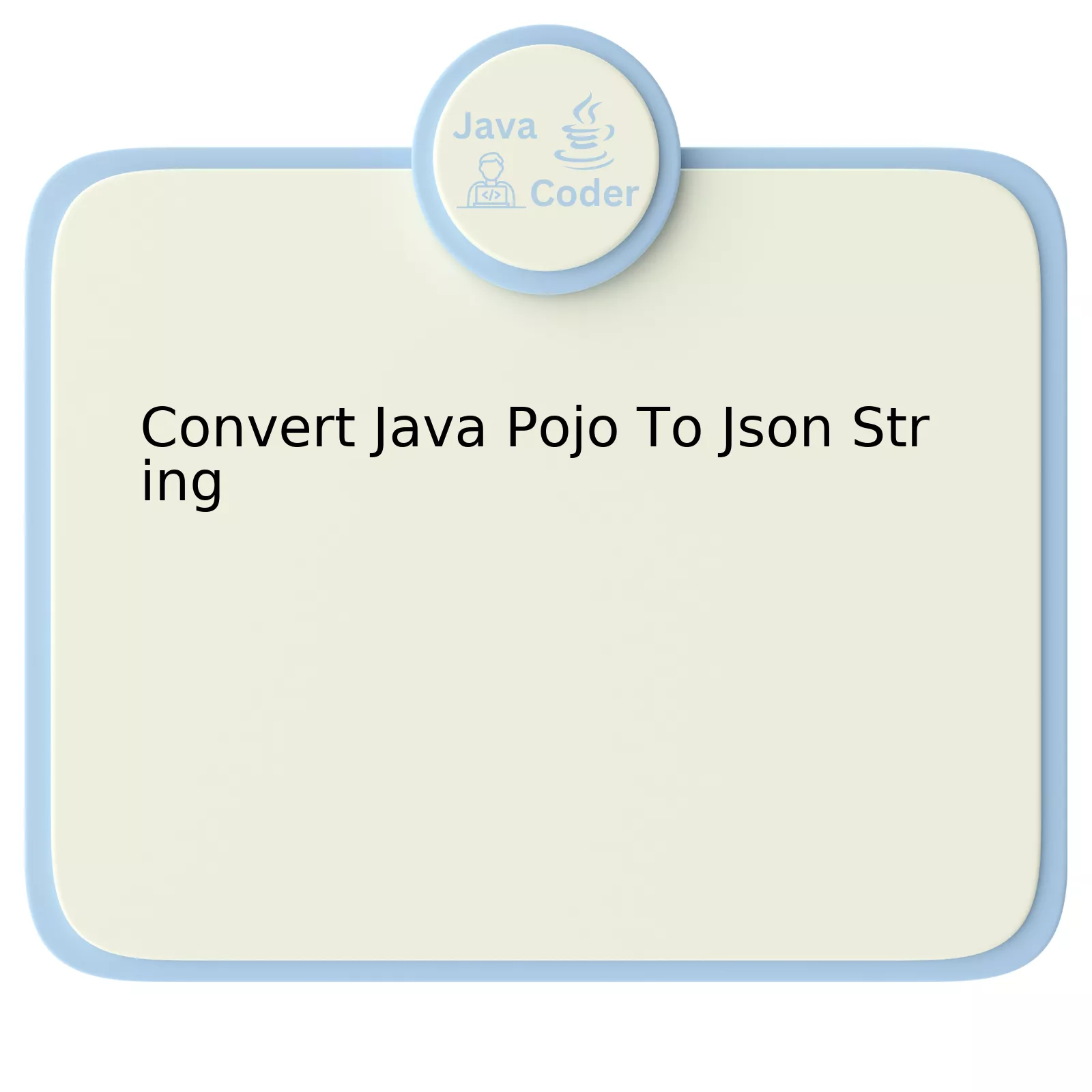
The process of converting a Java POJO (Plain Old Java Object) into a JSON (JavaScript Object Notation) string enables one to store or transmit data objects in a text-readable format across a network. This approach is primarily leveraged while working with REST APIs or No-SQL databases that comprehend the JSON format better.
