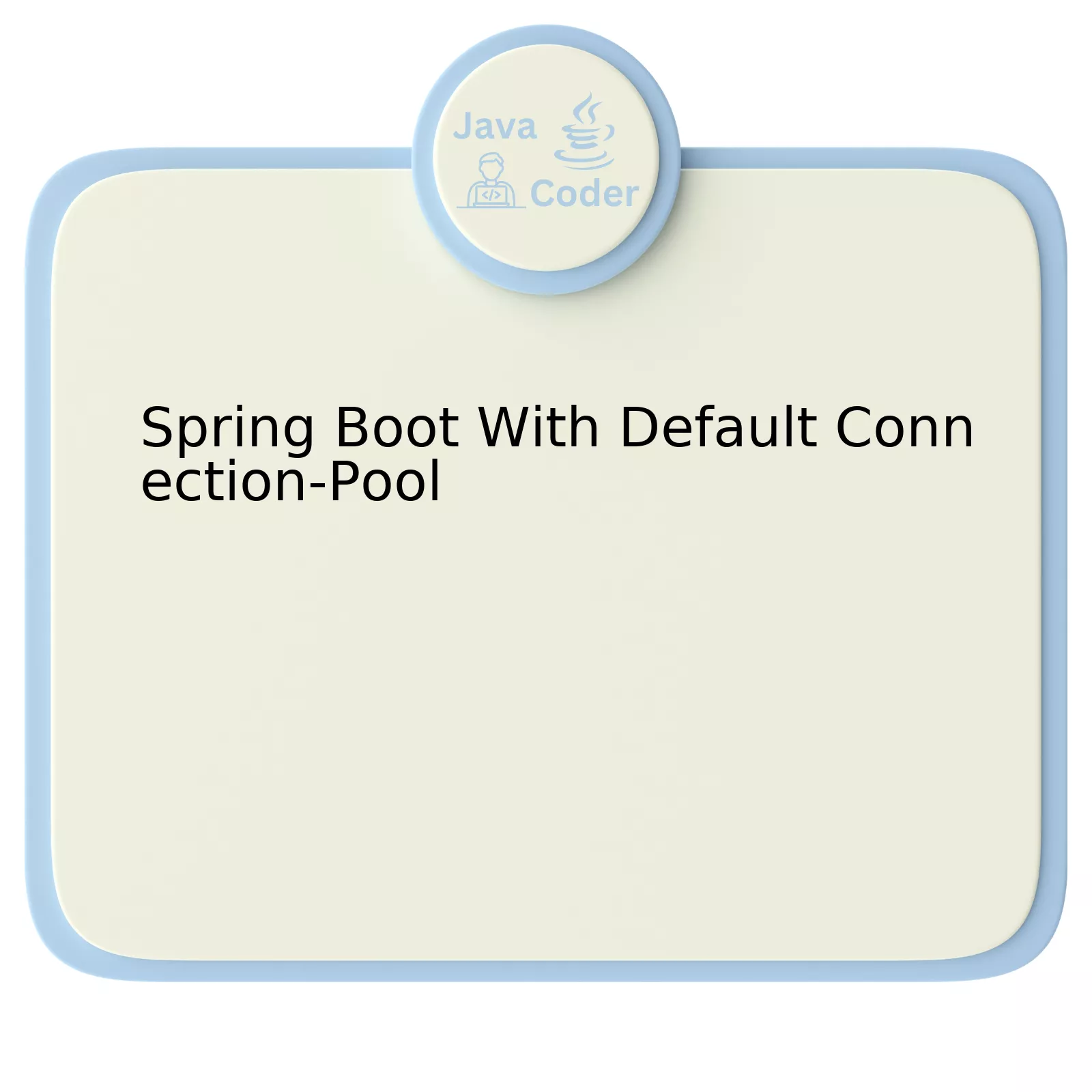
When we delve into Spring Boot’s default connection pool, you’ll find that it uses HikariCP due to its lightweight nature and better performance compared to other connection pools. However, if Tomcat, DBCP2, or C3PO are on the classpath, Spring Boot would also consider using them.
Let’s examine what three common connection pools look like with default values set by Spring Boot:
Connection Pool | Attribute Name | Default Value |
---|---|---|
HikariCP | MaximumPoolSize | 10 |
MinimumIdle | 10 | |
IdleTimeout | 600000 (10 minutes) | |
Tomcat | MaxActive | 100 |
MaxIdle | 100 | |
MinIdle | 10 | |
DBCP2 | MaxTotal | 8 |
MaxIdle | 8 | |
MinIdle | 0 |
This discernibly demonstrates how Spring Boot optimizes each connection pool’s configuration settings based on the defaults provided by the respective libraries. These values significantly influence the behavior of a web application, such as its concurrency, performance, and ability to recycle unused connections in an optimized manner.
For instance, MaximumPoolSize/MaxActive/MaxTotal determine how many database connections can be opened concurrently. If a pool has reached this limit and all the connections are busy, any incoming requests will need to wait.
