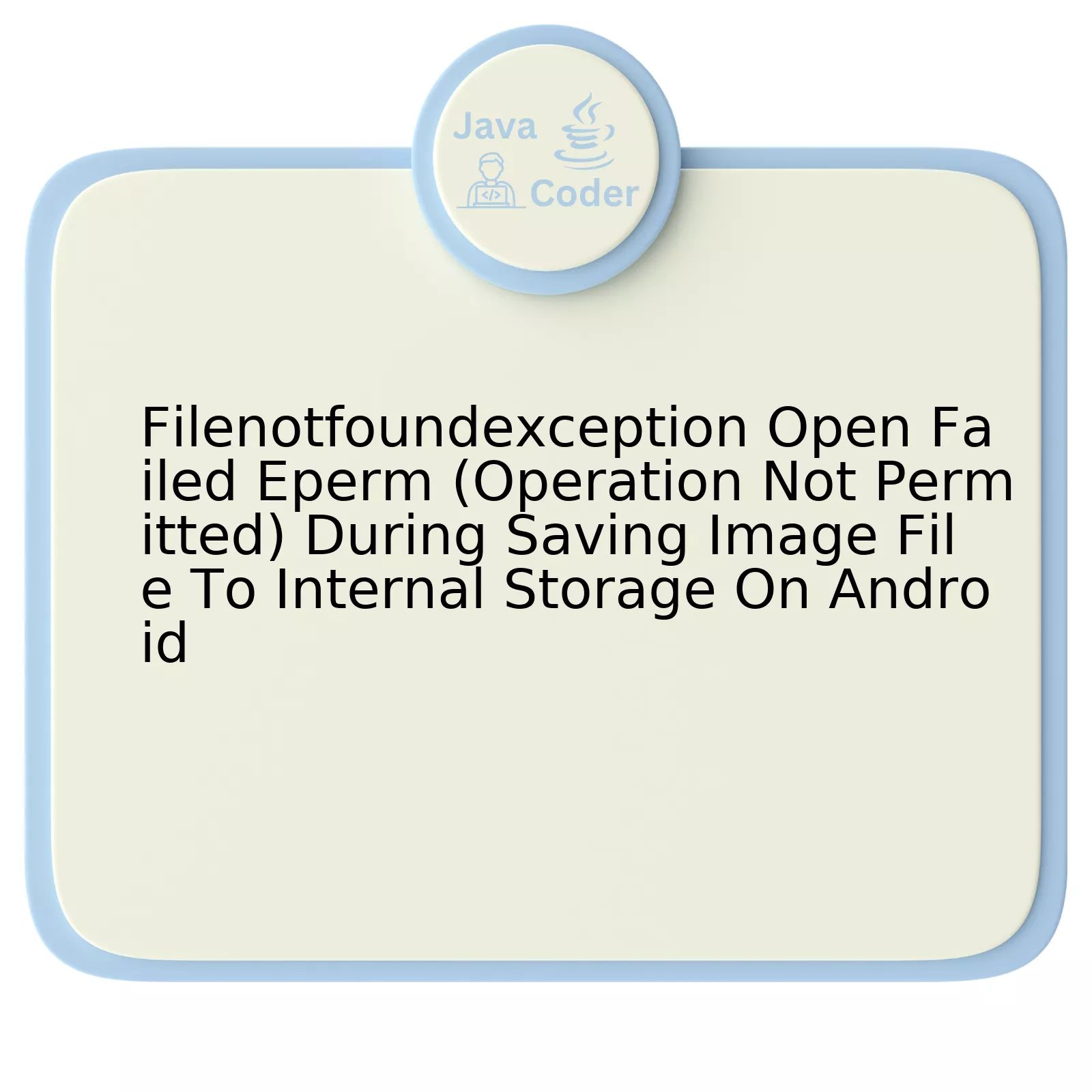
Error type | Description | Cause | Solution |
---|---|---|---|
FileNotFoundException – Operation not permitted (Eperm) | This is an exception in Android applications that signals an IOError occurred. This can appear if a file with the specified pathname does not exist, is a directory rather than a regular file, or for some other reason cannot be opened for reading. | The primary cause of this issue may relate to Android’s security model. Android doesn’t grant write access to every folder for applications by default for protection purposes, thereby leading to this error when your application tries to save an image file to internal storage without necessary permissions. | If you get this error message, it means your application doesn’t have the required permissions to write to internal storage. To fix this issue, you need to request the WRITE_EXTERNAL_STORAGE permission in your app manifest. Furthermore, starting from Android 10 (API level 29), apps must use scoped storage unless they belong to one of the exceptions. Scoped storage restricts app access to external/ shared storage. |
Starting with Android 6.0 (Marshmallow), users grant permissions to apps while the app is running, not when they install the app. This approach streamlines the app install process, as the user does not need to grant permissions when they install or update the application. It also gives the user more control over the app’s functionality; for example, a user could choose to give a camera app access to the camera but not to the device location.