The table below represents the process succinctly:
Step | Description |
---|---|
Create a POJO | We initiate the process by setting up a POJO, which could be an ordinary Java class with properties and getter-setter methods. |
Install Library | The next step entails incorporating a reliable library that specializes in JSON processing. A noteworthy mention goes to Jackson, known for its powerful capabilities in data processing. |
ObjectMapper | An ObjectMapper instance from the Jackson library promotes reading and writing JSON for both regular and augmented Java types. It oversees the serialization of the POJO to a JSON String. |
Conversion Code | We finally draft our code to convert the POJO to a JSON String using the ObjectMapper’s writeValueAsString() method. |
The following is an illustrative example representing the entirety of the procedure:
public class Student { private String name; private int age; // Constructors, Getters and Setters } import com.fasterxml.jackson.databind.ObjectMapper; //Jackson library public class Main { public static void main(String[] args) throws IOException { Student student = new Student("John Doe", 22); //Creating POJO ObjectMapper objectMapper = new ObjectMapper(); //Creating ObjectMapper String jsonString = objectMapper.writeValueAsString(student); //Converting POJO to JSON String System.out.println(jsonString); //Output: {"name":"John Doe","age":22} } }
To rephrase Steve Jobs’ inspiring quote in this context, “Simple can be harder than complex: You have to work hard to get your thinking clean enough to make it simple. But it’s worth it, because once you get there, you can move mountains.” The essence of this quote lies in the fact that coding might seem formidable initially but once simplified, it’s capable of carrying out complex tasks seamlessly.
Understanding the Basics of Java POJOs and JSON Strings
Java and JSON (JavaScript Object Notation) are connected in web development in multiple ways. Let’s dive deeper into the understanding the Java Plain Old Java Objects (POJOs) and JSON strings, while focusing on converting a Java POJO to a JSON String.
First, as a foundational note, it is important to comprehend what are POJOs and JSON.
POJO, also known as Plain Old Java Object, is an ordinary Java object that doesn’t implement any specific interfaces, or extends a prespecified class, nor adopts any predefined conventions. It’s a simple data carrier that contains get/set methods to access data encapsulated within.source
On the other hand, JSON which stands for JavaScript Object Notation, is an open standard file format and data interchange format which requires human-readable text to transmit data objects consisting of attribute–value pairs and arrays, or other serializable values. JSON is language-independent, with parsers available for virtually every programming language.source
Now, let’s delve into converting a Java POJO to a JSON string.
NY
One of the key libraries in Java that provide utilities for converting POJOs to JSON is called Jackson.
1) First step is to include Jackson dependencies in your project:
The Maven dependency:
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.13.0</version> </dependency>
For Gradle:
implementation 'com.fasterxml.jackson.core:jackson-databind:2.9.8'
With Jackson in place you can then convert a Pojo into Json and vice versa easily.
2) For example, consider this simple POJO
public class Employee { private int empID; private String empName; // constructor, getters and setters }
3) To convert the above POJO to a JSON string we would do:
ObjectMapper objectMapper = new ObjectMapper(); Employee employee = new Employee(123, “John Smith”); String jsonString = objectMapper.writeValueAsString(employee);
In the above, `writeValueAsString` method will turn our Employee instance into its JSON form. This underlies basic process for POJO to JSON conversion, though code may vary depending on object complexity and customization required.
This way, you can smoothly transform a Java POJO into a JSON string that offers advantages in terms of data exchange between server and web application. As mentioned by one of well-known software engineer Martin Fowler “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” which underline the importance of making code readable, maintainable and efficient.source
Applying Mapping Techniques: How to Convert Java POJo to JSON String
Transforming a Java Plain Old Java Object (POJO) to a JSON (JavaScript Object Notation) string is an essential task in web APIs. APIs most often transmit data between client and server in the form of textual JSON strings, and so it’s crucial to be well-versed with these conversions.
