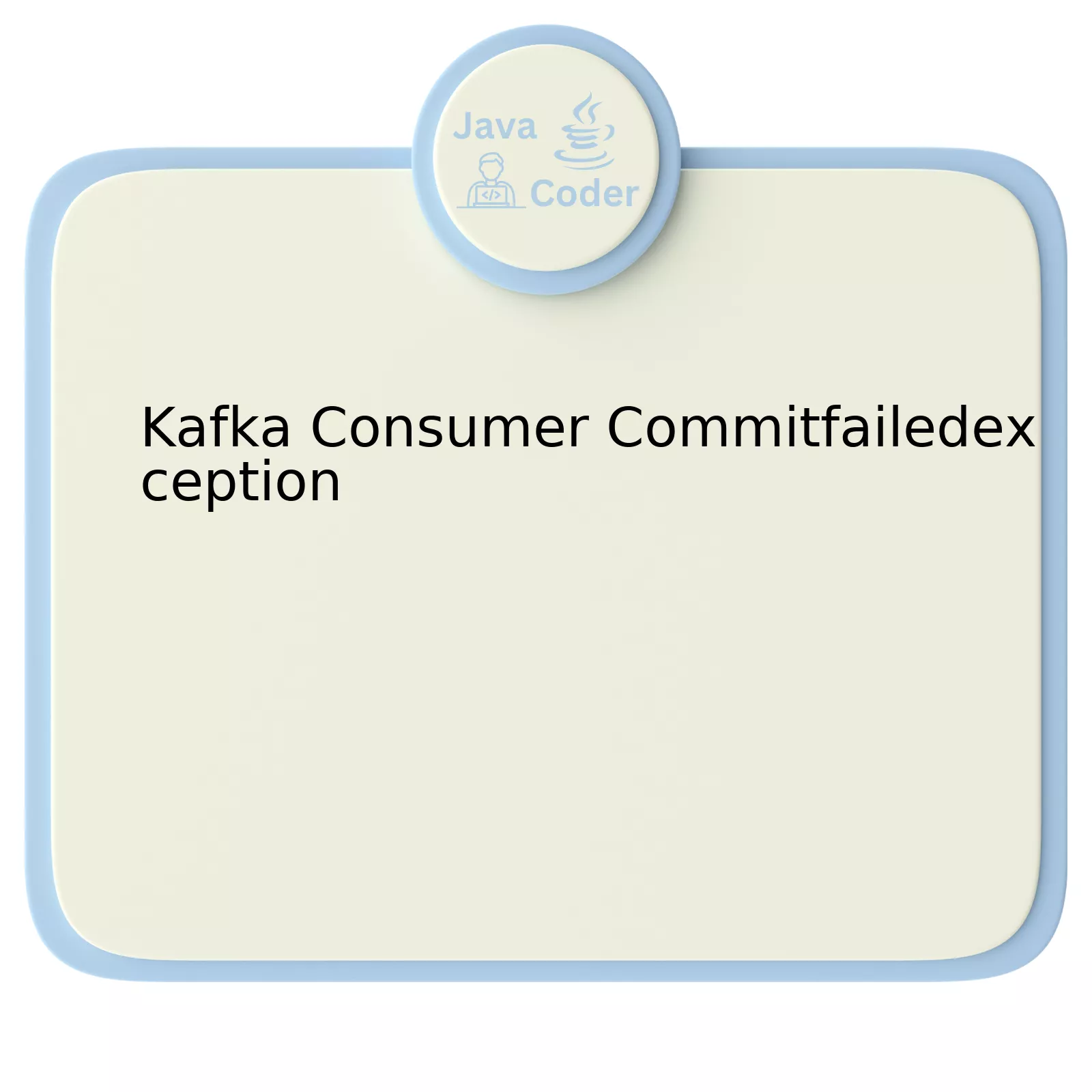
The Kafka Consumer CommitFailedException is an exception thrown during the Kafka consumer processing. This typically occurs when a consumer fails to commit an offset within the specified time limit while consuming messages from a Kafka broker.
To understand this concept deeply, let us delve into its main points; ‘Cause’, ‘Prevention’ and ‘Handling’.
