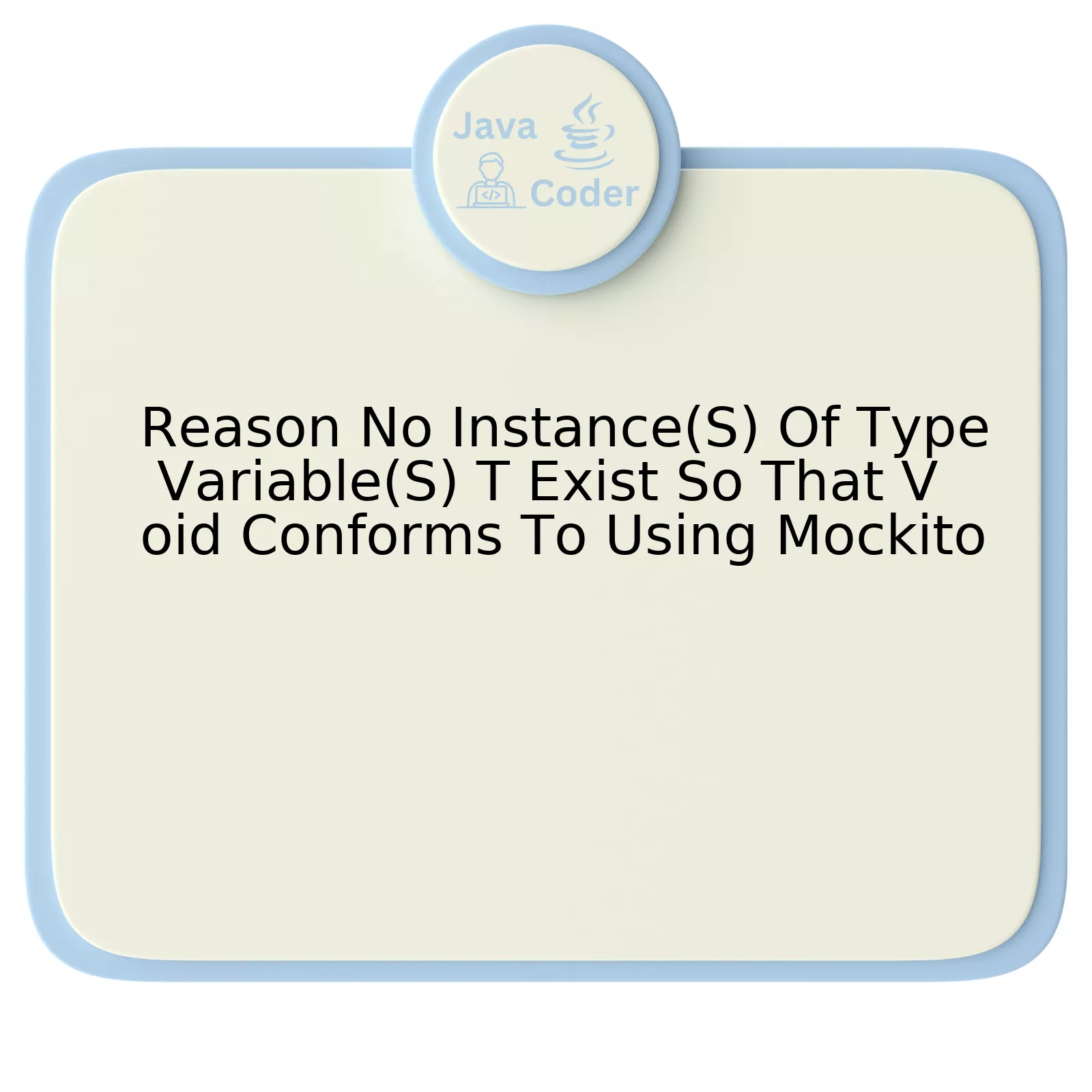
The “No instance(s) of type variable(s) T exists so that Void conforms to” is an error message generally encountered when working with Mockito, a robust mocking framework for unit tests in Java.
