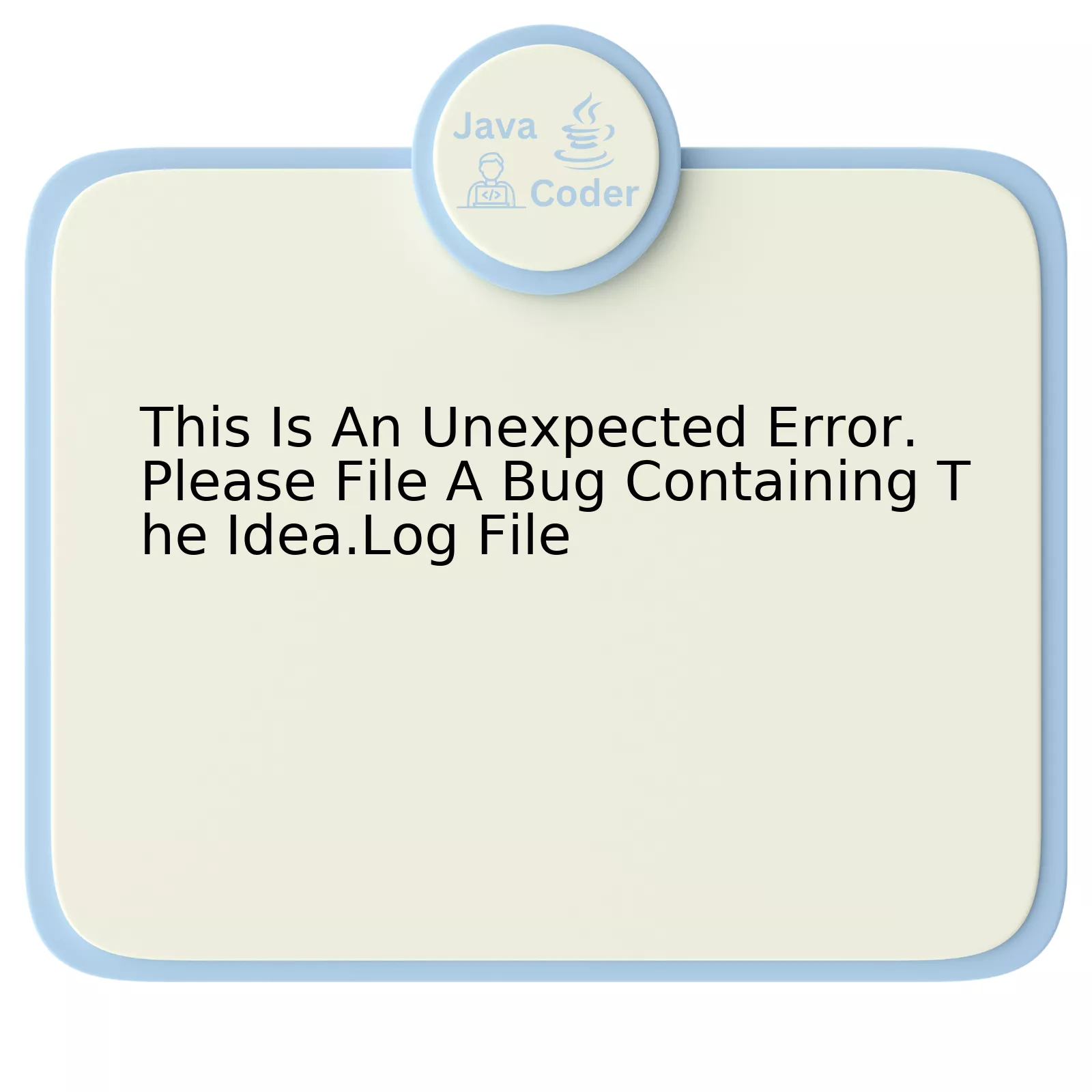
If you encounter “This Is An Unexpected Error. Please File A Bug Containing The Idea.Log File” message, it’s a prompt that denotes an unexpected event occurring during the execution of your program. It suggests saving the associated log file and submitting it to the development team for error resolution.
