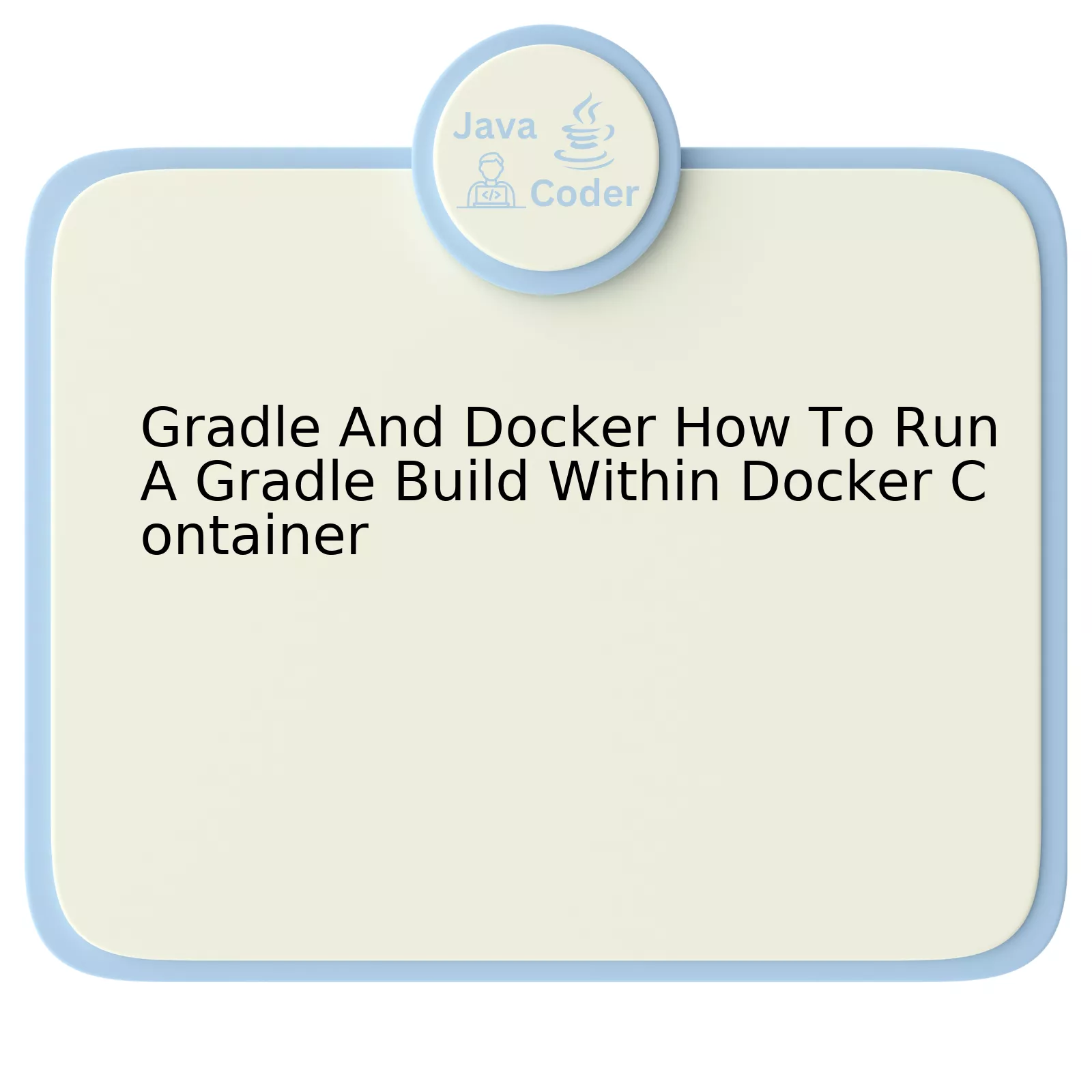
Before diving into the details, it’s crucial to first understand Gradle and Docker individually. Gradle is a powerful build automation tool known for its flexibility and performance. It allows developers to script their tasks using Groovy or Kotlin Domain-Specific Language (DSL). Meanwhile, Docker is an open-source platform that packages applications into containers — manageable, isolated environments equipped with all the libraries and dependencies needed to run the application.
The use of these two technologies can be incredibly beneficial in software development when they work together. But how exactly does one run a Gradle build within a Docker container?
