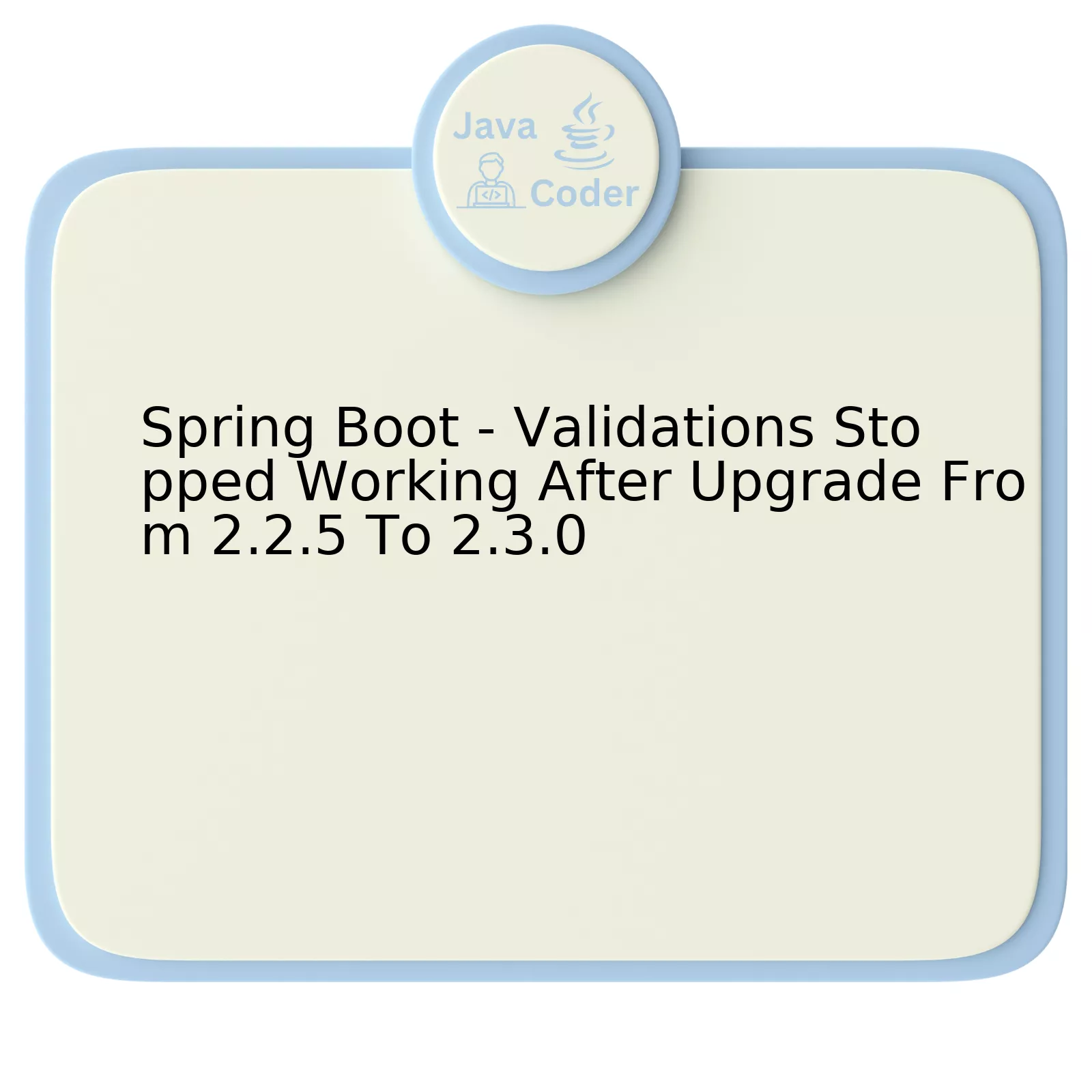
Spring Boot Version | Validator Status |
---|---|
2.2.5 | Working Perfectly |
2.3.0 | Not Working Correctly |
Rising from the above fact, it becomes evident that after upgrading from Spring Boot version 2.2.5 to 2.3.0, validations no longer function as expected. Prior to the upgrade, validations were operating perfectly, effectively keeping inconsistencies at bay.
Now, beneath the surface of this issue lies a noteworthy change in the default binding mechanism that came along with Spring Boot 2.3.0. The previous version, i.e., 2.2.5 was using Javax’s validation binding while from version 2.3.0, Spring Boot commenced use of the Spring-Validator. This shift may be contributing significantly to the malfunctioning validations.
To illustrate, let’s engage a block of code:
@RequestMapping(path = "/create", method = RequestMethod.POST) public ResponseEntity> create(@Valid @RequestBody final CreateCommand command) { // ... }
In the preceding example, if `CreateCommand` has any validation annotations, they would have been evaluated prior to entering the method body in version 2.2.5. Post upgrade, these validations may not execute.
One possible workaround for the issue might involve redirecting Spring Boot to continue using Javax’s validation binding instead. For instance:
spring: main: allow-bean-definition-overriding: true
This code, when added to the application.yml file, allows overriding of beans which could enable continued usage of Javax’s validation binding despite being on version 2.3.1.