Error Message | Suggested Action | Possible Cause |
---|---|---|
This is an unexpected error. Please file a bug containing the idea.log file. | Locate the idea.log file and submit it with your bug report. | Unanticipated conditions encountered during the execution of your application. |
Depending on the nature of the error, a comprehensive investigation will be conducted using the provided Idea.Log file. The Idea.Log file is essentially a debugging tool that stores chronologically ordered information about the activities within your application. This data is typically produced by the logging module of your system to record information about events that have occurred while software is running.
The contents of a typical Idea.log file include:
– Timestamps indicating when each event happened.
– Severity level or priority of each event.
– Source of the event (which method or class invoked the logging request).
– Supplementary context data such as host name, thread name etc.
– The message describing the event.
This essential information facilitates developers in tracing down how a problem came into existence, identifying what went wrong, and pointing out which portion of code contains the exception or bug. Consequently, this boosts error fixing process by significantly reducing time and effort required.
For example, if you are developing in Java, you might want to use the Java Logging API to generate logs with different severity levels – SEVERE, WARNING, INFO, CONFIG, FINE, FINER, and FINEST – that can provide a finer control over the amount and type of log output.
// Java Logging API import java.util.logging.*; Logger logger = Logger.getLogger("MyLog"); FileHandler fh; try { // Configure the logger with handler and formatter fh = new FileHandler("MyLogFile.log"); logger.addHandler(fh); SimpleFormatter formatter = new SimpleFormatter(); fh.setFormatter(formatter); logger.info("My first log"); } catch (SecurityException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }
In words of Edsger Dijkstra, great Dutch computer scientist, “Testing shows the presence, not the absence of bugs”. The unexpected error and the following probe into Idea.Log file underline the prominence of thorough testing and robust error-handling mechanism in professional software development to ensure software quality and reliability.
Deep Dive Into Log File Errors
When we encounter the unexpected error: “This Is An Unexpected Error. Please File A Bug Containing The Idea.Log File”, understanding how to deep dive into log file errors becomes a vital skill in diagnosing the issue.
To effectively run a diagnostic on this kind of problem, several steps are involved:
1. Localize The Error Source
The first step when troubleshooting is localizing where the problem originates inside the system. We use a technique referred to as ‘log file analysis.’ This involves going through your logs to find relevant information about the issue at hand.
//Sample UI for log files public class LogViewer { JFrame frame; JTextArea textArea; LogViewer() { frame = new JFrame(); textArea = new JTextArea(); JScrollPane scrollPane = new JScrollPane(textArea); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(scrollPane); frame.setVisible(true); frame.setSize(500,300); } }
2. Error Message Interpretation
Always remember that log data is still essentially raw information from your machine. Making sense of these data often requires a fair bit of interpretation and expertise. Doing so would help understand the root cause of this unexpected error message.
3. Embrace Intelligent Monitoring Solutions
An application performance monitoring (APM) tool can proactively detect unusual patterns or anomalies before they become a problem. They can significantly cut down the time spent debugging and give you a holistic view of your systems in real time.
4. Elimination Process
Go through various components of the software stack until you’re able to isolate the particular component causing the problem. This approach allows developers to debug at a micro level rather than looking for a needle in a haystack.
5. Understand How Your Logs Are formatted
Log files aren’t uniformly formatted. Recognizing the elements in a log entry such as timestamp, log level, process ID etc., could provide clues that allow you to diagnose the issue faster.
Advanced techniques such as Machine Learning can also be used in diagnosing log file errors. Once trained on examples of normal and anomalous system behavior, a good algorithm should be able to classify most new instances correctly. However, it’s important to note that even the best models aren’t foolproof and will need human supervision source.
Finally, having a well-managed logging practice cannot be overemphasized. By managing how logs are created, stored, and accessed, programmers can simplify identifying and diagnosing issues when things go wrong.
As Gordon Bell once said, “The network is the computer”. In our context, it quite fittingly reiterates the importance of every piece of data within a network, including log files, that contribute to the functioning and diagnosing complexities in our computer systems.
