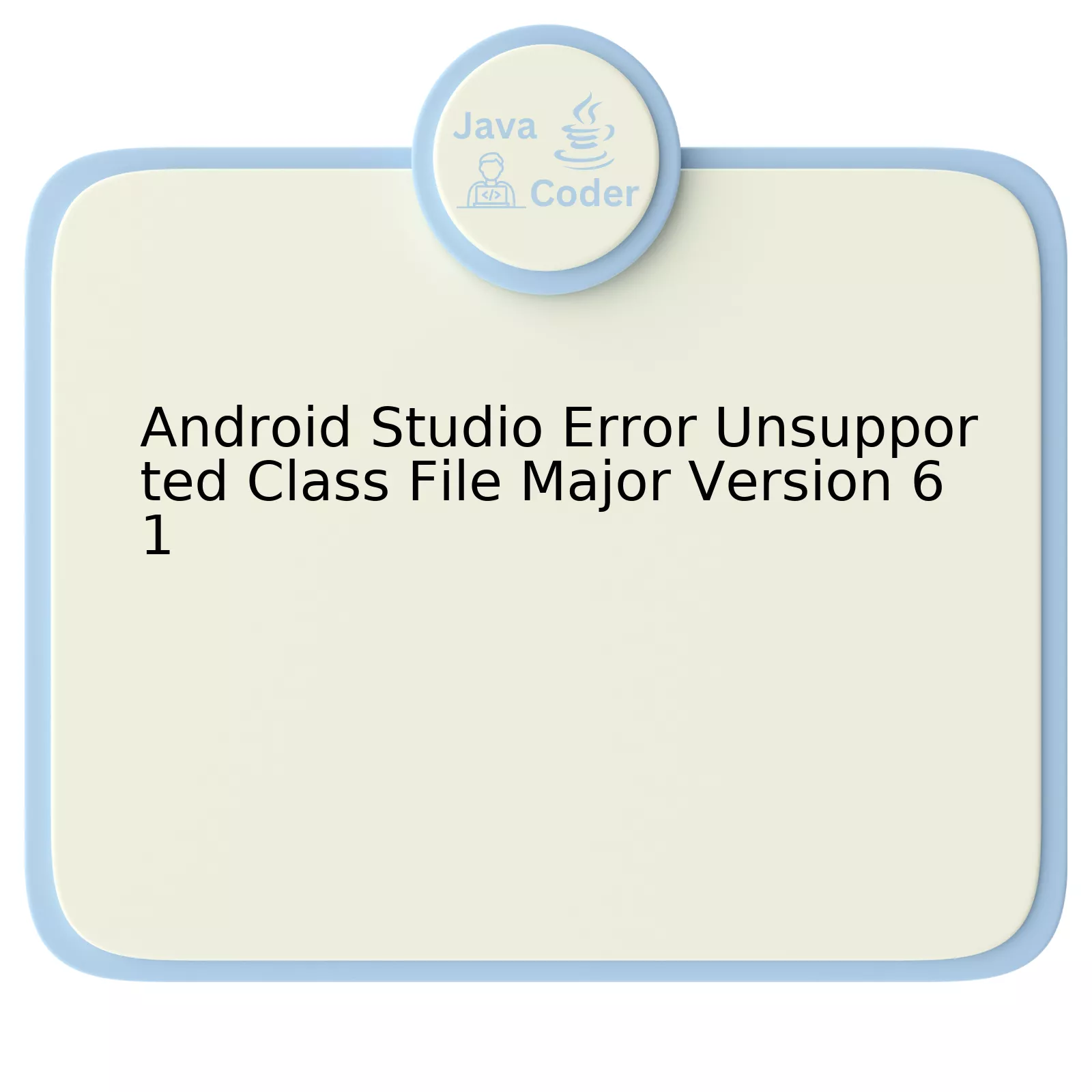
Error | Reason | Solution |
---|---|---|
Android Studio Error Unsupported Class File Major Version 61 | An outdated JDK/JRE, or conflict between Android Studio and a newer version of JDK/JRE. | Update JDK/JRE or adjust Android Studio’s settings to use a compatible version. |
More specifically, the “Unsupported Class File Major Version 61” error is generated when Android Studio encounters a Java class file compiled with a more recent version of the JDK (Java Development Kit) than what it understands through its internal JRE (Java Runtime Environment). This happens due to the numbered structure of JDK/JRE. The ‘major version’ number reflects the JDK’s release version.
