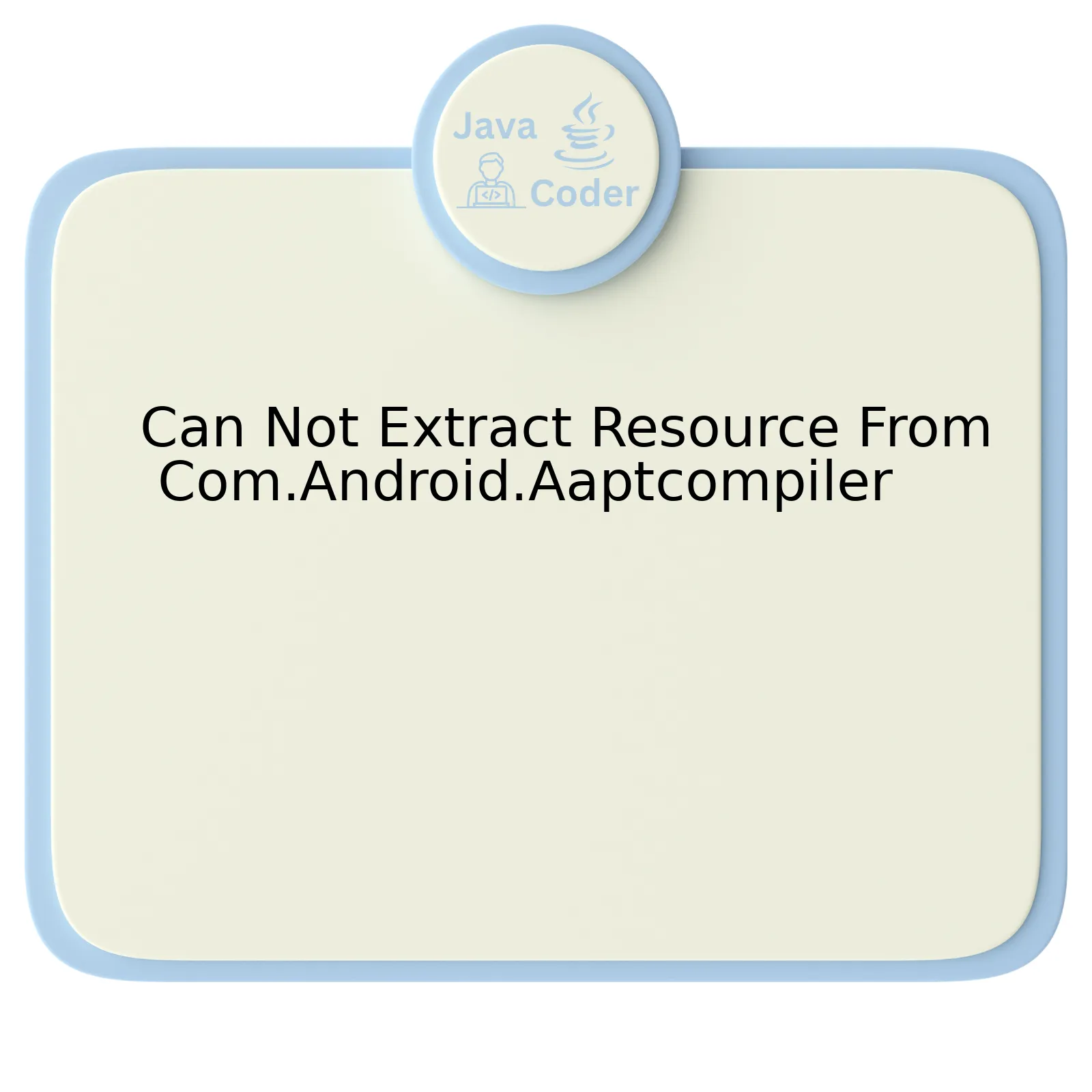
The issue of not being able to extract resources from Com.Android.Aaptcompiler is encountered frequently by the Java developers working on Android applications. The table below displays a high-level overview of this problem:
Error | Cause | Solution |
---|---|---|
Can Not Extract Resource From Com.Android.Aaptcompiler | Error in parsing XML file, Misplacement or missing resource file, Incorrect naming convention, Bug in AAPT tool | Checking XML files for errors, Ensuring that all resource files are in place and named according to Android’s specifications, Updating or changing AAPT tool |
Analyzed deeply, the “Cannot Extract Resource from Com.Android.Aaptcompiler” problem emerges as a multi-causal issue.
To begin with, parsing errors in the XML resource files may lead to this error. Parsing involves translating an XML document into an internal format, which is used by later processing stages within the Android Asset Packaging Tool (AAPT). If there are any structural issues in the XML files, it will result in a failed extraction. Therefore, ensuring that your XML files adhere strictly to the prescribed formatting rules is the first step in troubleshooting this issue.
Next, the issue could also arise out of misplaced or missing resource files. Android projects have a predefined directory structure where each type of resource file has a designated location. For instance, image files should reside under the drawable folder while layout XML files belong to the layout directory. In case these resources have been inadvertently placed in incorrect directories, it can lead to probe failure when Aaptcompiler attempts to extract them.
Subsequently, it is important to note that Android uses a specific naming convention for its resource files. Any deviations from this can make it difficult for Aaptcompiler to locate and extract these resources. So, ensure that all resource files are following Android’s name conventions i.e., lowercase letters, digits, underscores only and must start with a letter.
Lastly, the fault might be in the Android Asset Packaging Tool (AAPT) itself, especially if you are using an older version of AAPT. A bug in the AAPT tool can lead to this error as well. Upgrading to the latest version or changing the AAPT tool might help resolve this issue.
