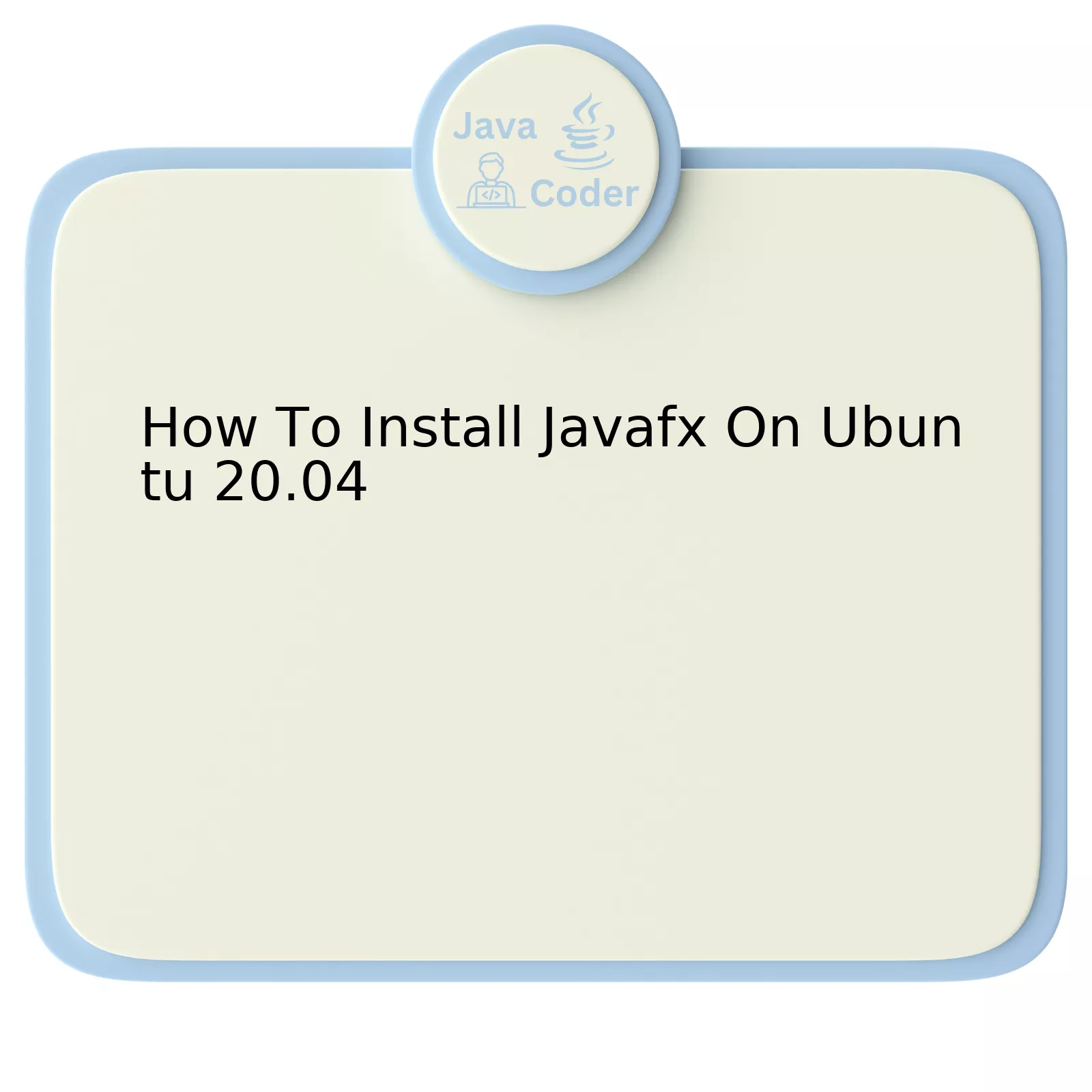
Steps | Explanation |
---|---|
Open Terminal | In Ubuntu, you will need to access the terminal since this is where all our necessary command lines will be written and executed. |
Update System | By typing
sudo apt update && sudo apt upgrade , your system updates itself, preparing for the new software installation. |
Verify If Java is Installed | Type
java -version in the terminal. If java is already installed, it will show the current version; if not, you will have to install it first. |
Install OpenJDK 8 | To do this, execute:
sudo apt install openjdk-8-jdk . JavaFX requires at least Java 8. The most recommended for Ubuntu is OpenJDK 8. |
Download JavaFX SDK | This can be downloaded from the official Gluon site. Keep track of the directory you place the downloaded file. |
Unpack the Downloaded File | |
Set Up JAVA_HOME | Ensure that Ubuntu recognizes the location of Java and JavaFX by setting
JAVA_HOME and PATH_TO_FX respectively. This process involves locating the path of the jdk and jfx files and exporting these paths using export command in the .bashrc file. |
Check the Installation | Create a simple program to verify the installation. If there are no errors returned when compiling and running this program, the JavaFX installation is successful! |
The above table provides a summary on how to install JavaFX on Ubuntu 20.04.
