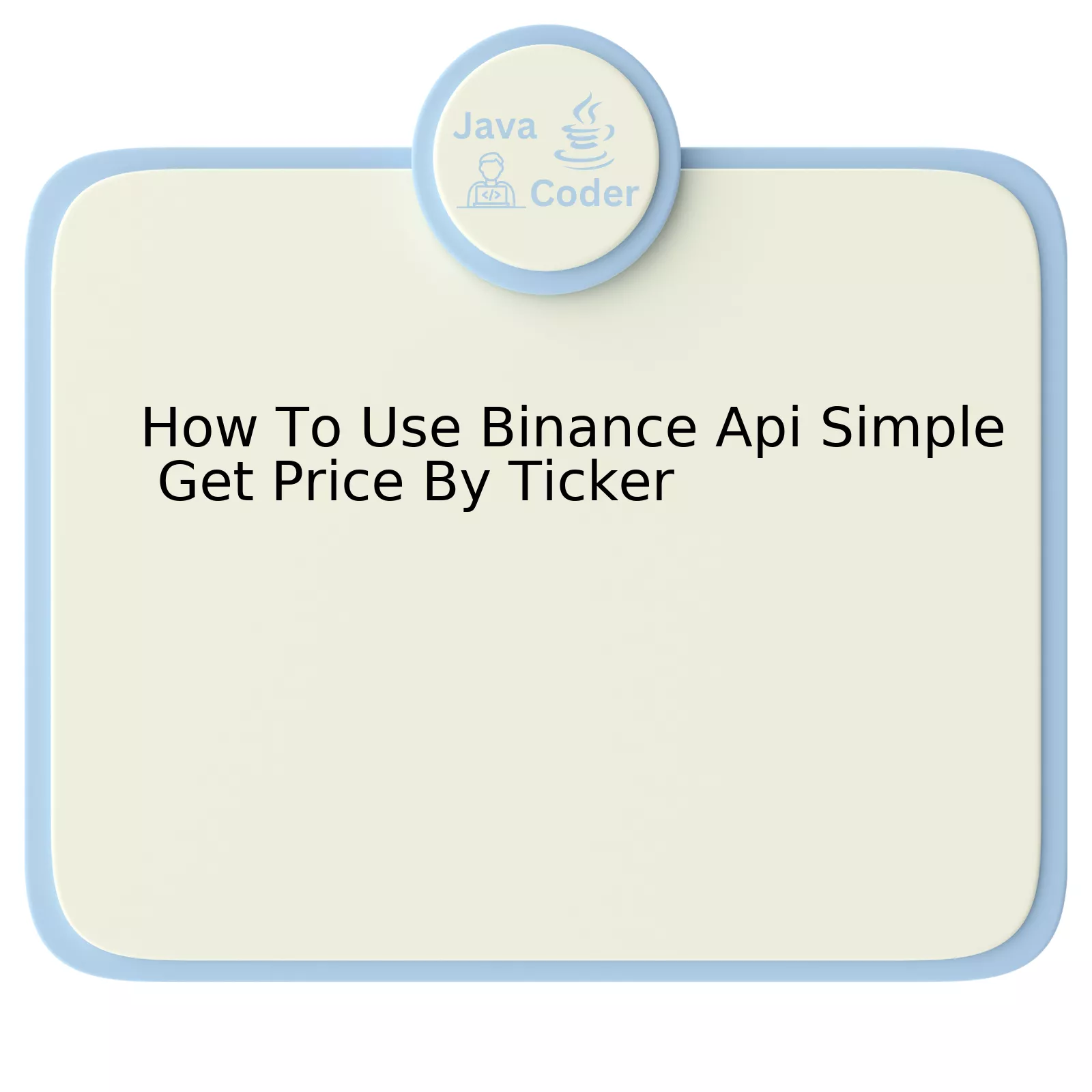
Let’s delve into the process of accessing price information via the Binance API. Here is a simplified representation of how it can be executed:
Step | Action |
---|---|
1 | Open an account on the Binance platform and generate your API key and Secret Key. |
2 | Instantiate BinanceApiRestClient using your keys. |
3 | Use get24HrPriceStatics() method, passing in the desired ticker as argument. |
After registering on the Binance platform, one can access the needed tools from the dashboard including generating personal API key and Secret Key. This secure pair of identifiers will give you access to utilize Binance’s API features.
