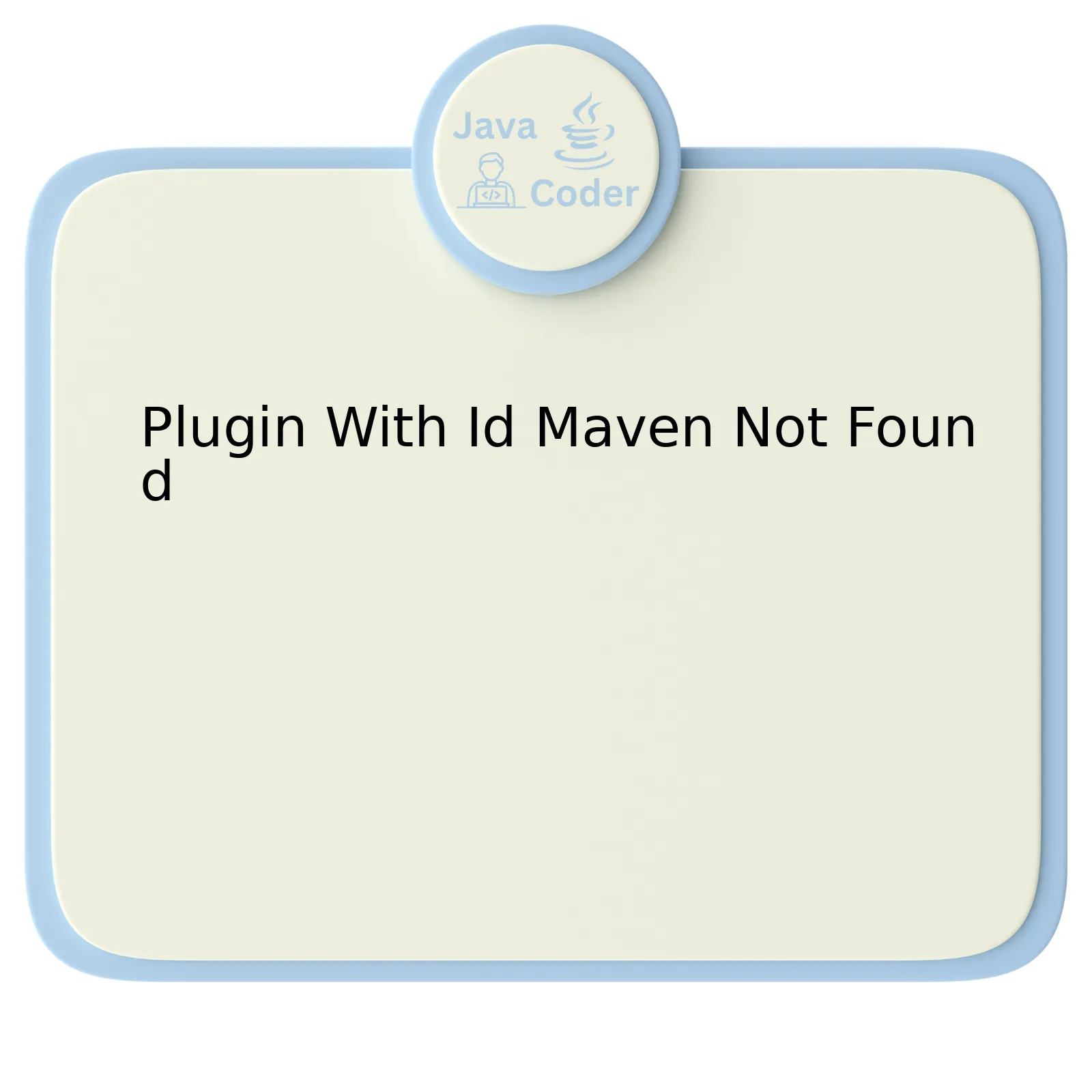
Error Category | Exact Issue | Potential Causes | Suggested Solution |
---|---|---|---|
Maven Plugin Error | Plugin with id ‘maven’ not found |
|
|
Delving further into the matter, this issue revolves around a specific maven plugin error where the system finds it unable to track down the prescribed Maven Plugin. Maven is renowned for managing projects primarily based on Java, and its function involves structuring, documentation, and numerous aspects related to developing a project. Thus, an error associated with a Maven plugin can halt the smooth progress of work.
One potential reason for bouncing against this bottleneck might be missing relevant sections in the ‘build.gradle’ file. It’s essential to correctly implement these sections to ensure smooth operation of your project. Code patterns may look something like:
plugins { id "com.github.dcendents.android-maven" version "2.1" }
Issues related to connectivity with the Maven Central Repository might also classify as plausible cause behind this problem manifesting itself. The central repository is responsible for globally providing updates and plugins required by Maven. If it remains unreachable due to network obstacles or any other unknown issues, such errors can occur.
Lastly, wrongly specifying the groupId, artifactId, or version for the Maven plugin might emerge a source of this anomaly too. It’s vital to maintain accuracy while defining these elements because your build platform depends crucially on them. If any irregularities arise here, they might hinder the loading process of the plugins and result in similar errors.