Here is a structured explanation featuring key steps through a hypothetical table representation:
Step | Description |
---|---|
Create a Dockerfile | This initial step involves creating a Dockerfile in your project directory. This Dockerfile should define a Docker image that includes everything needed to run your Gradle build. |
Define Dependencies | In the Dockerfile, specify all necessary dependencies (like Java Development Kit – JDK) required for your application. Also, set your working directory inside the container where your project will reside. |
Copy Project Files | Next, copy your entire project files from your host machine into the Docker container’s working directory you have set previously. |
Run Gradle Build | Within your Dockerfile, instruct Docker to run the Gradle command to start the build process once the image has been built— for example, using CMD [“./gradlew”, “build”]. |
Build Docker Image | Finally, on your terminal command line, navigate to the path containing Dockerfile and execute the Docker Build command to create a Docker image incorporating your application. |
Run Docker Container | Subsequently, use Docker Run command to instantiate a Docker container from the newly created image. During this\nprocess, your Gradle build will be executed within the container itself. |
Further, these steps in effect make sure that your environment is independent of any external influencing factors thereby promoting consistency across multiple build cycles. Marc Andreessen, co-founder of Netscape gives the apt quote, “In technology, the new thing is rarely as good as the old thing was when you got used to it.” Leveraging Docker with Gradle helps break this notion, bringing freshness into application builds without compromising on efficiency.
Code Snippet Example:
The following snippet outlines a simplified Dockerfile structure pertaining to what we described above:
FROM gradle:latest # Set working directory WORKDIR /app # Copy project files into docker COPY . /app # Finally, run Gradle build CMD ["gradle", "build"]
And another snippet presents commands to build the Docker image and create a new container:
docker build -t my-app:1.0 . docker run -d --name my-container my-app:1.0
Interested readers looking for more detailed steps and code snippets can visit the official Gradle user guide and the Docker’s official documentation.
Understanding Docker and Gradle Interoperability
Understanding the interoperability of Docker and Gradle can significantly enhance efficiency, especially for creating standardized development environments. Docker provides a platform to build, distribute, and run applications within self-sufficient containers while Gradle is an open-source build automation tool that handles dependency management.
Though they serve different purposes, Docker and Gradle can be utilized together harmoniously, particularly in instances where one may need to run a Gradle build inside a Docker container.
Running a Gradle build within a Docker container offers several benefits:
• Isolation: Docker encapsulates your project and its dependencies into a single stand-alone entity, ensuring your development environment remains consistent across platforms.
• Reproducibility: With Docker, you can confidently anticipate the execution process as it behaves identically in diverse settings, from local development to testing to production.
• Version Control: Docker allows you to version control not only your application but also the environment in which it runs. This eliminates the perennial “it works on my machine” syndrome.
To run Gradle builds within a Docker container, you need to create a Dockerfile with instructions tailored to your needs.
Here’s a basic example of what this Dockerfile might look like:
code
FROM gradle:jdk11
COPY . /home/gradle/project
WORKDIR /home/gradle/project
RUN gradle build –no-daemon
The
FROM
directive tells Docker to use an existing docker image, in this case, the official Gradle image with JDK 11. The copy instruction moves all files from your current directory (.) to the Docker container’s home/gradle/project directory. The working directory (working) within the Docker container is set to home/gradle/project. Lastly, the RUN instruction commands Docker to execute the Gradle build, including the flag –no-daemon to prevent the Gradle daemon from running inside our Docker container.
Notably, the Docker container won’t hold any cached Gradle packages after it terminates, which is generally desirable for CI/CD pipelines, as it keeps the build artifacts clean and small.
Implementing this strategy, wherein Docker and Gradle are mutually beneficial, can streamline processes and enforce strong development practices.
As expressed by Kevin Behr in The Phoenix Project: “Improving daily work is even more important than doing daily work.” Leveraging technologies such as Docker and Gradle can dramatically aid in improving daily coding tasks.
Strategic Steps to Execute a Gradle Build in a Docker Container
The strategic process of executing a Gradle build within a Docker container can be well articulated in seven key steps. We’ll dive deep into each of these parts, providing clarity on the fundamentals and aiding in the practical understanding.
Step 1: Understanding Gradle and Docker
Both Gradle and Docker are powerful tools widely adopted today for software development.
