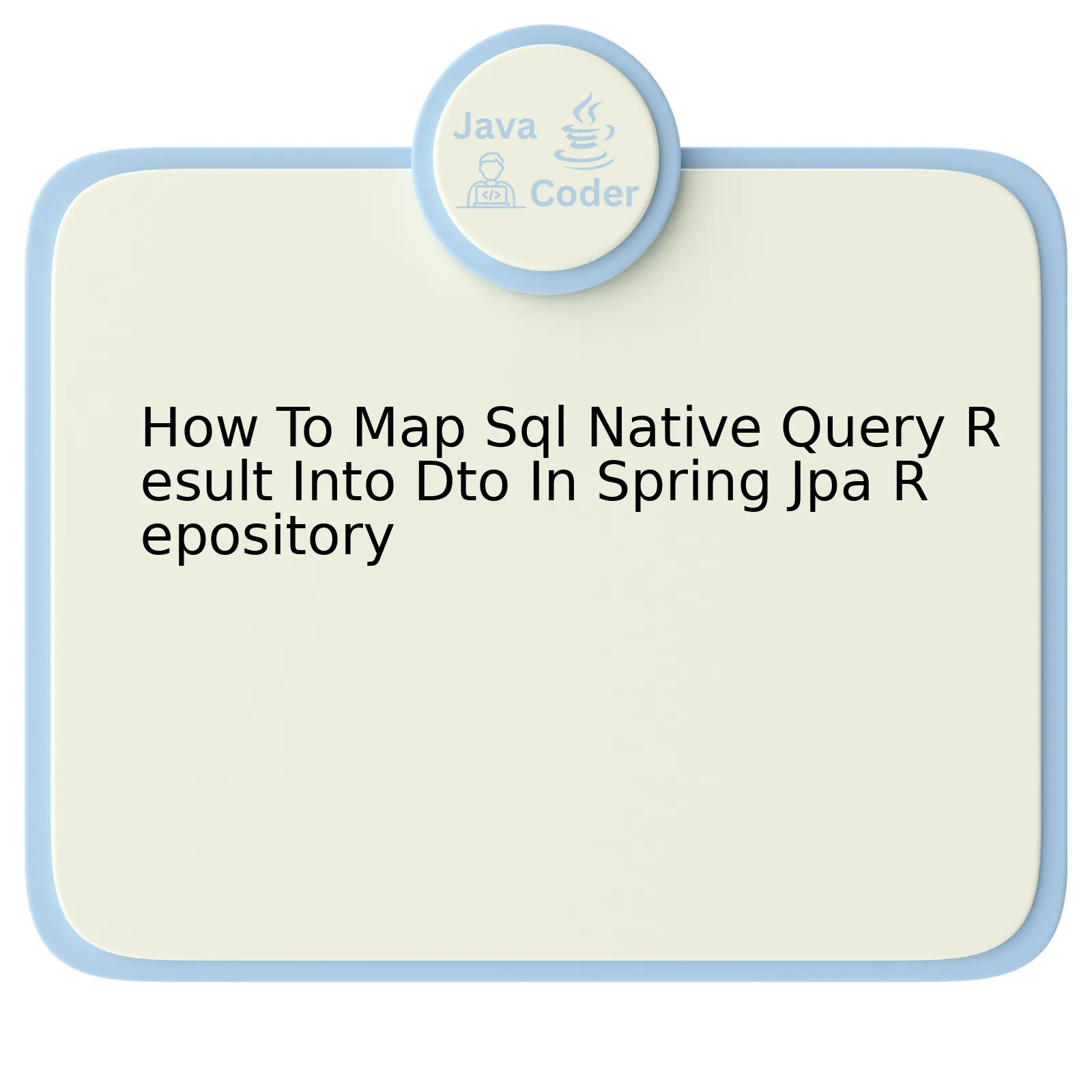
Mapping SQL native query result into DTO in Spring JPA repository is a process that involves the use of Data Transfer Objects (DTOs) to map the results of SQL queries into objects suitable for application use.