Cause | The main cause of Kafka Consumer CommitFailedException is an occurrence where a certain consumer in a group lags behind or fails to commit the message offset within a stipulated time. In response, Kafka usually rebalances the consumer groups novating the lagging consumer’s partitions to other consumers. |
---|---|
Prevention | This problem can be prevented by tuning some parameters like `max.poll.records` and `session.timeout.ms`. Adjusting `max.poll.records` ensures smaller data amounts are handled per single poll, preventing data overflow. On the other hand, increasing `session.timeout.ms` allows longer timeout durations before a consumer is deemed non-responsive. |
Handling | If the error arises despite parameter modification, it is advisable to handle the CommitFailedException in your code logic. This can be achieved by retrying to commit offsets after a failure, giving your consumer additional attempts before being rebalanced. |
For instance, consider this Java snippet as an example:
try { consumer.commitSync(); } catch (CommitFailedException e) { //log the exception logger.error("Commit failed", e); // Retry committing the offset. consumer.commitSync(); }
“A common and insidious class of hard-to-find bugs is caused by failing to account properly for exceptional conditions”, Brian Kernighan aptly put it. Therefore, incorporating strategies that mitigate exceptions like the CommitFailedException would significantly contribute to more robust systems leveraging Apache Kafka.
Reference on dealing with these exceptions can be found at:
Apache Kafka Documentation.
Understanding The Kafka Consumer CommitFailedException
The Kafka Consumer CommitFailedException is a common issue encountered by many programmers. This exceptional scenario generally occurs when a commit cannot be achieved successfully due to several potential reasons.
Before diving into the details of this exception, it’s important to understand the concept of committing in Kafka. In essence, committing offsets in consumers signifies that you’re acknowledging the consumption of messages up to a certain point. By committing, you inform Kafka that it does not need to retain data until that point anymore.
Now, let’s analyze the possible reasons as to why we might encounter a CommitFailedException and how to handle such cases:
- Consumer Instance Is no Longer Part of the Group: When a consumer takes too long to read messages, the Kafka broker might deem it unresponsive and decide to exclude it from the consumer group. As a result, any subsequent attempts to commit will result in a CommitFailedException. This calls for efficient processes that guarantee quick reading and processing of messages.
- Rebalance Before Acknowledgement: If a rebalance operation occurs before acknowledgement can be given, a CommitFailedException is thrown. To avoid this, you may consider reducing the time between commits or lowering session timeout configuration settings.
- Disrupted Communication With the Kafka Cluster: Disruptions while communicating with the Kafka cluster can also lead to a CommitFailedException. Here, ensuring the stability of the network infrastructure could potentially mitigate these issues.
When it comes to dealing with CommitFailedException, here’s how the Kafka consumer API handles it:
try { records = consumer.poll(100); processRecords(records); consumer.commitSync(); } catch (CommitFailedException e) { logger.error("commit failed", e); }
In this snippet, a standard try-catch block is used to isolate the instance where a CommitFailedException is encountered. Once caught, the error information is logged for further examination and resolution.
As Don Norman, a renowned figure in technology, cogently indicated that “User-centered design means working with your users all throughout the project.” Just like we ensure user satisfaction in project development, managing exceptions well contributes both towards efficient code execution and maintaining system robustness.
Dealing with Kafka Consumer CommitFailedException: Practical Approaches
When consuming messages from Apache Kafka using the Kafka Consumer API, a common exception that you may encounter is
CommitFailedException
. At a high level, this exception signals that there has been a failure when trying to commit the offsets consumed so far by a Kafka consumer. This indeed demands attention as disregarding it may lead to data loss or duplication, elevating certain complications in handling data consistently.
Let’s dive into understanding how we can deal with this error more effectively:
Analyzing the Root Cause
To solve any problem, the first step should take on finding out ‘why’ it occurred. When working with Kafka, some of the reasons that often cause the CommitFailedException are:
• Your consumer was considered dead or inactive by Kafka’s group coordinator. This could be due to the fact that the heartbeat sent by the consumer was insufficient with respect to the configured session.timeout.ms and max.poll.interval.ms parameters:
consumerProperties.put("session.timeout.ms", "60000");
consumerProperties.put("max.poll.interval.ms", "300000");
• A rebalance occurred, that means a new consumer joined the group or an existing consumer left the group:
By identifying these root causes, you can then proceed to design a strategy to overcome such a situation.