You’ll employ your keys to create an instance of `BinanceApiRestClient`. This client serves as your gateway to the many functionalities available through Binance’s API.
The process of getting the price by ticker symbol involves calling the `get24HrPriceStatistics()` method associated with the client, specifying your intended cryptocurrency pair.
For instance, suppose we are interested in the current price of Bitcoin in terms of USD (BTC/USD), we could make use of the following snippet of code:
BinanceApiClientFactory factory = BinanceApiClientFactory.newInstance("YOUR_API_KEY", "YOUR_SECRET"); BinanceApiRestClient client = factory.newRestClient(); TickerStatistics tickerStatistics = client.get24HrPriceStatistics("BTCUSDT"); System.out.println(tickerStatistics.getLastPrice());
With this approach, the terminal will output the latest price statistics for the specified ticker.
Remembering Bill Gates’ words, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.”, the idea is to make such integrations undetectable and smooth for end users. The ability to fetch real-time currency data can significantly enhance financial or trading applications. The Binance API allows developers to seamlessly integrate this feature into their projects.
There are more details in the official [Binance API Documentation](https://github.com/binance/binance-spot-api-docs), providing a variety of methods to interact with their marketplace data.
Understanding Binance API: Decoding the Basics
The Binance API, a mechanism that allows for the interfacing of external applications with Binance’s trading platform, offers a broad selection of endpoints you can utilize. For this discussion, we’ll narrow our focus to one pivotal function: retrieving price details by Ticker via a GET request. This type of request is one of the simplest yet most fundamental aspects that novice and seasoned developers alike need when using the Binance API.
To put this process into perspective, think of it as using a messenger or intermediary to fetch the latest pricing data from Binance’s exchange database, thereby negating the necessity for direct interaction with Binance’s interface.
Let us consider how you can make use of this function:
Firstly, it is crucial to understand the structure of the GET request URL involved in order to retrieve price by ticker:
https://api.binance.com/api/v3/ticker/price?symbol=YOUR_SYMBOL_HERE
In this URL:
– “https://api.binance.com” serves as the base endpoint.
– “/api/v3” provides the specific version of the API being used – in this observance, we are utilizing Version 3.
– “/ticker/price” refers to the endpoint for receiving pricing details of a specific cryptocurrency.
– “?symbol=” is the part of the URL where you insert the symbol of the cryptocurrency whose price you’re seeking, such as BTCUSDT for Bitcoin in terms of USD(Tether).
Next on the list is constructing and sending the request compliantly. Depending on your programming language of choice, this process may vary slightly. This example is illustrated using Java:
HttpURLConnection connection = (HttpURLConnection) new URL("https://api.binance.com/api/v3/ticker/price?symbol=BTCUSDT").openConnection(); connection.setRequestMethod("GET"); BufferedReader binanceResponse = new BufferedReader(new InputStreamReader((connection.getInputStream()))); String responseLine; while ((responseLine = binanceResponse.readLine()) !=null){ System.out.println("\n Price Data: " + responseLine); } binanceResponse.close();
Here, each line encapsulates these actions:
– Establishes a connection to the given Binance API URL.
– Sets the request type to “GET”.
– Initiates reading the response from the server.
– Loops through the server’s response, printing out the price data obtained.
– Closes the connection reader once the operation is complete.
There is an evident gravity in understanding the fundamentals such as these basics of using the Binance API. As reiterated by Ada Lovelace, who is often regarded as the world’s first programmer, “That brain of mine is something more than merely mortal; as time will show.” Hence, like any technological implement, its utmost potential is unveiled not just by knowledge of its wide-ranging capabilities, but also through deep comprehension of its foundational principles.
Lastly, keep track of the changes in the API versions and refer back to the Binance API documentation frequently to ensure adherence to all conditions, standards, and conventions provided by Binance. This prophylactic measure ensures efficient, uninterrupted operations that coincide with the evolving face of cryptocurrency technology.