To achieve this, it is crucial to follow a set of methodical steps as outlined below. However, bear in mind that these steps are underpinned by thorough understanding of SQL, Java, and Spring JPA.
Here’s a representation capturing key elements and their interrelationship:
Field | Description |
---|---|
DTO |
A Plain Old Java Object which serves to transport data between processes |
@SqlResultSetMapping |
An annotation marking a ResultSet mapping strategy |
@NamedNativeQuery |
An annotation storing SQL queries at runtime |
JPA Native Query |
The raw SQL for communication with the Relational Database |
Spring Repository |
The interface used for database manipulation in the Spring Framework |
This relationship can be further articulated by detailed explanation:
1. Create a specific
DTO
. This also known as a Plain Old Java Object (POJO) is created to transport data between processes in a way that transcends the preferences of a domain model layout.
2. Annotation Via
@SqlResultSetMapping
. This annotation is integrated into the Java class file, outlining the strategy for mapping the ResultSet returned from an SQL query into the object format required by your application.
3. Deploying
@NamedNativeQuery
. This feature annotates SQL queries at runtime, enabling their storage and subsequent execution at various points within your codebase.
4. Execution of the
JPA Native Query
. This is the raw SQL that communicates directly with your Relational Database, intending to return exactly the information needed with minimal overhead.
5. Use of
Spring Repository
. The object association gets mapped within the java interfaces used for database manipulation in the Spring Framework.
According to Bill Gates, “The computer was born to solve problems that did not exist before.” Just about right for this scenario, considering the problem solving capabilities of Java and SQL when synergistically harnessed in the Spring Framework.
Each of these steps is crucially important and has its part to play in ensuring that the resultant DTO contains the data in the desired format. This makes processes like querying, data transfer, and manipulation more efficient.
Understanding Spring JPA and DTO in SQL Mapping
Understanding Spring JPA and DTO in SQL Mapping entails a comprehensive grasp of the primary functions of these pivotal aspects of Java programming. This knowledge is crucial when mapping SQL native query results into DTOs in a Spring JPA Repository.
Essentially, Spring JPA (Java Persistence API) is a specification that facilitates object-relational mapping to manage relational data in Java applications. It simplifies the development process by providing a platform to perform powerful database operations. On the other hand, a Data Transfer Object (DTO) is an object carrying data between processes, aiding in the reduction of unnecessary methods to get and set data.
However, mapping SQL native query results into DTO directly cannot be achieved due to the projection limitation of the @Query annotation in Spring JPA Repository. The projections are only available for managed entities, hence the necessity to return an array of objects or list then manually map these elements into the DTO.
// Assume this is your DTO class MyDto { private int id; private String name; // getters and setters... } // In your repository @Query(value = "SELECT new com.example.MyDto(e.id, e.name) FROM Entity e WHERE e.name = :name") ListfindDtoByName(@Param("name") String name);
In the provided sample code, the Spring JPA is used to run a custom query using the @Query annotation. The query will return a list of type
MyDto
which can directly use an entity’s specific properties.
An alternative way of performing this task is through using a Result Set Mapping which maps the SQL query result into DTO by defining the mapping relationship via annotations.
@SqlResultSetMapping( name = "MyDtoMapping", classes = @ConstructorResult( targetClass = MyDto.class, columns = { @ColumnResult(name = "id", type = Integer.class), @ColumnResult(name = "name", type = String.class) } ) ) @NamedNativeQuery(name = "Entity.findDtoByName", query = "SELECT e.id, e.name FROM Entity e WHERE e.name = :name", resultSetMapping = "MyDtoMapping") // Then you can use the named query public interface MyRepository extends JpaRepository{ List findDtoByName(@Param("name") String name); }
This code illustrates the instruction by using Named Native Query and Sql Result Set Mapping annotations to define and map the named query result into
MyDto
.
The DTO concept utilized here creates a separation of concerns rendering lower coupling, higher cohesion and easier maintainability. Baeldung has vast resources on DTO usage within Spring applications.
As Reuven Harrison once said, “Treat your code like poetry and take it to the edge of the bare minimum.” Notably, this belief shines within DTO’s design principle; write less, achieve more.
