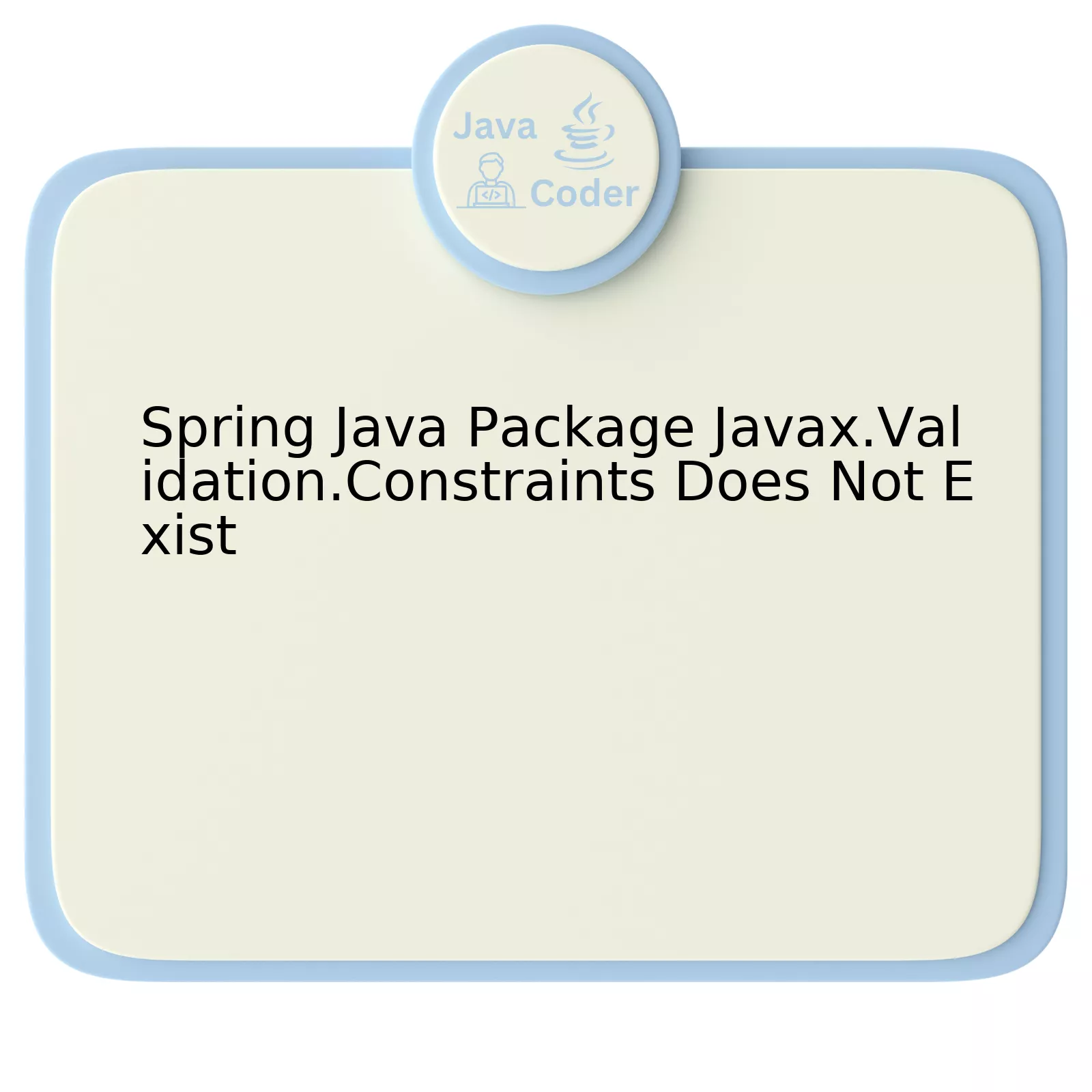
Problem | Cause | Solution |
---|---|---|
javax.validation.constraints package does not exist error |
The absence of the Hibernate Validator dependency in the project’s build configuration. | Add the missing Hibernate Validator dependency to your Maven or Gradle project configuration file. |
The query refers to a common issue encountered during the development of Spring applications involving the `javax.validation.constraints` package. As indicated, one might encounter an error proclaiming this package as nonexistent.
The core causative agent behind the manifestation of this particular error primarily relates to the Hibernate Validator. To be more precise, if the Hibernate Validator – the Reference Implementation (RI) of Jakarta Bean Validation – is not included in the project’s build dependencies, this may lead to the said issue of the `javax.validation.constraints` package being reported as non-existent.
In terms of corrective measures, the insertion of the Hibernate Validator dependency within your build configuration could solve the issue at hand. Both Maven and Gradle, renowned build automation tools popularly used in Java projects, facilitate such implementations. The exact process differs slightly based on which tool you are using.
