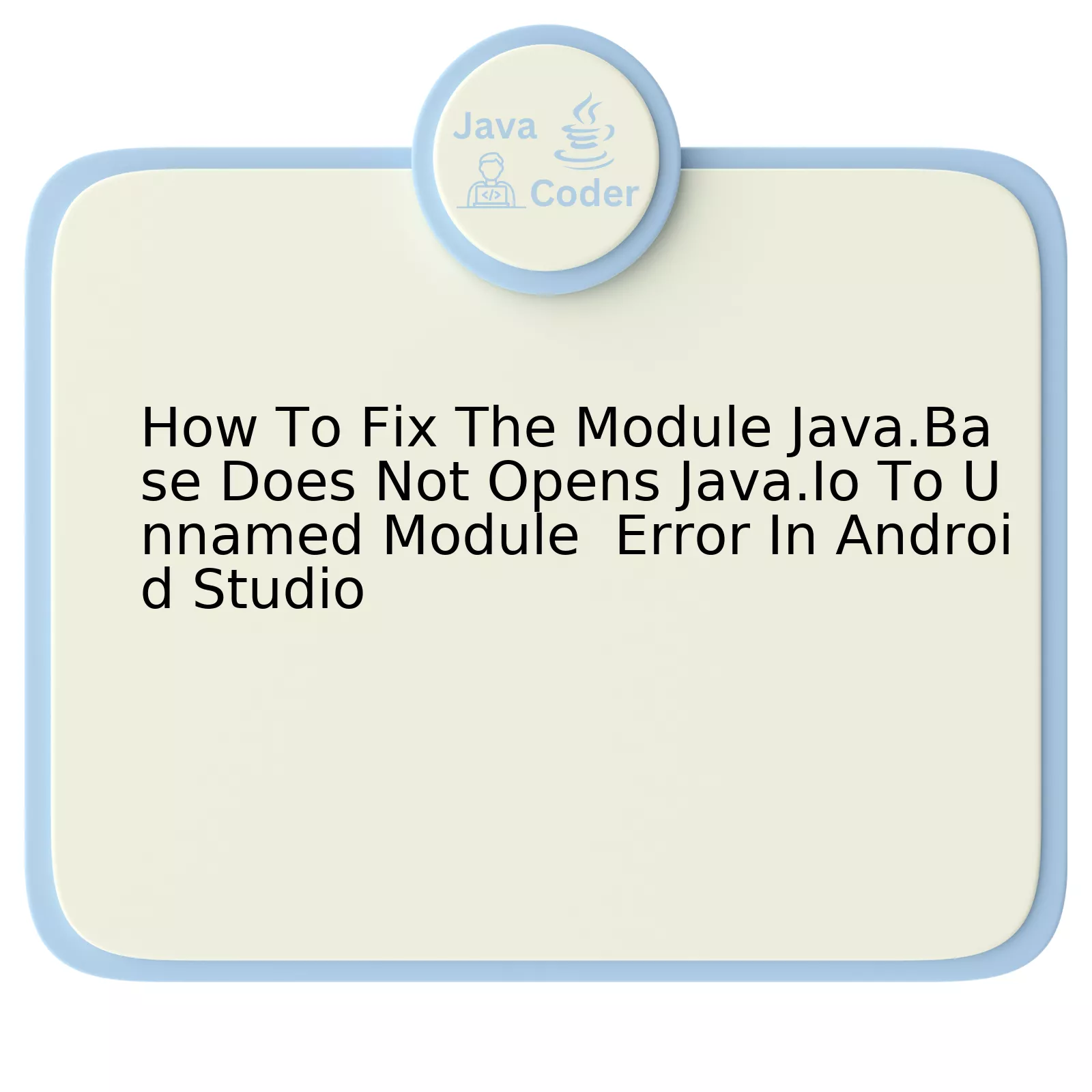
When one encounters an error stating “The module java.base does not open java.io to unnamed module” in Android Studio, it typically manifests as a result of the JDK (Java Development Kit) version compatibility issues. The following table delineates the steps required to resolve this problem.
Step | Action | Description |
---|---|---|
Verification | Confirm JDK Version | The preliminary action involves confirming the version of your system’s JDK. In Android Studio, navigate to “File > Project Structure > SDK Location”. |
Update | JDK Installation | If the JDK on your device is older, consider updating it to a recent version such as Java JDK 11. Proceed to the official Oracle website to download and install it. |
Configuration | Set JAVA_HOME Variable | Following installation, ensure your system recognizes the new JDK by setting the JAVA_HOME variable to point towards the directory where JDK 11 resides. |
Execution | Restart Android Studio | Lastly, execute Android Studio once more and this should rectify the said error. |
To provide further context:
Upon cross-verification of JDK version within Android Studio (via “File > Project Structure > SDK Location”), any outdated version may be the cause of this issue. Here upgrading to a newer JDK, for instance, JDK 11 becomes prudent. Fetching it from Oracle’s official site is recommended to avoid any potential complications.
When the updated JDK is installed, it is vital to guide the system to recognize its location accordingly. This is achieved by setting up the JAVA_HOME system variable. This set-up directs it to the corresponding directory where the recently updated JDK has been installed.