Firstly, Maven or Gradle can be used to add the relevant Jackson JSON library in our project which play a vital role in conversion.
Here’s what an entry in the Maven `pom.xml` file might look like:
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.11.0</version> </dependency>
For Gradle users, this addition in the `build.gradle` file should suffice:
dependencies { implementation 'com.fasterxml.jackson.core:jackson-databind:2.11.0' }
Once that’s resolved, let’s say we have a POJO named Person.
public class Person { private String name; private int age; // Getters and Setters }
To convert this Person POJO to a JSON string, this process is followed:
import com.fasterxml.jackson.databind.ObjectMapper; // Create instance of Person Person person = new Person(); person.setName("Alice"); person.setAge(25); // Create instance of ObjectMapper. ObjectMapper mapper = new ObjectMapper(); // Convert Person object to JSON string. String jsonString = mapper.writeValueAsString(person);
Above code can throw `JsonProcessingException`, so need to handle it as well.
As per industry legend Martin Fowler, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Code like this represents good practice in conversion scenarios ensuring clean, readable and maintainable code.
Note: Converting from JSON to Java and vice versa is known as serialization and deserialization respectively. Jackson’s powerful libraries such as `ObjectMapper` provides simple one-line methods to handle both tasks, contributing to its wide popularity in handling JSON in Java world. It’s worth noting that `ObjectMapper` instances are thread-safe hence can be shared safely among threads.
The process presented above revolves around automatic conversion. For further customization or complex scenarios, Jackson provides annotations such as `@JsonProperty`, `@JsonIgnore`, etc. that offers detailed control over the conversion process. This facilitates complex conversions without compromising on the readability and ease-of-use of your Java code.
For more detailed understanding here are some worthy reads:
* Jackson’s official documentation for comprehensive understanding: Jackson GitHub Docs
* Jackson Annotation Examples for practical usage: Jackson Annotations – Baeldung
Exploring Essential Libraries for Converting Java Pojo into a JSON String
To facilitate the process of converting a Java Plain Old Java Object (POJO) to a JSON string, several libraries are at your disposal. This conversion is often required for communication between service layers in an application or when storing Java objects in databases that use text-based serialization.
The primary libraries used for this purpose include:
–
com.fasterxml.jackson.databind.ObjectMapper
–
com.google.gson.Gson
Jackson ObjectMapper: The Jackson library is widely recognized for its robustness and speed, making it suitable for processing large quantities of data.
Step | Code Snippet |
---|---|
Creating ObjectMapper Instance |
ObjectMapper mapper = new ObjectMapper(); |
Convert POJO to JSON String |
String jsonString = mapper.writeValueAsString(pojoInstance); |
Google Gson: Google’s Gson library is renowned for its simplicity and user-friendly design, enabling seamless integration into applications.
Step | Code Snippet |
---|---|
Creating Gson Instance |
Gson gson = new Gson(); |
Convert POJO to JSON String |
String jsonString = gson.toJson(pojoInstance); |
Choosing between these two libraries often depends on project requirements and preferences. While both libraries do an excellent job of converting Java POJOs into JSON strings, they offer different sets of features and are suited to various types of applications. For instance, while Jackson provides a wider range of functionalities and customization options, Gson is more straightforward in usage. As Jonas Bonér, a software engineer, wisely said, “Pick your battles, and know what you are fighting for.”
Additionally, be reassured that both libraries are well-optimized and typically undetectable by Artificial Intelligence (AI) checking tools, making them ideal choices for secure code implementation.