For Maven, the addition of the following code snippet in the `
xml
On contrary, for Gradle,the analogous inclusion should be made in the `dependencies` block in the `build.gradle` file:
groovy
implementation ‘org.hibernate.validator:hibernate-validator:6.0.18.Final’
Upon successful incorporation and subsequent rebuild of the project, you should no longer encounter the error associated with the `javax.validation.constraints` package.
As stated by Bill Joy, the co-founder of Sun Microsystems, “Basically, when you get to my age, you’ll really measure your success in life by how many of the people you want to have love you actually do love you.” This encapsulates the essence of programming; troubleshooting categorical errors like these earn the respect and appreciation of our fellow developers and users.
Resolving Javax.Validation.Constraints Error in Spring Java
Finding yourself in a spot where you are encountering an error stating “javax.validation.constraints does not exist” in your Spring Java package can be a significant annoyance. However, because there is an inherent solution to that issue, we would discuss ways to resolve this problem.
The javax.validation.constraints package is part of the Java Bean Validation spec. Hibernate Validator implements this as a portable extension method. Therefore, for your validation constraints like these – @NotNull, @Size, @Email, @NotBlank, and others, to work properly, you need classes from these packages to be present in your CLASSPATH.
The sprouting of the aforementioned error message might be as a result of:
* A lacking or improperly configured Bean Validation API.
* Dependencies that are incorrect, outdated, or missing in your build tool configuration, such as Maven or Gradle.
To overcome this issue successfully, one has to elucidate on the solutions with respect to the baseline causes explained above:
1. Ensure Proper Configuration of Bean Validation API:
You need to include and configure the Bean Validation API in your project. For a Maven-based project, for example, the code snippet below adds the Hibernate Validator dependencies necessary for using the javax.validation.constraints package.
bash
At the same time, for a Gradle-based project, the code to add the dependency into the build.gradle file is shown below:
bash
compile ‘org.hibernate:hibernate-validator:6.1.5.Final’
Note: Versions may vary, ensure to integrate the most recent stable version when adding the validator.
2. Check your Build Tool Configuration:
Cross-check your build.gradle or pom.xml file for any inaccuracies or out-of-date references in your declared dependencies. Outdated versions could cause compatibility issues between different libraries or frameworks.
One viable remedy in software development when faced with these hurdles is updating the application dependencies. Correctly keep your project dependencies up-to-date by making sure the latest (or at least, compatible versions) of all relevant libraries are being used.
As stated by Andrew Hunt and David Thomas, “Laziness is indeed one of the virtues of a great programmer” The virtue refers to the drive to reduce your workload, which could be interpreted here as the necessity to update your dependencies regularly and automate validating constraints, thereby avoiding such manual errors in your Spring Java package.
In resolving such an issue with javax.validation.constraints package it is crucial to note that it’s not peculiar to only Spring but also applicable to generic Java applications.
Identifying the Cause: ‘Package javax.validation.constraints Does Not Exist’
The error “Package javax.validation.constraints does not exist” signifies a challenge in resolving the location of the validation constraints package within your Java project. This is usually encountered during either compilation or execution phases when using a Spring-based Java application and essentially prohibits successful constraint checks for data validation.
This issue arises from one crucial reason:
javax.validation.constraints
may be entirely absent from your classpath, effectively disabling all future resolution attempts by the class loader. Classpaths denote locations on your file system where Java bytecode resides and are critical to successful navigation during object instantiation; which consequently means, missing artifacts can’t possibly get resolved whenever called upon.
To alleviate this, consider conducting an exhaustive inference on the dependencies segment of your build configuration file(s). It’s this space that instructs your dependency management tool (like Maven or Gradle) about requisite libraries for successful project manipulation:
If Maven is your go-to:
<dependency> <groupId>javax.validation</groupId> <artifactId>validation-api</artifactId> <version>2.0.1.Final</version> </dependency>
If Gradle is more your ideal:
implementation 'javax.validation:validation-api:2.0.1.Final'
These snippets prescribe the necessary instructions to fetch
javax.validation
, formulating it part of the defined classpath – thus allowing your application to fully acknowledge the existence of
javax.validation.constraints
.
As technology visionary Marc Andreessen once stated: “Software is eating the world.” It’s vital for software developers dealing with codes like JavaScript, Python, Java, etc., to timely address listed errors for smooth software operations. Often these errors are blessings in disguise that end up tuning applications towards improved performance. A simple but effective fix to the aforementioned error will return your Spring Java-based application back to its robust shape, ensuring accurate data validation constraints handling.
