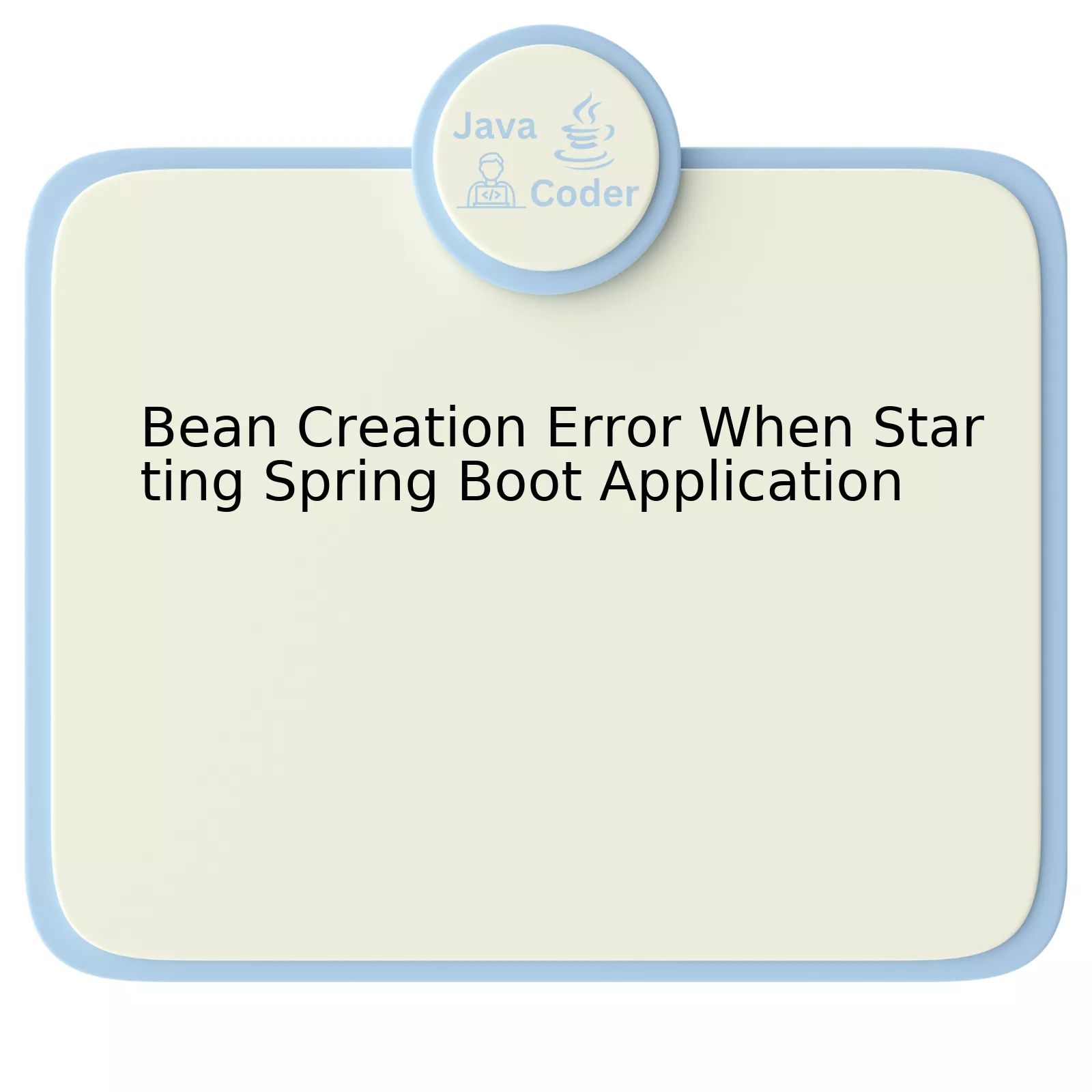
When discussing Bean Creation Errors during Spring Boot Application start-up, there could be various reasons causing these errors. For instance:
Trouble Area | Possible Cause |
---|---|
Code Structure | Incorrect use of Annotations or a missing Class Initialization. |
Dependencies | Misaligned Libraries or Version Conflict between the Dependencies. |
Configuration | Improper Java Configuration or issues in Property file values. |
Database Connection | Lack of Database Connection due to improper credentials on property file. |
The table provides a high-level view of various challenges leading to Bean creation errors. Evaluating each can help uncover potential issues disrupting your Spring Boot Application initiation.