As quoted by Margaret Hamilton, a pioneering woman in the field of software engineering, “Simplicity & clarity leads to good design”. Adhering to her advice, adopting straightforward solutions can resolve the issue.
Firstly, make sure to add necessary details related to the Maven plugin to your ‘build.gradle’ file. Check out official Maven repositories or documentations for guidance if needed.
Secondly, verify whether the Maven Central Repository is alive and fully operational from your end. Network diagnostics might be warranted if problems surface here.
Finally yet importantly, take correct measures to specify your Maven plugin. Consult prompt sources to find the appropriate groupId, artifactId, and version related to your particular project needs.
By addressing this issue in this way, you should overcome the “plugin with ID ‘maven’ not found” error, allowing you to proceed smoothly with your Java-based project using Maven. Further reference and instructions could be found here .
“Understanding the ‘Plugin with Id Maven Not Found’ Error”
The “Plugin with Id Maven Not Found” error can cause quite a bit of confusion and concern, particularly if you’re new to Maven or just starting your adventure with Java development. This error essentially indicates that either Maven itself is not installed or the specific plugin being referenced in the build script isn’t found.
Causes of Plugin with Id Maven Not Found Error
There are multiple reasons why you might encounter this error. Some of them include:
1. Maven isn’t installed:
Maven is an essential tool for managing Java projects. If Maven isn’t installed on your system, you’ll likely see the ‘plugin with id ‘maven’ not found’ error when running your Maven commands or scripts. Therefore, making the proper installation of Maven crucial.
2. The plugin is not added correctly in the pom.xml:
Every Maven project has a pom.xml file where each dependency and plugin used in the project needs to declared. If the Maven plugin is not correctly specified in the pom.xml, it can trigger this issue.
Below is an example of what a correct definition might look like:
html
3. A network issue prevented the plugin from downloading:
If you experience network issues while downloading and installing Maven plugins, you could encounter this error. A stable internet connection is crucial to smoothly download and add necessary plugins.
Solution to the Error
To address these issues, consider the following steps:
1. Check if Maven is installed: Verify if Maven is correctly installed on your machine. In the command prompt, type
mvn -v
which should return the version of Maven installed.
2. Review your pom.xml file: Make sure the Maven plugin is defined correctly in the pom.xml file. Ensure the defined groupID, artifactID and version match what’s listed in the Maven repository.
3. Verify your network connection: In case of network interruptions during the installation process, ensure you have a stable internet connection and retry the operation.
“Beware of bugs in the above code; I have only proved it correct, not tried it.” – Donald E. Knuth, the famed computer scientist once quoted. Just like him, we too need to perpetually stay vigilant of potential issues that might crop up unexpectedly when working with any programming language or related tool like Java or Maven and devise agile solutions.
“Solutions to Resolve ‘Plugin with Id Maven Not Found'”
When dealing with the “Plugin with Id ‘Maven’ not Found” issue in Java development environments, several solutions can be considered. Alluding before going into details to the root of this problem, we find it commonly arises when there’s a misconfiguration or absence of certain required files within the Maven project settings.
Issue | Solution |
---|---|
Misconfigured pom.xml | Ensure project object model (POM) file carefully written without any syntax error. Also, ensure all desired Maven plugins are properly defined within <build>…</build> tags |
Incomplete IDE Configuration | Certain integrated development environments (IDEs) such as IntelliJ or Eclipse may need additional Maven configuration within their settings to correctly identify and run the Maven plugin. |
Absence of Required Repository | In some cases, failure to find Maven artifact repositories via the specified URLs in your POM file could also lead to this issue. Make sure that you have valid repository URLs, or try switching to a different network connection if possible. |
Missing Maven Installation | You need Maven installed & configured on your machine. Many modern IDEs come with embedded Maven but if that isn’t working or applicable, consider installing Maven locally on your machine and then pointing either your environment variable `M2_HOME` or on IDE level setting to this location. |
The code snippet for a properly defined maven plugin will look like this:
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> </plugin> </plugins> </build>
As an additional note, always HTTPs instead of HTTP for the repository links in your POM file, ensuring secure download of dependencies.
Referencing Kent Beck, the creator of extreme programming: “First make the change easy (warning: this might be hard), then make the easy change”. This quote perfectly fits here as resolving ‘plugin with id maven not found’ might seem challenging at first, but once you’ve overcome it, it becomes a trivial task in your daily coding routine. For reference to more detailed steps, consider visiting online resources, such as Apache Maven’s official plugin guide.
“Impact of ‘Plugin With Id Maven Not Found’ on Project Management”
The error message ‘
Plugin with id: maven not found
‘ is one that developers may come across when they are trying to build projects using Gradle, which is a popular build tool used in Java development. This error message can have significant impacts on project management and the overall software development process.
– The Need for Swift Troubleshooting:
This error can stall the project’s progress because Maven is integral to managing the project’s build life cycle. The ‘maven’ plugin allows Gradle to use Maven conventions, which makes it easier for teams that are transitioning from Maven to Gradle. Therefore, this problem needs swift troubleshooting to maintain efficiency and keep the project timeline on track.