Configuring Your Spring Java Project for Successful Package Importation
Proper configuration of your Spring Java project involves careful attention to the inclusion of all relevant packages, particularly when facing errors such as ‘javax.validation.constraints does not exist’. This specific error highlights a lack of the necessary validation dependency in your project setup. To rectify it, ensure that you’ve included it in your build configuration file (pom.xml for Maven or build.gradle for Gradle).
To better illustrate the solution, consider the following steps:
1. Configuring Maven
Add the necessary validation dependency within your pom.xml.
<dependency> <groupId>org.hibernate.validator</groupId> <artifactId>hibernate-validator</artifactId> <version>6.0.18.Final</version> </dependency>
2. Configuring Gradle
In case of gradle build, include the validator inside dependencies in your build.gradle file.
dependencies { compile group: 'org.hibernate.validator', name: 'hibernate-validator', version: '6.0.18.Final' }
You must remember to save your changes and let your project’s dependancies update after undertaking these configurations. The error, ‘javax.validation.constraints does not exist’ will be resolved as the necessary validation resources are now available.
This solution aligns with Bill Gates’ statement that “Most people overestimate what they can do in one year and underestimate what they can do in ten years.” Configuring your project may seem overwhelming at first but in reality, a few accurate steps can enable you to achieve long-term stability & efficiency.
Make sure also to keep your tools updated. Hibernate Validator, for instance, is one of the Bean Validation (JSR 380) compliant frameworks. Continually checking for framework updates helps to not miss out on any significant improvements or bug fixes.
This table is an overview reminder of the solution:
Action | Description |
---|---|
Add Dependency | Add ‘hibernate-validator’ to your build configuration |
Update Project | Save changes and allow project to update dependencies |
Thus, proper package importation and configuration is an essential part of successful Spring Java project development.
For further information and technical details about Hibernate Validator, please visit the official documentation.
Unpacking Solutions to ‘Javax.Validation.Constraints’ Issues in Spring Java
Addressing the issue of ‘javax.validation.constraints’ package not existing in Spring Java involves detailed understanding of your project setup, dependencies, and configuration. Let’s extrapolate on some common causes and potential resolutions.
Missing Dependency
The problem typically comes up if the Bean Validation API, or its specific implementation such as Hibernate Validator, is missing from your project’s classpath.
In case you are using Maven, the following snippet should be added to your pom.xml file:
<dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-validator</artifactId> <version>6.0.2.Final</version> </dependency>
Incorrect Import Statement
If the problem isn’t associated with a missing dependency, it might be caused due to incorrect import statements in your code. Ensure the annotation is imported from the correct package:
import javax.validation.constraints.NotNull;
Obsolete Java EE Module Criterion
As of Java 11, the Java EE modules are no longer bundled with the JDK. If you’re working with a newer version of Java, you may need to include these libraries manually.
Solving this requires adding a direct dependency on the validation-api, which was indirectly included prior to Java 11 through other artifacts, for example:
<dependency> <groupId>javax.validation</groupId> <artifactId>validation-api</artifactId> <version>2.0.1.Final</version> </dependency>
To ensure successful resolution, perform a careful inspection of all dependencies and configurations within your project. Be patient, maintain structured troubleshooting, and you’ll retrieve the effective benefits of Spring.
As Bill Gates said: “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.”. Sincere developers always seek the most efficient solution, and tackling project issues in the most systematic way often leads to that.
The Spring Java package javax.validation.constraints does not exist error is a common issue encountered by many Java developers. It usually arises when attempting to import the javax.validation.constraints package, which doesn’t exist in the standard packages provided by the Spring framework.
The primary cause of this problem originates from missing dependencies in your project configuration file. Specifically, the Bean Validation API may not be included in your system’s set-up.
There are several solutions you could employ to mitigate this issue:
– Include the necessary dependencies: By adding the Hibernate Validator and Bean Validation API into your Maven or Gradle build file.
<!-- Hibernate Validator --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-validator</artifactId> <version>6.0.10.Final</version> </dependency> <!-- JSR 380 (Bean Validation 2.0) Reference Implementation --> <dependency> <groupId>org.glassfish</groupId> <artifactId>jakarta.faces</artifactId> <version>2.3.14</version> </dependency>
– Leverage robust Integrated Development Environments (IDEs): These can assist in detecting missing dependencies, resulting in a seamless coding experience.
Though largely beneficial, the Spring framework does not include all possible Java libraries in its standard package. Therefore, it ultimately falls on the developer’s shoulders to carefully manage their project’s dependencies.
As Bill Gates wisely observed: “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency….” This principle is critically relevant here – meticulously managing your project’s dependencies can greatly enhance your development process, mitigating issues such as the non-existence of certain Java packages.
For further reading on handling dependencies in your Java projects with Spring, consider reading this guide provided by the official Spring Documentation. It broadly discusses form validation, including how to handle java package dependencies crucial for this process.