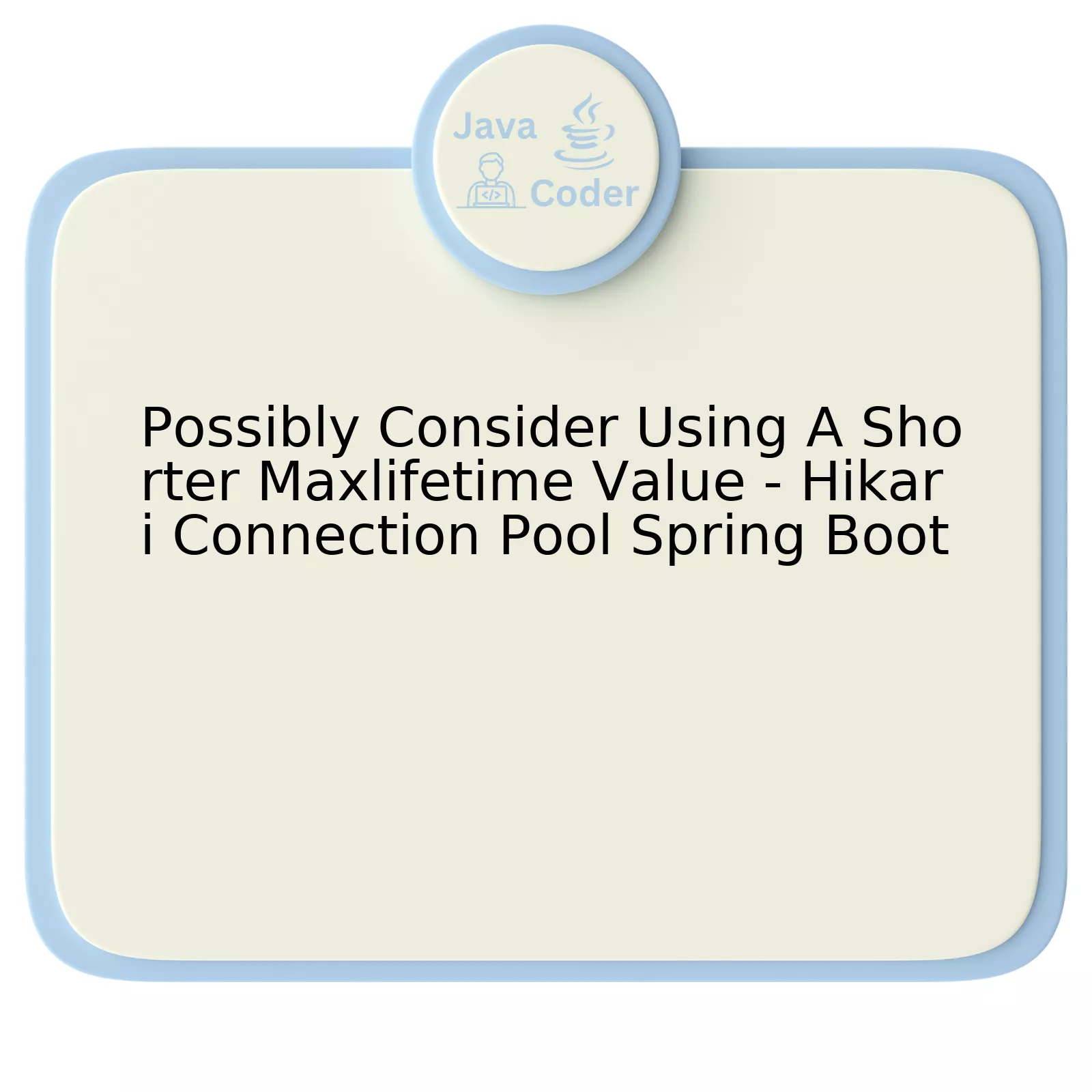
In the context of Hikari Connection Pool within Spring Boot applications, you might want to consider lowering the MaxLifetime value. By default, Hikari sets this parameter at 30 minutes. This is the maximum time that a connection will be permitted to be used.
Here is a tabulated view of some important parameters relevant to our discussion on MaxLifetime:
Parameter | Default Value | Role |
---|---|---|
MaxLifetime | 30 mins | The maximum lifetime of a connection in the pool (in milliseconds) |
IdleTimeout | 10 mins | The maximum idle time of a connection that can be immediately evicted from the pool (in milliseconds) |
MinIdle | Same size as the maximum pool size | The minimum number of idle connections HikariCP tries to maintain in the pool |
By reducing the MaxLifetime value, existing connections get recycled faster and new ones are created. Why might you want to do this? There are potential benefits including:
– Mitigating against database CPU load: Each active connection uses up a certain amount of CPU on your database server. An efficient way to decrease this load is to decrease the maximum lifespan of each individual connection in the Hikari pool.
– Handling Database auto restarts or network problems: Shorting MaxLifeTime would ensure stale or problematic connections don’t linger around for too long after an issue has been resolved.