Methods for Mapping Native Query Results to DTO
Mapping native SQL query results directly into DTOs (Data Transfer Objects) in Spring JPA repository can significantly simplify data presentation and transportation. However, it’s important to note that this isn’t a built-in feature of Spring Data JPA but requires specific configurations.
Method 1: Using a @SqlResultSetMapping Annotation
One common method is the utilization of
@SqlResultSetMapping
, an annotation that maps each returned column in your query to a field in your DTO. Here’s an example:
@SqlResultSetMapping( name="EmployeeMapping", classes = @ConstructorResult( targetClass = com.example.DTO.class, columns = { @ColumnResult(name="column1", type=String.class), @ColumnResult(name="column2", type=Integer.class)})) public class EmployeeEntity { }
You should apply the mapping and run the native query like this:
@PersistenceContext EntityManager em; @JsonProperty public ListgetDtoData { Query q = em.createNativeQuery("SELECT column1, column2 FROM Employee WHERE condition=something", "EmployeeMapping"); return q.getResultList();
Here,
@SqlResultSetMapping
is declared at the entity level while
@ConstructorResult
is used to map the returned columns to the constructor of the DTO class.
Method 2: Using Interface-based Projections
Another approach for mapping native query results into DTOs is using Interface-based projections. When you define an interface projection, you define a nested interface with getter methods for the properties you want to expose:
public interface EmployeeInterface { String getName(); int getId(); }
When the results are retrieved from the database, Spring Data will create proxy instances of these interfaces, effectively creating your “DTO”. The actual SQL query would look something like this:
@Query(value = "SELECT e.name AS name, e.id AS id FROM Employee e", nativeQuery = true) List<EmployeeInterface> findAllDtos();
Both methods provide a means to map your query results directly into DTOs. Your choice depends on the structure of your project and your personal preferences.
It’s undeniable that programming and technology offer a unique kind of creative outlet. As Steve Jobs once said: “I think everybody in this country should learn how to program a computer because it teaches you how to think.”
Remember to always be considerate about best practices depending on the complexity and requirements of your application. If you require more robust DTO transformations or would like to achieve better code structure or inheritance models, additional mappers such as MapStruct or ModelMapper can be utilized.
Advantages of Using DTO in a Spring JPA Repository
Turning towards the relevance: When SQL native queries are used in Spring Data JPA repositories, mapping the results directly into DTOs becomes not only possible but also beneficial in multiple respects:
- Improved Data Security: – By employing DTOs with native query results, you essentially carry out a projection of your data with selective exposure. This means that you can limit exactly what parts of the dataset you want to transport or expose to another layer, thereby improving security.
- Optimization of Network Traffic: – Transferring entire entity objects can lead to unnecessary network usage, especially when only a fraction of the object data is needed. With DTOs, you bundle only the required attributes together, significantly reducing the amount of data transferred across the network which in turn optimizes network traffic.
- Data Customization: – DTOs give you an opportunity to shape your data according to your specific needs. You can mold your DTO with various data combinations, derived properties, or computed fields leveraging the SELECT clause of SQL native query .
Here is how you can map SQL native query result into DTO:
Firstly, define your DTO class:
public class EmployeeDto { private Long id; private String name; // getters and setters }
Then, use it in the Repository interface:
@Repository public interface EmployeeRepository extends JpaRepository<Employee, Long> { @Query(value = "SELECT new com.example.EmployeeDto(e.id, e.name) FROM Employee e WHERE e.name = :name") List<EmployeeDto> findByName(@Param("name") String name); }
In this example, we defined a custom query using the @Query annotation. The key aspect here is the “new” keyword in the query which tells Hibernate to use the specified constructor of the DTO class to populate the database results.
As per Benjamin Franklin’s adage— “An investment in knowledge pays the best interest.” DTO implementation and its proper utilization ultimately lead to improved software efficiency and maintainability.
For more information refer to the Baeldung tutorial on DTO projections in Spring Data JPA.
