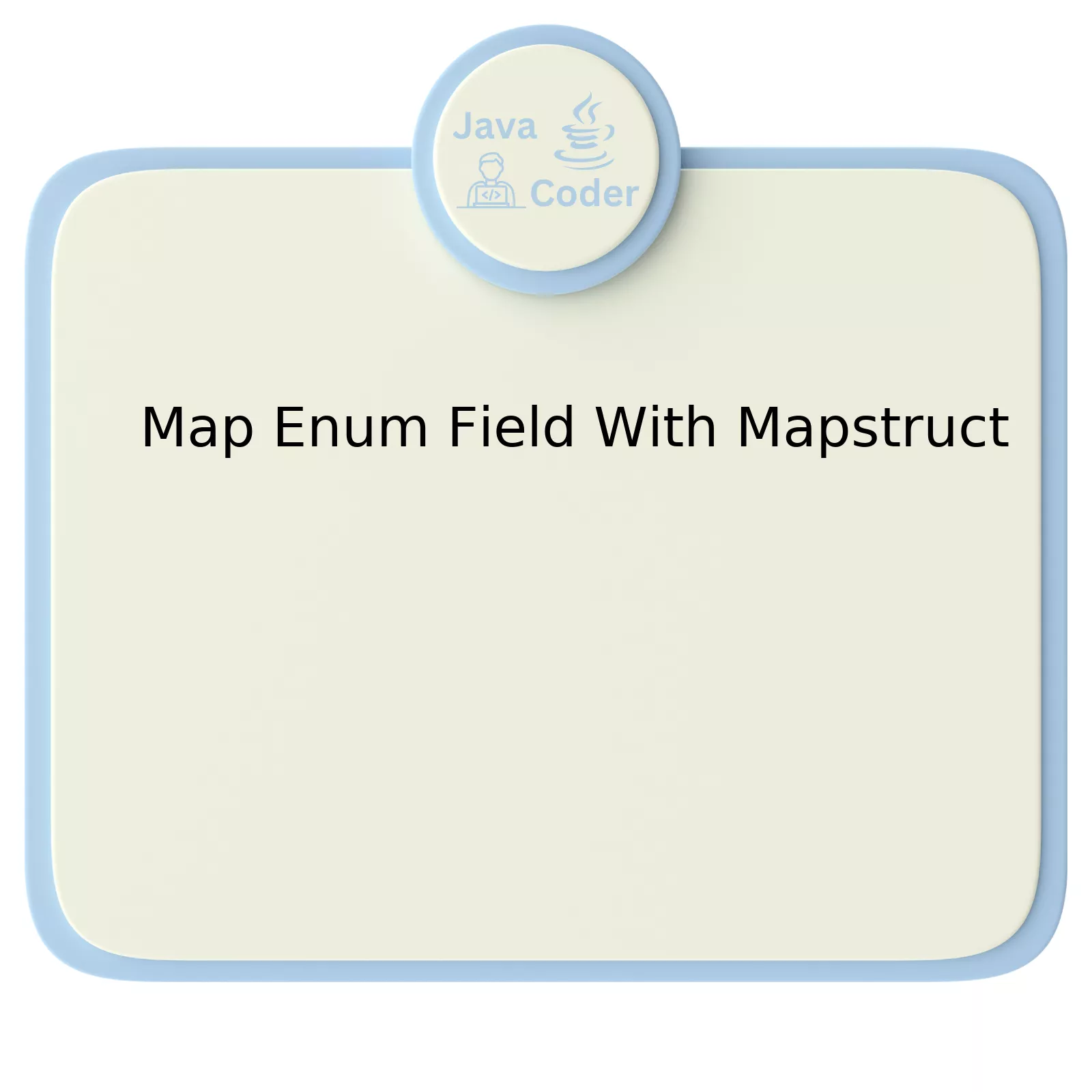
Mapping Enum fields with Mapstruct can be accomplished by leveraging the built-in methods of Mapstruct. Firstly, let’s dive into what a typical data structure might look like using this technique through the illustration below:
Original Enum field | Mapped Result |
Status.IN_PROGRESS | “In Progress” |
Status.COMPLETED | “Completed” |
Status.FAILED | “Failed” |
Herein, the `Status` is an Enum and the output is its mapped String value.