– Potential Delays Project Delivery:
Since Gradle and Maven are essential components in the build and testing phases of a project cycle, an unresolved ‘
plugin with id: maven not found
‘ issue can potentially slow down or halt these phases, causing delays in the project delivery.
– Increased Time and Resource Expenditure:
Addressing this particular error might require additional resources and time, resulting in unplanned costs. Teams may need to consult external experts, spend additional hours diagnosing the issue, or even require training for team members unfamiliar with such problems.
To rectify the ‘
Plugin with id: maven not found
‘ issue, it’s crucial first to confirm the Maven plugin’s presence in the project. You can verify this by checking the Gradle scripts to ensure the Maven plugin is applied correctly within the project setup.
For a regular Java project, the script should resemble:
apply plugin: 'java' apply plugin: 'maven'
Should there be no errors in the project setup, you might want to revise your local setup. Steve McConnell, author of Code Complete, advises “Good programmers know what to write. Great ones know what to rewrite (and reuse).” This wisdom applies well here, as occasionally reinstalling Gradle and Maven on your local machine might resolve the conflict.
Despite facing such challenges, as Linus Torvalds said, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.”
— Remember the end goal, work through obstacles, and enjoy the process.
Maven Lifecycle |
Gradle Build Tool |
Android Dependencies Configurations
“Preventative Measures for the ‘Plugin with Id Maven Not Found’ Issue”
Preventing the occurrence of the ‘Plugin with Id Maven not found’ issue revolves around a few key solutions. This infamous error usually shows up when your development environment fails to recognize Maven, disrupting the smooth flow of your development process. The following information will guide you in tackling this issue efficiently.
Maintaining Correct Directory Structure:
One preventative measure is ensuring the correct directory structure for your projects. Many times, developers overlook the importance of maintaining a proper directory structure. Maven operates based on a standard layout that needs to be organized correctly to prevent errors.
A usual Maven project employs the following structure:
src |-main |--java
This structure needs to be adhered to as it lets Maven identify your source code and compile it without hassles.
Verifying Maven Installation:
Importantly, verifying the successful installation of Maven can ultimately prevent the ‘Plugin with id Maven Not Found’ error. Though seemingly basic, some programmers fail to confirm their Maven setup’s efficiency, leading to issues later on. Developers can verify by running
mvn -version
in the terminal or command-line. If Maven is installed properly, the result should display the specific Maven version in use.
Inclusion of Appropriate Dependencies:
Maven operates based on a project object model (POM), implying that all dependencies required for each Maven project must be accurately represented in the pom.xml file. Neglecting this could cause Maven to return an error due to unidentified dependencies.
For instance, if we need String Utils from Apache Commons, we would add this to our pom.xml within
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.5</version> </dependency>
It is worth noting here, Bill Gates once stated: “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency.” Automation here refers to tools like Maven, which make Java development smoother while enhancing overall productivity. However, using such tools also demands adherence to stipulated principles, including the accurate representation of dependencies in the POM file.
Proper IDE Configuration:
Lastly, configuring your Integrated Development Environment (IDE) appropriately enables seamless recognition of Maven. Ensure that the Maven plugin is enabled and configured with the right settings. Also, the IDE should effectively communicate with your local Maven repository to prevent potential issues.
Solving the ‘Plugin with Id Maven Not Found’ issue predominantly involves an understanding of Maven’s operations and implementing practices aligned to this understanding. This prevention strategy offers an efficient way to combat potential rigors associated with this issue, making your Java development process hassle-free.
From the perspective of a Java developer, encountering a message such as ‘Plugin with Id Maven Not Found’ could be due to several reasons. It might pertain to your project’s
pom.xml
file or it might be because Maven is not properly installed and configured in your system. In both cases, these issues need to be systematically analysed and resolved.
An error like ‘Plugin with Id Maven Not Found’ implies that Maven (a powerful tool widely commercialized for carrying out Java-based project functions, similar to ANT and Gradle), has failed to recognize a certain plugin ID. This incongruity gives rise to problems during the build lifecycle process of your codebase.
Problem | Solution |
---|---|
Maven is not correctly initialized in your System | Validate if Maven has been properly installed. Use the command
mvn --version to check if your system recognises Maven, if not, consider reinstalling it. |
Incorporation issue with the Plugin in
pom.xml |
Ensure firstly that the plugins used in the
pom.xml are compatible with Maven. Secondly, examine the spelling and syntax linked with the plugin ID. Normalizing these can often set right the problem. |
The Maven repository is responsible for managing the library dependencies required by the Maven project. If an essential plugin is not located, the necessary functionality will be removed from this aggregation of add-ons, resulting in an error message.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
As this quote suggests, resolving this issue doesn’t necessarily require rigorous coding but an understandable and logical approach. Rectifying the issue of ‘Plugin with Id Maven Not Found’ primarily involves effective debugging, adept configuration management skills, and proficient handling of XML files. In simple terms, developers are encouraged to ensure correct installation of Maven, and watchful use of plugins in their
pom.xml
file to avoid such discrepancies.
For more details on using plugins in Maven, refer to: Maven Introduction to Plugins.