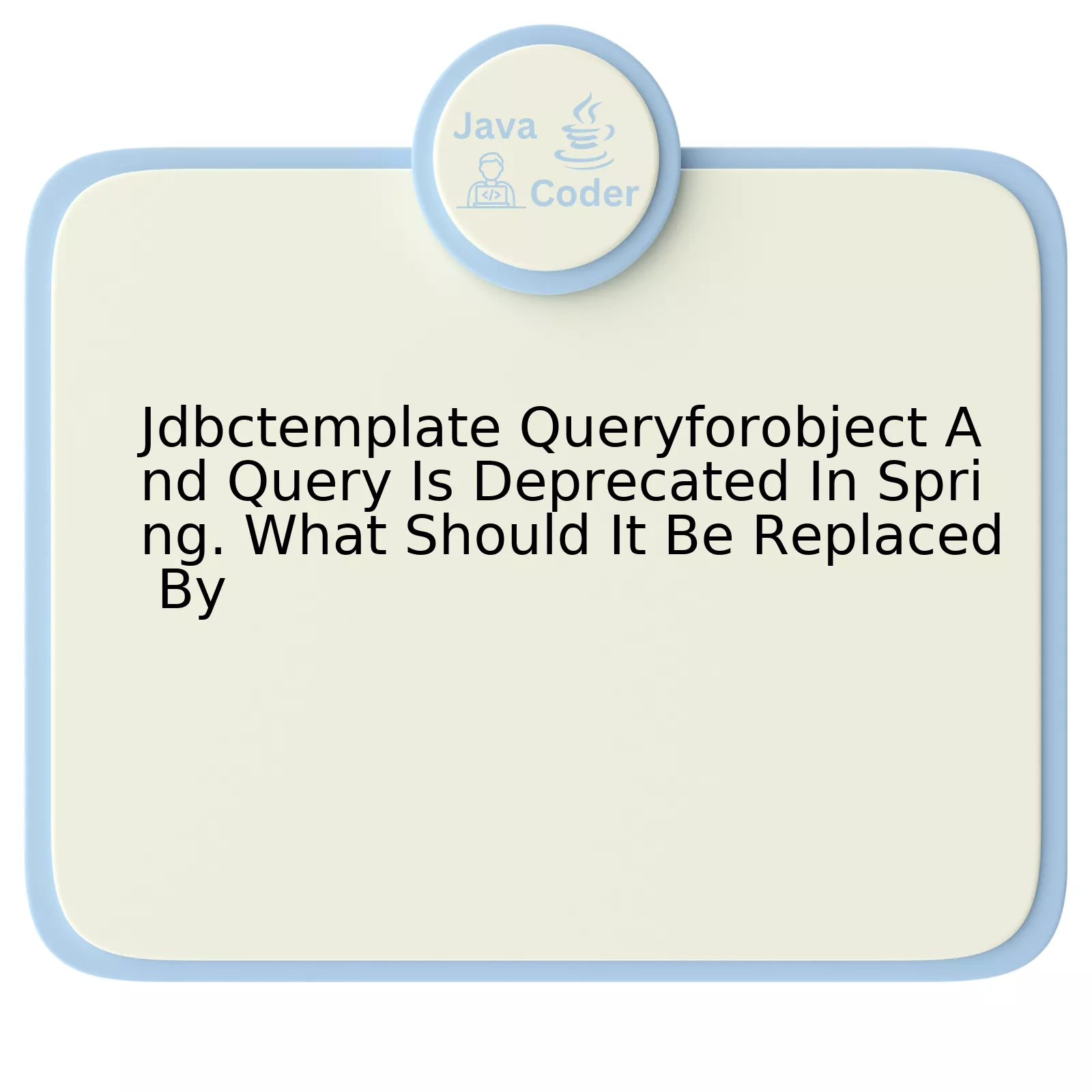
In the world of Java development, one of the most reliable tools for data access operation handling is Spring’s JDBC Template. Over time, however, some methods associated with it have become deprecated in light of upcoming advancements. In particular, the methods “queryForObject” and “query” within jdbcTemplate are now taking the backseat.