Let’s proceed to understand how we can achieve this mapping using Mapstruct.
Primarily, you’ll need to define a Mapper interface using “@Mapper” annotation. Inside this interface, create a method to transform Enum values to their applicable String format. For example:
@Mapper public interface StatusMapper { @Mapping(source = "IN_PROGRESS", target = "In Progress") @Mapping(source = "COMPLETED", target = "Completed") @Mapping(source = "FAILED", target = "Failed") StatusToString(Status status); }
Assign source as the Enum field and target as the intended final String representation. The Mapping annotations will tell MapStruct how to convert from one to the other.
However, it’s important to know that certain caveats exist in this approach. Developer discretion is required to handle null or undefined values. Additionally, anytime a new Enum value is added, corresponding changes are needed in the Mapper definition which might increase maintenance effort.
This highlights why Steve McConnell, renowned author of Code Complete, says “_Good code is its own best documentation. As you’re about to add a comment, ask yourself, ‘How can I improve the code so that this comment isn’t needed?’_”. In line with his statement, it emphasizes on writing clear and self-explanatory code which is both efficient and easy to maintain. Hence, choosing appropriate mapping techniques can tremendously enhance the readability and maintainability of your code.
To have a further deep understanding on the topic, refer to Mapstruct Official Documentation.
Understanding Map Enum Field with Mapstruct
Mapstruct is a well-recognized code generation library that helps Java developers optimize their application’s performance. Its use case involves converting DTO (Data Transfer Objects) to entities and vice versa with simplicity and ease, minimizing repetitious coding effort. An intriguing aspect of using Mapstruct is it’s ability to map Enum fields, which can be a bit challenging for developers due to the conceptual complexity involved in linking such distinct data resources.
Consider this scenario: You have an Enum field in both your Entity class and DTO class, but in one, it is stored as a String, while in the other, it’s stored as an Enum. Here’s how you might configure this:
The Entity class:
java
public class Employee {
private long id;
private String name;
private String employeeStatus; // Employee status stored as a string.
}
The DTO class:
java
public class EmployeeDTO {
private long id;
private String name;
private EmployeeStatus employeeStatus; // Status field defined as an Enum.
}
In this context, an Enum could be declared as follows:
java
public enum EmployeeStatus {
FULL_TIME,
PART_TIME,
CONTRACT;
}
Following is the way how Mapstruct would help in mapping Entity and DTO:
java
@Mapper
public interface EmployeeMapper {
@Mappings({
@Mapping(target = “employeeStatus”, source = “employeeStatus”, qualifiedByName = “stringToEnum”)
})
EmployeeDTO employeeToEmployeeDTO(Employee employee);
@Named(“stringToEnum”)
default EmployeeStatus map(String employeeStatus) {
return EmployeeStatus.valueOf(employeeStatus);
}
}
So, Things we should notice here:
-
@Mapper
annotation identifies our interface as mapper.
-
@Mappings
annotation where we specified how to map the non-identical fields.
- A default method
map
which takes a String as a parameter and returns corresponding Enum value.
- A single line of code
EmployeeStatus.valueOf(employeeStatus)
, automatically handles the conversion from the String ’employeeStatus’ to Enum ‘EmployeeStatus’.
This approach by Mapstruct facilitates efficient, secure and reusable mapping, thereby simplifying complex code management tasks.
As the Software Engineer Robert C. Martin said “Clean code always looks like it was written by someone who cares” Mapstruct certainly allows Java developers to show that care through their code.
Mapstruct’s Role in Converting Between Java Enums
MapStruct, a notable tool in Java’s ecosystem, plays a pivotal role when converting between Java Enums. Leveraging this annotation processor, developers can promptly implement mappings between differently named Enum fields, setting the stage for cleaner and more maintainable code.
Initially, you might find yourself battling with how Map Enum Field functions using Mapstruct, especially if you’re new to it in your development journey. But fear not, the task is straightforward! Let’s peek into how this happens by breaking down the steps:
1. Establishing Enums:
In our Java application, let’s assume we have two different enums – LocalStatus and ExternalStatus. The former is used within our application, while the latter is from an external API.
java
public enum LocalStatus {
ACTIVE,
INACTIVE,
ARCHIVED
}
On the other hand, we have:
java
public enum ExternalStatus {
ON,
OFF,
OLD
}
Our aim here is to map these disparate statuses together.