Furthermore, MinimumIdle/MinIdle dictate the minimum number of idle connections a pool attempts to retain for future requests. This becomes especially significant when your application is expected to handle unpredictable bursts of requests.
The IdleTimeout attribute in HikariCP will relieve idle connections after this timeout has passed (10 minutes by default).
As Ken Thompson aptly said, “One of my most productive days was throwing away 1,000 lines of code.” We should aim to keep our configuration lean and adhere to the principle of sensible defaults, although these configurations may vary based on specific application needs.
These characteristics prove Spring Boot’s commitment to efficiency, flexibility, and performance – the framework ‘Boot’-straps applications with reasonable foundation configurations out-of-the-box, allowing developers to further tweak settings in pursuit of fine-tuned performance. For deeper customization, consult the official Spring documentation.
Understanding the Basics of Spring Boot Default Connection-Pool
To understand the basics of Spring Boot’s default connection pool, it is important to first comprehend what a connection pool is. A database connection pool primarily promotes software interaction with databases. It is a cache of database connections, held in memory for quick accessibility whenever software requests need it. Hibernate ORM, Java Database Connectivity (JDBC), and Java Persistence API (JPA) are some of the Java libraries that utilize database connection pooling. Hibernate and JPA take advantage of this technique via data-source abstraction classes.
In the context of Spring Boot, it does not come pre-configured with a database connection pool; instead, Spring Boot has HikariCP as its default connection pool library.
What makes HikariCP Spring Boot’s appointed choice?
- Fast performance: HikariCP offers superior speed when compared to other contenders.
- Simplicity: Hikari boasts fewer lines of code which translates to lesser potential points of failure.
- Efficient usage of hardware resources: With HikariCP are multiple layers of batching employed to reduce the duration threads remain suspended.
While integrating the connection pool into your Spring Boot application, you add dependencies in your Maven or Gradle file like:
<dependency> <groupId>com.zaxxer</groupId> <artifactId>HikariCP</artifactId> </dependency>
Then, adjust properties in your application.properties file as per your needs. Here are a couple of properties you can fine-tune:
spring.datasource.hikari.maximumPoolSize=10 spring.datasource.hikari.minimumIdle=2
As Bill Gates once mentioned, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” This sentiment is echoed in the simplicity and efficiency within which Spring Boot allows developers to implement and manage connection pools, contributing to better applications.
Key Benefits and Features of Using Spring Boot With Default Connection-Pool
Spring Boot Default Connection-Pool: The default connection pool in Spring Boot is highly efficient due to the automatic selection of the best available on the classpath. Usually, it picks either HikariCP, Tomcat pooling, or Commons DBCP2.
Features and Benefits |
---|
Performance Optimization |
HikariCP, which Spring Boot often defaults to if available, has been touted for its superior performance optimization. It has a simpler design aimed at delivering faster results by reducing software overhead. |
Reliability and Robustness |
The default connection-pool in Spring Boot provides greater reliability through built-in robust features like connection testing, timeouts, and retry attempts. |
Ease-of-Use |
The Spring Boot framework automatically configures your Spring application based on the JAR dependencies you added in the project. This simplicity extends to its handling of database connections. Plus, configurable properties make tailoring the pool to your need fairly straightforward. |
Consistency Across Applications |
With Spring Boot’s choice of default connection pools, developers maintain a consistent environment across different applications even if they are communicating with different databases. |
// Here's an example of how to configure HikariCP in Spring Boot: spring: datasource: url: jdbc:mysql://localhost:3306/mydb username: myuser password: mypassword hikari: minimumIdle: 5 maximumPoolSize: 20 idleTimeout: 30000 poolName: MyHikariCP maxLifetime: 2000000 connectionTimeout: 30000
Rod Johnson said, “One of the challenges that we – as industry – face is finding a balance between disruption and continuity.” And it’s clear Spring Boot helps strike this balance helpfully, by offering efficient database connection handling while ensuring seamless transition amongst different applications. By defaulting to the most intelligent option for connection-pool, it continues to simplify and streamline java development.
Further reading can be found at:
Practical Guide: Implementing Spring Boot With a Default Connection-Pool
Spring Boot is a resilient and production-ready framework that provides default configuration for developing Spring applications. One of the key aspects of this default configuration features is its database connection pool set up which significantly enhances performance and scalability of Java applications that interact with databases.
Talking about connection pools, they are highly important in any enterprise application as they manage the database connections. A connection pool works by opening multiple connections to the database at once. These open connections are then shared among all the users who need access to the database. Using a connection pool can reduce the latency of your database queries, providing faster response times for your users.
The primary benefit of Spring Boot lies in its ‘convention over configuration’ model. This means Spring Boot uses intelligent defaults to reduce the amount of boilerplate configuration we need to do. Thanks to this methodology, Spring Boot integrates seamlessly with connection pooling libraries such as HikariCP, Tomcat, and DBCP2 right out-of-the-box.