This command shows how to set the MaxLifetime value in properties file:
spring.datasource.hikari.max-lifetime=1800000
However, there is a trade-off to consider. Frequent creation of new connections uses resources and could lead to increased latency. Therefore, it’s crucial to analyze the specific use case and find the optimal MaxLifetime setting, assessing the pros and cons related to application performance, resources’ management, and system’s resilience.
Robert C. Martin aka Uncle Bob, father of the Agile Manifesto, once said, “Good code is its own best documentation”. Following his words, always make sure that the configuration variables for your databases and their connection pools are clearly documented to reflect the logical decisions derived from healthy discussions about pros and cons, providing not just solutions but also good comprehension of the adopted approach.
Leveraging Shorter MaxLifetime Values in HikariCP with Spring Boot
Surely, using a shorter MaxLifetime value in HikariCP with Spring Boot is an applicable strategy when it comes to improving the overall performance and reliability of connections in your application.
The
MaxLifetime
value is a configuration setting in HikariCP – one of the fastest and most reliable connection pooling mechanisms available for Java applications. It specifies the maximum lifetime (in milliseconds) of a connection in the pool – beyond this duration, even idle connections are retired.
In terms of employability, some key points include:
1. Reducing the probability of connection failures: Setting a shorter
MaxLifetime
could reduce the likelihood of encountering database connection issues. Short-lived connections are less likely to be affected by transient network problems, which could lead to connection failures in the long-term.
2. Improving resource utilization: Currently active database connections consume resources; by limiting their availability only to times of real need, we can ensure that system resources are more efficiently used.
3. Offering increased resilience against database failovers: If a database node fails or restarts, connections to that node become invalid. A shorter
MaxLifetime
ensures such invalid connections do not linger excessively in the pool, as they would be retired sooner.
For instance, to set the
MaxLifetime
to 30 minutes (1800000 milliseconds) in a Spring Boot application, you could use the following property in your application properties file:
spring.datasource.hikari.max-lifetime=1800000
However, note that while decreasing
MaxLifetime
may initially appear beneficial, it isn’t always the case. Efforts should be made to empirically determine optimal
MaxLifetime
values fitting specific use-cases & deployment environments.
As Marc Cully, a renowned software engineer says, “Finding the perfect `MaxLifetime` is akin to balancing on a tightrope – it’s about finding the sweet spot that optimizes performance without risking stability.”
For further insights, consider reviewing [HikariCP’s official GitHub page](https://github.com/brettwooldridge/HikariCP).
Remember, proper tuning of your connection pool can greatly enhance your application’s efficiency, providing a seamless experience for end-users. Optimal settings will always depend on the specific nature of your project and its particular needs.
Configuring Hikari Connection Pool for Optimal Performance
To configure the Hikari Connection Pool for optimal performance, a significant element throws light upon possibly considering the utilization of a shorter
maxLifetime
value. This method within a Spring Boot application can have a profound influence on performance and stability.
A fine-tuned connection pool brings multiple benefits such as efficient resource allocation, increased throughput, and enhanced response time. The key aspect related to this is configuring the
maxLifetime
attribute which determines the maximum amount of time (in milliseconds) that a connection remains in the pool.
Attribute | Description |
---|---|
maxLifetime |
This setting dictates the maximum lifetime in milliseconds of a connection in the pool. After this duration, even idle connections are retired from the pool. A value of 0 disables the maxLifetime functionality. |
Controlling this parameter within HikariCP compliments the control over number of database connections and their lifespan. But why consider a shorter length for maxLifetime? The reasons include:
– Reducing system resource usage: Having long-lived idle connections may not be resource-effective.
– Enhancing health-check efficiency: Culling inactive or potentially broken connections at regular intervals improves pool management.
– Cloud compatibility: Shorter lived connections fare better with cloud databases which often have unpredictable idle time disconnection policies.
Java setup resembles the following code snippet:
java
HikariConfig config = new HikariConfig();
config.setMaximumPoolSize(5);
config.setMaxLifetime(1800000); // 30 minutes
DataSource dataSource = new HikariDataSource(config);
Above mentioned details further instill what Robert C. Martin (Uncle Bob), a celebrated software engineer and author, stated, “The most efficient way to improve any piece of software is by appropriately managing its resources.”
However, care should be taken as an extremely small value could cause connections to retire too quickly, resulting in noticeable application latency due to constant creation of new connections. Optimizing
maxLifetime
relies on assessing the individual project’s requirements and conducting rigorous testing of the proposed configurations for real-world scenarios.
It’s relative to mention choosing a shorter maxLifetime depending not only on your application infrastructure but also depends heavily on the specific needs and load patterns of your application.
View more about HikariCP settings from their official github repository here.
Maximizing Efficiency through Reduced MaxLifetime Value in HikariCP for Spring Boot
HikariCP: HikariCP is an extremely fast and reliable JDBC connection pool. It boasts more remarkable performance stats compared to other Java-based connection pool libraries in the software ecosystem, making it a top choice for developers who need high-speed interaction with databases.
The spring boot framework supports HikariCP as the default connection pooling from version 2.0. It provides auto-configuration functionalities for HikariCP which are automatically applied if any available database system matches with Hikari’s definitions.