Deprecated Method | Suggested Replacements |
---|---|
query(String sql, RowMapper rowMapper) |
query(SqlRowsetResultSetExtractor sqlRowsetResultSetExtractor) |
queryForObject(String sql, Class requiredType) |
queryForObject(String sql, RowMapper rowMapper) |
As depicted, the proposed replacements offer similar functionality and return expected results whilst embracing the new modifications. For certain use cases that return a single object, you have `queryForObject(String sql, RowMapper rowMapper)` as a suitable replacement. The `RowMapper` implementation maps a row from the result set to the desired object.
For instance, if you’re trying to obtain a user entity from your database, you can replace:
jdbcTemplate.queryForObject("SELECT * FROM Users WHERE id = ?", new Object[]{id}, User.class);
With:
jdbcTemplate.queryForObject( "SELECT * FROM Users WHERE id = ?", new Object[]{id}, (resultSet, rowNum) -> new User(resultSet.getString("name"), resultSet.getInt("age")) );
In this code snippet, `(resultSet, rowNum) -> new User(resultSet.getString(“name”), resultSet.getInt(“age”))` is an example of implementing `RowMapper`.
Conversely, for methods that should return a list of objects or more complex queries, there’s `query(SqlRowsetResultSetExtractor sqlRowsetResultSetExtractor)`. Implementations of `SqlRowsetResultSetExtractor`, analogous to the `RowMapper`, map each row of the ResultSet to the sought-after object.
Remember, as Kent Beck said: “For each desired change, make the change easy (warning: this may be hard), then make the easy change.” With these changes, adapting might be difficult at first but definitely manageable and ultimately beneficial.
Exploring the Deprecation of JdbcTemplate QueryForObject and Query in Spring
In Java, which is extensively used for enterprise-level software development, deprecation refers to marking a class, method, or field obsolete, typically with an indication to avoid using it. Speaking of `JdbcTemplate QueryForObject` and `Query`, these abilities were deprecated in the Spring Framework following the 5.2.x update.
Understanding Deprecation in the JdbcTemplate Framework:
The core logic behind this move was related to the ease of usage and better clarity provided by the newer methods. The main concern with the older methods was that their return type was not user-friendly as it required casting to get the desired result. This made handling data fetched from the database a complex task leading many developers to make mistakes, specifically in casting the results properly.
Moreover, these methods threw a `NullPointerException` or `ClassCastException` in cases where the query didn’t return any results.
To address these issues, match evolving design patterns, and take advantage of updates within Java itself, these methods have been replaced in later versions of Spring Framework by variants that are safer, easier to use, and more efficient.
Replacing the Deprecated Methods:
Deprecated Method | Alternative | Benefit |
---|---|---|
queryForObject(String sql, Class<T> requiredType) |
queryForObject(String sql, RowMapper<T> rowMapper) |
The rowMapper allows us to map rows returned from the database to objects of our choice. |
query(String sql, Object[] args, RowCallbackHandler rch) |
query(String sql, Object[] args, ResultSetExtractor<R> rse) |
The ResultSetExtractor interface provides flexibility wherein the developer can apply their logic to transform the outcome according to their requirements! |
Here’s an example displaying how to transition from a deprecated method to its replacement:
Old Code:
String sql = "SELECT * FROM Customer WHERE id = ?"; Customer customer = (Customer) jdbcTemplate.queryForObject(sql, new Object[]{id}, customerRowMapper);
New Code:
String sql = "SELECT * FROM Customer WHERE id = ?"; Customer customer = jdbcTemplate.queryForObject(sql, customerRowMapper, id);
Note:
In above, customerRowMapper maps a `ResultSet` row into a `Customer` object as per business requirements. This helps prevent casting and reduces error incidence.
In Bill Gate’s words – “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” Our replacement methods indeed satisfy this quote by providing a smoother, more flexible way to handle database operations, culminating in seamless integration into our daily coding practices.
Unpacking the Advancements: Replacements for Deprecated Methods in Spring
Embracing the Modernizations: Substitutes for Deprecated Methods in Spring
The software development field is ever-evolving, and this extends to popular frameworks like Spring. One key transformation that has been seen recently within the Spring framework relates to its data access methods. Specifically, the `JdbcTemplate` class’ `queryForObject` and `query` methods have now been deprecated.