– Gradle is a versatile build tool that’s flexible enough to build almost any type of software. Its ease of use and flexibility allows developers to automate complex builds and integrate seamlessly with continuous integration systems.
– Docker, on the other hand, is an open-source platform designed to package, deploy, and run applications by using containers. It offers a lightweight, consistent environment that’s highly portable and allows applications to run anywhere.
“Docker is what you need when your application starts asking for more consistency and less complexity.” – Javin Paul
Step 2: Setting Up Docker
Before we start building our Gradle project inside a Docker container, we need to install Docker in our system. Follow the official Docker installation guide for a comprehensive walkthrough on getting Docker up and running on your preferred platform.
Step 3: Creating Dockerfile
Next, create a Dockerfile in your project root directory to define your Docker image. This file instructs Docker on how it should build our images. Here is an example:
FROM gradle:jdk-alpine WORKDIR /home/gradle/project COPY --chown=gradle:gradle . /home/gradle/project CMD ["gradle", "build"]
This Dockerfile creates a new Docker image based on the official Gradle image and sets the working directory to our project location inside the container.
Step 4: Building Docker Image
With the Dockerfile ready, execute the following command in the terminal to build the Docker image:
docker build -t my-gradle-project .
This command generates a Docker image labeled ‘my-gradle-project’.
Step 5: Running the Gradle Build Inside the Docker Container
Having our Docker image in place, we can now run our Gradle build inside the Docker container. Use the following command:
docker run --name my-build my-gradle-project
This command creates a new Docker container with the name ‘my-build’ and runs the Gradle build inside it.
Step 6: Analyzing Build Reports
After the build is complete, you can access the reports generated during the build from the container. You can copy these reports from the Docker container to your host machine using the docker cp command.
Step 7: Managing Docker Resources
Finally, don’t forget to manage your Docker resources correctly. Make sure to remove unused or unnecessary Docker images, containers, and volumes regularly. This will free up system resources and keeps your Docker environment clean and efficient.
By following these strategic steps, you can ensure a seamless execution of a Gradle build within a Docker container, enabling a streamlined, reproducible, and isolated build environment that enhances your DevOps pipeline.
Effective Tips for Improving Gradle Performance within Docker
Enhancing the performance of Gradle within Docker entails a combination of fine-tuning techniques both from the Gradle and Docker perspectives. These performance boosts are crucial for maintaining shorter build times, efficient usage of resources, and flexible development environments, especially when running a Gradle build within a Docker container.
Tip 1: Leverage Gradle’s Build Cache
Gradle’s build cache reuses task outputs from any previous build, effectively chopping off time consumed by re-executing unchanged tasks. It is beneficial when building multiple Docker images which sport identical layers.
settings {
buildCache {
local {
enabled = true
directory = file(“/cache”)
}
}
}
To enable the Gradle’s build cache, you need to configure your `settings.gradle` as illustrated above. The `/cache` can be a shared Docker volume that stores the cache across builds.
Tip 2: Applying Docker BuildKit
Docker BuildKit is a collection of builder toolkit for converting source code into Docker image. It optimizes Dockerfile interpretations, parallelizes build stages, skips unused stages, and allows for quick cache retrievals. Enabled through an environment variable, it provides dramatic improvements over standard Docker builds.
export DOCKER_BUILDKIT=1
Tip 3: Utilize a Minimal Base Image
The larger the base image, the more disk space it occupies both on your Docker host and in your Docker image registry. Using smaller base images not only speeds up your Docker build but also makes downloads faster every time there’s a fresh deployment. From a Java perspective, consider openjdk’s JRE (Java Runtime Environment) image or AdoptOpenJDK.
Tip 4: Incremental Compilation and Annotation Processing
Gradle’s incremental compiler funnels build time into executing tasks that have modified since the last build. This reduces build time considerably, and it should automatically kick into action unless directly impeded. Also vital is setting incremental annotation processors.
tasks.withType(JavaCompile) {
options.incremental = true
options.annotationProcessorGeneratedSourcesDirectory = file(“$buildDir/generated/sources/annoProcessor/java/main”)
}
This configuration tells the
JavaCompile
tasks to use compilation with incremental annotation processing.
As famously quoted by Robert C. Martin,
“There is no good software without a good architecture!”