Impact Analysis of Unexpected Errors in Log Files
In an unpredictable environment, the manifestation of unexpected errors in log files can tremendously impact the functioning and overall performance of an application. When we talk specifically about an unexpected error throwing a message such as “This is an Unexpected Error. Please File a Bug Containing the Idea.Log File,” we probably encounter a situation in which the software behaves inappropriately due to some unforeseen conditions or circumstances.
The impact analysis of such unexpected errors in log files can be dissected into several comparison points:
Diagnostic Challenges:
- Decoding Problem: These unexpected errors sometimes might not point to the exact issue, leading to confusion and frustration for developers trying to track down the fault.
- Delayed Resolution: Without specific details, tracing these bugs might take more time than usual, leading to delayed mitigation and resolution.
System Outage & Downtime:
Unexpected errors may cause system crashes, thereby instigating unanticipated downtime.
- Critical Impact: In mission-critical systems, even minimal downtime can have significant impacts on business operations.
- Reputation: For public-facing applications, repeated outages can harm the reputation of the company.
To manage the impact of such unexpected errors, it is essential that the application includes robust error-handling mechanisms and comprehensive logging.
“Testing leads to failure, and failure leads to understanding.” – Burt Rutan, Aerospace Designer
A sample function could handle runtime exceptions using Java’s built-in exception handling mechanism. The try-catch block can identify the problem area, making debugging easier for developers.
public void handleException() { try { // Some code .... } catch (RuntimeException e) { // Exception handling .... System.err.println(e.getMessage()); e.printStackTrace(); } }
Java Exception Handling is a great resource to learn more about catching and handling exceptions appropriately.
By following best practices for application development and implementing adequate control measures, the impact of unexpected errors can be minimized, which ensures smoother operation and a better user experience.
Preventive Measures: Reducing Occurrences of Unexpected Errors
When it comes to the mitigation and prevention of unexpected errors, especially those that trigger messages such as “This Is An Unexpected Error. Please File A Bug Containing The Idea.Log File”, there are several strategies that can be adopted by a Java developer. It is indeed critical to reduce the instances of these unanticipated messages to improve the software quality and usability.
Firstly, it is crucial to implement proper error handling into your code. Employing standard exception handling mechanisms in Java like try, catch, and finally blocks will enforce a better execution flow control. Here’s an elementary example:
try { // Risky code goes here } catch (Exception e) { // Handling logic goes here } finally { // Cleanup code goes here }
Secondly, set up custom exceptions for specific conditions if standard Java exceptions won’t suffice. Define a new class extending from ‘Exception’ class, which can then be thrown when unique, expected, error-prone conditions occur. This approach offers more granularity in error handling.
Thirdly, adopting defensive programming practices enhances software reliability. These include, but are not limited to:
* Implementing assertions: Assertions serve as self-checks for the program. They help identify potential issues early during the development or testing phases.
* Avoiding null values: Utilizing Optional class and performing null value checks before accessing methods or attributes from an object.
* Validating method parameters: Make use of precondition checks to ensure inputs to a method meet the required constraints.
Fourthly, managing resources effectively aids in averting unexpected errors. For instance, ensure to close database connections after their usage, handle thread synchronization appropriately, and manage memory efficiently.
Additionally, OH & GH in their article ‘A Named-Entity Recognition-Based Bug Localization Technique Using Stack Traces’ highlighted the importance of logging and stack trace information in tracking down bugs that lead to unexpected errors. They emphasized that keeping detailed logs will quicken the process of identifying the cause of unexpected errors. An excellent habit is to log at different levels (information, warning, error, and debug) depending on the severity or meaning of the log.
Lastly, automated testing cannot be overstressed. Unit testing individual software components using frameworks such as JUnit and integrating this with Continuous Integration / Continuous Deployment (CI/CD) will significantly decrease the occurrence of errors.