Carefully Designing Poll Intervals
Make sure to configure your poll interval meticulously considering the actual network conditions, processing time of the messages, and the total number of consumers in your consumer group. Remember, it’s crucial to keep polling at a frequency higher than ‘session.timeout.ms’ and process messages within the period defined by ‘max.poll.interval.ms’.
Handling Exceptions Gracefully
Whenever
CommitFailedException
occurs, handle it gracefully. For instance, you can log it for further analysis or simply consume the message again. Here’s an example of how you may do this:
try { consumer.commitSync(); } catch (CommitFailedException e) { // log the exception and re-consume the message }
Anthony Altieri stated, “Coding is not just about creating things, but also fixing them.” True enough, dealing with errors and exceptions like
CommitFailedException
in Apache Kafka is essential in ensuring stability, predictability and correctness of your system. It highlights the importance of robust application design that gracefully handles failures and ensures data consistency.
Factors Contributing to a Kafka Consumer CommitFailedException
The Apache Kafka is a robust message-processing library. However, like all powerful systems, it sometimes has hiccups. For java developers exploring its realms, an especially significant Kafka pain-point is the ”
CommitFailedException
“. This exception occurs when a Kafka consumer fails to commit an offset update, thereby halting the consumption of further messages and disrupting your data pipeline.
Understanding this exception requires deep-diving into five key factors:
1. Unhandled Consumer exceptions:
When an unhandled exception occurs while consuming records (perhaps due to faulty business logic or a system-level issue), the application might choose not to catch and handle that exception appropriately. As a result, the consumer gets terminated abruptly, priming a stage for a
CommitFailedException
. It’s best to always handle exceptions with care, ensuring they don’t interfere with primary functions.
2. Overlapping Re-balances:
Rebalancing – Kafka’s protocol to evenly distribute the consumption of partitions across different consumers in a group – can trigger a
CommitFailedException
. If committing an update takes longer than the rebalance timeout set by the Kafka consumer (
max.poll.interval.ms
), rebalancing initiates before the commit completes. The outcome? A disgruntled Kafka instance throwing a
CommitFailedException
.
3. Excessive time between polls:
Kafka consumer api is designed to poll for new messages regularly from the broker (
poll()
method). If there’s too much time between two consecutive polls, the session may timeout. Concurrently, if another consumer comes into picture during this scenario, Kafka forces a rebalance causing
CommitFailedException
on the originally heartbeating consumer.
4. Network Issues:
Network problems can upset Kafka’s way of functioning. Disconnections, latencies, or patchy networks leading to missed heartbeat updates or prolonged responses to polls can trigger
CommitFailedException
.
5. Inappropriate Kafka configurations:
Like any resilient system, Kafka provides several configuration settings. Misusing these options, like extreme values for
session.timeout.ms
,
heartbeat.interval.ms
, and
max.poll.records
, can unwittingly create a setup welcoming
CommitFailedException
.
Alan Kay, the pioneering computer scientist, once said, “Simple things should be simple, complex things should be possible.” While demystifying Kafka’s CommitFailedException might initially seem impenetrable, breaking down the contributing factors reveals it as nothing more than a series of manageable issues.
Problem | Solution |
---|---|
Unhandled Consumer Exceptions | Catch and handle exceptions appropriately within the consumer. |
Overlapping Re-balances | Keep the duration of processing records less than max.poll.interval.ms |
Excessive time between polls | Poll more frequently with smaller batches. |
Network Issues | Ensure a stable network connection. |
Inappropriate Kafka configurations | Set the properties keeping in mind your use case and Kafka’s design philosophy |
Addressing these areas will make working with Kafka consumers more predictable and potent – a step closer to realizing Kay’s vision about simplicity and possibility.