For instance, JDK 15 corresponds to major version 59; whereas, JDK 17 equates to major version 61 in question. Thus, if your Android Studio is set up to work with an older version, such as JDK 15, but you compile a class with JDK 17, it causes a clash leading to this error.
Following are two potential solutions:
1. Upgrade your JDK: You can handle this issue by updating your JDK to the necessary version (in this case, version 61 correlating to JDK 17). This step ensures that the versions of JDK used for compiling your code and the one recognized by your Android Studio are in sync – no clash occurs.
2. Adjust Android Studio settings: If upgrading JDK does not serve your purpose (perhaps due to restrictions on your project requiring an older JDK), then you need to instruct Android Studio to use a specific JDK version instead. To do this, modify the ‘Gradle JDK’ setting under ‘Build, Execution, Deployment -> Build Tools -> Gradle.’
As Michael Feathers, a renowned software developer and author, once stated, “Even bad code can function, but if the code isn’t clean, it can bring a development organization to its knees.” It is crucial in programming to comprehend and rectify errors with precision to maintain clean code. (source)
Understanding the Android Studio Error: Unsupported Class File Major Version 61
Your addressing the issue with Android Studio Error: Unsupported Class File Major Version 61 indicates that you’re using a Java version high (for example, Java 17), and it’s not well-compatible with your Android Studio setup. Android Studio presently supports JDK 8 through to JDK 11.
The error it throws is essentially informing you that it does not support the class file’s version; this is produced when a higher version of JDK compiles a java file.
There are several strategic steps you can take to resolve these Android Studio Errors:
Problem | Solution |
---|---|
Android Studio JDK version not compatible | You can try downgrading your JDK from its current version to JDK 11 or below. Remember to set the JAVA_HOME environment variable to the path of your new JDK installation after changing the JDK. |
Gradle uses different JDK | In Android Studio, navigate to “File” – “Settings” – “Build, Execution, Deployment” – “Build Tools” – “Gradle”. Under Gradle JDK, change to a version that’s well-compatible like JDK 11. You can also locate your gradle.properties file in Android Studio and ensure that org.gradle.java.home is set to your preferred JDK location. |
JDK Command Line Tool uses higher JDK | Check if your command line tool has been set to use a higher JDK. If so, consider altering the JAVA_HOME environment variable to align with a JDK version that’s compatible. |
The infographic above provides possible origin points of the problem and viable paths towards resolution. It’s important to understand that these means of rectification relate to two primary factors: software compatibility and version alignment.
Also as Brian Kernighan, one of the original contributors to UNIX and the co-creator of AWK and AMPL programming languages, once said:
"Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it."
This quote emphasizes the essence of debugging which indeed entails meticulous examination of code compliance, software configuration, even operating system specifications. By effectively unifying all these aspects, you’ll be able to resolve the Android Studio Error: Unsupported Class File Major Version 61.
For further reading on understanding JDK errors, follow this link.
Various Causes of ‘Unsupported Class File Major Version 61’ Error in Android Studio
The error message ‘Unsupported Class File Major Version 61’ is common in Android Studio when you’re using a JDK version that your Android Gradle plugin hasn’t yet started supporting. Java developers often face this sort of challenge.
Android Studio utilizes the Gradle plugin for building and testing Android applications. However, upgrading to an unsupported JDK may likely cause such errors because the new JDK features result in a class file with a newer major.minor version.