A closer investigation into these areas unveils an array of considerations for Spring Boot developers:
- Regarding Code Structure: Ensure correct use of annotations like @Component, @Repository, @Service, or @Controller. These annotations are instrumental in class initialization; without proper implementation, Beans will not be correctly initialized.
- On Dependencies: It’s crucial to check all libraries and their versions. Sometimes, one library relies on an old version of another, causing conflicts between dependencies. Using build tools like Maven or Gradle ensures appropriate library management, mitigating version conflicts.
- Focusing on Configuration: Configuration problems typically revolve around Java Configurations or Property file values. Validate the correctness of your @Beans in Configuration class (like spelling mistakes, incorrect method names, etc.) Also, review your property files for consistency and correctness.
- About Database Connection: Often, a lack of database connection also results in Bean creation error. In such cases, recheck your database drivers and connection details in your properties file, ensuring they reflect accurate credentials and connection strings.
To address the Bean creation error, it is usually helpful to investigate the stack trace and locate the bottom-most error which usually gives you the root cause of the issue.
As Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it”. Hence, using analytical thinking to solve these Bean creation errors might get to the root cause more effectively.
Understanding the Bean Creation Error in Spring Boot Applications
When optimizing your Spring Boot application performance, becoming adept at diagnosing and troubleshooting Bean creation errors is an integral aspect. Two primary categories define these errors:
1.
BeanInstantiationException
: This error typically occurs when the framework fails to instantiate a bean class, perhaps due to a missing constructor or other issues related to bean definition.
2.
NoSuchBeanDefinitionException
: Represents a failure to locate a bean that matches the criteria provided, such as unable to find any beans of expected type within the Spring container.
To diagnose Bean creation errors in Spring Boot applications proficiently, maintain focus on three principal areas:
Error Logging Symptomatology
Unraveling the root cause of any bug begins with meticulously observing log outputs. Detailed logs are especially helpful when understanding vague error messages like “Error creating bean with name ‘exampleComponent’ defined in file [./example/ExampleComponent.class]”“.
“Logs are the best place to start hunting down bugs.” — Brendan Gregg, Senior Performance Architect at Netflix
Accurate Bean Definition
Incorrect Bean definitions often result in
BeanInstantiationException
. The crux zeroes into ensuring constructors, autowired fields, and methods are well-defined. For example, when incorporating a parameterized constructor, do not forget to provide an empty constructor unless using the
@Autowired
for the parameterized constructor explicitly.
Ensuring Bean Availability
NoSuchBeanDefinitionException
often originates from unsatisfied bean dependencies. If a bean ‘B’ depends on another bean ‘A’, ensure that ‘A’ is defined and initialized before ‘B’. Utilizing the lazy initialization feature of Spring Boot can potentially cause this problem, especially if beans are not configured correctly.
As much as programming instrumentations worked relentlessly to make debugging hassle-free, Bean Creation Errors might sometimes seem slightly convoluted, particularly to beginners. However, embracing debugging skills is vital—painful corners of software development were it not for the embedded insight-rich diagnostic tools.
Here’s a simple adjustment to your code that could help sidestep common pitfalls that trigger bean-creation errors:
bstineman @Before public void setUp() { context = SpringApplication.run(Application.class); }
This block ensures the Spring ApplicationContext is fully constructed before running any tests. Such approach helps mitigate potential timing issues linked to misconfigured bean dependencies leading to errors.
Echoing the words of Linus Torvalds, creator of Linux and Git –
“Talk is cheap. Show me the code!”
Remember, good coding habits coupled with adequate knowledge of how Spring Beans work can minimize the frequency of encountering these errors, thereby enhancing application stability and reliability.
Feel free to consult the comprehensive Spring documentation for more information about handling Beans creation in Spring Boot.
Delving into Potential Causes of a Spring Boot Application Start-Up Failure
When we talk about the beans failure to create in a Spring Boot application, it’s tantamount to a detective piecing together clues until they locate the root cause. This error is quite common and can be attributed to a variety of reasons that need to be addressed thoroughly.
BeanNotOfRequiredTypeException
This error occurs when a specified bean cannot be cast to the required type. For example, you may have mistakenly attempted to inject a
UserRepository
into a
UserService
, but instead defined
UserService
instance in your configuration classes.
<beans> <bean id="userService" class="com.example.UserService"> <property name="userRepository" ref="userRepository"/> </bean> <bean id="userRepository" class="com.example.UserRepository"/> &tl/beans>
UnsatisfiedDependencyException
This issue arises when you require a particular bean but the framework fails to meet your dependency needs; i.e., the needed bean was likely not created or properly configured. For example, there could be an explicit instruction somewhere for the creation of an
OrderRepository
which does not get created due to some misconfigurations in the Bean creation process.