The Steps to Retrieve Price Information Using Binance API
In tackling the question on how to use Binance API to retrieve price information by ticker, it’s important to understand that the process involves making HTTP requests to endpoints defined in the Binance API documentation.
To obtain the price information of a particular asset (represented by its ticker), the following steps could be considered:
1. API Key Creation: Before interacting with Binance’s API, one will first have to create an API Key from the Binance exchange website. This key stands as a password granting access to Binance’s digital data and functions.
String apiKey = "your-api-key";
String apiSecret = "your-api-secret";
2. API EndPoint Selection: It is imperative to select the correct API endpoint. In this scenario, we utilize the "/api/v3/ticker/price" endpoint, allowing us to retrieve the price of a specific ticker.
<h1>Endpoint URL for retrieving price:</h1>
<p>https://api.binance.com/api/v3/ticker/price?symbol={tickerSymbol}</p>
Here "{tickerSymbol}" represents the symbol of a trading pair e.g., 'BTCUSDT' would represent Bitcoin to USDT pair.
3. Usage of HTTP Client Libraries: For Java, there are several HTTP client libraries available to perform HTTP requests, such as OkHttp, Retrofit, etc.
OkHttpClient client = new OkHttpClient();
4. Response Capture: Once a request is performed, the response data needs to be captured and processed.
Request request = new Request.Builder()
.url("https://api.binance.com/api/v3/ticker/price?symbol=BTCUSDT")
.build();
try (Response response = client.newCall(request).execute()) {
String res = response.body().string();
System.out.println(res);
}
As computer scientist Alan Kay said, "Simple things should be simple, complex things should be possible." These simplified steps show how even complex tasks such as using an API can be broken down into understandable piles, illustrating the beauty of programming and APIs.
Remember before accessing any functionalities of Binance API you must adhere to their usage policies to prevent unauthorized actions and protect your account.
For additional resources, visit the Binance API Documentation. It's a treasure trove of relevant information that would assist with insightful knowledge on different API endpoints, rate limits, and security practices.
Optimizing Your Use of the Simple Get Price Feature in Binance API
Excelling in your use of the Binance API Simple Get Price feature requires a grounding in key concepts and an in-depth understanding of methods for information extraction. In relevance to the topic of utilizing Binance API Simple Get price by ticker, the aim is to accomplish efficient and quick data procurement for particular cryptocurrency trades on Binance.
Here's how one can go about it:
Firstly, setting up your environment is crucial. There are many libraries that facilitate easier interaction with Binance API. An example would be using
binance-java-api
, a Java-based library which becomes almost indispensable when channeling requests through the Binance API.
java
import com.binance.api.client.BinanceApiClientFactory;
import com.binance.api.client.BinanceApiRestClient;
...
BinanceApiClientFactory factory = BinanceApiClientFactory.newInstance("YOUR_API_KEY", "YOUR_SECRET");
BinanceApiRestClient client = factory.newRestClient();
String price = client.getPrice("BTCUSDT");
System.out.println(price);
In this snippet, replace 'YOUR_API_KEY' and 'YOUR_SECRET' with your respective Binance credentials, and BTCUSDT with your required trade.
There is a noteworthy quote by Steve Jobs, "You can’t just ask customers what they want and then try to give that to them. By the time you get it built, they’ll want something new.". This emphasizes the fact that real-time data, like prices in the crypto market, are vital as they change rapidly. Users would always prefer updated data.
Secondly, understanding the data obtained is also important.
When you fire a getPrice request like shown above, the Binance server responds with a JSON object holding two fields - symbol and price. 'Symbol' carries the coin pair value e.g., 'BTCUSDT', while 'price' provides their last traded price on Binance.
Adept handling of the Binance API thus condenses to accessing real-time cryptocurrency trading data effectively.
Keep track of the rate limits Binance imposes on requests, to avoid IP bans. It is possible to make 1200 requests every minute from a single IP.
Choose wisely, whether your application should access data either synchronously or asynchronously, depending upon the user interface responsiveness and network efficiency.
