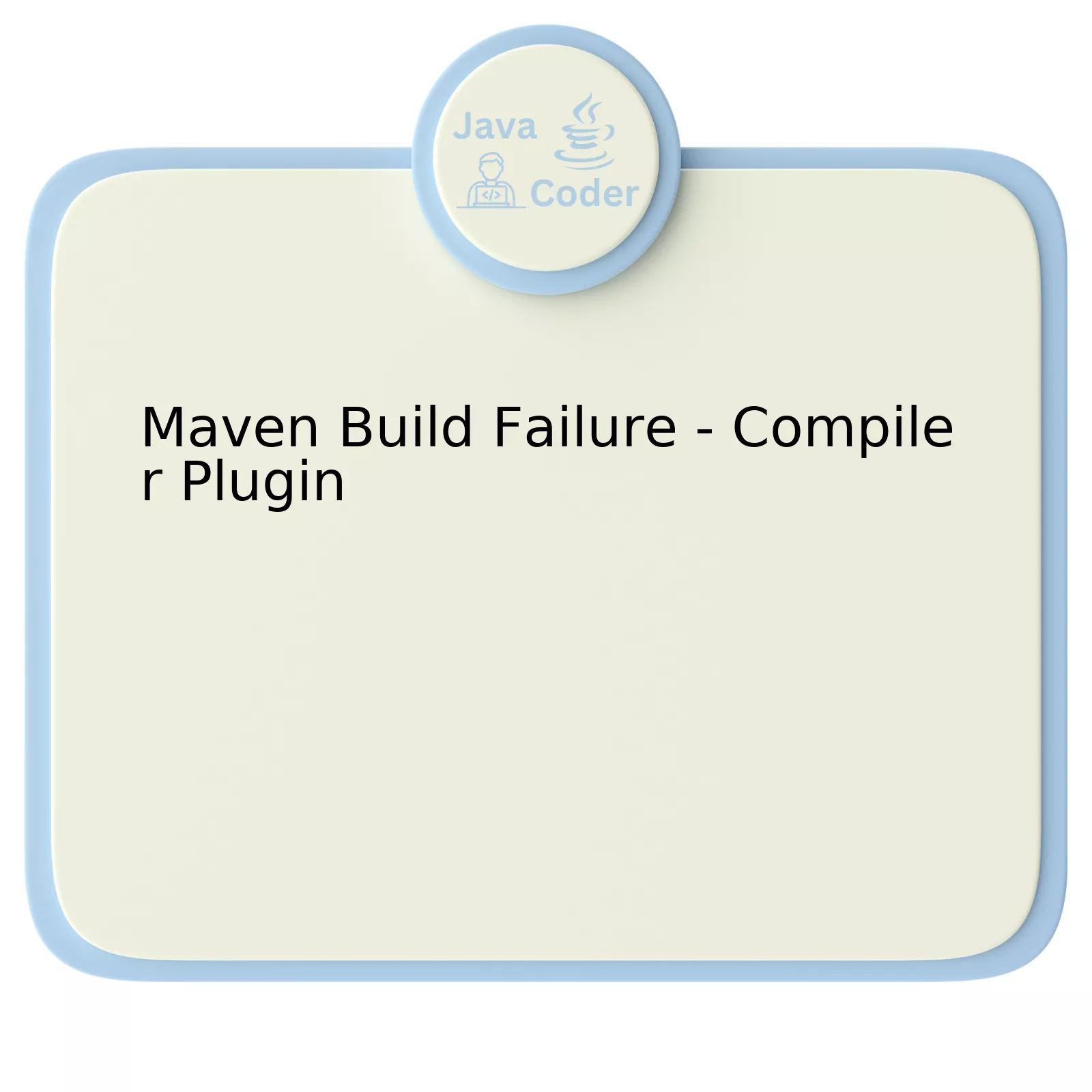
The issue of Maven Build Failure primarily happens due to issues with the compiler plugin. A detailed explanation of this issue and a potential solution can be found here.
Issue | Description | Possible Solution |
---|---|---|
Compiler Plugin Error | This is usually an error thrown when Maven cannot find the Compiler Plugin used to compile your code. | You may need to specify the version of the Compiler Plugin in your POM file or ensure that it’s correctly installed and configured on your system. |
The core problem lies in the ‘Compiler Plugin Error’. Essentially, this error arises when Maven is unable to discover the Compiler Plugin that’s required for compiling your source code. This could happen due to a number of reasons including but not limited to missing dependencies, incorrect version specified, or an improperly configured environment. As you might know, Maven utilizes the Compiler Plugin to transform the source code into bytecode which the JVM (Java Virtual Machine) can execute. If Maven can’t find or use this plugin, it won’t be able to build your application leading to a build failure.