Firstly, ensure that you have access to the terminal in Ubuntu since this is where you will write and execute all the necessary commands. Always start with updating your system to prepare it for the new software installation using
sudo apt update && sudo apt upgrade
command line.
It’s important to check if Java is already installed in your system which can be done by simply typing
java -version
in the terminal. In case Java isn’t installed, it needs to be installed first.
JavaFX needs at least Java 8 and for Ubuntu, the most recommended one is OpenJDK 8. It can be installed by executing
sudo apt install openjdk-8-jdk
.
Next, download the JavaFX SDK from the Gluon’s site and take note of the directory you place the downloaded file. Unpack the downloaded file into a memorable directory as you will use it later.
After that, set up the `JAVA_HOME` variable and `PATH_TO_FX` to ensure Ubuntu recognizes the location of the installed Java and JavaFX respectively. Identify the path of the jdk and jfx files and export them using the `export` command in the .bashrc file.
Lastly, always remember to validate your installation. Create and run a simple program. Should the program compile and run without returning any error, the JavaFX installation is deemed successful!
Like Brian Kernighan said, “Don’t write big programs as a spec. I like small sharp tools.” So break down the problem and solve it effectively and efficiently. With the correct installation of JavaFX, you are ready to create those amazing GUI applications. Happy coding. [source](https://www.brainyquote.com/quotes/brian_kernighan_537744)
Understanding the Prerequisites for Installing JavaFX on Ubuntu 20.04
Understanding the prerequisites for installing JavaFX on Ubuntu 20.04 is an integral part of your journey towards leveraging this powerful platform for creating and delivering immersive internet applications. So, let’s delve into the nitty-gritty details of the installation process.
Firstly, it’s imperative to understand that the installation process for JavaFX hinges heavily on existing Java Development Kit (JDK) on your system. For running JavaFX applications, JDK 8 or any later version is required; hence checking its availability is paramount. You can verify the presence of Java and its version with the following command in your terminal:
java -version
The output should reflect a version number greater than or equal to 1.8. If JDK is missing, install it using these commands:
sudo apt update sudo apt install openjdk-11-jdk
Starting from JDK 11, JavaFX is not included in JDK, so it must be included manually, which brings us to the main objective: installing JavaFX. Those essentials being established, head onto this direct path to install JavaFX:
1. Download the JavaFX SDK from here.
2. Extract the downloaded file to the desired location.
3. Set the path to our JavaFX installation in the environment variable by adding the below line to either /etc/profile (for all users) or ~/.bashrc (for the current user).
export PATH_TO_FX='path/to/javafx-sdk-15.0.1/lib'
4. Now you have setup JavaFX and can compile JavaFX program with
javac --module-path $PATH_TO_FX --add-modules javafx.controls,javafx.fxml HelloFX.java
5. And run it with
java --module-path $PATH_TO_FX --add-modules javafx.controls,javafx.fxml HelloFX
Remember the wise words of Ellen Ullman, a software engineer and writer, who once notably said “We build our computer (systems) the way we build our cities: over time, without a plan, on top of ruins.” Therefore, as programmers, it’s our duty to ensure each step taken is methodical, deliberate and accurate.