Java SE | Major Version |
---|---|
SE 16 | 60 |
SE 17 | 61 |
Reasons for this error’s occurrence include:
1. **A recent upgrade to JDK 17**: Often, when a developer updates their JDK, there might be compatibility issues like we see here.
2. **Multiple Java Development Kits**: There may be multiple directions to the JDK from within your computer system or server, causing confusion about which one to use.
3. **Gradle Version**: Your compatible Gradle version may not support the newer JDK.
To resolve this, consider falling back to a JDK version supported by Android Studio, typically JDK 11 or below. Here’s an example of how to downgrade JDK in Android Studio:
Alternatively, maintain separate JDK installations for different projects demanding divergent versions of the JDK.
It’s appropriate to quote James Gosling, the father of Java – “One of the things we wanted to do in Java was to make it so that people could write programs that could run anywhere.”
Linking the concepts referred in the answer:
• [Gradle Plugin](https://docs.gradle.org/current/userguide/plugin_reference.html)
• [Java Development Kit (JDK)](https://www.oracle.com/java/technologies/javase-jdk11-downloads.html)
This ensures a uniform experience irrespective of the underlying infrastructure, reflecting the intent behind the popular slogan—’Write Once, Run Anywhere.’ The same should be expected while handling different JDK versions within our development environments, including the error mentioned: ‘Unsupported Class File Major Version 61’.
Best Practices for Troubleshooting ‘Unsupported Class File Major Version 61’ Error
Fixing the ‘Unsupported Class File Major Version 61’ Error in Android Studio can be challenging. Threat not, following some best practices can certainly expedite the resolution process.
Understanding the Problem
Android Studio is an Integrated Development Environment (IDE) for developing Android apps and is based on the JetBrains’ IntelliJ IDEA software. The error ‘Unsupported Class File Major Version 61’ typically happens when you are using a higher version of JDK than what your Android Studio supports. JDKs newer than version 11, to be precise.
It is essential to note that each JDK has a major-minor version number associated with class files, indicating the compiler version used. JDK 16 corresponds to Major version 60, and JDK 17 corresponds to Major version 61, which is unsupported by Android Studio. If this is the case, you need to configure Android Studio to use a JDK compatible with it.
Changing the SDK in Project Structure
The first method to resolve the issue would be changing your Project Structure settings. Navigate to
File -> Project Structure -> Project
. From there, change the ‘Project SDK’ to a supported JDK version.
It should look something like:
project {
…
compileSdkVersion 22
buildToolsVersion “22.0.1”
defaultConfig {
…
minSdkVersion 15
targetSdkVersion 22
…
}
…
}
Modifying gradle.properties
You can also specify the JDK version in gradle.properties file. Add/change the line in or create a new file called
gradle.properties
, add this:
org.gradle.java.home=C:\\Program Files\\Java\\jdk-11.0.3
Note: Make sure to replace the path with your exact path to JDK 11.
Invalidate Caches/Restart
Sometimes, the issue may persist due to cache-related problems. In such a case, clearing the cache offers a plausible solution. Go to
File -> Invalidate Caches / Restart...
and confirm your action.
To quote Mike Acton, Engine Director at Insomniac games – “First, solve the problem. Then, write the code.”, it’s important to figure out a system of diagnosing and solving coding issues swiftly, enhancing productivity over time.
For more details about Java SE’s supported versions, you can refer [here](https://www.oracle.com/java/technologies/javase-products.html). The above-discussed steps should hopefully resolve the ‘Unsupported Class File Major Version 61’ error experienced in Android Studio.
Detailed Guide on Preventing Android Studio Errors: Focusing on ‘Unsupported Class File Major Version 61’
Understanding the Android Studio Error “Unsupported Class File Major Version 61” involves comprehending that it’s a lifecycle compatibility issue between different versions of Java – a widely used high-level, class-based, object-oriented programming language. There could be various causes for this problem to occur and several ways to resolve it.