Implementing and Optimizing SQL Native Queries with Spring JPA
The process of mapping SQL native query results into DTO in Spring JPA repository involves the use of `@SqlResultSetMapping` together with `@ConstructorResult` and `@ColumnResult`. This approach offers a beneficial alternative when JPQL doesn’t suffice, allowing more complex database operations that are only possible with native SQL.
Here I’ll lay out an example characterizing the achievement of such an implementation and optimization. Before venturing further, let’s briefly accost ourselves to a set of necessary concepts:
– DTO: A Data Transfer Object is a plainly structured object that holds data for later processing, primarily used for transferring data over a network or between different application layers.
– @SqlResultSetMapping: An annotation that we use to map whatever comes as a result from a native query to an entity class or other classes.
– @ConstructorResult: It’s embedded inside `@SqlResultSetMapping`; this annotation maps columns in the resultSet to the constructor arguments.
Herein, consider you’ve User Data Transfer object (UserDto) and you want to map the result of a native SQL query into it:
public class UserDto { private long id; private String name; private String email; public UserDto(long id, String name, String email){ this.id = id; this.name = name; this.email = email; } // Getters and Setters here... }
We’ll create a mapping for UserDto using `@SqlResultSetMapping` & `@ConstructorResult`. These annotations go to any entity class:
@Entity @SqlResultSetMapping( name = "UserDtoMapping", classes = @ConstructorResult( targetClass = UserDto.class, columns = { @ColumnResult(name = "id", type = Long.class), @ColumnResult(name = "name", type = String.class), @ColumnResult(name = "email", type = String.class) } ) ) public class User { ... }
Next step is to write our native query inside our Repository interface:
public interface UserRepository extends JpaRepository{ @Query(value = "SELECT u.id AS id, u.name AS name, u.email AS email FROM User u WHERE u.name =?1", nativeQuery = true) List findByName(String name); }
The query indicated will be executed against the database, and its result will be mapped to the UserDto using the specified resultSet mapping.
As Edwin H. Land said, “An essential aspect of creativity is not being afraid to fail”. Mapping native queries might seem advanced at first, but once adopted, they contribute extensively to scalable applications.(source). SQL native queries paired with Spring JPA present a stronger database interaction approach, sustaining the application’s optimization and performance.
Developers dealing with persisting relational data in Java applications often find themselves utilizing SQL native query for certain database operations. More specifically, the direct usage of JDBC is sometimes required to perform SQL-specific queries or updates that are not possible through JPQL or HQL.
In such scenario, it’s integral to understand how to map SQL native query results into DTO in Spring Data JPA Repository. This mapping allows developers to manage and optimize complex database transactions without having to navigate issues related to object-relational mapping.
Utilizing DTO (Data Transfer Object) provides the opportunity to control exactly what data your application needs to ensure efficiency in passing data across layers. A usual class can be transformed into a DTO by implementing serializable interface and having fields corresponding to the columns returned by your native SQL query.
@Entity @SqlResultSetMapping(name="ReviewMapping", classes = @ConstructorResult( targetClass = ReviewDto.class, columns = {@ColumnResult(name = "review_id"), @ColumnResult(name = "user_name"), @ColumnResult(name = "rating")}) ) public class Review {} ReviewDto.java : import java.io.Serializable; public class ReviewDto implements Serializable { private Long reviewId; private String userName; private Integer rating; // constructors, getters and setters }
Running a native query involves @Query annotation combined with ‘nativeQuery=true’.
@Query(value = "SELECT review_id, user_name, rating FROM my_table WHERE rating = :rating", nativeQuery = true) ListfindReviewsWithHighRating(@Param("rating") int rating);
This code snippet maps SQL native query result into ReviewDto using Spring Data JPA Repository. The ‘nativeQuery=true’ instruction tells the persistence provider to utilize the SQL as it is written.
The ability to directly use SQL in your Java applications opens up doors that otherwise wouldn’t be possible. You can tailor your SQL to a specific DBMS if needed and perform complex actions on the server side. As Alan Kay, a computer scientist, once said, “The most dependable way to predict the future is to create it.” Mapping SQL Native queries into DTO in Spring JPA Repository allows you to create a future where Java applications coexist harmoniously with native SQL interactions, leading to more efficient and adaptable software.