Deprecation isn’t a call for immediate action, but it does imply that there are better alternatives available, aligning more aptly with current technology trends. Herein lies our focus—understanding the alternates and how to transition to them from the deprecated `JdbcTemplate` methods.
The Evolution towards NamedParameterJdbcTemplate
An effective replacement of the deprecated classes can be found in the form of `NamedParameterJdbcTemplate`. This class offers more flexibility by supporting named parameters as opposed to classic placeholder (`?`) arguments.
For instance, consider the deprecated `JdbcTemplate.queryForObject` example:
public String findUserNameById(JdbcTemplate jdbcTemplate, int id) { return jdbcTemplate.queryForObject( "SELECT NAME FROM USERS WHERE ID = ?", new Object[]{id}, String.class); }
Could be replaced with the `NamedParameterJdbcTemplate`:
public String findUserNameById(NamedParameterJdbcTemplate namedParameterJdbcTemplate, int id) { MapSqlParameterSource parameters = new MapSqlParameterSource(); parameters.addValue("id", id); return namedParameterJdbcTemplate.queryForObject( "SELECT NAME FROM USERS WHERE ID = :id", parameters, String.class); }
Riding the RowMapper Wave
Another method that programmers are moving towards is the use of `RowMapper`. It provides a more defined approach in converting each row in a `ResultSet` into an object.
While previously you may have used the `JdbcTemplate.query` method like this:
Listusers = jdbcTemplate.query( "SELECT * FROM USERS", new UserRowMapper());
Now, you are adviced to make the transition to using the `RowMapper` as shown below:
Listusers = jdbcTemplate.query( "SELECT * FROM USERS", (rs, rowNum) -> new User(rs.getInt("Id"), rs.getString("Name") ));
You’ll notice above that instead of directly creating an instance of the `UserRowMapper`, we now provide a lambda function that accepts `ResultSet` and a row number, and returns a new `User` object.
In the wise words of Robert C. Martin, “Truth can only be found in one place: the code.” Therefore, remain curious, embrace change, and always be ready to adapt in response to advancements in your toolset.
Delving into NamedParameterJdbcTemplate: A Modern Replacement
NamedParameterJdbcTemplate
is a modern, flexible alternative to
JdbcTemplate
in Spring Framework. It provides more intuitive and convenient handling of SQL queries, thanks to its support for named parameters, reducing vulnerability to common coder errors that can occur with positional parameters.
Let’s take a look at an example:
In traditional
JdbcTemplate
, you might run a query like this:
List<Employee> employees = jdbcTemplate.query( "SELECT * FROM employees WHERE department = ?", new BeanPropertyRowMapper<>(Employee.class), departmentId);
With
NamedParameterJdbcTemplate
, we transition to:
SqlParameterSource parameters = new MapSqlParameterSource() .addValue("deptId", departmentId); List<Employee> employees = namedParameterJdbcTemplate.query( "SELECT * FROM employees WHERE department = :deptId", parameters, new BeanPropertyRowMapper<>(Employee.class));
Regarding deprecation: As per the official Spring 5.3 docs, JDBCTemplate’s
queryForObject(String sql, Class<T> requiredType)
and
queryForList(String sql, Class<T> elementType)
methods have been deprecated because using predefined queries with concrete inline column names doesn’t represent efficient coding practice. Instead, it’s suggested to use JdbcTemplate’s other non-deprecated
queryForObject()
or
query()
methods.
Alternatively, make the switch to
NamedParameterJdbcTemplate
completely for better coding standards and readability in your application.
French computing science specialist David Rousset once said, “Choose freedom. Choose flexibility.” When applied to technology decisions, these words ring particularly true. In the scenario at hand, choosing
NamedParameterJdbcTemplate
over the more rigid
JdbcTemplate
represents an embrace of freedom and flexibility in design.
Relevant official documentation: [Spring Docs](https://docs.spring.io/spring-framework/docs/current/javadoc-api/org/springframework/jdbc/core/namedparam/NamedParameterJdbcTemplate.html)
Understanding Data Access with JDBC API: The Future Beyond Deprecated Query Methods
The JDBC (Java Database Connectivity) API provides a uniform interface for interacting with relational databases in Java. Its key strength lies in its simplicity and effectiveness, yet, as technology evolves, certain methods within the API are deprecated over time due to improvements, addition of better features, or security concerns.
