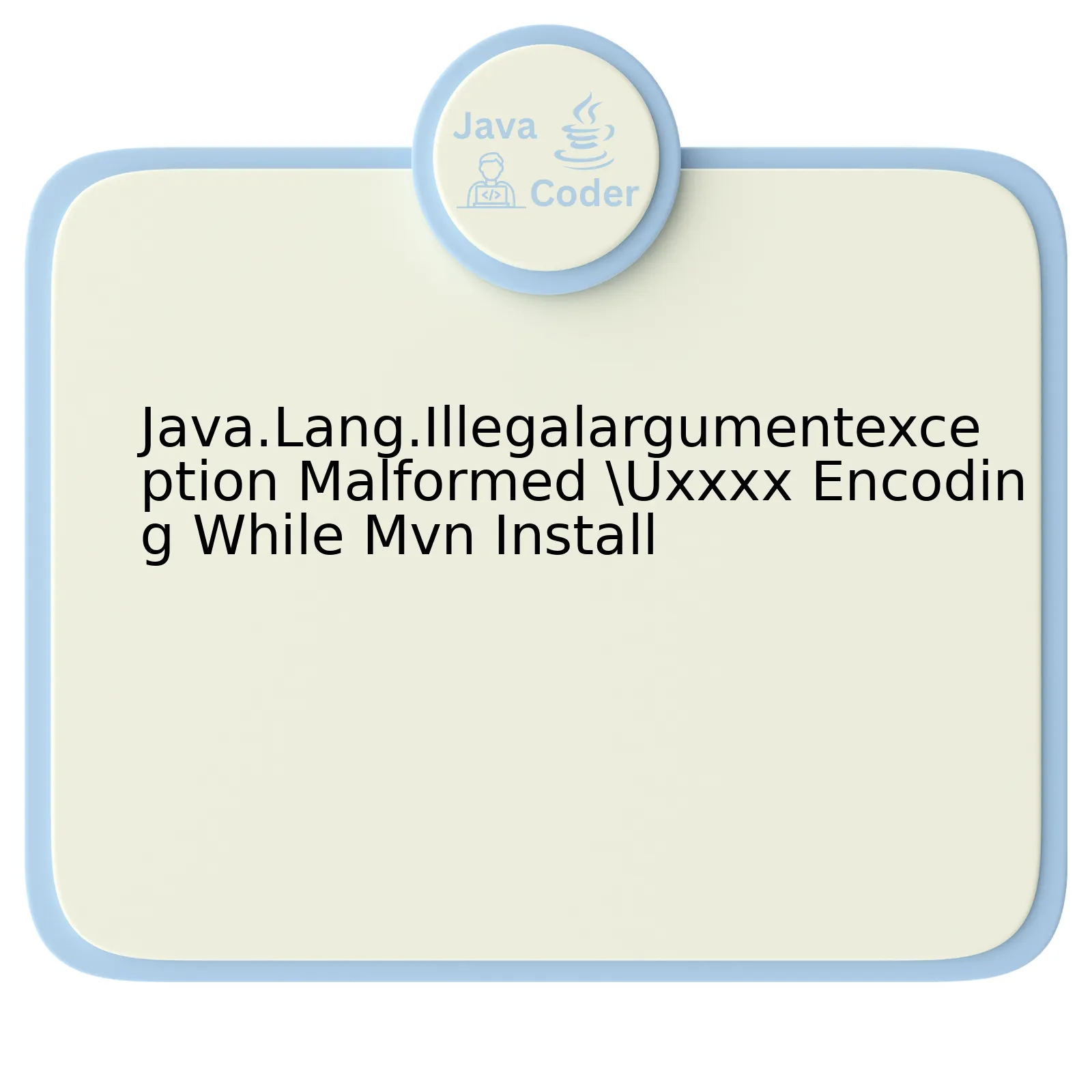
The Java.Lang.Illegalargumentexception Malformed \Uxxxx Encoding error typically occurs when Maven is interpreting a universal character name incorrectly, often due to an errant Unicode escape sequence in your code. The issue arises as Unicode sequences in Java are denoted by the marker ‘\u’ followed by a four-digit hexadecimal number.
Error Type | Causes | Solution |
---|---|---|
Java.Lang.Illegalargumentexception Malformed \Uxxxx Encoding |
|
|
To clarify the solution utilizing coding examples:
When defining your String variables in Java, ensure you’re using proper Unicode format. Improper example:
String myString = "\uXYZA"; // This will throw the IllegalArgumentException.
Corrected example:
String myString = "\u0123"; // This string is properly encoded.
As advocator of programming and best practices in our field, Robert Martin (Uncle Bob) stated: “A long descriptive name is better than a short enigmatic one. A long descriptive name is better than a long descriptive comment.” The same practice applies to handling codes and formats. Every character or line of code should follow the standards and guidelines set forth in documentation, ensuring smooth performance and avoiding exceptions like the Java.Lang.Illegalargumentexception Malformed \Uxxxx Encoding.
