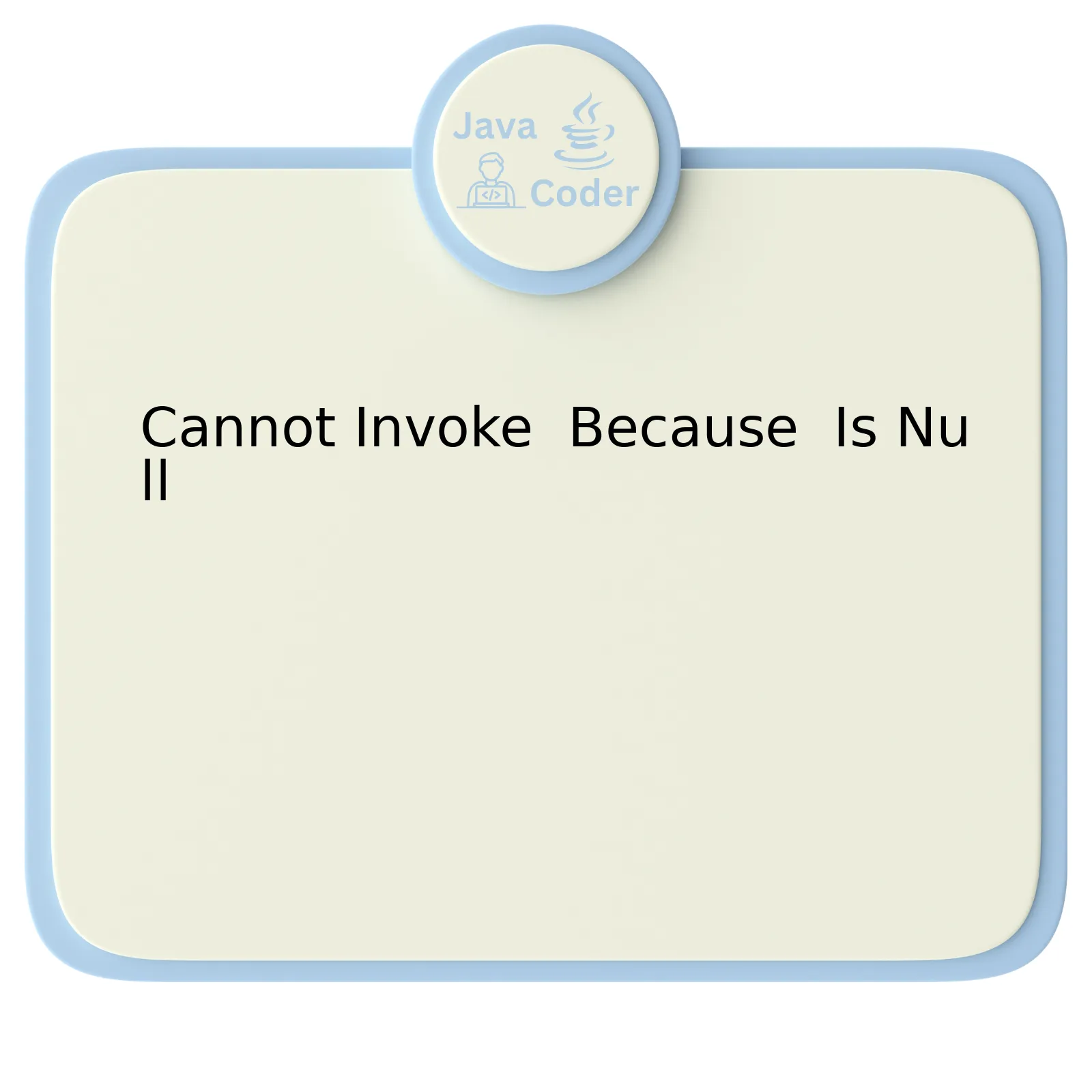
Error Scenario | Problem Statement | Solution Approach |
---|---|---|
Call on Object Method | The program attempts to call a method on an object which is currently null. This results in a
NullPointerException . |
Initialize the object before calling its method or use null-check before attempting to invoke a method. |
Accessing Object Attribute | An attempt is made to access or modify an attribute of a null object. | Ensure that objects are properly initialized before accessing their attributes. Also, ensure you have necessary checks to avoid
NullPointerException . |
The “Cannot Invoke Because Is Null” error message is typically encountered when we attempt to invoke a method or to access an attribute of a null object in Java. A null denotes the absence of any instance therefore, trying to call a method on it or accessing an attribute results in what’s known as ‘NullPointerException’.