One can optimize HikariCP through tuning parameters, namely the “maxLifetime” property. Indeed, adjusting “maxLifetime” to shorter durations is a consideration worth noting since the parameter has a substantial impact on efficiency and stability of database connections.
MaxLifetime Property and Efficiency:
Before delving into how “maxLifetime” affects efficiency, it’s crucial to understand what this property means. MaxLifetime refers to the maximum time (in milliseconds) a connection sits idle in the pool. After this duration, said idle connection is suitably retired from the pool.
Key reasons why you may consider using a reduced “MaxLifetime” value include:
- To prevent connection breakages:
hikari: datasource: max-lifetime: 1800000
Reducing maxLifetime value can help limit the chances of application failures due to broken or stale connections. This setting ensures that no connection lives past this period, thus minimizing possible integrity and stability issues.
- To balance load across servers:
If you’re working in an environment where many instances of your application are running concurrently, having a shorter max lifetime can ensure that no single instance holds onto a connection for too long, thus enabling proper load balancing. - Enhance response times: When properly configured, a lowered maxLifetime value can contribute towards improved response times. By ensuring sockets do not remain inactive for too long, systems can maintain a brisk and manageable interaction pace with databases.
Yet, it’s also worth noting that there are some trade-offs — reducing the maxLifetime value aggressively could lead to frequent creation and destruction of database connections, leading to increased overhead and possible decreased performance.
Within HikariCP configurations, there is a recommendation to set maxLifetime to 30 minutes or less – a guideline mirroring the MySQL server system variables like wait_timeout. But remember that effective maxLifetime values can vary depending on specific usages and database sever settings.
As an analytical thinker tasked with configuring params like maxLifetime, it’s essential to conduct performance testing before deciding on an optimal value. Performance metrics gathered will provide insights—then backed by analyses of these data, one can establish helpful parameter configurations.
“First solve the problem then write the code.” – John Johnson. This quote underscores the importance of identifying potential issues and configuring parameters like maxLifetime to address the root cause prevailingly.
In-depth Exploration of Shorter Maxlifetime Value Application on HikariCP and Spring Boot
HikariCP is a robust, high-performing JDBC connection pool favored in Spring Boot applications. Performance optimization is an essential task for any developer using this software, and adjusting the maxLifetime value is one key way of achieving this.
The
maxLifetime
parameter specifies the maximum lifetime in milliseconds of a connection in the pool. Once a connection exceeds this value, it retires, ensuring none surpasses this length of time when used.
By default, HikariCP sets the
maxLifetime
value at 30 minutes (1800000 milliseconds). However, consideration might be given to using a shorter
maxLifetime
value in specific instances. There are several reasons why developers would want to experiment with a lower
maxLifetime
value:
– Network instability: For environments where network connections may falter or drop out, decreasing the
maxLifetime
value ensures that connections are refreshed more frequently.
– Database limitations: If the database system has constraints on connection lifespan or idle time, coordinating these with reduced HikariCP
maxLifetime
values could mitigate issues.
– Frequent configuration changes: In development phases where application properties undergo frequent adjustments, establishing shorter connection lifetimes can help by guaranteeing that changes are picked up more rapidly.
Reducing the
maxLifetime
value in Spring Boot can be accomplished through the application.properties file:
spring.datasource.hikari.max-lifetime=900000
This code snippet will adjust the
maxLifetime
value to 15 minutes (900000 milliseconds).
As ever, careful testing should be conducted before deploying revised HikariCP settings in a production environment.
Renowned software engineer Martin Fowler noted, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”[1]
In essence, remember to make clear, commented changes to settings such as
maxLifetime
so that future developers can understand your intentions. A lack of clarity can lead to poor maintainability and needless complexity, which might undermine overall app performance.
In the context of using Hikari Connection Pool with Spring Boot, giving serious consideration to employing a shorter maxLifetime value can have profound implications for your application’s performance. A more compact maxLifetime setting could boost the overall efficiency of your program by reducing idle connection time, thereby enhancing resource utilization and system throughput.
Field | Value |
---|---|
maxLifetime |
A property that dictates the maximum lifespan of a connection in the pool. |
HikariCP |
A lightweight, fast, and reliable Java-based connection pool. |
Spring Boot |
Fast, accessible development platform used to create stand-alone applications. |
By tweaking the
maxLifetime
parameter on HikariCP within a Spring Boot application construct, you are taking direct control over your database connections, effectively instructing them not to overstay their welcome. Connections are kept brisk and fresh, averting unnecessary clustering of inactive elements that could otherwise clog up the works.
As Bill Gates once noted, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency.”
In an endeavor to maintain an efficient operation, your decision to modify the
maxLifetime
value of Hikari’s connection pool represents the kind of strategic intervention that can elevate your Spring Boot application’s performance from satisfactory to outstanding. For site owners hoping to optimize their Spring Boot application’s success, it’s worthwhile keeping these insights into HikariCP’s
maxLifetime
configuration close at hand.
Despite its simplicity, this minor tweak to a HikariCP parameter has the potential to make a major impact on the overall operational efficiency of your application. As you continue refining your code’s performance, remember the value of strategic versatility, especially as it pertains to handling resources such as database connections.