For instance, if you include HikariCP on your project’s classpath, Spring automatically configures it as your default connection pool. To illustrate, consider the Maven dependency below:
<dependency> <groupId>com.zaxxer</groupId> <artifactId>HikariCP</artifactId> </dependency>
Once your project includes HikariCP dependency (as above), you can configure it via properties in application.properties file:
spring.datasource.hikari.connectionTimeout=20000 spring.datasource.hikari.maximumPoolSize=10
Furthermore, if the HikariCP library is not included, Spring Boot decides the connection pool based on the availability of libraries in the following order: Tomcat > HikariCP > Commons DBCP2.
However, the Common DBCP2 only comes into the picture if you exclude both HikariCP and Tomcat from Spring Boot Starter Data JPA or JDBC dependencies.
As Bill Gates rightly said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” So is the case with Spring Boot’s default connection pool. By setting sensible defaults, it abstracts away much of the complexity involved in the connection pool management, making it an integral part of our everyday coding lives. The beautiful part? We might not even realize how heavily we have been relying on it.
Interestingly, there’s considerable online discussionhere on StackOverflow devoted to understanding and implementing Spring Boot’s default connection pool.
Therefore, embracing Spring Boot’s ‘convention over configuration’ philosophy is a practical solution that ensures productive software development while managing a robust default connection pool. Reviewing each choice will provide insight and facilitate better utilization of configuration properties provided by Spring Boot and in specific, aid in enhancing connection pool management efficiency.
Exploring Troubleshooting Techniques for Spring Boot Default Connection-Pool Issues
In relation to this topic, below diagnostic and resolution techniques can be applied when facing issues associated with the Spring Boot default connection-pool:
Troubleshooting Techniques | Solution |
---|---|
Poor Performance | The application is often slowed down by reduced response time or increased database load, which could possibly due to the fact that initial-size, max-active, or max-idle settings of your connection pool are not properly balanced. By tuning these parameters carefully, ideal balance between the number of simultaneous connections and resource usage can be achieved. |
Connection Timeouts | By adjusting the pool configuration setting, “spring.datasource.hikari.connectionTimeout”, you can prevent abrupt termination of your application due to database response delays. The timeout limit here should ideally match up with the common response delay of your database allowing smoother operation of the app. |
Inefficient Pool Utilization | Analyze the utilization pattern of the application. A very low pool utilization may indicate that your connection maximum pool size is too high. Likewise, a consistently hight utilization may indicates that your maximum pool size is too low. Monitor your application’s performance and adjust accordingly. |
Unexpected Shut Down Of Connections | This issue may attributed due to time-out happening because of long lasting idle-connections in the pool. To tackle this, setting properties like “spring.datasource.hikari.minimumIdle” can beneficial to maintain some idle connections at all times which ensures better performance on sudden demand spike. |
Let’s take an example of how to set the properties to achieve optimal performances using Tomcat JBDC connection pool,
spring: datasource: tomcat: initial-size: 15 max-active: 50 max-idle: 15 min-idle: 10
To draw on the wisdom of Elon Musk on challenges and solutions, he said, “When something is important enough, you do it even if the odds are not in your favor”. Identifying and dealing with the potential problems regarding Spring Boot default connection-pooling indeed comes under such criterion. This adds to the robustness of your application and ensures smoother operations. Online resources like official Spring Boot Documentation offers extensive knowledge on advanced level, specific troubleshooting tactics that you can incorporate.
Remember that the key is to understand your application and its needs. Observing trends over time and testing different approaches will help you to definitely optimize your applications.
Spring Boot greatly improves developer productivity by automating numerous configuration tasks. One such automation aspect is connection-pooling; Spring Boot comes with a default connection-pool configuration that is fairly optimized for general use cases.
The default connection-pool in Spring Boot utilizes the HikariCP library, known for its excellent performance and concurrency. This pre-configured pooling library minimizes the overhead of managing open connections to the database by reusing them, thus efficiently handling the application’s database access needs. Crucial configuration parameters like maximum pool size, connection timeout, idle timeout, etc., are managed automatically, but can also be adjusted manually using Spring Boot properties if necessary.
spring.datasource.hikari.maximum-pool-size=10 spring.datasource.hikari.connection-timeout=20000 spring.datasource.hikari.idle-timeout=300000
Despite being excellently equipped for most scenarios, the default connection-pool may not fit all situations. Depending on specific demands like the expected load, hardware capabilities, or the complexity of your transactions, it may be preferable to opt for customized configurations or different libraries like Apache DBCP or C3P0.
Here’s what Brian Goetz, Java Language Architect at Oracle, says about optimization: “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.” Even though he was primarily talking about code optimization, the concept applies equally well to connection pooling. Therefore, it’s recommended to start with the default settings and optimize only when and where necessary, based on careful observations and measured data.
You can discover more about customizing the Spring Boot Data Source Configuration on the official Spring Boot documentation.
Spring Boot’s out-of-the-box solutions, including its default connection pool, offer great starting points. Still, as with every automated tool, one must understand its working properly to leverage it fully and effectively.