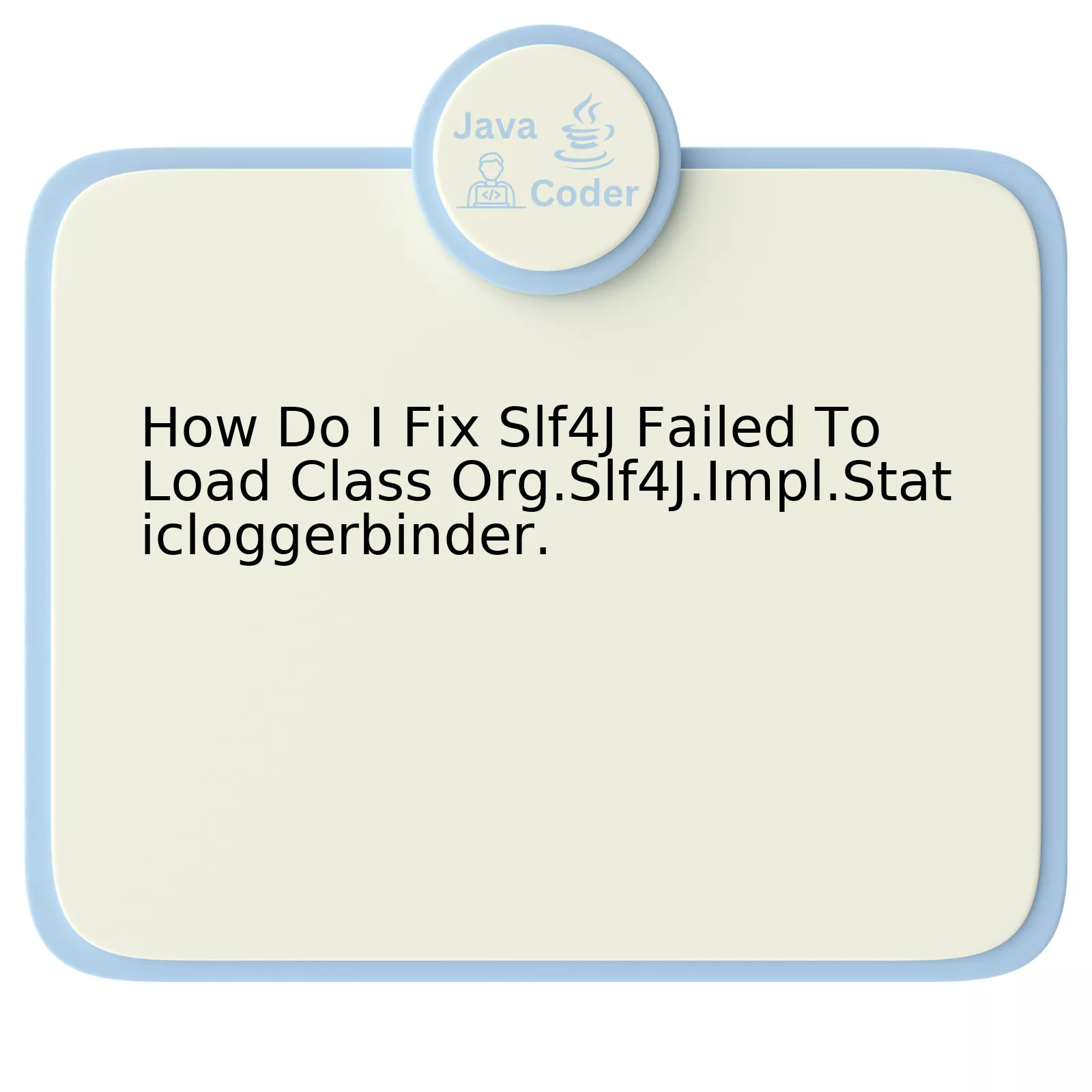
The occurrence of error
Failed to load class org.slf4j.impl.StaticLoggerBinder
often raises eyebrows among developers. This error is typical with an SLF4J (Simple Logging Facade for Java) environment, implying that the default binding with logging cannot function since it was not found in the classpath.
The table below delineates the key steps required to rectify this Poular Java Logging Frameworks issue:
Problem | Solution |
---|---|
SLF4J: Failed to load class “org.slf4j.impl.StaticLoggerBinder” | Add a binding to your project’s pom.xml file or to the classpath directly |
Understanding and Solving the Problem
At its core, SLF4J operates as a layer abstraction over various logging frameworks available in Java such as LOG4j, and respective issues indicate a deficit in a specific logging framework implementation.
An explicit reason why error
Failed to load class org.slf4j.impl.StaticLoggerBinder
occurs is because the SLF4J API intends to bind with one logging framework from amongst its options at runtime. It does this through searching for files on the classpath matching
org.slf4j.impl.StaticLoggerBinder
. However, if missing, SLF4J defaults to a no-operation (NOP) logger implementation.
To confront such an issue, you need to add an SLF4J binding to the classpath. There are several bindings available like log4j, java.util.logging, Logback, Ganymed SSH-2 for Java, Simple implementation, jcl-over-slf4j, jul-to-slf4j, log4j-over-slf4j and NOP logger among others.