NoUniqueBeanDefinitionException
This error indicates that more than one eligible bean could satisfy the dependency. For instance, if two implementations share an interface and there’s no clear indication as which to choose for autowiring, then this exception will occur.
BeanCreationNotAllowedException
It implies bean creation is prohibited since the Beans factory is already shut down. A classic scenario is when you attempt to compile non-lazy singleton beans using
getBeans
methods during the destruction phase of the Beans Factory.
“Hence, make sure you understand the lifecycle events in the Spring Application better before dealing with this specific error” – Oliver Gierke, Spring Data Project Lead.
BeanDefinitionStoreException
The difficulty comes from an invalid bean definition: perhaps due to XML syntax problems or incorrect annotations. Be sure to carefully review your bean definitions to avoid this.
To sum up, Spring Boot facilitates seamless application development by managing bean creation and injection. However, being familiar with potential causes of failure, such as those detailed above, covers you should any issues arise during Spring Boot application startup. Often, these problems can be managed by careful attention to details such as precise naming conventions, annotation use, and planned configuration.
Troubleshooting Guide: Resolving the Bean Creation Error
A ”
BeanCreationException
” is a commonly encountered exception when working with Spring Boot applications. It usually occurs during application startup and means that the Spring Framework failed to create a bean – a basic building block of any Spring Boot Application.
To understand how this error occurs, we need to grasp the concept of Beans in the context of Spring Boot Applications. In simple terms, a Bean is an object that is instantiated, assembled, and managed by Spring IoC (Inversion of Control) container. These beans are created with the configuration metadata that provides to the Spring IoC container through either XML-based or Annotation-Based Configuration.
Now let’s focus on identifying and resolving the Bean Creation Error:
Identification of the Error:
The identification process of the ”
BeanCreationException
” is relatively straightforward. The exception stack trace should explicitly state it. Here’s an example of what it might look like:
org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'beanName': Injection of autowired dependencies failed; nested exception is org.springframework.beans.factory.BeanCreationException: Could not autowire field...
Possible Causes and Solutions:
Below are the most common causes and solutions for a ”
BeanCreationException
”
– Mismatching between the names of Beans: This occurs when you give the wrong name for a Bean in your configuration compared to the actual defined Bean. Always ensure that the given bean name matches the correct class or method responsible for creating the instance of that bean.
– Autowiring issues: This issue often happens when you’re trying to
@Autowire
a bean that Spring Boot doesn’t know about, perhaps because you didn’t annotate it with
@Component
,
@Service
,
@Controller
, etc., or omitted to include the package from the scan. Make sure to annotate your classes and check your packages scan configuration.
– Dependency conflicts: If there are different versions of the same dependency being pulled in by the build system (like Maven or Gradle), Spring Boot might fail at creating a bean because of incompatible classes or methods in these versions. You can resolve this with the appropriate version management in your build system configuration.
– Circular Dependency: Circular dependencies occur whenever you have a cyclic relationship between your beans, which makes it impossible for Spring to determine which Bean should be initialized first. In such a situation, one option is to set one of the beans to have a scope prototype. Another is the usage of
@Lazy
annotation.
Remember, the stack trace will be one of your greatest weapons for understanding and diagnosing ”
BeanCreationException
“. It’ll guide you towards the cause of the problem. As Alan Lakein quoted, “Planning is bringing the future into the present so that you can do something about it now.” Understanding what is causing your Spring Boot application to throw a BeanCreationException is the first step towards resolving it. For more detailed information about Bean creation, you can visit the Spring Boot Official Documentation.
Implementing Solutions and Prevention Strategies for Future Errors
Bean creation error when starting a Spring Boot application is quite common and often pertains to the “initialization of bean failed” problem. This could result from various factors such as incorrect configuration or an implementation flaw, in turn preventing the Spring container from initializing the bean properly. Delving deeper into this issue would engender a more robust understanding, thereby helping to implement reliable solutions and cultivate proactive prevention strategies for future errors.
Error Trigger Factors and Strategies
Misconfigurations, naming conflicts, dependencies not being met, implementation issues, etc., may trigger Bean Creation Error. Understanding these triggers can considerably ease the troubleshooting process.
- Incorrect Annotations: One major cause is using the wrong annotations or improperly configuring them.
