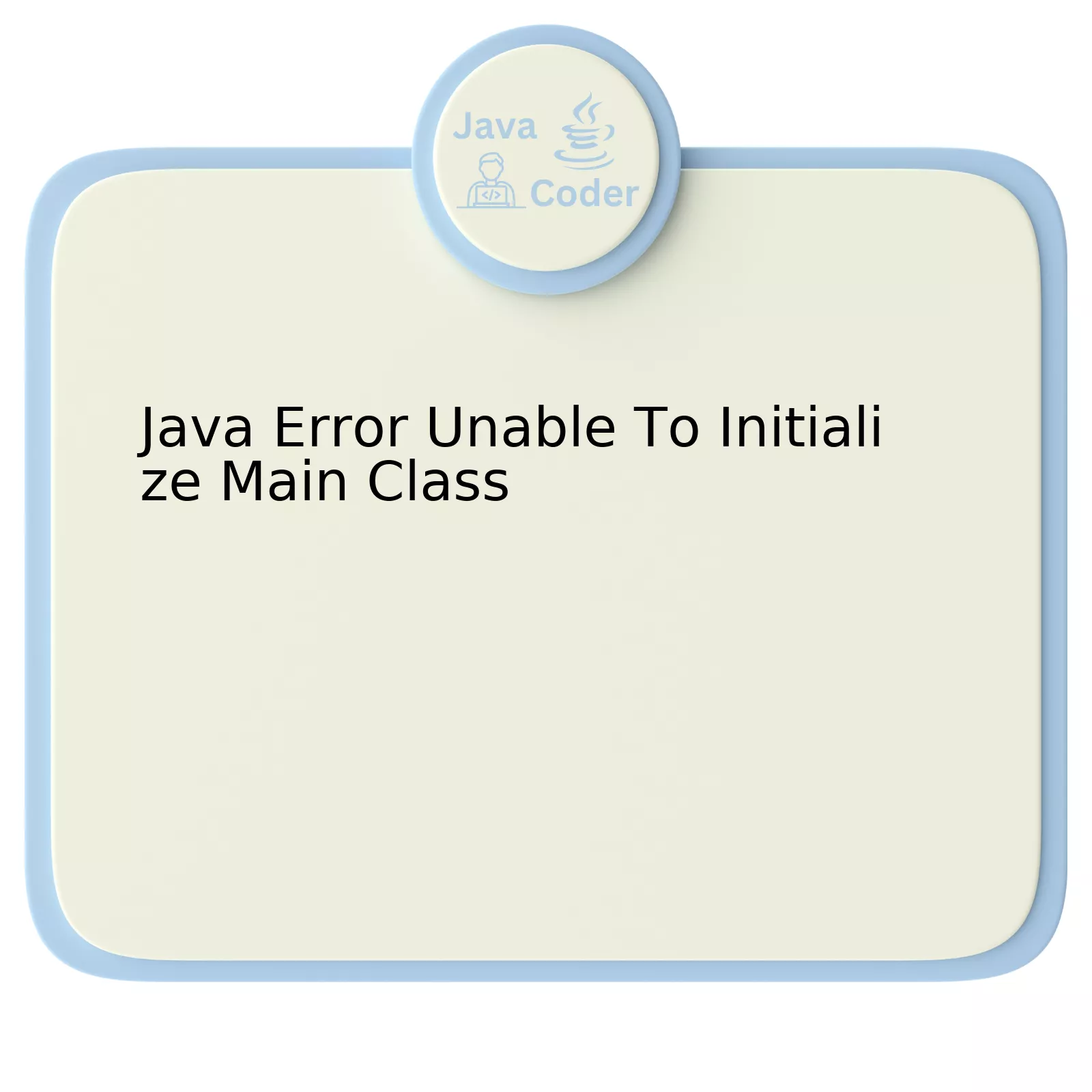
Error Category | Error Message | Possible Causes |
---|---|---|
Initialize Error | Unable To Initialize Main Class | Main class not found or incorrectly named, Incorrect classpath configuration, Buggy Code in static initializer block |
One of the most common errors Java developers encounter is being “unable to initialize the main class.” This error falls under the category of initialization errors, and its key symptom is an explicit error message which states, “Unable to Initialize Main Class.”
The primary cause of this error often deals with issues around the main class. The Java Virtual Machine (JVM) might be unable to find the main class, despite it being defined within your code. This usually occurs if the main class has been incorrectly named or it doesn’t exist at all. Therefore, always ensure that the main class is correctly specified and defined within your code.
Another significant contributing factor to this error is improper configuration of classpath. The classpath is essentially a parameter—either on the command-line (as an environment variable setting) that specifies the location of user-defined classes and packages. The JVM will use classpaths to hunt for .class files to execute your program. If there are any discrepancies in the classpath configuration, it could result in the “Unable to initialize main class” error.