Mitigating the Impact of Kafka’s Consumer CommitFailedException
Apache Kafka is a popular distributed streaming platform that has significant repute in the field of Big Data for its event-driven approach. Its ability to handle real-time data feeds has captivated many developers across the globe. However, working with this technology might sometimes yield errors such as the Consumer CommitFailedException. A comprehensive and in-depth understanding of the cause, nature, and appropriate handling techniques of this exception is integral to harness major potential of Apache Kafka.
Understanding the Exception
The
CommitFailedException
in Apache Kafka arises when a Kafka consumer encounters an obstacle while committing an offset. This usually happens when:
– The group of the consumer, which was trying to commit the offset, rebalances before it could effectively finish off the task.
– The consumer instance was considered dead or inactive by the group coordinator due to no heartbeat for a considerable period.
These scenarios can lead to a disruptive experience causing delayed processing or even duplications in processing in extreme cases. Hence, understanding how to mitigate this is crucial.
Mitigation Techniques
Fortunately, there are several ways developers can mitigate the impact of Kafka’s Consumer CommitFailedException, including albeit not limited to automatic retries, manual commits, setting appropriate timeout periods, and more:
1. Autonomous Retry: Enable automatic retry mechanisms. In case of temporary network anomalies, an autonomous try-catch block that allows the consumer to retry committing after a specified period can prove beneficial.
try { consumer.commitSync(); } catch (CommitFailedException e) { // Possibly log the exception and then retry }
2. Manual Commits: Encouraging manual commits over automatic commits can also be an effective way towards mitigation since it gives greater control allowing to manage offsets even if duplications occur.
3. Setting Appropriate Timeouts: By configuring session.timeout.ms to an optimal time which will hold the member’s spot in a consumer group during a partition rebalance procedure.
While these steps can help reduce the probability of a `CommitFailedException`, it’s important to remember that in a distributed system like Kafka, exceptions and failures are inevitable. Testing applications for failure scenarios and investing in monitoring frameworks that enable observability can go a long way in ensuring an organization’s Kafka streams are healthy and robust.
As software pioneer Martin Fowler mentioned, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Therefore, while handling technical hiccups like CommitFailedException in Kafka, it is essential for developers to anticipate, investigate, and effectively fix errors instead of just running away from them, fostering a resilient coding culture.
The Kafka Consumer CommitFailedException is considered a notable stumbling block in the world of data streaming. This particular exception primarily stems from the internal balancing act whereby the Kafka consumer tries to handle multiple threads within the same group id. When these concurrent threads encounter a hitch, there’s an auto-rebalance disruption that results in a CommitFailedException.
When it comes to dealing with this exception, developers might find themselves needing to approach the problem from various angles:
- Maximizing session.timeout.ms and max.poll.interval.ms: Larger numbers can help reduce rebalancing by allowing more leeway for long-running tasks.
- Using Smaller Payloads: If jobs are too bulky, they’ll take longer to process and be more likely to run into timeouts.
- Appropriate Error Handling: Catching CommitFailedExceptions and handling them properly can also help overcome this issue. Programmed solutions that treat such exceptions as transient interruptions may serve better.
//Use try...catch block to handle Kafka Consumer Commitfailedexception try { //Your normal Kafka consumer code here }" catch (CommitFailedException e) { //Handle exception here }
All technological advances require us to learn, adapt and grow in order to leverage their capabilities fully. As Marc Andreessen stated, “Software is eating the world”. The challenge presented by Kafka Consumer CommitFailedException helps to underline this – the fact that even though software development routinely presents problems, our ability to surmount them lies at the heart of our innovation and growth.
By adopting measures like increasing timeout values, reducing payload sizes, or developing sophisticated error handling procedures, developers can anticipate and circumvent the occurrence of a Kafka Consumer CommitFailedException. Overcoming such obstacles paves the way for more opportunistic utilization of the Apache Kafka platform. Furthermore, understanding the nuances of such exceptions can vastly enhance data stream processing and increase the robustness of your application.
This has implications on the broader scale – when your consumer handles data loads efficiently, you pave the way for stellar system performance paired with superior data reliability. That’s why understanding the Kafka Consumer CommitFailedException not only maximizes the efficacy of your consumer, but also allows for the optimized operation of your entire system.