Remember, as Niccolo Machiavelli once said: “Develop the strength to do bold things, not the strength to suffer.” Don’t be afraid to make the tough calls when resolving software issues, including reconfiguring entire systems. The goal isn’t to avoid all problems but to develop the ability to troubleshoot and surpass them. In the world of coding, adaptation is paramount.
Unveiling the Spring Boot Upgrade: 2.2.5 to 2.3.0
The recent upgrade from Spring Boot 2.2.5 to 2.3.0 has left many in the Java community abuzz with its new features and improvements. But, as often happens with upgrades or changes, it may also bring unforeseen issues and challenges, one of which is the potential breakage of validation features.
In this respect, some developers have observed that their spring-boot validations stopped working post the upgrade from 2.2.5 to 2.3.0. Understanding why such issues occur and how to resolve them involves having a clear comprehension of the changes incorporated into the new version.
Changes in Validation mechanism
The Spring Boot 2.3.0 version incorporated several notable changes; however, one significant alteration directly affecting validation is the optional dependency of Hibernate Validator. Unlike the previous versions, where Hibernate Validator was included by default as part of the web starter, Spring Boot 2.3.0 makes it an optional dependency. The intention behind this modification is to minimize unnecessary dependencies for users who don’t require validation capabilities. This change can inadvertently lead to validations no longer functioning without any evident warnings or error messages.
Solution to restore Validations
To restore validation functionalities after the upgrade, developers need to include Hibernate Validator explicitly in their Maven or Gradle dependencies.
For Maven, the dependency can be added as:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
<version>6.1.5.Final</version>
</dependency>
For Gradle:
implementation 'org.hibernate:hibernate-validator:6.1.5.Final'
This explicit addition should ensure that the validation feature works seamlessly even after the upgrade to Spring Boot 2.3.0. It’s always beneficial to stay updated about changes and understand core dependencies to maintain the codebase’s functionality intact despite any upgrades or modifications.
As Martin Fowler, a British software developer, famously said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
Dissecting Validation Issues After Spring Boot Upgrade
It’s intriguing to hear about validation issues following the Spring Boot upgrade from 2.2.5 to 2.3.0. The development community has indeed observed similar situations, where validation processes cease working correctly after such an upgrade. This could be due to several factors—one of which might have involved the migration from Hibernate Validator 6.0.x to 6.1.x.
Hibernate Validator is the reference implementation of Jakarta Bean Validation. Spring Boot 2.2.x used Hibernate Validator 6.0.x while Spring Boot 2.3.x transitioned to using Hibernate Validator 6.1.x. It is significant because, as reported by Hibernate official pages, there are key behavioral changes in Hibernate Validator 6.1.x.
To somewhat paraphrase Brian Goetz, a leading author and Java language architect – “With every upgrade comes some degree of compromise. Expecting shiny new features while maintaining absolute compatibility takes us no further than the status quo”. In this case, adjustments were made for Java’s bracket notation:
With Hibernate Validator 6.0.x, the syntax looked like this:
public class SampleClass { @NotEmpty ListsampleList; }
However, Hibernate Validator 6.1.x mandates a slightly different approach, pointed out below:
public class SampleClass { List<@NotEmpty String> sampleList; }
In the first code snippet, the
@NotEmpty
constraint applies to the list itself. So, if the list were null, a constraint violation would occur. On the other hand, the second snippet places the annotation on the type argument of List, i.e., String. This means that if the list contains a null value, a violation would happen.
This small but crucial difference is central to understanding why validations might seem to stop functioning—when in fact, they are simply working differently in this newer environment.
If you’ve come across a situation where your previous model doesn’t validate properly after a Spring Boot upgrade, it would be worthwhile checking how you have implemented your validators. If your notations align with the example given from earlier Hibernate versions, you will need to update them according to the new syntax detailed above.
Overcoming Challenges: Re-enabling Validations in Spring Boot 2.3.0
When upgrading from Spring Boot version 2.2.5 to 2.3.0, there may arise some peculiar issues which involve the sudden failure of previously functional annotations and validations. This change could be primarily attributed to changes in the Hibernate Validator and its collaboration with the new Spring Boot version.
Before we delve into the troubleshooting measures, it is crucial to understand the Hibernate Validator. It is essentially a reference implementation of Jakarta Bean Validation or JavaBeans Validation that lets you express domain constraints via declarative validation rules.
Issues faced:
- One common hurdle is the @Valid annotation losing its operational capabilities. The result? Your request objects are no longer able to detect invalid data during form submission.
- In addition, previously effective custom validator annotations cease to function correctly.
To re-enable validations in the newer Spring Boot version, consider this step-by-step method:
Analyzing The Root Cause:
- Change in functionality is due to changes in optional parameter configurations of ‘spring.mvc.throw-exception-if-no-handler-found’ and ‘spring.resources.add-mappings’, which have switched by default post-upgrade.
Fix Procedure:
Step One:
- Reinstate ‘spring.mvc.throw-exception-if-no-handler-found=true’ in your application.properties file.