First, let’s look at the table highlighting the main components comprising this issue:
html
Component | Description |
---|---|
Type Variables (T) | A type variable is a conceptual placeholder for a concrete type that will be used at runtime, most commonly used within generics. |
Instance(s) | An `instance` typically refers to a concrete realization of any object. When speaking of ‘no instances’, it pertains to a situation where no specific realization of the mentioned type can be found or used. |
Void | In Java, `void` is a keyword that signifies ‘nothing’ or ‘no value’. It’s predominantly utilized to declare method types which do not return any value. |
Conforms to | ‘Conforming to’ in the context of types usually denotes following norms of a certain relation or being usable interchangeably. |
Mockito | Mockito is a prevalent and robust open-source testing framework in Java, which aids in writing high-quality unit tests by creating ‘mocks’ or simulated versions of dependencies. |
Interestingly, in a Java universe, `void` often can’t conform to a Type Variable `T` because `void` is not a type and we also cannot create an instance of `void`. As a result, attempting to do so would produce the said error, particularly while using Mockito.
In the context of Mockito, usually, you’ll encounter this error when trying to mock methods with a `void` return type. Mockito traditionally uses `thenReturn()` for defining mocked behavior which doesn’t work with `void` return type and consequently lands us in the problematic scenario of no instance of `void` conforming to a Type Variable `T`.
Let’s illustrate this with a code example:
java
public interface MyInterface {
void performAction();
}
// In test class
MyInterface mock = Mockito.mock(MyInterface.class);
Mockito.when(mock.performAction()).thenReturn(null); // This line causes the error
Here, Mockito attempts to use `thenReturn()` on a method that returns `void`, which is fundamentally incorrect. The solution here is to use Mockito’s `doNothing()`, `doThrow()`, `doAnswer()` etc. instead:
java
Mockito.doNothing().when(mock).performAction(); // The correct way to mock a void method
In the words of Robert C. Martin, aka Uncle Bob, one of the most important pioneers in software craftsmanship, “The best way to verify your coding is to write a test.” Hence the significance of understanding testing tools like Mockito and their potential errors like “No instance(s) of type variable(s) T exists so that Void conforms to”.
Understanding the Concept: Why Void Doesn’t Conform to Mockito
The concept of using
void
with Mockito can be a bit perplexing for new users due to its peculiar nature in the Java Programming Language. In short,
void
is not a type; instead, it’s a keyword which indicates that a method doesn’t return a value. Therefore, if you’re trying to mock or ensure a particular course of action on a
void
method while unit testing using Mockito, you may encounter some challenges.
This problem can be translated as follows: the conceptual structure holding null (“no instances”) does not match any conceivable data type (“Type Variable(s) T “), including “void”, such that they can get along (“conforms”) smoothly with Mockito during mocking process.
The Reason:
-
Mockito
, like any other tool, has its limitations. For instance, Mockito’s intent is to help design tests for ordinal scenarios but it struggles when an attempt is made to mock void methods.
- Generic Mocks — The difficulty with generic mocks essentially lies in the way generics are used and handled in Java and this misalignment is reflected in error messages similar to ‘no instances of Type Variable(s)’.
- This is further compounded by the fact that ‘void’ isn’t a data type in Java, but merely implies that a method doesn’t return anything.
Thus, the best practices would advice to avoid trying to mock
void
methods. In circumstances where it is absolutely necessary however, “‘”doAnswer()” or “doThrow()” can be used.
As Kevin Henney, a renowned developer and author once said, “The real difficulty in testing and mocking comes from complex dependencies, it does not come from the use of simple returns.”
An example demonstrating how to handle this could look something like:
// Assuming we have a list ListmockedList = mock(List.class); // Mock void method in Mockito doThrow(new RuntimeException()).when(mockedList).clear(); // Using the mock mockedList.clear(); // Will throw RuntimeException
In this example, we’ve successfully mocked the
void
method
clear()
from
List
interface using
doThrow()
built-in Mockito behavior.
For more details, you might want to refer to the official Mockito documentation[1].