- Naming Conflicts: Implicit or explicit naming conflicts between different beans could also be contributing to this error.
- Dependency Conflicts: If bean A depends on bean B and bean B has not been correctly initialized or defined, then the initialization of bean A that depends on it also fails.
- Implementation Flaws: Incorrectly implemented code, bad practices, or lack of required parameters during instantiation can also lead to this issue.
Solution Implementation
// Correction in annotation @Component("sampleBean") public class SampleBean ... // Correction in naming public interface DataSource { .. } @Component("dataSourceImpl") public class DataSourceImpl implements DataSource ... // Dependency correction @Autowired private SomeClass someClass; // Implementing required parameters public MyClass(String requiredParam) { // your initialization logic }
Link Related : [Spring Annotation](https://docs.spring.io/spring-framework/docs/current/reference/html/core.html#beans-annotation-config)
Each issue mentioned above can be addressed by deploying specific solutions:
- Incorrect Annotations: Always ensure correct usage and placement of annotations. Providing appropriate names through attributes can prove useful.
- Naming Conflicts: To rule out naming conflicts, utilize clear names that provide context. Custom names can be assigned via attributes within certain annotations. If an ambiguity persists despite everything, consider using
@Qualifier
annotation.
- Dependency Problems: When dealing with dependencies, adopt Autowiring the dependencies.
- Implementation Flaws:Execution of best coding practices is advisable – this includes utilizing constructs such as Constructors and methods efficiently and fulfilling any prerequisite instantiation requirements.
Preventive Measures
Quote by Benjamin Franklin “An ounce of prevention is worth a pound of cure” speaks volumes about the importance of foreseeing potential issue triggers and dismantling them beforehand.
Strategies | Benefits |
---|---|
Implementing error handling mechanism | Prompt identification and treatment of errors |
Code verification through regular testing | Identification and rectification of defects |
Applying best coding and design practices | Avoidance of triggering factors leading to bean initialization failure |
Overall, eliminating the underlying causes and chalking out preventive strategies before the onset of an issue plays instrumental role in maintaining a bug-free environment, ultimately aiding a smooth Spring Boot application run.
Addressing the “Bean Creation Error When Starting Spring Boot Application” error is of significant importance, given its potential effect on running applications in a hitch-free manner. The nature and complexity of this issue may vary depending largely on various factors brought to the fore within the context of the application.
Firstly, an understanding of what Spring Boot does under the hood is crucial. Spring Boot engineers have designed it to automatically configure your Spring Application based on the jar dependencies you added in the project. The Spring Framework takes care of the creation and management of beans, which are objects that form the backbone of a user’s application. These beans are created via a configuration metadata supplied by the developer, such as XML, annotation-based configuration or java-based methods.
However, sometimes encountered is the “Bean Creation Error”, due usually to some form of conflict or incorrect setup in the application. Here’s one possible solution, making use of the
Application.java
file:
@SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } }
This snippet signifies a central class for Spring Boot applications. This class should always be kept clean and free from any business logic. If your Application class is cluttered with additional annotations or functionality, relocation of these might just solve your Bean Creation Error.
Let’s focus on another common situation. Sometimes, developers inadvertently create two beans of the same type, leading to a conflict. Fortunately, Spring gives developers the tools to handle this. If you realize this is your scenario after revisiting your code, consider using the
@Primary
annotation to specify which bean should take precedence:
@Service @Primary public class PrimaryBeanClass implements BeanInterface { ... }
In this circumstance, Spring will give priority to PrimaryBeanClass whenever multiple implementations of BeanInterface are present.
Lastly, errors relating to “NoSuchBeanDefinitionException” could happen if you’re trying to use a bean before it has been fully initialized. The
@DependsOn
annotation can help manage such inter-bean dependencies effectively. For instance:
@Bean @DependsOn("otherBean") public MyBean myBean() { return new MyBean(); }
Here, “MyBean” won’t be initialized until “otherBean” has been set-up completely.
Optimizing and troubleshooting the process of bean creation essentially aims at promoting seamless interactions among different components within any given Spring Boot application. This ensures efficiency, stability, and consistency in practically driving both the operational and supportive functionalities therein. Check out this article from Baeldung for more nuanced insights about handling Spring’s “NoSuchBeanDefinitionException”.
“A good software engineer doesn’t debug their program; they verify it.” – commenter on Stack Overflow
The above discussion marks a great step in dealing with the “Bean Creation Error When Starting Spring Boot Application”. Remember, software development isn’t just about getting things done. More importantly, it’s about getting things done right!