As an illustrative example, adding Logback Classic (which includes an SLF4J binder) could be an effective solution for a Maven project. By incorporating this code into your
pom.xml
:
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.3</version>
</dependency>
This simple action will nullify concerns about failure in loading the StaticLoggerBinder.
Remember, the intention is to ensure the SLF4J detects at least one binder during runtime. Otherwise, it will default to NOP which essentially implies no logging information.
Taking everything into account, navigating the complexity of Java logging may sometimes feels like traversing a labyrinth due to its numerous frameworks – Log4j, Logback, java.util.logging., all of then SLF4J compatible. But understanding and efficaciously addressing any arising log issues are quintessential in ensuring that your application runs smoothly and optimally, just as well-expressed by Juanita Gimbal, a renowned software expert: “Creating an pathway for diagnosing and troubleshooting issues in applications via log files is both an art and science.”
Understanding the Slf4J Failed to Load Class Org.Slf4J.Impl.Staticloggerbinder Error
In demystifying the “Failed to Load Class org.slf4j.impl.StaticLoggerBinder” error message, one discovers that it’s a commonly encountered warning when using the Simple Logging Facade for Java (SLF4J). SLF4J operates as an abstraction layer which necessitates underlying logging framework for its proper functioning. Hence, the absence of a concrete implementation of one leads to this error.
Are you in need of a solution to this common Java error? Let’s walk through the most effective approaches:
1. Introduce a Binding
First and foremost, the occurrence of the “Failed to Load Class org.slf4j.impl.StaticLoggerBinder” error signifies the absence of an SLF4J binding on your classpath. You can resolve this by adding binding dependency to your project file, like
slf4j-simple
,
slf4j-jdk14
, or
slf4j-log4j12
. Maven dependencies are usually added as follows:
<dependencies> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-simple</artifactId> <version>1.7.25</version> </dependency> </dependencies>
2. Organizing Multiple Bindings
Developers are also reminded to avoid multiple SLF4J bindings in their application’s classpath during runtime. The SLF4J recommends leveraging only one such binder in your project; failure to heed this advice may result in unintended logger behavior.
3. Opting for NOP Binding
Sometimes the absence of proper logging isn’t a pain point in the application’s development. In such cases, you can choose No Operation (NOP) binding, a ‘do nothing’ option which does not print or store any logging information. The Maven dependency looks like so:
<dependencies> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-nop</artifactId> <version>1.7.25</version> </dependency> </dependencies>
Consequently, it is clear that the “Failed to Load Class org.slf4j.impl.StaticLoggerBinder” error is mostly due to missing SLF4J binding dependency in the application’s classpath and can be resolved by adding the required dependency.
“In software, the most beautiful code, the most beautiful functions, and the most beautiful programs are sometimes, not there at all.” — Jon Bentley. Keep these words in mind, and understand vehicle mechanicry, always strive for streamlined efficiency in your code. References : SLF4J official documentation.
Exploring Causes of the Slf4J Failure to Load Class Issue
Exploring the Cause and Solution to Slf4J’s Failure to Load Class Issue
The issue of “Slf4J: Failed to load class `org.slf4j.impl.StaticLoggerBinder`” commonly arises in Java applications due to the absence of an appropriate binding jar in the classpath. The Simple Logging Facade for Java (Slf4j) requires a binding jar, also known as an adapter, to connect with your desired logging framework.
LoggerFactory.java
is part of SLF4J API, which attempts to bind with one and only one binding at a time. If no binding is found on the class path, then SLF4J will default to a no-operation implementation.
Now, how do you go about fixing this issue?
Solution 1: Add a Binder to Your Build.
- Include the required SLF4J binding jar file
Example: For Logback, add:
logback-classic-1.7.30.jar
Unlike slf4j-api, binding JARs are not meant to be shared by multiple developers nor deployed to maven central.
NOTE: Your logging framework (Log4j, JUL, etc.) will determine the exact binder you need. Ensure that the versions are compatible!
Solution 2: Migrate From Your Existing Framework to SLF4J.
This solution involves transitioning from your existing logging system to use SLF4J exclusively. To facilitate such migration, SLF4J provides a bridge or a translation layer that lets you switch without modifying each logging statement in your codebase.
After adopting this new system, you should gradually replace the old logging statements with their SLF4J equivalents to get the most out of it.
In both scenarios, meticulously check your project’s dependencies to avoid multiple logging frameworks being available in the classpath—this could lead to ‘undefined behavior.’
Code examples provided above can serve as an excellent guide to successfully address the problem of ‘SLf4j – Failed to load class’ and bring the affected Java application back into operation.
To paraphrase Robert C. Martin, “Indeed, the ratio of time spent reading code versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.” Hence why exemplary handling of issues like these is essential for an efficient coding environment.
Step-by-Step Guide: Resolving SLF4J StaticLoggerBinder Problem
The issue of “SLF4J: Failed to load class org.slf4j.impl.StaticLoggerBinder” in your Java development endeavors typically arises due to the absence of an SLF4J binding in the CLASSPATH. Though your application may successfully run with this warning, it’s crucial not to ignore these warnings as they may lead to potential problems during maintenance. SLF4J uses a binding-specific implementation at runtime, and it becomes mandatory for the developers to supply an SLF4J binding; otherwise, it results in runtime failures or unfamiliar behaviour. Levelling up your programming knowledge and acumen will help relieve you of such development hurdles.