We can extract from this concept; managing and optimizing processes will lead to output efficiently. Be it architectural designs or Gradle builds within Docker containers, the diligence spent on fine-tuning leads to noteworthy results.
Relevant Reading:
– Docker Build enhancements using Buildkit
– Gradle User Guide
– AdoptOpenJDK
Troubleshooting Common Issues of Running Gradle Inside Docker Containers
Moving forward with the topic “Gradle And Docker: How To Run A Gradle Build Within Docker Container”, there are indeed potential problems that could be encountered while running Gradle inside Docker containers which users may face and should be aware of. Here’s how to troubleshoot common issues:
1. Gradle Build Failing Within Docker
This can occur when Docker doesn’t have enough resources allocated, especially memory. Troubleshooting this involves increasing allocated resources in your Docker settings.
2. Network Issues
Network problems can affect downloading dependencies in a Gradle build process. This issue could potentially be solved by setting a longer timeout or retrying failed connections.
repositories { mavenCentral { url "https://repo.maven.apache.org/maven2" configuration { timeoutInMillis = 120000 } } }
3. Permission Problems
Permission problems might happen if your Gradle scripts need to modify system files or open privileged ports. In these cases, ensure that you’re running your Docker container with the appropriate permissions.
4. File-system Related Issues
Docker containers have their own file systems, separate from the host. Any file-system related operations should consider this difference.
Here is an example of how one might delegate Gradle daemon files to avoid conflicts between builds:
org.gradle.workers.max=1 org.gradle.jvmargs=-Xmx256m -XX:MaxMetaspaceSize=512m -XX:+HeapDumpOnOutOfMemoryError -Dfile.encoding=UTF-8
In Natan Lid’s Medium article about running Gradle within Docker, he finds “Having smaller heap sizes reduces the pressure on Docker Desktop.”
5. Version Incompatibility
Given our constant move towards updating and integrating different versions of development tools, it’s probable for a version mismatch issue to arise. One should always ensure that they’re using compatible versions of both Gradle and Docker.
As Reginald Lynch, an expert in technology, and programming once said, “When it comes to writing code, the devil is in the details. Even the smallest of considerations like version compatibility can save you hours of debugging down the line.”
Adopting the right practices for building with Gradle in Docker will help circumnavigate these troubleshooting issues. Always remember, to note the resources consumed during build, give a keen eye to the network aspects, run with the right permissions set, handle the file-system diligently, and keep a watchful eye on version compatibility.
Without a doubt, the amalgamation of Gradle and Docker technologies offers developers an efficient approach to build and deploy software within isolated environments. Strategically running a Gradle build in a Docker container ensures operational consistency by eliminating unwelcome surprises arising due to discrepancies between development and production environments.
Utilizing Docker with Gradle
Working hand-in-hand, Docker provides a platform for packaging applications for smooth deployment while Gradle offers an advanced build toolkit to automate and manage complex projects. By encapsulating a Gradle build process within Docker, you are set to leverage:
Dockerfile
: Used to build a Docker image from the Gradle project, it can contain instructions that install necessary dependencies and copy your project into the Docker container.
build.gradle
: This designates the tasks required for packaging and deploying the application, including dependencies, test configurations or plugins. Using Docker, Gradle can target application delivery across various system environments without code modification.
To succinctly run a Gradle build inside a Docker container, first create a Dockerfile inside your Gradle project with necessary instructions. Then, through your terminal or command line interface (CLI), navigate to your project directory and utilize Docker commands to build and run the container.
Here is a simple code example demonstrating Docker’s commands:
java
//Building an image from Dockerfile
docker build -t gradle_project .
//Running the created Docker image
docker run gradle_project
This technological collaboration immensely benefits the software lifecycle since Docker containers provide system-level isolation and resource bundling, enabling Gradle’s consistency in project builds irrespective of the runtime environment. As Tim Berners-Lee, the inventor of the World Wide Web said, “We need diversity of thought in the world to face new challenges” – equally applicable to technology, bringing Docker and Gradle together reinforces flexibility and situational adaptability in developers’ workflow.
To delve deeper into specific use-cases and further enhance your understanding, consider Gradle’s Official Documentation and Docker’s Comprehensive Guide. Consequently, exploiting this blend of technologies not only escalates productivity but also unravels new possibilities to streamline software development and deployment pipelines.