Finally, restart Android Studio after completing the abovementioned steps. Upon restarting, the manifestation of the “module java.base does not open java.io to unnamed module” error should cease.
Daniel Spiewak, a renowned developer and programmer once remarked, “Sometimes it’s better to leave something alone, to pause, and that’s very true of programming.” Dealing with errors can be tiring, yet it’s within these challenges that significant learning happens. Hence, think of these as opportunities for growth and mastery of programming concepts.
Understanding the “Module java.base does not opens java.io to unnamed module” Error
The error “Module java.base does not open java.io to unnamed module” typically surfaces while working with Java 9 and later versions due to the implementation of the Java Platform Module System (JPMS). This system introduces a new level of accessibility control, restricting access even to public resources, if they have not been specifically opened to other modules.
When working in Android Studio, an environment predominantly JVM-based, this error can be prompted by various circumstances. Here are possible causes and their corresponding solutions:
Cause 1: Using an outdated library or SDK that isn’t compatible with JPMS
Libraries, SDKs, or plugins written for Java 8 or earlier may attempt to access classes in `java.io` without requisite permissions, triggering this error.
Solution:
– Update your libraries or plugins to the newest versions. Most current releases are designed for compatibility with JPMS.
– If an update isn’t available, replace the library/plugin with an alternative, ensuring that it adheres to JPMS specifications.
Action | Command/Tasks |
---|---|
Updating Libraries |
dependencies { |
Cause 2: Reflection attempting Access Control rules circumvention
In Java, reflection can gather information about classes and manipulate fields, methods, and constructors of a class. Incorrectly utilized, this might violate the established access control rules of JPMS.
Solution:
Global opening of packages, while inadvisable due to security drawbacks, is a viable solution when debugging/testing or when no feasible alternative exists. You’ll accomplish it using the `–add-opens` command-line option, then proceed to specify the package and module to be opened thus:
--add-opens java.base/java.io=ALL-UNNAMED
In words reminiscent of Edsger Dijkstra’s wisdom, “Testing shows the presence, not the absence of bugs”. Always remember that solutions such as the ones provided above aren’t necessarily long-term fixes but measures aimed to understand better and, potentially, resolve the underlying issue.
Note: Online references frequently updating their content would provide an excellent study resource for keeping abreast with these types of Java/Android issues. Examples include leading tech forums like StackOverflow, specialized guides from Baeldung, or the prolific tutorials at Tutorialspoint.
Exploring Possible Causes of Unnamed Module Errors in Android Studio
One of the more common errors faced by Android Studio users is the “module java.base does not open java.io to unnamed module” error. This occurs when there’s an issue in the interaction between various modules within your Java code. Let’s delve deeper into this problem, identify possible reasons for its occurrence, and offer you analytical steps to resolve it effectively.
Understanding a Module Error
A module in Java is a named set of packages intended to be deployed together. These are designed to hold a certain group of related packages (classes) and resources inside them. When developers deal with the error ‘module java.base does not open java.io to unnamed module’, it usually points towards two interacting problems:
– The code could be trying to access a package in the java.base module (namely java.io), which isn’t shared with other modules.
– An unnamed module might be trying to use the java.io package, which hasn’t been opened up in the java.base module.
How To Fix The Error?
Here are suggested solutions for the issue “The module java.base does not opens java.io to unnamed module”:
- Usage of ‘–add-opens’: By launching the program with the flag `–add-opens java.base/java.io=ALL-UNNAMED`, we command Java to open java.io to all unnamed modules.
- Making use of Named Modules: Unnamed modules can lead to issues, as they don’t have a clear declaration of what they require or provide. Transitioning to named modules could avoid similar issues.
You’d add this in your Android Studio VM options as follows:
--add-opens java.base/java.io=ALL-UNNAMED
Example:
Given an unnamed module, an implicit readable edge is drawn from every named module back to the unnamed module. However, if we make our module a named one, things change.
module ProjectName { requires java.base; }
This will now grant us the permission to utilize classes from the java.base module.
Brian Goetz, Oracle’s Java Language Architect once said: “Modularity is the key to creating large programs made up of cohesive, loosely coupled modules.” Understanding such key aspects of modularity in Java applications can ultimately lead to smoother development processes and fewer runtime errors like the one discussed in this article.Source. Therefore, bear in mind that understanding and addressing module relationship obstacles proactively allows you to develop more efficient and resilient applications in Java and Android Studio.
While these recommendations should resolve the specific error addressed here, always remember that coding hurdles often spring from diverse sources. It’s crucial to familiarize yourself with the standard conventions and principles of Java programming for a comprehensive approach to debugging and trouble-shooting.
Effective Strategies for Resolving the Java.Base Module Issue
The
module java.base does not open java.io to unnamed module
error in Android Studio often arises due to complications within the specified module configuration. This error indicates that your Java module system demands special attention immediately to enhance its applications’ functionality and compatibility.