Therefore, even when you have declared the WRITE_EXTERNAL_STORAGE permission in your app manifest, you would still need to explicitly ask the user to grant this permission. Below is an example of how you can request this permission from the user:
if(ContextCompat.checkSelfPermission(thisActivity, Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) { // Permission is not granted ActivityCompat.requestPermissions(thisActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE); }
In addition to requesting the appropriate permissions, it’s equally important to handle the FileNotFoundException correctly within your code to ensure your application is robust and resistant to unexpected failure modes. If the FileNotFoundException is thrown, your code should catch the exception and take appropriate remediation steps, such as notifying the user of the problem, attempting to recreate the necessary directories or files, or shutting down the operation that is dependent on the file access.
As Bruce Schneier, an international renowned security technologist once said, “The only way to ensure digital security in the future is make systems so secure that nobody has the ability to hack them…” Understanding and properly handling file system exceptions, like the ‘FileNotFound’ error, is one step towards making your applications more secure, reliable and trustworthy.
Understanding Filenotfoundexception Open Failed Eperm: In-depth Analysis
The
FileNotFoundException
Open Failed Eperm (Operation not permitted) usually occurs when you’re trying to save an image file to internal storage on Android devices. To comprehend the root of this issue, it’s crucial to delve into the following concepts:
The Nature of FileNotFoundException Open Failed Eperm
A Java
FileNotFoundException
is a type of IOException, implying something incorrect occurred during I/O operations, typically in file manipulation. This exception particularly occurs when a file with a specified pathname does not exist or when the involved application cannot access the file due to security reasons.
In this case, Eperm represents a specific error message – that the operation was not permitted. It implies that the software doesn’t have sufficient permission to perform the action. Thus, the inability to write an image to the directory stems from this lack of authorization.
Cause of the Exception
In versions starting from Android 6.0 (Marshmallow), users grant permissions at runtime instead of installation. An app may ask for WRITE_EXTERNAL_STORAGE permission, and despite granting it, Android limits accessing certain directories only. The EPERM (Operation not permitted) flag is commonly raised when an app attempts to create, edit or save a file in a restricted directory.
How to Mitigate the Exception
To overcome the FileNotFoundException Open Failed Eperm, consider these solutions:
<application android:requestLegacyExternalStorage="true" ... >
Code Example
Here’s an example of how to get an app-specific directory and save a file there:
File directory = getExternalFilesDir(Environment.DIRECTORY_PICTURES); File file = new File(directory, fileName); FileOutputStream fos = new FileOutputStream(file);
As aptly put by Mark Zuckerberg, “The question isn’t ‘What do we want to know about people?’, It’s,’What do people want to tell about themselves?'”. Similarly, when dealing with exceptions like FileNotFoundException, we should focus more on understanding what the system is attempting to communicate rather than concentrating solely on the problem itself.