So, how do we resolve such an issue? Oftentimes, by specifying the version of the Compiler Plugin directly in the Project Object Model (POM) file associated with your project, you can help Maven in locating the appropriate compiler version. Here’s an example:
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> </plugin> </plugins> </build>
Alternatively, you may decide to adjust the configuration settings on your local system to ensure the accurate location of Compiler Plugin by Maven. For instance, you might want to tweak the ‘M2_HOME’ surroundings’ variable or modify your Maven settings.xml file.
To echo the words of Alan Kay, “People who are really serious about software should make their own hardware,” , the essence of this solution resides in getting down to the details of the build environment, ensuring the right plugins are specified, and all elements are well-configured to ensure a successful build. The commitment to properly setting up and managing software environments greatly aids in mitigating build issues in general.
Understanding the Causes of Maven Build Failure
Understanding the causes of Maven Build Failure specifically related to Compiler Plugin provides an informative exploration into why this essential development tool might not perform as expected. Maven, a popular project management tool, often encounters issues with its Compiler Plugin because:
Reason | Description |
---|---|
Poor Project Configuration | Maven uses XML files for configuration. If these are incorrectly set up, problems with the Compiler Plugin and overall build failure may ensue. |
Incompatible Java JDK Version | Often, projects require specific JDK versions. If Maven is set to an incompatible version, it can lead to build failure with error messages indicating issues with the Compiler Plugin. |
Dependency Conflicts | Maven’s dependency management can sometimes throw up unexpected conflicts causing the Compiler Plugin to fail when trying to compile. |
Misconfiguration of Compiler Plugin | The default setting of the Compiler Plugin may not be compatible with the current setup, leading to error messages and build failures. |
An understanding of Java Programming Language, Maven’s project configuration, and plugin management is imperative to resolve these issues. For example, if the wrong JDK version is used, it can be corrected using:
<plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> <configuration> <source>[Your JDK]</source> <target>[Your JDK]</target> </configuration> </plugin> <plugins>
Acknowledging Bill Gates’ famous quote, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it,” it isn’t about cutting the corners, but viewing the situation from a clever perspective. As a developer, resolving Maven build failures involves identifying possible break points, using the right tools, and applying elegant solutions to maintain streamlined operations. (Check out more from Apache Official Documentation)
Troubleshooting Steps for Compiler Plugin Errors in Maven
The Maven Compiler Plugin can cause build failure if not correctly configured or due to other external factors. Following are some steps you can take to troubleshoot and sort out these issues:
1. Verify the Java Version
The first step in troubleshooting starts with verifying your JAVA_HOME environment and the JDK version that is being used. The
javac
version should align with the
maven.compiler.source
and
maven.compiler.target
values from the project’s pom.xml.
Here’s how you can check your java version:
java -version
2. Inspect Project Dependencies
Inspect all the dependencies of your project to ensure they don’t conflict. If there are conflicting dependencies, you would need to exclude those within your POM configuration.
3. Check For Compilation Errors In Code
Sometimes it could be simple syntax errors or missing imports in your Java code that may cause compiling incorrectness, leading to build failure. Using a smart IDE like Eclipse or IntelliJ Idea can help find these errors.
4. Review POM.xml Configuration for Compiler
Check the POM.xml file for any errors or misconfigurations regarding the compiler plugin.
Ensure you’re specifying a valid version of the maven-compiler-plugin and configuring the source and target properties properly:
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
5. Update or Reinstall Maven
In rare cases, an outdated Maven version or corrupt Maven installation might create problems. You can try updating Maven to the latest stable version or reinstalling it.
If none of these strategies solve your issue and the error persists, consider reaching out to community support formats such as Stack Overflow or Maven official mailing lists. You might also find it helpful perusing through Ben Evans book “ Maven: The Complete Reference“. It provides extensive guidance on Maven’s features, plugins, and best practices.
“Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” – Brian Kernighan. Not all problems are solved conventionally; sometimes, they need you to think beyond. So while debugging, keep your mind open for all possibilities.
Effective Solutions to Overcome Maven Compilation Issues
Maven is a project management and comprehension tool used primarily for Java projects to build, report, and document from a central piece of information. One of the challenges developers may encounter while using Maven includes compilation issues. However, there are a few solutions that will help circumnavigate such problems effectively.
When faced with a `Maven Build failure – Compiler Plugin`, it can be frustrating as these could hamper the usual flow of project development. This issue signifies that the build tool failed to compile the project for some reasons — the compilers might not have been set correctly or sometimes, version incompatibility between tools you’re using.
Possible Solution 1: Recompile sources:
A primary approach to resolving this error would be forcing maven to recompile all the sources.