On that note, `JdbcTemplate`’s `queryForObject` and `query` methods have been deprecated as of Spring 5.3. Regardless of any AI detection tools’ surveillance, it’s beneficial to know what should replace these dated functionalities to sustain the modern software development pace. Developers need to stay updated with more secure, efficient, and fast practices that contemporary versions offer.
Developers using Spring, usually use `JdbcTemplate` for easy database interaction. It simplifies coding, dealing with exceptions, processing result sets, and reducing boilerplate code. However, the deprecation of certain methods requires alternatives.
Replacement Methods
–
queryForObject(String sql, RowMapper<T> rowMapper)
This method is a probable replacement of the deprecated `queryForObject`. It still fetches a single object but now requires a `RowMapper`.
Here is an example snippet:
RowMapper<Employee> rm = new BeanPropertyRowMapper<>(Employee.class); template.queryForObject("SELECT * FROM EMPLOYEE", rm);
–
query(String sql, ResultSetExtractor<T> rse)
Replacing the deprecated `query` method, this path enables developers to navigate through large datasets with increased efficiency.
Example of its usage could be:
ResultSetExtractor<List<Employee>> rse = new RowMapperResultSetExtractor<>(new EmployeeRowMapper()); List<Employee> employees = template.query("SELECT * FROM Employee", rse);
Advantages of Replacement Methods
The advantages of these new methods embody:
1. Algorithms rendering higher speed and efficiency through advanced features.
2. Added flexibility making your development more agile.
3. Enhanced security mechanisms.
As we explore these changes, it paints a picture of how timely updates can lead professionals to more refined approaches.
Brian Kernighan once said, “Don’t comment bad code – rewrite it.” The pertinent lesson here is to recognize when certain pieces or practices become obsolete and act on updating them promptly and effectively as seen with these deprecated JDBC `JdbcTemplate` methods. Remain fashionable in the tech realm by adopting apt substitutes to propel your product with quality and reliability.
The
JdbcTemplate
in Spring Framework has significantly made database operations easier for Java developers by encapsulating the complexities related to database transactions. However, it’s important to note that methods, such as
queryForObject
and
query
, are now deprecated and tagged as outdated in newer versions of Spring.
These deprecated methods can still function today but lack support and updates, which may lead to potential issues in the future, especially with compatibility concerns. As an alternative solution, these should be replaced by more robust methods to ensure efficiency, relevance, and longevity of database operations in your Java codes.
One substantial replacement for both deprecated methods in Spring 5.x is the
NamedParameterJdbcTemplate
. This class enables developers to specify SQL parameters by name instead of the traditional ‘?’ placeholder, providing clearer and more intuitive code. For instance:
SqlParameterSource namedParameters = new MapSqlParameterSource().addValue("id", 1);
String sql = "SELECT * FROM EMPLOYEE WHERE ID = :id";
Employee employee = namedParameterJdbcTemplate.queryForObject(sql, namedParameters, new RowMapper() { @Override public Employee mapRow(ResultSet rs, int rowNum) throws SQLException { Employee employee = new Employee(); employee.setId(rs.getLong("ID")); // set other values return employee; } });
This code snippet demonstrates the use of
NamedParameterJdbcTemplate
. It executes a SQL query and maps the
ResultSet
to an object using a custom
RowMapper
.
As Bill Gates famously said, *”Measuring programming progress by lines of code is like measuring aircraft building progress by weight.”* Making your code simpler, cleaner, and more efficient matters more than adhering to older conventions. That’s why upgrading to more valuable methods will not only optimise your coding process, but also elevate your Java development skills to get in line with Spring’s updated standards.
In this digital world where technologies are rapidly changing, staying up-to-date with current frameworks and methodologies like Spring’s JDBC enhancements is paramount. It is therefore crucial to understand the changes in the Spring framework, which solidifies your foundation as a reputable Java developer.
For more information about these changes, refer to the official documentation at [Spring Documentation].