Lastly, the presence of buggy code, particularly in a static initializer block, also can lead to this error. In Java, static initialization blocks get executed when the class is loaded into memory. If there’s any exception thrown during this phase and it’s unhandled, the JVM will exit.
Here’s an example :
public class MyClass { static { // Static initialization block incorrectMethod(); / /This does not exist, leading to an error } public static void main(String [] args){ System.out.println("Hello World"); } }
As such, fix any errors presented in these blocks to prevent the “unable to initialize main class” error.
Remember, as Brian Kernighan once stated, “Debugging is twice as hard as writing the code in the first place … if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it”. It underscores the importance of clean and error-free coding practices.
Understanding the Java Error: Unable to Initialize Main Class
Delving into the realm of Java programming, a common issue that software developers may confront is the error:
Unable to Initialize Main Class
. This error usually indicates that either the Java Virtual Machine (JVM) is unable to locate your main class or your class doesn’t contain a main method.
To better understand this issue, it’s crucial to unravel what a main class in Java signifies. A main class is the entry point of any standalone Java application with a main method as its core element. The signature of the main method must remain accurately structured and static. Here’s how it should typically look:
public static void main(String[] args) { // Your code // }
The main method gets automatically executed when a Java application starts, serving as the initiation point for any standalone Java software. If the JVM cannot identify this method due to an erroneous structure or if the class is inaccessible, it leads to the
Unable to Initialize Main Class
error.
For instance, let’s consider you have a class named HelloWorld stored in a file called HelloWorld.java, and it consists of the main method:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Compiling and running the program involves the use of the following commands:
1. javac HelloWorld.java – Compiles the Java file.
2. java HelloWorld – Runs the resultant bytecode on JVM.
However, if you attempt to run the file without properly compiling it or misallocate the filename, you will face the
Unable to Initialize Main Class
error.
Another scenario involves an incorrect package declaration at the top of your Java file, which could also trigger this error.
To effectively counter this issue:
* Validate that the class name specified while executing the “java” command matches the one in the .java file.
* Ensure the command-line arguments are correct, especially while dealing with packages.
* Maintain accurate structure and accessibility of the main method.
As famously quoted by the renowned computer scientist Donald Knuth – “Beware of bugs in the above code; I have only proved it correct, not tried it.”- debugging forms a crucial aspect of any coding journey and the understanding of such errors enhances our proficiency in Java programming.
Common Causes of the ‘Unable to Initialize Main Class’ Error in Java
When you encounter the “Unable to Initialize Main Class” error in Java, it implies your JVM (Java Virtual Machine) is not identifying the main class for execution. This stumble can be accredited to a multitude of reasons, including but not limited to:
Invalid Classpath
The classpath refers to the parameter that your Java Compiler uses to define the location of user-defined classes and packages. Acquiring an error may indicate an improper setting or declaration of the classpath, making it impossible for the JVM to locate and initiate your main class.
java
javac -classpath . HelloWorld.java
In the command above, the period signifies the current directory. It tells the compiler that our classes are in the current directory.
Incorrect Packaging
This situation arises when the package structure of the class isn’t effectively replicated in the filesystem. The Java compiler requires consistency between your class package configuration and file system directories to correctly locate class files.
Here’s what your package-filesystem relationship should look like:
java
package com.example.hello;
public class HelloWorld {
}
With such package declaration, `HelloWorld` class should reside `com/example/hello` folder relative to the classpath root.
Missing Main Method
The `
Main()
` method serves as the entry point for any standalone Java applications, and its absence would trigger this error. When working on multiple classes, ensure one of them has the main method defined appropriately.
java
public static void main(String[] args)
Naming Mistakes
Incorrect spelling or case of the main class at runtime causes the JVM to fail in recognizing the main class. Double-check case sensitivity also since Java is case-sensitive.
Here is the correct way to run a program with fully qualified classname:
java
java com.example.hello.HelloWorld
Renowned computer scientist Tony Hoare, known for his contributions within the realm of algorithms and data structures, once asserted, “There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other way is to make it so complicated that there are no obvious deficiencies.” This statement accentuates the essence of these solutions – they offer simplicity in troubleshooting a complex problem.
For more information regarding these errors and their appropriate fixes, drive towards Official Oracle’s Troubleshooting guide.
Proven Solutions for Resolving Java Initialization Errors
Developers often encounter “Error: Unable to initialize main class” in their Java applications. This error arises due to a number of scenarios, and typically inhibits the initialization process of the main method – the entry point for any Java application. Here are some proven solutions to tackle and mitigate this Java initialization error:
Check Your Classpath:
Your Java application environment leverages the classpath to locate compiled classes. When it cannot find your ‘main’ class file, an initialization error comes up. Countercheck that your classpath includes the directory or jar file containing your main class.
Consider this sample command line approach to run your Java application:
java -cp /path/to/your/classes com.example.YourMainClass
In the above command:
- -cp is used to specify the class path.
- /path/to/your/classes should be replaced with the actual directory containing your classes.