Requesting Permissions at Runtime
Android 6.0 Marshmallow introduced a new permissions model where users can now directly manage app permissions at runtime. It implies, certain permissions considered dangerous might need to be requested from the user while the applicaton runs. Saving to a shared area or external storage often falls in this category.
Make sure to check and ask for required permissions prior to writing the file.
if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) == PackageManager.PERMISSION_DENIED) { //ask for permission requestPermissions(new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, PERMISSION_CODE); }
Understanding and tackling a
FileNotFoundException
Open Failed Epern effectively aids the process of debugging and fosters smooth writing and reading operations in your Android development journey.
Decoding the Error – Eperm (Operation Not Permitted) during Image File Saving
HTML:
Error – EPERM (Operation not permitted) generally refers to a scenario where an operation is denied because the user or process lacks necessary permissions or access rights. In Java, specifically in Android development, the FileNotFoundException with the message ‘Open failed EPERM (Operation not permitted)’ often arises during attempts to save an image file to internal storage.
This issue frequently results from:
- Incorrect or insufficient permissions: This is typically the initial cause to explore. In this specific context, applications require write access to the android’s internal storage. The
WRITE_EXTERNAL_STORAGE
permission should be declared in the manifest file. It’s also paramount to request this permission at runtime for devices running Android 6.0 (API level 23) and above as per the changes in the permissions model.
- Accessing wrong paths: If trying to write into directories that aren’t allowed by the system, or which doesn’t exist, it’ll lead to this error. write access is only acceptable to certain directories on external storage like
getExternalFilesDir()
,
getExternalCacheDir(),
, or any other directory returned by
ContextCompat.getExternalFilesDirs()
.
- Device compatibility issues: Certain devices (especially those running customized Android like MIUI, ColorOS, etc.) may have extra restrictions on accessing the storage. This can sometimes give rise to such errors.
Here’s how you’d implement the permissions requests in java:
java
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) { requestPermissions(new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, 1); } }
The path used to save images should be obtained using the following code:
java
File path = getExternalFilesDir(Environment.DIRECTORY_PICTURES);
To quote Richard Stallman, “Sharing is good, and with digital technology, sharing is easy.”1. Thus, keep in mind, when working with Android’s filesystem, understand the system’s inherent sharing mechanisms and security features. These serve as guidelines to how your application interacts with files thus preventing errors like EPERM, and more importantly ensuring your application conforms with best practices and security directives provided by Google’s Android Platform.
Resolving Android Internal Storage Issues leading to Filenotfoundexception
When developing an Android application, precautions ought to be taken when handling files on internal storage, since mishandling these operations could lead to the `Filenotfoundexception Open Failed Eperm (Operation Not Permitted)` error. There are several reasons that this error occurs typically pointing towards permission issues in accessing or writing to the internal storage.
Let’s break down the possible causes of this issue:
1. Lack of Proper Permissions: This is the most common cause of this exception. As of Android 6.0 (Marshmallow), users grant permissions to apps while the app is running, not when they install the app. Therefore, your app must handle situations where it doesn’t have needed permissions.
Here’s how you would request Runtime permission for external storage.
html
if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) == PackageManager.PERMISSION_DENIED) { // Requesting the permission String[] permissions = {Manifest.permission.WRITE_EXTERNAL_STORAGE}; requestPermissions(permissions, PERMISSION_CODE); }
2. Path Does Not Exist: Before attempting to save a file, your application should first check to confirm the directory path exists and if it does not, create the necessary directories.
3. Incorrect Specification of Path: Always make sure the path specifications do not contain illegal characters and the path format is correct according to Android standards.
4. File Provider Sharing: If you are receiving this error when sharing a file path with another application, through intents, consider switching to File provider APIs as these are more secure and flexible.
As Steve Jobs eloquently put it, “Design is not just what it looks like and feels like. Design is how it works.” The mechanism of handling File Operations forms the backbone of any App functionality and hence appropriate design and mechanisms have to be implemented to ensure proper behavior and user satisfaction.