2. Enum Mapping Configuration:
Making use of Mapstruct, we can establish an interface – statusMapper – which will perform the mapping operation for us:
java
import org.mapstruct.Mapper;
@Mapper
public interface StatusMapper {
StatusMapper INSTANCE = Mappers.getMapper( StatusMapper.class );
@Mapping(source = “ON”, target = “ACTIVE”)
@Mapping(source = “OFF”, target = “INACTIVE”)
@Mapping(source = “OLD”, target = “ARCHIVED”)
ExternalStatus toExternalStatus(LocalStatus localStatus);
}
The mappings are defined explicitly between each pair of corresponding Enum values. Evidently, ‘ON’ maps to ‘ACTIVE’, ‘OFF’ to ‘INACTIVE’, and ‘OLD’ to ‘ARCHIVED’.
3. Putting the Mapstruct to Work:
Once the configuration interface is set, use the following command to convert LocalStatus to ExternalStatus using MapStruct:
java
LocalStatus localStatus = LocalStatus.ACTIVE;
ExternalStatus externalStatus = StatusMapper.INSTANCE.toExternalStatus(localStatus);
Voila! Your conversion is complete.
As always, remember that Java enums add robustness to our code, letting us constrain variables to predefined constants while ensuring errors are caught at compile-time rather than runtime. In conjunction, tools like MapStruct help streamline conversions between them, thereby upholding code elegance and simplicity.
“A good programmer is someone who looks both ways before crossing a one-way street.” – Doug Linder. This holds true when working with technologies like Java and MapStruct. Always look out for ways to reduce boilerplate code, improve reusability, and enhance overall code quality. Overlooking a simple enum mapping could lead to pitfalls in the future. So, be thorough and reap its technical rewards.
Optimizing the Use of MapStruct for Enum Mapping
MapStruct is a highly-efficient Java annotation processing utility used for the purpose of generating type-safe and performant code. Particularly for enumerations (enums), utilizing MapStruct could be very fruitful in enhancing productivity while maintaining cleaner code.
Firstly, we must understand the way enums are generally mapped. Typically, an enum-to-enum type conversion using MapStruct entails having similar enum types on both sides (Source class and Target class).
Below code snippet uses
AnimalEnum
from source to map
PetEnum
in target:
java
public enum AnimalEnum {
DOG,
CAT,
HAMSTER
}
public enum PetEnum {
DOG,
CAT,
HAMSTER
}
The mapping interface will look like this:
java
@Mapper
public interface AnimalToPetMapper {
PetEnum animalToPet(AnimalEnum animal);
}
However, often our source/target enums are not identical. In such cases, MapStruct allows us to create custom mappers. For example:
java
@ValueMappings({
@ValueMapping(source = “HAMSTER”, target = “GUINEA_PIG”),
@ValueMapping(source = “DOG”, target = “DOG”),
@ValueMapping(source = “CAT”, target = “CAT”)
})
PetEnumEnhanced animalToPet(AnimalEnum animal);
These examples depict a fairly simple use-case. Enumerations get more complex when dealing with databases where marks/labels don’t always correspond with schema labels.
When it is necessary to map enums to String (or another basic data type) and vice versa, MapStruct wouldn’t do it automatically. But, we can employ Expression or Constant mapping strategy which Mapstruct provides. Let’s see an example:
java
@Mapper
public interface AnimalToStringMapper {
@Mapping(target = “pet”, constant = “Dog”)
String animalToString(AnimalEnum animal);
}
In this case, regardless of what the input enum value is, the returned output string will always be “Dog”. This approach comes in handy while dealing with default values.
Steve Jobs once said, “Simple can be harder than complex.” While that might be true for transforming raw creativity into elegant products, in coding, we have tools like MapStruct to alleviate the complexities of object mapping.
Remember to refer to MapStruct’s API documentation which comprehensively covers these implementations and more.
Incorporating MapStruct for enum mapping requires a thoughtful plan – pay attention to each source and target field and plan your mappings accordingly. MapStruct offers several methods and strategies to optimize conversions between different enum types.