- com.example.YourMainClass represents your main class, including its package name.
Verify The Package Declaration:
Every Java class is part of a package; the default package if not explicitly defined. If you have packaged your main class, ensure you run the class with its fully qualified name (i.e., include the package name).
For example, consider a Main class declared as follows:
package com.myproject; public class Main { public static void main(String[] args) {...} }
You ought to run the class using its fully qualified name like this:
java com.myproject.Main
Errors regarding unable to initialize the main class can be very frustrating, however understanding the root cause can help expedite resolution. In the words of Adele Goldberg, a renowned computer scientist, “A successful design is not the achievement of perfection but the balancing and integration of the parts.”
Remember—a key principle in software development is ensuring accuracy in defining the structure and dependencies of your project. Applying these methods should provide useful for resolving Java Initialization Errors related to initializing the main class.
Preventing the ‘Java Error: Unable To Initialize Main Class’ In Future Projects
The “Java Error: Unable to Initialize Main Class” is a common issue that developers encounter while running Java applications. Such an error can be quite vexing, disrupting the normal execution flow of your application. Its resolution is imperative for the smooth functioning of your work.
Firstly, this error arises primarily when the Java Virtual Machine (JVM) cannot locate the main class mentioned in your MANIFEST.MF file within the JAR archive of your Java project or when no class containing the primary method has been defined. When using the
java
command on your terminal or interacting with your IDE, it’s intended to locate the main() method which serves as your application’s entry point.
To avoid encountering the ‘Unable to Initialize Main Class’ issue in future projects, you can imbibe these practices in your Java application development process:
• Ensure Accurate Main Class Declaration: Always validate the correctly spelled and correct main class name including its package name if it exists. For example, if you have a package named
com.company.project
and the main class is
MainApplication
, the complete main class reference will then be
com.company.project.MainApplication
. Consider the case-sensitive nature of Java classes; therefore, ensure accurate nomenclature at all times.
// MainApplication.java package com.company.project; public class MainApplication { public static void main(String[] args) { // Your code here } }
• Proper Manifest File Configuration: The MANIFEST.MF file primarily used in packaging a JAR file always specifies the main class of the application. To prevent the aforementioned error, double-check both existence and correctness of this file. If the appropriate main class is
com.company.project.MainApplication
, your MANIFEST.MF file should showcase the following line:
Main-Class: com.company.project.MainApplication
• Leverage Build Tools: Use sophisticated build tools such as Maven or Gradle that automatically generates the appropriate MANIFEST.MF file for you, significantly reducing both the risk of human errors and time spent resolving them.
In the eloquent words of Robert C. Martin, “A programming language is for thinking about programs, not for expressing programs you’ve already thought of. It should be a pencil, not a pen. As you’re coding, you’re invariably modifying your code, and manipulating your thoughts”. In accordance with this quote, adapting preventive strategies, rather than reactive ones, can provide a seamless coding experience across various programming languages including Java.
Finally, keep the lines of communication open with online coding communities such as StackOverflow, GitHub, among others, as they often provide valuable insights and solutions into prevalent programming issues. [StackOverflow](https://stackoverflow.com/) is a commendable resource for any developer, irrespective of their level of expertise.
Remember there’s no magic bullet to prevent this Java error. It often involves a keen understanding of your codebase, paying close attention to details like correctly specifying your main class, accurately configuring your MANIFEST.MF file, effectively using build tools, and engaging in pertinent discourse within coding communities. Adopting these practices not just resolves the ‘Unable to Initialize Main Class’ error, but also enriches your overall programming proficiency.
Delving deeper into the situation, when encountering the Java error ‘Unable to initialize Main Class’, a seasoned Java developer would immediately realize that this error indicates problems with locating or loading the correct “main” method which serves as the entry point for any standalone Java application.
Essentially, this could mean three distinct things:
- The specified class doesn't exist in the provided classpath. - The class exists, but does not have a recognized 'public static void main(String[] args)' method. - There's a version conflict. Perhaps, your class was compiled using a newer version of Java than you're running.
As a first step towards resolving this issue, validate the name and location of your class file carefully. Make sure it is compiled correctly and resides in the appropriate directory. Crosscheck the classpath and verify if the ‘main’ method declaration is rightfully coded as per standards.
Expectantly, tackling these initial steps should resolve the ‘Unable to initialize Main Class’ error. However, if the problem persists, then one may resort to extensive debugging strategies or consider seeking help from online java developer communities [Stack Overflow], [Oracle’s Java Community] where a pool of experts provide prompt resolutions to myriad Java related issues every day.
Coding errors like ‘Unable to initialize Main Class’ can indeed be a little daunting at first. It invokes what renowned computer scientist Donald E. Knuth would say: “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” But fear not! With systematic approach and sound knowledge of Java basics, resolving such errors becomes a breeze even for novice programmers. So keep learning and hone your Java skills to transform these hurdles into stepping stones towards your stride in becoming an adept Java developer.