Further reading available at Official Binance API Documentation
Troubleshooting Common Issues While Fetching Prices with Binance API
Accessing real-time prices is a crucial functionality in various financial applications. Binance API is a popular choice for this task due to its widespread use, stability, and comprehensive data coverage. However, it is not unusual to encounter typical problems while fetching asset prices with Binance API. While our focus is on using the Binance API to fetch prices by ticker in a simple way, awareness of these common issues can contribute to a more efficient problem-solving process.
A predominant obstacle developers face involves the encounter of errors or unexpected responses when making GET requests to the Binance API endpoint. Below are some probable reasons and corresponding solutions:
- Invalid API Key: One possible issue could be the invalidity of the API key. It's important to note that Binance API requires unique keys (both public and secret) generated from your Binance account. Thus, if the API key is missing, incorrect or expired, you'll likely receive error messages. To fix this issue, ensure to obtain valid keys from Binance and securely store them for use.
- Rate Limiting: Binance imposes certain restrictions on the frequency of requests to their servers, so as to prevent abuse. If your application sends too many requests within a short time, the API might respond with "429 Too Many Requests" status code. To address this, you may want to consider slowing down your requests or look into Binance’s ‘Weighted’ rate limiting system and adapt your request pattern accordingly.
- Outdated Endpoints: Sometimes maintenance updates could lead to changes in the Binance API endpoints. If your application is aiming at an expired endpoint, it can cause failures in fetching prices. Always keep track of latest updates and adjust your code to suit new changes.
In the context of fetching a price for a particular ticker symbol using Binance API, here is a rudimentary example of how this can be implemented:
html
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class Main {
public static void main(String[] args) throws Exception {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.binance.com/api/v3/ticker/price?symbol=BTCUSDT"))
.build();
HttpResponse
System.out.println(response.body());
}
}
The above Java code makes a HTTP GET request to the API endpoint that returns the current price for the BTC-USDT symbol.
Note: \''Make sure you replace 'BTCUSDT' with your specific ticker symbol in 'symbol'.
This answer is close to Jeff Atwood's point of view who believes that "Coding is not about building something, it’s about solving a problem in an elegant way". Understanding potential issues that you might stumble upon while trying to retrieve data via an API will help in arriving at more refined and reliable solutions wherever such APIs are deployed.
Your quest in understanding how to use Binance API to simply get the price by ticker has led you on an insightful journey. Understanding the Binance API is of critical importance in today's trading space, whether it's for algorithmic trading or data analysis.
The advantage of the Binance API gives developers and traders the power to interact with Binance's extensive database of cryptocurrency information. This includes access to real-time trading data, account management functionalities, and different trading operations.
On a fundamental level, Binance API works in three basic steps:
// Step 1 - Setting up the environment // Obtain your API Key and Secret Key from Binance // Step 2 - Making the request // Use an HTTPS client to send a GET request to the Binance API endpoint using your keys for authentication // Step 3 - Analysing the response // Parse through the returned JSON data to find the specific 'ticker' price you're looking for
However, the Binance API isn't just limited to getting prices by ticker. Your journey could take you to explore further functionalities of the Binance API such as order placements, withdrawals requests or even account updates.
The art and science of utilizing these APIs need not be daunting, as long as one has a clear understanding of REST principles and the language that they are working in. With Java, for instance, leveraging libraries like Unirest can greatly simplify this task. Here's an example of how:
Unirest.get("https://api.binance.com/api/v3/ticker/price") .queryString("symbol","BTCUSDT") .asJson();
With this knowledge under your belt, journeying into the world of algorithmic trading or advanced data analytics will not seem as formidable as it might have when you first started out. From here, it’s now feasible to build sophisticated trading algorithms, manage multiple accounts, or investigate market trends undeterred.
William Gibson once rightly said, "The future is already here — it's just not very evenly distributed." With the pace of innovation in fields like AI and blockchain technology, more and more complex problems are continually being solved with creative and elegant solutions. Understanding and applying these advancements, such as the Binance API, is a significant step towards being part of that future.