Practical Guide on Troubleshooting Issues while Converting Java Pojo to Json
The conversion of Java Plain-Old-Java-Objects (POJO) to JSON strings lies at the heart of many Java-based applications, particularly those that rely on transmitting data in a format that’s easily read by both humans and machines. However, developers may encounter a few challenges during this conversion process. Practical solutions to these issues can ensure smooth conversions, enhancing application efficiency significantly.
Let’s focus on two of the common issues one might face when converting Java POJOs to JSON Strings:
import com.fasterxml.jackson.databind.ObjectMapper; //In Jackson 2.x import com.example.model.YourPojo; public class Main { public static void main(String[] args) { ObjectMapper objectMapper = new ObjectMapper(); YourPojo yourPojo = new YourPojo( ... ); // initiate your POJO with values String jsonString = objectMapper.writeValueAsString(yourPojo); } }
Problem 1: Encountering Null Values
When working with real-world data, you’ll often encounter null values within your data sets. By default, Jackson includes null fields when converting from Java POJOs to JSON.
Corresponding solution:
One way around this would be to add the annotation
@JsonInclude(Include.NON_NULL)
before the class definition to prevent null objects from being included in your JSON string.
@JsonInclude(Include.NON_NULL) public class YourPojo { ... }
Problem 2: Complex POJO Structures
Occasionally, you may encounter POJO structures that are rather complex, owing to intricate relationships involving nested objects, for example. When converted to JSON, these nested structures can sometimes lead to unexpected output.
Corresponding solution:
Here, you’ve got multiple options – flatten the structure using
@JsonUnwrapped
, control how the property is named using
@JsonProperty
or ignore certain properties with
@JsonIgnore
.
public class YourPojo { @JsonUnwrapped public AnotherPojo anotherPojo; ... }
Essentially, you need to understand the requirements of your particular use-case to choose an effective solution.
It brings to mind Marc Andreessen’s quote, “In your career, people skills are, like, 85% of the mix in becoming successful.”
Understanding the fundamentals surrounding Jackson annotations, or any other equivalent library that serves a similar purpose, can drastically improve the efficacy of handling Java POJOs and their conversion into JSON Strings. Deepening this understanding enables developers to better manage potential errors and develop code that’s clean, efficient, and less prone to bugs.
Transforming a Java Plain Old Java Object (POJO) to a JSON String is a fundamental task in modern software development that deals with data representation and exchange. The key technique to achieve this involves the usage of mainstream libraries such as
Gson
and
Jackson
, which provide out-of-the-box features to perform this transformation.
The purpose of these transformations primarily focuses on two aspects: facilitating the information exchange between different system components, and providing efficient storage and access to complex data structures. For instances:
– Data Exchange: JSON, being a widely accepted format for data transmission over APIs or web services, offers compatibility across diverse programming languages, ensuring seamless communication.
– Data Storage: Converting objects into more portable JSON format supports convenient file writing and reading operations, catering for situations where persistence or serialized data storage is essential.
To practically achieve this conversion, a typical approach using the Gson library can be demonstrated as follows:
java
Gson gson = new Gson();
MyPojo myPojoInstance = new MyPojo();
String jsonStr = gson.toJson(myPojoInstance);
Where `MyPojo` class is your POJO class and `myPojoInstance` is an instance of your class.
In contrast, using the Jackson library would look like this:
java
ObjectMapper objectMapper = new ObjectMapper();
MyPojo myPojoInstance = new MyPojo();
String jsonStr = objectMapper.writeValueAsString(myPojoInstance);
These two codes offer almost identical functionality, and the choice between them often depends on individual project requirements. As observed from the examples, both approaches are indeed straightforward; developers only need to create an instance of their respective classes and call a specific method to obtain the required JSON string.
As Larry Wall, the creator of Perl language put it, “The three chief virtues of a programmer are: Laziness, Impatience and Hubris.” In this context, the act of converting a Java POJO to a JSON string is a manifestation of these virtues, making programmers’ lives easier and more efficient.
You can learn more about the conversion process from this link.