Remember, always verify your environment settings on both client and server end, as this often contributes to such issues arising during mvn install. A robust validation of server-side settings that handle Unicode can eliminate this exception from being triggered.
Understanding the Java.Lang.Illegalargumentexception Error in Maven Install
The Java.Lang.Illegalargumentexception error encountered during a Maven install typically arises when there’s an incorrect formatting or invalid argument in your code. More specifically, the “malformed \uxxxx encoding” error is mainly associated with issues related to the format of Unicode characters.
Deep Dive into the Issue
To properly comprehend the issue, it’s necessary to understand what “\uxxxx” implies. In Java, Unicode characters can be represented using the escape sequence “\uxxxx”, where “xxxx” stands for the hexadecimal value of the character. Hence, the error “malformed \uxxxx encoding” signals that the unicode representation is malformed or not well-structured.
// correct char ch1 = '\u0061'; // represents 'a' // incorrect char ch2 = '\u006Z'; // 'Z' is not a valid hex value thus would cause malformed \uxxxx encoding error
This error is a common one because unfortunately compilers sometimes fail to detect this during the initial stages of development. Then, the issue surfaces during later stages such as during a Maven install causing headache for developers.
Possible Solutions
Below are several ways to resolve such errors:
* Carefully examine your code and look out for any non-standard Unicode representations. Ensure each Unicode character adheres to the standard format.
* It’s worth mentioning that some text editors automatically convert special characters into their respective Unicode representation while saving the file (especially if the file has UTF-8 encoding). To tackle this issue, consider changing the file’s encoding or opt for different text editors that don’t auto-convert these characters.
* Another solution could be to use native2ascii, a tool provided by JDK which converts files with non-latin characters into files with Unicode encoded ASCII characters.
native2ascii input.java output.java
These solutions help prevent complications during the Maven install process and eliminate misleading Java exceptions. A note of advice from Robert C. Martin, software engineer and author – “A good error message first tells you the symptom of the problem, then the location, and finally the nature of the problem.” This insight rings particularly true with regards to deciphering the Java.Lang.Illegalargumentexception error.
To learn more about the compiler used by Apache Maven, the official resources found here can be insightful.
Resolving Malformed \Uxxxx Encoding Issues During Mvn Installation
The issue “Illegalargumentexception Malformed \Uxxxx Encoding” during Maven (Mvn) installation can occur primarily due to unicode conversion errors. Maven interprets characters that follow the backslash u (\u) as part of a unicode escape sequence. If it encounters an invalid sequence, it throws this error.
To troubleshoot and resolve this issue,
Step 1: Review your pom.xml file:
It’s important to review your pom.xml file or other Maven configuration files for the presence of any unicode escape sequences. Here’s an XML snippet that may cause such an error:
<configuration> <archiveName>some\u0020name</archiveName> </configuration>
In this case, ‘\u0020’ is a Unicode escape sequence corresponding to a space character.
Step 2: Proper Formatting:
Ensure your unicode escape sequences are correctly formatted with precisely four hexadecimal characters following the ‘u’. For instance, “\u0020” is correct, but “\u20” or “\u002” would be malformed.
Step 3: Preserving Unicode Characters:
If you want to use a literal ‘\u’ without triggering the start of a Unicode escape sequence, use a double backslash before the ‘u’: ‘\\u’.
Step 4: Environment Variables:
Checking, correcting, or adjusting environment variables related to Java or Maven may resolve certain issues.
Step 5: Update Maven:
Updating your version of Maven may also help, as some earlier versions were more prone to these encoding errors. The newest versions offer better ways to handle such scenarios and often come with bug fixes.
As a relevant quote from the respected co-author of “Continuous Delivery”, Dave Farley, reminds us, “Debugging is like being the detective in a crime movie where you are also the murderer.” Therefore, attention to detail, especially when setting up complex builds, is vital.
Nonetheless, the above steps should effectively help you resolve the “Java.Lang.Illegalargumentexception: Malformed \Uxxxx Encoding” issue during Mvn Install.
Using Special Characters While Dealing with Java and Maven Install
While coding in Java and utilizing Maven for project management, it is common to encounter exceptional cases like `java.lang.IllegalArgumentException: Malformed \uxxxx encoding while mvn install`. This situation arises primarily due to usage of special characters in the path variables or properties defined in the project.
The `\uxxxx` error typically indicates that somewhere within your code, or within a resource being used by your build, there’s a Unicode sequence that hasn’t been correctly formed. These sequences should represent valid Unicode codepoints with ‘xxxx’ being replaced by 4 hexadecimal digits.
To rectify this issue, you need to be aware of a few critical pointers:
* Use escape character correctly: Avoid using backslashes (\) as direct file separators, or include the escape character correctly. For example, opt for `C:\\path\\to\\file` instead of `C:\path\to\file`. Alternatively, you can use forward slashes (/).