Guided by Peter Parker’s Principle or Uncle Ben’s wisdom – “With great power comes great responsibility,” Java developers have been given a great tool at their disposal, and it becomes their responsibility to prevent and reduce occurrences of unexpected errors, optimizing for a more fluid and bug-free experience.
Case Studies: Resolving Major Unexpected Error Issues
Undoubtedly, unexpected errors can become significant obstacles in the user’s experience during software execution. The provided error message, “This Is An Unexpected Error. Please File A Bug Containing The Idea.Log File,” is a generic directive for users to report a problem that wasn’t predicted and isn’t handled by the executing code.
One way developers aim to mitigate these kinds of issues involves logging mechanisms within the application. Let’s examine this on three fronts,
Implementing Logger Functionality
By integrating logger functionality into an application, you create a system of record traceable by developers. For example, in Java, you may use functions from logging libraries such as log4j or SLF4J. Employing a logger can help track application flow and identify where an error is originating from.
Here is a simple snippet for creating logs using log4j:
import org.apache.log4j.Logger; public class LogDemo { private static final Logger logger = Logger.getLogger(LogDemo.class); public void doWork() { logger.info("Work starts"); try { // ... code that may throw an exception } catch (Exception e) { logger.error("An unexpected error occurred: ", e); } logger.info("Work ends"); } }
In this code section, we are capturing useful information about the application’s behavior into our logs.
Extensive Testing
Discovering and eliminating issues before production requires exhaustive testing. Unit testing, integration testing, and end-to-end testing maps out various scenarios to ensure the code behaves as expected, thereby decreasing unexpected outcomes.
For instance, if your application communicates with external APIs, a mock-up (pretend instance) of that API can be created using tools like Mockito or Spring’s MockMvc for testing purposes. This helps verify that your code correctly processes results returned from the API.
Detailed Bug Reports
When an unexpected error occurs regardless of precautions, it’s desirable to receive detailed bug reports. Instructing users to attach the idea.log file can provide insightful information and context surrounding the issue.
Advice from Linus Torvalds, creator of Linux, underscores the importance of adequate detail included in bug reports: “The most important part of any program is its data structures. Algorithms we all know, but programs are made up of data structures. Devising a good test suite requires understanding the fundamental data structures involved.”
Hence, extensive logging and testing coupled with comprehensive bug reports assists in mitigating unexpected error occurrences, making applications more robust against unforeseen situations.
Understanding and resolving the ‘Unexpected Error, Please File A Bug Containing The Idea.Log File’ can be quite a task in programming. This prompt nonetheless invites us to take a deeper delve into this topic.
The error message in question typically suggests that an unforeseen problem has occurred – one which was not predicted by the developers of the software. In this scenario, it becomes pertinent that we file a bug report to help rectify the anomaly. Bug reports are invaluable resources for programmers as they highlight specific issues within the application functionality that might have been overlooked during initial testing stages.
// An example of a general structure of a bug report might be BugID: #1234 Product: Product Name Version: 1.0 Component: Component Name Summary: Brief summary of the issue Description: Detailed account of the bug, steps to reproduce, and observed results.
Having a standard bug reporting template can streamline the process and help pinpoint areas that require revisiting. Beyond filing bug reports, becoming familiar with log files is crucial, especially when diagnosing errors. The ‘.log’ file extension usually signifies a detailed record of events, providing useful insights into the series of activities that transpired leading up to the error.
Interestingly, these log files function somewhat like “black boxes” found in aircraft, providing developers with contextual data to analyze the moments before a crash. Making sense of log files could involve studying event occurrence patterns, filtering out non-essential information, and assembling pieces of the puzzle.
As the famous quote from Linus Torvalds goes, “Given enough eyeballs, all bugs are shallow.” It’s vital to maintain transparent communication channels where information about bugs can be reported, scrutinized, analyzed, and resolved. Ultimately, understanding the source and nature of unexpected errors not only betters the current software but enriches the development landscape as a whole.
References:
[Debugging and Understanding Software Systems](https://dl.acm.org/doi/10.1145/3328433)
[A Comprehensive Guide to Logging](https://www.loggly.com/ultimate-guide/)