Java’s platform module system, launched with Java 9, essentially defined a different way to bundle applications and libraries. It provides a robust system of interconnecting modules, declaring dependencies, and giving a more secure encapsulation mechanism. Nonetheless, when handling legacy code or libraries not yet migrated to modules, you may encounter certain issues—such as “unnamed module” problems.
To troubleshoot this issue, consider applying the following strategies:
Modify Your Module Path:
Given error can be a result of running code which is neither in a named nor an automatic module. In such a scenario, if a library from the classpath reads classes from the modulepath (especially java.base), it triggers the error. Transitioning your libraries to the module path might resolve this issue. For instance, switching from classpath to modulepath in your IDE settings or build script can offer a solution.
Use –add-opens command:
Another strategy involves utilizing the
--add-opens
command line option that comes with JDK 9+. This solution explicitly instructs the JVM to break open the encapsulation of certain packages to unnamed modules. You would require to use the flag format
--add-opens java.base/java.io=ALL-UNNAMED
. Consider consulting the Java Tool Documentation for more information about the usage.
Leverage Automatic Modules:
Automatic modules render all of their packages open and export even reflective access. If it’s feasible to make the jars into automatic modules—by adding them to the modulepath instead of the classpath—it could solve the problem.
Upgrade Your Dependency Libraries:
Libraries frequently trigger the given error when they attempt to access core Java APIs reflectively. Frequently, these libraries have later versions compatible with the Java module system. So, a simple upgrade to a newer version might alleviate the problem.
As Brian Goetz, the eminent Java Language Architect at Oracle, once stated,
“The right thing is rarely the easy thing.”
Remember that solving these kinds of issues usually demands a bit of time and patience but paves the path towards sustainable and error-free development practices.
Keep in mind that any actions should maintain the readability, clarity, and efficiency of your codebase, as digging into quick hacks may lead to complexity down the line. Strive for systematic and comprehensive solutions that benefit your understanding of Java Modularity while keeping your Android Studio environment clean and functional.
Implementing Solutions: Debugging and Fixing The Module Error In Android Studio
When it comes to dealing with the `
java.base does not open java.io to unnamed module
` error, Android Studio offers a robust debugging apparatus that aids developers in tracing and rectifying errors methodically. The paramount focus when dealing with this issue is understanding why the issue occurs, possible solutions, and the implications of these solutions for your application.
At a high level, the `
java.base does not open java.io to unnamed module
` error highlights a conflict within your modules dependencies. Specifically, this error indicates that the `java.base` module does not open the `java.io` package to an unnamed module, which is oftentimes a result of running Java bytecode that uses features from later versions in earlier JVM versions. Essentially, the expected access restrictions are in place in the JVM not recognizing named modules.
This error can be addressed and resolved via different strategies:
• **Changes to the Module Path:** Traditionally, jar files used class paths for configuration. However, with newer Java versions running in modular mode, there may be conflicts between the older classpath mechanism and the new module system. Resolving such conflicts might involve moving the contested classes into a named module or changing their access levels.
// You can indicate to the JVM that you want all packages in the java.base module to be available to the unnamed module by using command-line flags as so: --add-opens java.base/java.io=ALL-UNNAMED
Utilizing the `–add-opens` flag informs the JVM to loosen its enforcement of access restrictions on the `java.io` package for unnamed modules, thereby resolving the issue.
• **Upgrading Your Project’s Dependency Versions:** If you’re using outdated libraries or dependencies, upgrading them might also help resolve this problem. Developers leveraging APIs implemented in later JDK versions might encounter a backwards compatibility issue when the bytecode tries accessing APIs using a features not recognized by the lower-versioned JVM. Hence updating would maintain compatibility.
“Writing the first 90 percent of a computer program takes 90 percent of the time. The remaining 10 percent also takes 90 percent of the time.” – N.J. Rubenking.
However, it’s important to note while using the `–add-opens` option or modifying your dependencies may act as a short term solution, experts advise moving towards named modules and away from using the classpath over long term, as the domain continues to move in the modularization direction. Therefore, refactoring your project to remove dependencies on the classpath and properly configure modules might be the best solution. This can be achieved by segregating your code base into well-defined, explicit modules—each encapsulating specific functionality—and maintaining clear, one-directional dependences among them.
The issue of “Module `java.base` does not open `java.io` to an unnamed module” is a common one that developers face when working with Android Studio. This occurrence often links to the improper configuration or mismatched JVM module versions in project settings.
The main solution revolves around ensuring you’re using the correct version of Java, such as Java 8 which offers more compatibility than the latest versions in dealing with this error. It’s also crucial to adjust your build settings and consider redirecting your reads through other allowed modules.
dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'androidx.appcompat:appcompat:1.1.0' implementation 'androidx.constraintlayout:constraintlayout:1.1.3' testImplementation 'junit:junit:4.+' androidTestImplementation 'androidx.test.ext:junit:1.1.1' androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0' }
In the example above, dependencies are defined to help mitigate any clashes between modules.
While fixing the problem, remember to bear in mind the words of Martin Fowler: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Thus, maintainable code should always be your priority.
For more insights on resolving Android Studio issues, explore Android Developers official site.