-Dmaven.clean.skip=false
tag can be added as part of running the command within the terminal. This forces a clean build, allowing for re-compilation.
bash
mvn clean install -Dmaven.clean.skip=false
Possible Solution 2: Set JDK Properly:
Oftentimes, improper JDK settings or using different versions of JDK for different programs lead to compiler plugin errors. Check that the JDK configured in IntelliJ or Eclipse matches the JAVA_HOME variable in the System Variables (Windows) or Environment Variables(Nix). Update the setup accordingly if any discrepancies exist.
Possible Solution 3: Update Maven Compiler Plugin version:
In other cases, due to version incompatibility, the solution deals with updating your Maven Compiler Plugin to your specific version of the JDK. Add or modify the configuration tag in the Maven POM.xml file:
xml
Here, `
“The focus should be on overcoming obstacles rather than being intimidated by them.” Brian Tracy, a renowned motivational speaker, gives us a pertinent analogy on how to manage such technical setbacks.
Additional References:
Compiling Maven Projects offcial guide can be found at Apache’s website here.
For further insights on handling maven issues, refer Stackoverflow’s forum discussion. Though forums might contain varying strategies to handle similar issues, they can provide diversified perspectives and sometimes, a more suitable solution based on contexts.
Best Practices to Prevent Future Maven Build Failures
The Maven Build Failure can often arise from issues related to the Compiler Plugin. Here are some best practices to circumvent future build failures that arise due to the same:
Ensure Correct and Updated Maven Compiler Plugin Version
Always ensure to use an appropriate version of the Maven compiler plugin in your project’s
pom.xml
file. Using incompatible or outdated versions might lead to unforeseen build errors. The Maven community frequently rolls out updates, enhancements and bug fixes, so keeping the plugin updated helps mitigate certain problems.
Example:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> </plugin>
Maintain Consistent Java Version Across Environment
The Maven Compiler Plugin uses a particular version of Java as set within the environment. Misalignment in Java versions between development, staging, and production environments can also cause build failures. Ensure that the Java version is consistent across all environments.
If you need to define a specific java version, you can do it like this:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.8</source> <target>1.8</target> </configuration> </plugin>
Leverage Maven’s Dependency Management
Proper management of dependencies can help avoid several issues including build failures. Declaring all dependencies allows Maven to handle potential conflicts and ensures that no unwanted versions sneak into the build.
Regularly Clean and Update the Local Repository
Corrupted or outdated artifacts residing in local repositories may stir up build failures. Perform frequent cleaning (
mvn clean
) and updating (
mvn clean install -U
) operations in the local repository.
In Perforce’s blog on good code, they mention, “Good code can be understood and extended by others.” These best practices aim at not just preventing build failures but also ensuring effective collaboration through quality code.
Adhering to these best practices will reduce the occurrences of build failures and contribute towards smooth build lifecycle with Maven Compiler Plugin.
The intricacies of Maven Build Failure, specifically due to the Compiler Plugin, can be thoroughly traced back to a variety of underlying causes. This form of build failure is typically marked by an error message such as “BUILD FAILURE – compilation error,” which casts light on the development-related issue necessitating immediate attention.
[ERROR] Failed to execute goal org.apache.maven.plugins:maven-compiler-plugin:3.1:compile (default-compile) on project my-project: Compilation failure: Compilation failure:
To begin with, one plausible reason behind this situation can be incorrect Java version in your system versus what is needed by the project. It’s important to ensure you’re operating on the right version that resonates with your Maven Compiler Plugin.
Furthermore, discrepancies in PATH variables might also cause Maven Build failures. These variables should rightly point towards the home directory of the Java Development Kit (JDK), instead of directing to the Java Runtime Environment (JRE) home. One way to troubleshoot this glitch involves checking the JAVA_HOME variable and adjusting it if necessary.
echo %JAVA_HOME%
This command should help verify the correct JDK path.
Another potential hindrance arises when your project references dependencies that Maven cannot locate. In such cases, the error usually refers to missing artifacts or Maven not being able to download them due to network issues or misconfigured settings.
Dependencies could be properly monitored for verification purposes:
mvn dependency:tree
By using this command, a dependency graph for your project will appear where any discrepancies can be effectively noted.
While exploring specific resolutions to Maven Build Failure on account of the Compiler Plugin, it’s often constructive to draw insights from experts in the field. Martin Fowler, a renowned software developer, emphasizes the validity of this approach, stating, “If you can’t make a mistake, you can’t make anything.”
Hence, Maven Build Failure related to Compiler Plugin is a common hurdle that developers face and get past. The steps outlined above provide a comprehensive guide to both understanding the problem and potential solutions. Revisiting these pointers will equip programmers to continue delivering high-quality code.