Techniques for Handling Complex Enum Mapping Cases with MapStruct
As Java developers, it’s often important to navigate around complex Enum mapping situations while utilizing MapStruct, a popular tool known for simplifying bean mappings. MapStruct operates via generating implementation code at compile-time, driving high performance runtimes with no reflection needed. However, when dealing with Enum fields, certain complexities might surface, requiring special handling techniques.
Here are a few strategies involved in managing advanced enum mapping cases using MapStruct:
Default Mapping
A straightforward technique is the default mapping offered by MapStruct. This approach works well when the source and target Enums share the same constant names. The mapping process, in this case, matches both Enums based on consistency among these designations.
For instance, consider two Enum classes –
SourceStatusEnum
and
TargetStatusEnum
. MapStruct can successfully map these if both Enums contain same constants.
public enum SourceStatusEnum { ACTIVE, INACTIVE } public enum TargetStatusEnum { ACTIVE, INACTIVE }
Mappings Annotation Usage
When the constant names across those Enum classes differ, MapStruct’s @Mappings annotation comes into play. You would use the @Mapping annotation to specify the source and target constants explicitly. It’s essential here to remember that each mapping is unidirectional unless otherwise specified.
For example:
@Mapper public interface StatusMapper { @Mappings({ @Mapping(source = "OLD", target = "NEW"), @Mapping(source = "NEW", target = "OLD") }) TargetStatusEnum sourceToTarget(SourceStatusEnum source); }
Custom Converter
If you must manipulate more complicated scenarios or make transformations beyond name mapping, a custom converter method proves beneficial. In such conditions, you define functions in your mapper interface to handle conversions and MapStruct integrates them during the generation process of the implementation code.
For example:
@Mapper public abstract class CustomEnumMapper { public abstract TargetTypeEnum mapSourceTypeToTargetType(SourceTypeEnum state); protected Status convert(String status) { return Status.valueOf(status.toLowerCase()); } }
Take heed of the wise words from Robert C. Martin (Uncle Bob), renowned software engineer, on the realistic take on coding: “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code…[Therefore,] making it easy to read makes it easier to write.”
Unquestionably, this quote reaffirms the approach taken by tools like MapStruct to simplify code readability and maintainability while working with Enum fields mapping. By embracing these methodologies, you can render complexity into simplicity through deft handling of Enum options.
References:
MapStruct User Guide
Intro to MapStruct | Baeldung
Understanding Map Enum Field With Mapstruct
The nuanced concept we explore is mapping enum fields using Mapstruct. Mapstruct conveniently provides mapping capabilities, including the efficient handling of enum field convertion; essentially turning a cumbersome task into a breeze. It involves utilizing annotations for automating type conversions, creating clean and maintainable code for developers.
-
@Mapping(source = "sourceFieldName", target = "targetFieldName")
: This annotation maps source fields to target fields.
-
@Mappings({@Mapping..., @Mapping...})
: These are used when multiple mappings are required.
Developing a mapping strategy for enum fields could involve the setup as illustrated in the code snippet below:
public enum StatusEnum { ACTIVE, INACTIVE } public class Entity { private String status; // other fields, getters, setters } @Mapper public interface EntityToDtoMapper { @Mapping(source = "status", target = "statusEnum") EntityDto entityToDto(Entity entity); }
In this setup, the enum
StatusEnum
and string
status
are mapped efficiently by MapStruct.
It’s fascinating to ponder on Linus Torvalds words, “Talk is cheap. Show me the code.” The intricacies of mapping an Enum field can only be comprehended when experienced with actual coding.
A profound understanding of associating enum fields using Mapstruct not only aids in quality code production but also accelerates the development process. Mapping techniques such as these offer undisputed advantages like cleaner code, simplified debugging, and automatic conversion between types.
You don’t have to see the whole staircase, just take the first step., Martin Luther King Jr.’s thoughtful reflection is very apt in this context. Taking the initial step towards adopting MapStruct for mapping enum fields can translate into significant game-changer for Java developers.
However, the benefits bestow themselves only upon those who venture down this road of implementation. As given MapStruct, each stride yields productivity gains and higher quality code while decreasing potential points of failure.
Each mapping technique has its specific place and use within your codebases. Utilizing these tools correctly essentializes the role of effective software practitioners, bringing us one step closer to creating efficient, maintainable, and bug-free applications. Embrace Mapstruct’s powerful mapping features to leverage these advantages within your own Java projects.