These steps should effectively guide you through the entire process for Ubuntu 20.04, equipping you with robust tools necessary for developing compelling JavaFX applications. In case the steps fail, double-check the versions and cross-referencing them against official documentation is advisable. Lastly, always remember to troubleshoot one issue at a time if anything comes up, instead of attempting to resolve everything simultaneously.
Detailed Steps in Setting up OpenJDK 11 on Ubuntu 20.04
OpenJDK 11, also known as Open Java Development Kit, is a free, open-source version of the Java programming language. Ubuntu 20.04 LTS (Focal Fossa) provides delegated access to this software via its official repositories. Follow the steps outlined below to install OpenJDK 11 on Ubuntu 20.04.
STEP 1: Update your system
Begin by updating your package list using the command:
sudo apt update
Executing this command ensures that you are installing the latest version available in your Ubuntu repository.
STEP 2: Install OpenJDK 11
To install OpenJDK 11, use the following command:
sudo apt install openjdk-11-jdk
Once successfully installed, you can verify by running the command:
java -version
The output should confirm that OpenJDK 11 has been installed.
STEP 3: Set default Java Version
If you have several versions in your system, set OpenJDK 11 as the default by executing the command:
sudo update-alternatives --config java
After this initial setup, shift focus from setting up OpenJDK 11 to installing JavaFX on Ubuntu 20.04.
JavaFX is a software platform used to create and deliver desktop applications, as well as embedded systems. It improves upon previous Java GUI toolkits such as AWT and Swing, providing smoother graphics and more functionalities.
STEP 4: Install JavaFX
JavaFX isn’t included in JDK since Java 8 and needs to be downloaded separately. Install JavaFX SDK from Gluon’s website. Save the downloaded .zip file in your preferred directory and extract it:
unzip openjfx-11.0.2_linux-x64_bin-sdk.zip
STEP 5: Setup Environment Variables
Store the path to the lib folder inside the extracted JavaFX sdk directory in an environment variable:
export PATH_TO_FX=path/to/javafx-sdk-11.0.2/lib
Later, when running a JavaFX application, you need to pass the module-path of the JavaFX sdk and the javafx.controls,javafx.fxml modules to the java command as shown below:
java --module-path $PATH_TO_FX --add-modules javafx.controls,javafx.fxml HelloFX
As Linus Torvalds once said, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” The above process may seem tedious, but its mastery is part of the journey of enjoying programming with Java platforms such as OpenJDK 11 and JavaFX.
Downloading and Integrating JavaFX with OpenJDK
To install JavaFX on Ubuntu 20.04 and successfully integrate it with the OpenJDK requires an accurate understanding of the steps involved, ensuring that the complete process is followed through without any missteps.
Acquiring the JavaFX SDK
The foremost step in this process involves downloading the appropriate version of the JavaFX SDK [here](https://gluonhq.com/products/javafx/). The page lists a host of different versions compatible with various versions of Java. To maintain compatibility with OpenJDK, it’s advisable to acquire the one corresponding to your specific Jave version.
The JavaFX Software Development Kit downloaded will be in ZIP format. You’ll have to extract its contents and move it into the prefered directory. This could be done using the
unzip
command:
unzip openjfx-12.0.2_linux-x64_bin-sdk.zip sudo mv javafx-sdk-12.0.2 /usr/lib/
Setting up JAVA_HOME and PATH for OpenJDK
Secondly, you must set both JAVA_HOME and PATH variables to link with the OpenJDK installed on your Ubuntu system. You will need to locate where your OpenJDK has been installed. This can be accomplished via the terminal using the
update-java-alternatives
command. Use the
export
command to update the JAVA_HOME variable as such:
export JAVA_HOME=/usr/lib/jvm/java-11-openjdk-amd64
Same way, to add OpenJDK in PATH:
export PATH=$PATH:$JAVA_HOME/bin
Integrate JavaFX to OpenJDK
The final step is the integration of JavaFX and OpenJDK. This comes down to updating the PATH_TO_FX environment variable as follows:
export PATH_TO_FX=/usr/lib/javafx-sdk-12.0.2/lib
Now that everything is configured correctly, let’s test our setup by writing a simple JavaFX program:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.stage.Stage; public class HelloWorld extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage stage) throws Exception { Label helloWorld = new Label("Hello, world!"); Scene scene = new Scene(helloWorld, 200, 100); stage.setScene(scene); stage.show(); } }
Compile the program using this command in your terminal:
javac --module-path $PATH_TO_FX --add-modules javafx.controls HelloWorld.java
And proceed to run the program:
java --module-path $PATH_TO_FX --add-modules javafx.controls HelloWorld
If all the outlined steps are correctly followed, then your installation and integration process is rightly executed. Consequently, exploring and experimenting with the abundant unique features that JavaFX brings along suffices.