// Incorrect String path = "C:\path\to\file"; // Correct - Using double backslash (\\) String path = "C:\\path\\to\\file"; // Correct - Using forward slash (/) String path = "C:/path/to/file";
* Inspect Property Files: Check .properties files carefully. In these files, the equal sign (=), colon (:), space ( ) and # sign require an escape sequence.
* Examine XML files: XML files parsed by Apache Maven are susceptible to having incorrectly escaped characters. Sometimes, symbols such as less than (<), greater than (>), ampersand (&), single quote (‘) and double quotes (“) can cause issues if they aren’t properly encapsulated in CDATA tags or not properly replaced with their corresponding entity names or numbers.
// Incorrect <element>This is "sample" & 'demo' text</element> // Correct - Using Character Entities <element>This is "sample" & 'demo' text</element> // Correct - Using CDATA Sections <element>
I’d like to share a quote by Jeff Atwood, a renowned software developer and author, who once wrote, “We have to stop optimizing for programmers and start optimizing for users”. This principle applies here as well, indicating that efforts must be made to ensure sustainability and simplicity of codes, thereby refraining from unnecessary complications caused due to improper use of special characters.
References:
– Dealing with Special Characters in Java
– Maven and Java – Apache Maven Project
Inside the Mechanisms of java.lang.IllegalArgumentException during Mvn Install
The `java.lang.IllegalArgumentException` during Mvn Install refers to an application program interface (API) that throws an exception when a method receives an argument it wasn’t expecting or can’t process. This specific error message “Malformed \uxxxx encoding” usually arises due to incorrect representation of Unicode characters in the Java programming language.
First and foremost, you need to understand `
Unicode
`. This is a universal character encoding standard that represents almost all of the writing systems in the world. The notation `\uxxxx` is used for this type of character sequence where xxxx denotes four hexadecimal digits.
If you try to execute Mvn Install and meet with this `IllegalArgumentException`, it suggests one or more Unicode sequences in your project are incorrectly formatted or invalid. One common pitfall area in running Mvn Install involves properties files localization—they use `\u` to escape other characters such as newline (`\n`) or tab(\t). Consequently, JVM interprets them as Unicode characters hence causing an issue.
A remedy situation is to double-check the properties files or any resource bound input files. We see in the following snippet if the content isn’t format precisely as expect, e.g., 4 hex numbers (0-9, A-F), Java will throw this `IllegalArgumentException`.
Properties prop = new Properties(); InputStream input = new FileInputStream("config.properties"); prop.load(input); System.out.println(prop.getProperty("data"));
Alternatively, confirmation of file encoding could solve the problem. Should they be encoded in UTF-8 without a Byte Order Mark (BOM), convert them accordingly, ensuring a match in your project build settings.
Understanding these technicalities forms a crucial part of our work as developers. As software craftsman Robert C. Martin rightly puts it, “The programmer, like the poet, works only slightly removed from pure thought-stuff. He builds his castles in the air, from air, creating by the exertion of imagination.”
In online resources such as StackOverflowhere, you can find extended discussions on methods to resolve this issue. Remember, it’s about maintaining correct code syntax and ensuring compatibility with various coding standards.
The
java.lang.IllegalArgumentException: Malformed \\uxxxx encoding
error during Maven installation is a result of incorrect unicode conversion. The \uxxxx notation is for expressing unicode in java properties file. However, if the implementation or syntax of that escape sequence is off by even a small degree, it can result in a malformed \uxxxx encoding exception.
Consider this snippet:
Properties prop = new Properties(); FileInputStream fis = new FileInputStream("test.properties"); prop.load(fis);
If the test.properties file contains any unicode characters that do not meet the \uxxxx format, such as \u000g (where g is not a valid hexadecimal number), the java compiler throws
java.lang.IllegalArgumentException: Malformed \\uxxxx encoding.
To address the issue, below are some solutions:
- Validate input : Make use of regular expressions to validate the unicode escape sequence prior to storage in the properties file. Once the unicode string passes the validation, it can be safely stored in the properties file.
- Unicode converter tool : There are tools available online that allow for proper unicode conversion. These instruments ensure that all unicode entered into the property files are in correct \uxxxx format.
As Jeff Atwood, co-founder of StackOverflow, once said, “We have to stop optimizing for programmers and start optimizing for users.” To apply his quote here, instead of doing manual verifications, utilizing an automated tool helps to minimize errors, increasing productivity and software quality.
Though resolving
java.lang.IllegalArgumentException: Malformed \\uxxxx encoding
may seem complex, be ensured that with the correct approach it could be easily fixed. It’s just a matter of analyzing the problem correctly and applying the required solution step-by-step. Consequently, producing a more reliable and efficient code base and execution process.
For a deeper understanding of the topic, you might find this StackOverflow discussion helpful.