As illustrated in the table, there are two common scenarios where this problem may occur:
- Call on Object Method: If we haven’t properly initialized an object and then we try to call one of its methods, we encounter this error because technically, we’re asking the program to perform a function on nothing.
- Accessing Object Attributes: Similar to the previous case, if we try to access or modify an attribute of a non-initialized (null) object, we get this error.
Java example:
// Assuming Employee is a class with some method sayHello() Employee employee = null; employee.sayHello(); // NullPointerException is thrown since employee is null
To prevent the occurrence of such errors, we should always initialize objects before calling their methods or accessing their attributes. Additionally, we can also employ null-checks to ascertain whether an object is null before attempting to invoke a method on it.
“Null Pointer Exception is like a civil war happening inside the system and no matter how hard you pretend, external affairs are not going to shield you from internal disorders.” ~ Architect Nm.Spaces [source](https://www.quora.com)
Understanding the “Cannot Invoke Because is Null” Error in Programming
The error message “Cannot Invoke Because is Null” is a common issue developers encounter in Java programming. It usually occurs when you call a method or an operation on an object that has been set to null.
This essentially means that the computer memory space allocated for the given object is currently empty, thus, invoking operations on it leads to a `NullPointerException`. This exception is one of most common ones a Java developer would come across.
Let’s take a look at this with an example:
public class Main { public static void main(String[] args) { String str = null; System.out.println(str.length()); } }
Here, the variable `str` is declared but it isn’t assigned any value. When we try to invoke the `length()` method on `str`, it throws a `NullPointerException` as there is no actual object to perform the operation upon.
So how do we prevent the occurrence of the “Cannot Invoke Because Null” error?
- Check for null before invoking methods: Before calling a method on an object, check if it is not null. For instance, in our earlier example, we should have ensured `str != null` before proceeding to call `str.length()`.
- Usage of Optional Class: As from Java 8 onwards, you can use the Optional class to represent nullable type and to define alternative behaviors for null values.
- Avoid Null Assignment: If feasible, avoid assigning null values to variables. Instead, use default or dummy values.
public class Main { public static void main(String[] args) { String str = null; if(str != null) { System.out.println(str.length()); } } }
public class Main { public static void main(String[] args) { Optional<String> opStr = Optional.ofNullable(null); System.out.println(opStr.isPresent() ? opStr.get().length() : "Value was null"); } }
To end off with a quote by Tony Hoare, inventor of NULL references in ALGOL language: “I call it my billion-dollar mistake…At that time, I was designing the first comprehensive type system for references in an object-oriented language (ALGOL W). My goal was to ensure that all uses of references should be absolutely safe, with checking performed automatically by the compiler. But I couldn’t resist the temptation to put in a null reference, simply because it was so easy to implement.”
Debugging Tactics: Solving the “Cannot invoke because is null” Issue
The “Cannot invoke because
Firstly, it is essential to identify the source of the null object. Comb through your code to verify where the object is supposed to be instantiated. This is often the first step in fixing null objects errors in Java. You can make use of the built-in debugging tools in your IDE (Integrated Development Environment) to help do this. These software tools often allow you to evaluate individual sections of your code line by line, inspect variable values inside methods, and even change values dynamically during runtime – invaluable features for debugging purposes.
public class Main { static String str; public static void main(String[] args) { System.out.println(str.toUpperCase()); } }
In the above code snippet, we’re trying to modify a string to uppercase using
.toUpperCase()
method on an uninitialized string ‘str’. The java compilier will throw an error: “Cannot invoke because is null”.
Secondly, your refactored code should involve instantiating the object before the method call or perform a null check.
If initializing the object isn’t possible, add null checks before calling methods on objects. A null check in Java ensures a piece of code doesn’t get executed unless a certain condition (in this case, the object is not null) is met. If implemented properly, null checks effectively prevent NullPointerExceptions, thereby preventing your application from unexpected shutdown.
public class Main { static String str; public static void main(String[] args) { if(str != null){ System.out.println(str.toUpperCase()); } else { System.out.println("String is null"); } } }
Last but not least, ensure the logic flow of your code does not lead your object variable to null assignments unintentionally.