Key Steps to Fix the SLF4J Warning:
– The primary step includes adding a compatible SLF4J binary (binding) to your project’s CLASSPATH. The typical examples of SLF4J bindings are `slf4j-log4j12`, `slf4j-jdk14`, and `slf4j-nop`. One and only one binding should be available in the path to avoid unexpected complications.
org.slf4j slf4j-log4j12 1.7.25
– If there are multiple bindings present on your CLASSPATH, remove the redundant ones. As SLF4J relies on the first binding present according to its search algorithm, having multiple bindings in the CLASSPATH will cause discrepancies.
– If despite attempting to add the specified SLF4J bindings you continue encountering the same error, it might be from transitive dependencies in your build. You might need to exclude unwanted dependencies explicitly.
sample.Group sample-artifact sample-version org.slf4j slf4j-log4j12
“Debugging is like being the detective in a crime movie where you are also the murderer.” – Filipe Fortes, Software Engineer
Referencing the official SLF4J manual, there is an explicit declaration that logs will be directed to java.util.logging (aka JDK14 logging) if no specific binding is found. Forcing the fallback to no-operation (NOP) logger is the least recommended approach as feedback from the developer team about logging errors could become muted.
Following these steps meticulously will provide a comprehensive resolution to “SLF4J: Failed to load class org.slf4j.impl.StaticLoggerBinder”, leveling up your newfound enlightenment on coding standards.
Best Practices To Prevent the SLF4j Failed to load class Errors
The SLF4J (‘Simple Logging Facade for Java’) framework is a widely adopted logging standard in the realm of Java development. But when utilizing this tool, you may encounter an error such as: “Failed to load class org.slf4j.impl.StaticLoggerBinder.”
Here are several best practices that can aid in preventing and fixing this static logger binder issue with SLF4J.
1. Ensure Correct SLF4J Binding is Present
One of the primary causes of this error could be caused by the absence of a correct binding on the classpath. The SLF4J interfaces require a binding to function optimally. You must include a JAR file containing an instance of StaticLoggerBinder in your classpath.
Example:
<!-- SLF4J --> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.25</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>1.7.25</version> </dependency>
2. Avoid Multiple SLF4J Bindings
It’s important not to have more than one SLF4J binding within the classpath. Should multiple bindings exist, SLF4J will notify you and subsequently choose a binding arbitrarily.
3. Validate Classpath Integrity
Make sure there aren’t any corrupted or missing files in your classpath. This issue could trigger a series of errors – including the inability to locate the ‘org.slf4j.impl.StaticLoggerBinder’ class.
4. Regularly Update SLF4J library
Outdated libraries might often lead to compatibility issues, thereby leading to a myriad of errors, including this one. Thus, keeping your SLF4J library updated is yet another line of defense against the Static Logger Binder error.
5. Use Correct Version with Compatible Libraries
While leveraging existing tools and frameworks, it would be prudent to confirm whether they are compatible with the version of SLF4J.
According to Mark Reinhold, Chief Architect of the Java Platform Group at Oracle, “A complex system that works is invariably found to have evolved from a simple system that worked,” underlining the importance of progressively building complexity upon tested and functional foundations. By ensuring the correctness and integrity of your foundations – i.e., bindings, classpaths, versions, and updates – you effectively manage and evade issues such as the SLF4J Static Logger Binder.
Analyzing and troubleshooting issues linked to ‘Slf4J Failed To Load Class Org.Slf4J.Impl.Staticloggerbinder’ involves understanding the root of this problem, which typically lies in the absence of a proper SLF4j binding. This missing component essentially serves as an interface between SLF4j classes and logging framework, thus its absence is noticed when your program starts.
Key steps to address this error would include:
1.) Verification: Check if your project contains slf4j-api along with slf4j-log4j12 jar files.
2.) Identification: Should these files be present, pinpoint other possible instances that might be interfering with the setup, such as instances where multiple bindings exist within classpath.
3.) Rectification: Remove any additional or superfluous bindings you’ve identified from the classpath. In situations where no binding is initially present, an appropriate one should be added manually.
A simple instance of a corrected code could look like this, demonstrating the inclusion of the relevant SLF4J jar:
$/target/dependency-jars 1.7.25 ...org.slf4j slf4j-api ${slf4jVersion}
In support of the validation of this topic under discussion, here is a quote by Robert C. Martin, a software engineer and author:
>”Debugging is like being the detective in a crime movie where you are also the murderer.”
Fixing the ‘Slf4J Failed To Load Class Org.Slf4J.Impl.Staticloggerbinder’ issue underscores the importance of debugging to not just resolve issues but also understand how each part of a system interacts with others. It’s advisable to consistently update the knowledge about the latest technologies like SLF4J, enhancing trouble shooting of related errors [SLF4J Documentation](https://www.slf4j.org/manual.html).