[1] Official Mockito Documentation
Mystery Unveiled: The Absence of Instance(s) in Type Variable(s)
Type variable absence in Java, particularly regarding Mockito’s usage, is a topic where few ventures, and confusion often lurks. Instances of type variables like
T
are typically avoided due to their generic nature; they don’t correspond directly to any specific type, making them challenging to instantiate or conform using Mockito.
Suppose you ponder upon the method
void conformsTo(TestInterface<T> test)
. This method takes an instance of an interface, which involves a type variable
T
. Here’s the issue: void methods cannot return any value, including instances of type variables. Additionally, Mockito, a popular framework used primarily for unit testing, isn’t built in a way to create instances of type variables due to inherent limitations.
Why so? Let’s delve deep:
• Type Erasure: Generics in Java, such as
T
, get stripped away at runtime. This process known as Type Erasure, cast generics to their bounds or Object if unbounded makes it impossible for Mockito (operates at runtime) to know what T should be.
• Mockito’s Objective: Mockito was designed with a focus on interfaces mock-up having definitive types. By its very design, dealing with type variables (having unknown specificity by definition) emerges as a formidable challenge to Mockito.
Dealing With Such Cases
For handling such situations, it is advisable to have a constraint on these type parameters like
T extends SomeType
. One also can resort to expressing
T
as a concrete class while using instances of it in place of type variable
T
.
It opens up the possibility to use Mockito for creating mock objects bypassing the limitations posed by using type variables.
As suggested by Alan Kay, a pioneering figure in computer science:
“Simple things should be simple, complex things should be possible.”
Although Java Generics bring along certain complexities, the mechanism supporting software reusability despite intricacies is a testament to the wisdom behind this eminent quote.
Deeper Dive: Insights into Conformation Problems using Mockito with Void
Mockito, a widely used Unit testing framework in Java, allows you to write tests to check the functionality of classes and methods in your program. It’s capable of creating objects and defining return values for method calls on the fly. While Mockito can be very powerful, it does have its limitations. One of the aspects where developers commonly encounter problems is when trying to work with void methods. Furthermore, an error message “Reason No Instance(S) Of Type Variable(S) T Exist So That Void Conforms” frequently perplexes developers during testing.
Generally, this particular error originates when programmers attempt to use Mockito’s ‘when()’ method call on a method that returns void. Yet, by design, Mockito’s ‘when()’ function needs a ‘thenReturn()’ clause, utilising a method returning void leads to the dispatched error since void doesn’t “comply” with any type.(source)
For instance consider:
public class DummyClass { public void doStuff(){ //do stuff } }
Now, let’s assume we’re attempting to perform:
Mockito.when(dummyClassInstance.doStuff()).thenReturn(true);
This will result in a problem because
doStuff()
is void and cannot return anything, thus the error: “Reason No Instance(S) Of Type Variable(S) T Exist So That Void Conforms”.
An appropriate solution involves using
doNothing()
or
doThrow()
rather than
when()
for methods that return void. The correct implementation would be:
Mockito.doNothing().when(dummyClassInstance).doStuff();
or
Mockito.doThrow(new RuntimeException()).when(dummyClassInstance).doStuff();
With the above solution, there will be no more exceptions regarding “No instance(s) of type variable(s)”, as methods that return void are now conforming the Mockito norms.
Jay Fields once said: “I get paid for code that works, not for tests.” This quote highlights that while unit tests are crucial to ensure functionality, the primary aim should always be to build working codes; in other words, ‘functioning software’ should remain the central focus without disregarding the need for robust testing practices.
In-Depth Analysis: Exploring Reasons behind Non-Conformance of Void to Mockito
The non-conformance of void to Mockito, primarily observed in cases where “No instance(s) of type variable(s) T exist,” can be linked back to the complexity of generic types and method invocations in Java, coupled with current Mockito limitations when dealing with these areas.
Here’s an analytical overview of why this non-conformance issue tends to arise:
Understanding the Complexities of Generic Types
Java’s generics are a source of considerable intricacy. When defining generic types, the compiler imposes specific constraints that can prove challenging to work with, particularly in the realm of mocking frameworks. Mocking requires us to understand the target objects’ interfaces, and herein lies the difficulty. The problem occurs because void methods cannot return any value—an invariant principle in Java programming language.