As per renowned software architect, Robert C. Martin’s famous quote “With a new language, it’s not the syntax that is hard to learn, It’ll be how to implement SOLID, DRY, KISS, etc., with the language”. Solving and avoiding ‘Null’ issues involves understanding the basic principles of coding.
This article provides further insights into effective strategies to tackle NullPointerExceptions in Java.
Exploring Different Scenarios of “Cannot Invoke Because Is Null”
The “Cannot Invoke Because Is Null” exception in Java is a class of problems usually met when dealing with objects and their methods. More often than not, it is the direct result of trying to invoke a method or access a member of a data object that has not been initialized – it’s null.
Here is a simple code snippet to illustrate
public class Main { public static void main(String args[]) { String str = null; str.length(); } }
This code will throw a NullPointerException because we are trying to use the ‘length()’ method on a string that is set to null.
To prevent such issues from occurring, there are several effective strategies you should adhere to:
java
String name;
if (name != null) {
name.isEmpty()
}
Typically these are edge cases and the root cause depends heavily on the specific coding behaviour and sometimes, and just sometimes… the intricacies of the programming environment.
It makes me recall a relevant quote by Tony Hoare who invented null references in ALGOL W language: “I call it my billion-dollar mistake — at that time I was designing the first comprehensive type system for references in an object-oriented language.”
Moreover, understanding this error is essential not only for better coding, but also to devise efficient debugging and error handling strategies. baeldung is a great resource to further explore this topic.
Proactive Measures to Prevent “Cannot Invoke Because Is Null” Errors
If the issue you are encountering is a “Cannot Invoke Because Is Null” error in Java, it generally indicates that you are trying to invoke or call a method on an object that has not been initialized. This results in a NullPointerException. In Java language, this is one of the most common runtime problems developers encounter. Here are several proactive measures to prevent these errors:
1. Implement null check
The simplest mechanism to remedy this problem is by implementing a null check before invoking any methods on the object. You can do so as follows:
if(object != null){ object.method(); }
2. Use Optional Class
A helpful approach is using the Optional class, which was introduced in Java 8 and helps reduce the chances of a NullPointerException. The intent of Optional is to provide a type-level solution for representing optional values instead of null references.
An example use is given below:
OptionalstringOptional = Optional.ofNullable(getString()); if (stringOptional.isPresent()) { // check if value present System.out.println(stringOptional.get()); }
3. Use Try/Catch Blocks
Another strategy involves handling the potential errors proactively with try/catch blocks. If the code encounters such an exception, Java will catch it, allowing your program to continue running instead of crashing outright.
try { object.method(); } catch(NullPointerException ex) { System.out.println("Object is null"); }
4. Avoid null values
One proactive measure against “Cannot Invoke Because Is Null” errors is to avoid nulls altogether. One way is to initialize variables upon declaration. Another strategy involves using empty collections/arrays instead of null ones.
Robert C. Martin, author of “Clean Code” says – “Don’t pass null. Don’t return null. Don’t accept null.” So, where you can eliminate the use of null, do so.
Additional References: Oracle Java documentation – Exceptions.
Unraveling the concept “Cannot Invoke Because Is Null” – a typical NullPointerException in Java programming, it offers an enlightening opportunity to delve into Java’s object-oriented nature. This issue arises when a specific call is made to an object that hasn’t been instantiated properly. Therefore, the object is ‘null’ and cannot logically invoke any method.
To comprehend this better, consider the code snippet:
Student student = null; student.getName();
The ‘student’ object here is declared as ‘null’. Subsequently, when attempting to invoke the ‘.getName()’ method or function on the ‘null’ student object, you encounter “Cannot Invoke Because Is Null,” or in simple language, a NullPointerException.
The best way to avoid encountering such issues includes:
- Always initializing objects before invoking methods on them.
- Applying null-checks systematically. This ensures no methods are called upon on null instances.
- Utilizing tools like Optional class in Java 8 for handling nulls effectively.
As David Parnas rightly quoted “Precision and accuracy are important in programming because neglecting tiny details has a tendency to grow over time and cause big problems.” Reflecting on this thought, it becomes apparent how even the minute detail of forgetting to initialize an object can lead to a significant problem – “Cannot Invoke Because Is Null”. By strictly adhering to the initialization rule, practicing persistent null checks, and making effective use of available tools, developers can circumvent the NullPointerException issue with ease.