What Causes The ‘Unsupported Class File Major Version 61’ Error
The ‘Unsupported Class File Major Version 61’ error is generated due to an inconsistency in Java version compatibility – JDK (Java Development Kit) and JRE (Java Runtime Environment). This may happen when your environment is using a lower Java version and attempting to run a class file compiled with a higher version. For instance, if you’re using Java 11 and trying to use a library or package compiled with Java 17, as the class file structure has changed over newer versions of Java.
How to Resolve The Error
Fixing this error involves aligning the version of Java used by both Android Studio and your system’s Java installation. Here are the steps to accomplish this:
- Step 1: Identify the Java version used by Android Studio. This can be seen under
File > Project Structure > SDK Location
.
- Step 2: Confirm the system’s default Java installation by running
java -version
in a terminal/command prompt.
- Step 3: If the versions do not match, then you need to update your system’s JDK or switch to a compatible version. You could download and install it from the official Oracle website.
- Step 4: Once installed, reset the JAVA_HOME environment variable to point to the new JDK’s directory.
- Step 5: Restart Android Studio so it picks up the correct Java version.
As Linus Torvalds, the mastermind behind Linux says: “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” While resolving errors can sometimes be frustrating, every solved issue leads us to become better developers.
Maintaining Compatibility
Beyond just solving the error, it’s essential to ensure backward compatibility with older versions of Java. It’s best practice to use the lowest Java version that your project can support to maintain compatibility with as many target systems as possible. In Android Studio, this can be set by navigating to
File > Settings > Build, Execution, Deployment > Compiler > Java Compiler
and setting the bytecode version to a preferably lower version of Java (like Java 8), considering the backward compatibility feature.
Conclusion
Staying updated about the compatibility requirements between your software modules and troubleshooting any discrepancy lies at the core of nuanced software development. Remember, “The only way to learn a new programming language is by writing programs in it.” – Dennis Ritchie
Understanding the heart of the “Android Studio Error Unsupported Class File Major Version 61” involves digging into the fundamental components of Java system development. This specific issue arises primarily due to a mismatch between different Java versions, particularly Java JDK 16 and its compatibility with Android Gradle plugin version 4.2.1 or lower.
public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } }
Now, let’s discuss this problem in details:
– An Introduction to Java Class File Version: In the world of coding, every class file has an associated “major version,” which depicts its compatibility with various Java versions. When we encounter the “Unsupported Class File Major Version 61,” it indicates that the class file was compiled with Java 17, but you are attempting to execute it on a lesser version, possibly Java 16 or lower. Consequently, issues dawn because the previous JDKs may not support some features provided by their newer counterparts.
– The Role of Android Studio: As a widely adopted Integrated Development Environment (IDE) for developing Android Apps, Android Studio largely relies on Java for building dynamically innovative applications; hence Java compatibility plays a paramount role here. However, when Android Studio experiences challenges deciphering the major classfile version of Java, the resultant is the “Unsupported Class Version Error”.
This issue can be rectified by considering the following points:
– Ascertain Java SDK Version: It’s critical to verify your system’ Java Software Development Kit version, ensuring harmonization with the most recent stable release. Checking java version can easily be done via your system’s command prompt or terminal using the command –
java -version
.
– Update Android Gradle Plugin: If you’re working on an older version of Android Gradle plugin, consider updating it to version 7.0.0 or higher. Though doing so might necessitate you to upgrade to Android Studio Arctic Fox (2020.3.1).
In the words of Jamie Zawinski, a reputed programmer, “Some people, when confronted with a problem, think ‘I know, I’ll use regular expressions.’ Now they have two problems.” Even though the quote sounds playful, it remarkably underlines how choosing the right tool or version accordantly assists in solving a problem expeditiously vis-a-vis creating another one due to incompatibility.
For more detailed information on updating Gradle and potential impacts, see Gradle plugin release notes.
By unraveling these aspects of error related to class file versions, this deep-dive discussion should assist users encountering the “Android Studio Error Unsupported Class File Major Version 61”, offering practical solutions and insights into preventing such hitches in future projects.