For instance, Mockito’s regular syntax:
when(someObject.someMethod()).thenReturn(someValue);
This presents an issue as you cannot do:
when(someObject.someVoidMethod()).thenReturn(someValue);
because someVoidMethod() is supposed to be a void method.
Dealing with Method Invocations
Any() method is one Mockito matcher method used to match any argument of its kind. Using Java generic methods, matchers define their arguments which pose another complexity for developers as they don’t have real types.
These complexities lead to an inability to create instances of generic-typed objects or methods in some scenarios. Consequently, leading to the error: “No instance(s) of type variable(s) T exist so that Void conforms to T.”
The Void Paradox
Establishing PriorityQueue as the object of mock generates an issue in Mockito that impedes correct matching of void methods. In essence, void methods cannot conform, given that void itself contains no elements to match.
Mockito syntax for void methods:
doNothing().when(mockedList).clear();
In use repository:
doThrow(new RuntimeException()).when(repository).save(user)
As they are shown above, you can only perform certain actions such as throwing an exception, but not return a value.
Dealing with the Issue
To deal with this issue, Mockito provides other methods to handle void values like doAnswer(), doNothing(), doThrow() among others.
Consider revisiting how you manage void returns within your code or investigate alternative testing strategies for better alignment with testing framework requirements.
Also, understanding this from Bryan Gardner – software developer experience manager at IBM – “[A]ll systems we develop need to be tested…the more complex the system, the more extensive the testing required.”
In other words, when faced with complexities such as these, we must adapt our testing measures to ensure all aspects of our systems are thoroughly checked. Fundamental understanding of how these testing tools operate and their exceptions represent vital knowledge in achieving comprehensive and effective software tests.
Delving into the topic of ‘Reason no instance(s) of type variable(s) T exist so that Void conforms to’ while using Mockito in Java development unveils complexities, variances, and nuances. This occurrence is prominently seen during the execution of generic testing in Java, where Mockito—a popular mocking framework—plays a vital role.
Term | Description |
---|---|
Type Variable(T) | A placeholder name for a type to be provided by a programmer. |
Void | A keyword in Java used to specify that a method should not have a return value. |
Mockito | A mocking framework broadly used in writing tests for Java applications. |
Code comprehension often becomes obscured when it highlights ‘no instance(s) of type variable(s) T exists so that Void conforms to’. In simplification, this essentially signifies the non-compliance of Void with the speculated instances or objects of the Type variable T.
public <T> void someMethod(T t) { }
The Void here serves as the return type of the method, whereas T gauges to be a type variable passed as a parameter.
The problem surfaces when Mockito steps in to orchestrate unit tests. Mockito’s stance on allowing mock creation primarily for interfaces and non-final classes underlines its limitations. Peering into the scenario of mocking a Void-returning method might trigger anomalies.
try { Mockito.doThrow(new RuntimeException()).when(mockedList).clear(); } catch (RuntimeException e) { }
This code snippet strives to stimulate exception handling during a mock test. Still, if Mockito stumbles upon Type variable errors, one must examine class implementations, modify generic parameters, or devise alternatives to bypass the stumbling block.
Adjustments involving readjustment of function signatures with well-structured return types replacing Voids can present possible shifts in tackling the message of ‘no instance(s) of type variable(s) T exists so that Void conforms to’.
Overall, gaining extensive knowledge about Mockito behavior combined with a deep understanding of Java generics refines the skills needed to maneuver through intricate testing scenarios. Examining detailed technical documentation such as Mockito Javadoc potentially amplifies your capacity to confront and combat real-time challenging issues pertaining to Mocking, thereby strengthening your software reliability engineering expertise.
“As developers, we are not only programmers; we are designers and architects. We build software that interacts in real life situations. That’s why it is necessary to always keep in mind that our ultimate job is to solve problems, not just write code.” – Sandro Mancuso