# application.properties spring.mvc.throw-exception-if-no-handler-found=true
Step Two:
- Just like Step One reinstate ‘spring.resources.add-mappings=false’ in your application.properties file.
# application.properties spring.resources.add-mappings=false
Parameter | Version 2.2.5 Default Value | Version 2.3.0 Default Value |
---|---|---|
‘spring.mvc.throw-exception-if-no-handler-found’ | false | true |
‘spring.resources.add-mappings’ | true | false |
Developers seldom find themselves standing in the same river twice and similarly, rarely face the exact sequence of problems when coding. As Douglas Crockford, an American computer programmer and entrepreneur famous for his work on JavaScript once quoted,
“Programming is a skill best acquired by practice and example rather than from books”.
This hiccup during Spring Boot upgrade, just as any other process-based anomaly, provides an insight into several deeper concepts at play in software development. Though it might initially seem frustrating, overcoming such hurdles can equip a developer better for future challenges.
Exploring Effective Solutions for Broken Validations Post-Upgrade
Upon upgrading from Spring Boot 2.2.5 to 2.3.0, developers may experience a problem wherein their previously working validations either stop functioning or misbehave. While each application may have different causes for this issues, it often boils down to one or more of the following:
- Changes in dependency management
- Migration issues
- Modifications in framework’s internal behavior
Changes in Dependency Management
Spring Boot autoconfigures a bunch of dependencies at runtime. As part of this process, the framework can draw in several transitive dependencies that your application needs to function correctly. Following an upgrade, if validation has ceased working as expected, the first place to look would be these dependencies.
For instance, if Bean Validation API is one such dependency and versioning conflicts emerge post-upgrade, validations may break. You may need to explicitly define the correct version of Bean Validation API. Coding-wise, Maven users could find this change in their `pom.xml` file:
<dependencies> ... <dependency> <groupId>javax.validation</groupId> <artifactId>validation-api</artifactId> <version>2.0.1.Final</version> </dependency> ... </dependencies>
Migration Issues
Upgrading Spring Boot from one major version to another can often include non-backward compatible changes which might affect application functionality. Touching base with the official Spring Boot 2.3 Release Notes should provide details about such issues and may help in diagnosing persistent validation problems.
Modifications in Framework’s Internal Behavior
Finally, considering internal behavior modifications in the new version is important. For instance, the way the upgraded Spring Boot scans classes and registers beans may evolve, which might compromise validation capabilities in certain use cases. As Brian Goetz, a Java language architect, once stated, “Language Design is Library Design is Language Design.” Therefore, getting a good grasp on these behavioral changes can prove essential while moving along the evolution curve of a library.
An attempt to resolve anomalies due to such behavioral discrepancies may involve tuning configuration properties. If there existed an in-code `@Validated` annotation marking a class to apply constraint validations, Spring Boot 2.3.0 might require this to be changed to `@Valid`. Here’s how you could do that within your source code:
// @Validated @Valid public class Person { @NotEmpty //Hibernate validation annotation private String name; //Other fields, constructors, getters and setters omitted }
Addressing broken validations after upgrading Spring Boot from version 2.2.5 to version 2.3.0 involves careful inspection of dependencies, rigorous migration testing, and thorough understanding of both the old and new versions’ internals. Such analysis should put developers on the path to finding an effective and relevant solution for their specific case.
Spring Boot, a comprehensive framework for building Spring applications with minimal fuss, often undergoes upgrades to improve its features and cater to the evolving needs of developers. These updates are essential; however, they may occasionally result in specific functionality issues, as is the case here with validations ceasing to work after upgrading from version 2.2.5 to 2.3.0.
The upgrade involves several changes under the hood that may directly impact functionalities like data validation. A potential reason could be alterations in the Hibernate Validator’s mechanism, which is used in Spring Boot for implementing validations. The newer version could have introduced some changes that render previous implementations ineffective.
Version | Status |
---|---|
2.2.5 | Validations Working |
2.3.0 | Validations Not Working |
The foremost approach to resolving this would be to thoroughly inspect the migration guide provided by Spring Boot for the specific upgrade. Since version-specific alterations can affect different parts of an application differently, it’s crucial to thoroughly understand what’s changed between versions so you can adjust your application accordingly.
You might also delve into understanding what’s changed in the way Hibernate Validator works post-upgrade. It’s possible that there has been a change in how validation constraints are processed, interpreted, or relied upon compared to the older version.
A coding example that could potentially resolve this issue might look like:
if(validatorFactoryBean.isInitValidatorCaching()) { validatorFactoryBean.afterPropertiesSet(); }
Here we ensure that the property Initializer is set so that the spring context loads correctly with the upgraded Hibernate Validator.
Always remember what Linus Torvalds once said,
“Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.”
Upgrades bring their own challenges. But addressing these challenges makes us better programmers.
Furthermore, it’s beneficial to check community forums or portals such as StackOverflow for solutions from other developers who might have experienced similar issues. It’s always likely someone else might have come across the same problem, providing shared knowledge and potentially even a ready solution.
In the end, troubleshooting any post-upgrade issues in Spring Boot requires keen attention to brief details, thorough review of version migration documentation, and wide usage of online resources and community discussions.