The intricacies in setting up JavaFX in some Linux distributions appear to discourage users. However, as the famous quote from Dennis Ritchie, creator of the C language, goes, “The only way to learn a new programming language is by writing programs in it.” With persistence and practice, it becomes simpler.
Troubleshooting Common Issues during JavaFX Installation on Ubuntu 20.04
The installation of JavaFX on Ubuntu 20.04 comes with its unique challenges that can leave users frustrated if not properly addressed. This section sheds light on possible issues one might encounter and provides well-thought-out solutions to them.
1. Unresolved or Missing Modules Error
One common error often encountered while installing JavaFX on Ubuntu is the
unresolved modules
error or a situation where some required modules seem missing. The following solution ameliorates this issue:
--add-modules javafx.controls,javafx.fxml,javafx.media,javafx.web
Adding
--add-modules
with all necessary modules as arguments resolves the above-mentioned error.
2. Inappropriate Environmental Configuration
Your environmental configuration plays a crucial role in successful JavaFX installation. It’s essential to configure your PATH environment variable correctly and point it to your Java installation directory. A wrongly configured PATH may inhibit JavaFX from being installed successfully. You can modify your PATH using the export command:
export PATH=$PATH:/usr/lib/jvm/java-11-oracle/bin/
3. Wrong Java Version
Incompatibility between the installed Java version and JavaFX often triggers errors. As JavaFX 11 (LTS) onwards, JVM is not bundled with the SDK. Hence, it’s important to check if you’ve installed JDK-11 or newer versions.
To check your java version:
java --version
For Java version compatibility, it’s advisable as reflected in the words of Brian Goetz, Oracle’s Java language architect, “When in doubt, check your versions! They could save you hours of debugging time.”
4. javafx does not exist Error
JavaFX’s applications fail to compile when the package does not exist. This error usually crops up when the classpath lacks the JavaFX SDK path. To fix this, add the path to the JavaFX SDK library to the system’s classpath.
-cp /path/to/javafx-sdk-/lib/*:
While hoping for easy installation steps, encountering these hitches is normal, and understanding how to troubleshoot them remains fundamental. Utilize the insights provided to leap over any hurdles while trying to install JavaFX on Ubuntu 20.04.
Here is an excellent reference to guide you more on JavaFX installation on Ubuntu.
Thus, streamlining the process of installing JavaFX on Ubuntu 20.04 involves intricate steps ranging from installing OpenJDK 11, downloading your latest JavaFX SDK, to setting up environment variables amongst other measures. With these in play, you’re equipped and ready to develop compelling desktop and web applications using this software framework. By following through every stage with finesse, we establish a firm ground for swift programming in this fast-paced technological era, giving us an edge in application development projects.
Here is a brief code snippet to showcase how you can check if Java is installed on your system:
$ java -version
By executing this command, you should be able to view the version of Java present on your system.
The subtle beauty of programming lies in our ability to innovate while continually learning. A commonly shared sentiment by Edsger W. Dijkstra is, “Program testing can be used to show the presence of bugs, but never to show their absence!” This aligns perfectly with our continual journey of exploration and improvement in the world of programming.
For further clarification and steps-by-step guide, refer to this online resource: Introducing JavaFX – Installation Guide. Stick around and stay driven for more enriching insights into Java and related technologies.