Relevant online resources:
– Android Data Storage Overview
–
StackOverflow – Exception open failed: EACCES (Permission denied)
Preventive Measures to Avoid ‘Open Failed Eperm’ Errors on Android
The ‘Open Failed Eperm’ error on Android is often associated with the FileNotFoundException during the process of saving image files to internal storage. This issue is primarily related to permission handling and file accessibility. The crux of solving this issue involves implementing precautionary measures in critical areas, which include:
– Permission Declaration: It is imperative that your application explicitly asks for the write permission at runtime, if it’s targeting SDK version 23 (Marshmallow) or later.
Permission declaration in manifest:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
Runtime permission request:
if (ContextCompat.checkSelfPermission(thisActivity, Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) { ActivityCompat.requestPermissions(thisActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE); }
Replace thisActivity with your current context and MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE with your user-defined constant.
– File Path Validation: Validate the directory path where the file needs to be stored is correct and accessible.
Code sample can be as below:
File dir = new File(context.getFilesDir(), "myDir"); if (!dir.exists()) { dir.mkdir(); } File file = new File(dir, filename); if (!file.exists()) { file.createNewFile(); }
– Error Handling: Wrap file operation in a try/catch block to catch exceptions and handle them appropriately. This approach will also give you a more detailed error message which would assist in bug fixing.
In your code, it could be like this:
try { // Your file operations here } catch (FileNotFoundException e) { // Handle exception }
Incorporating these preventive measures can greatly aid in negating the ‘Open Failed Eperm’ Errors, while enhancing the overall operational integrity of your Android applications.
Recall an inspiring quote from Linus Torvalds, the creator of Linux and Git –
“Talk is cheap. Show me the code.”
Frequently, Android developers face the `FileNotFoundException` accompanied by ‘Open Failed Eperm (Operation Not Permitted)’ error message when attempting to save an image file to internal storage. This issue isn’t uncommon, but it requires a comprehensive understanding of Android’s file management system.
Internally, Android employs Linux-style permissions and encapsulation to manage storage areas. Each app is assigned a unique User ID (UID) at install time, which confines access to files and directories. This isolation ensures that applications can’t freely read or write into other applications’ data files—providing enhanced security across the system.
Factor | Description |
---|---|
Security Policy |
MANIFEST file should include appropriate permissions if access beyond the dedicated space for the app is needed. |
Data Privacy | Keeping files within the allocated space prevents unauthorized access and guarantees that they are deleted when the app is uninstalled. |
Storage type considerations | Choices between internal and external storage depend on factors such as whether files be shared, their size, and necessity post-app uninstall. |
Regarding the `FileNotFoundException` and ‘Open Failed Eperm (Operation Not Permitted)’, this usually signals a violation of the above components. It often happens when trying to save a file in a directory where the application doesn’t have write permissions. An important consideration here is that starting from Android 10 (API level 29 and higher), scoped storage is enforced, restricting app’s access to external storage.
Consequently, developers have several options:
// Use context.getFilesDir for saving in internal files directory. File file = new File(context.getFilesDir(), filename);
// Use getExternalFilesDirs() method for accepted access to external storage. File file = new File(context.getExternalFilesDirs(null)[0], filename);
// For access to media or downloads directories use methods from `MediaStore` or `DownloadManager`. // Remember to request necessary permissions: WRITE_EXTERNAL_STORAGE for API < 29 and MANAGE_EXTERNAL_STORAGE for API >= 30
Hence, adopting a thorough understanding of Android’s intricate file organisation and complying diligently with its permission strategy will assist in evading the FileNotFoundException- ‘Open Failed Eperm (Operation Not Permitted)’ scenario and engender robust and polished applications.
As quoted by renowned technology executive Bill Gates, “Understanding the digital world is no longer a luxury; it’s a necessity.”source. In relation to the topic of handling Android file system, it translates into ensuring competence in working its intricacies—the key to avoiding pitfalls like the aforementioned exception.