In the words of Linus Torvalds, the creator of Linux, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” Indeed, addressing complex errors like this not only bolistically refine programming skills, but also makes the journey more rewarding and fun!
Understanding the Com.Android.Aaptcompiler Error
The com.android.aaptcompiler error is one that you may encounter during Android development process. Typically, this message pops up when the Android Asset Packaging Tool (AAPT) fails to compile your app resources. While it can be caused by several different issues, it is often related to missing essential files or problems with the syntax of your XML.
When specifically discussing about ‘Cannot extract resource from com.android.aaptcompiler’, this error generally points towards issues in extracting resources from assets or resource folders. It might be triggered due to particular issues such as improper naming conventions and improper placement of these resources.
Understanding the intricacies of com.android.aaptcompiler is easier with the following key takeaways:
XML Syntax
Android heavily utilizes XML layouts, which should strictly adhere to proper XML syntax. A minor oversight or typo can trigger com.android.aaptcompiler error.
Here’s an example of incorrect syntax:
<TextView android:id="@+id/text_view" android:layout_width="wrap_content"> </TextView>
In the above snippet, there’s a missing attribute, i.e., android:layout_height that requires rectification.
Resource Naming Conventions
All resource files should be named using lowercase letters with words separated by underscores. Avoid using digits at the start of file names. Not adhering to these naming conventions could prevent the AAPT from recognizing these resources, leading to the error message.
Placement of Resources
Resources, such as images, must be placed in the correct folder, like drawable or mipmap. Placement discrepancies might lead to resource extraction errors.
Lastly, according to Linus Torvalds “Talking is cheap. Show me the code”, always ensure you debug your code effectively and use tools available to cross-check your XML syntax and guidelines.
Always remember that each error teaches us something new. With these key understandings, recognizing and rectifying issues around ‘Can not extract resource from com.android.aaptcompiler’ will gradually turn into a smooth sail for you. Always aim to comprehend the root issue behind each error message, instead of merely seeking solutions. When encountering a com.android.aaptcompiler error, visualize what the AAPT does, how it interacts with your project, and the possible reasons it cannot proceed as expected.
Effective Strategies to Troubleshoot Resource Extraction Issues
Troubleshooting resource extraction issues, particularly those that arise from the ‘com.android.aaptcompiler’ can sometimes be puzzling for Java developers. However, having a grounded understanding of the problem aids in developing efficient mitigation strategies. These include the examination of error messages, debugging, adapting the right coding practices and keeping your tools updated.
The ‘com.android.aaptcompiler’ is responsible for processing resources into a format that Android understands. It packs all resources files such as xml layouts, png images, etc., into one binary file which gets shipped with the apk. Hence, if you cannot extract a resource, it implies an error has occurred during this process.
Let’s delve into more pertinent strategies to troubleshoot this issue:
- Examination of Error Messages:
When extraction issues surface, the compiler usually displays error or warning messages. These messages should not be overlooked as they provide hints on what went wrong.
Error: java.lang.RuntimeException: AAPT: error: unable to open file for read: No such file or directory.
This piece of log shows a common issue where AAPT2 can not find a given file. Carefully inspect these messages and ensure the mentioned files are present at the right location.
- Debugging:
Java provides several debugging tools that can help pinpoint issues. Use the stack trace provided by exceptions thrown to identify affected areas in your code base.
debug{ jniDebuggable true }
Running the above snippet in your build.gradle file will enable debugging in your native code.
- Adapting Suitable Coding Practices:
A good practice involves referencing resources properly. Missing assets and files often lead to extraction errors. Especially relevant when using exogenous libraries, verify that all assets referenced in Xml or styles are available in the project structure.
<ImageView android:src="@drawable/myPic" ... /> //Ensure 'myPic' exists in the drawable folder of res directory.
- Up to date Tools:
Resource extraction errors could appear because the development environment is out of date or misconfigured. Therefore, regularly updating your IDE (like IntelliJ IDEA or Android Studio) and essential tools might resolve some of these issues. Also, check your Android SDK Tools and ensure AAPT is correctly installed and configured.
As Richard Branson rightly said, “You don’t learn to walk by following rules. You learn by doing and falling over”. There is no foolproof way to avoid bugs but there are certainly improved ways to fix them once identified. Ultimately, effective troubleshooting of resource extraction issues primarily relies on careful examination of error logs, efficient utilization of debugging tools, adoption of proficient coding habits, and sustaining your toolbox up to date.
Exploring Potential Causes of Aaptcompiler Failures
The Aaptcompiler or Android Asset Packaging Tool is a critical component in the Android development environment. However, it can sometimes fail due to various reasons. Let’s explore some potential causes of these failures and how they pertain to the inability of extracting resources from Com.Android.Aaptcompiler.
Syntax Errors in Resource Files
Any inconsistencies in XML or other resource files can lead to complications with Aaptcompiler. These files need to conform to specific standards and formats set by Android. Mistakes such as typos, invalid attributes, unrecognized tags or the lack of corresponding closing tags can cause breakdowns in compiling resources.
Example: <TextView android:id="@+id/sample_id" android:layout_width="wrap_content" /* closing tag missing */
Incompatible Image Types
Aaptcompiler supports specific image types. If your project contains incompatible images files (e.g., CMYK format JPEGs), you’ll likely encounter errors during the build.
Resource Naming Conflicts
If multiple resources share identical names but differ in case (android considers these as same), it could confuse the Aaptcompiler. It is advised to follow standard naming conventions for differentiating between similar resources.
Example: drawable/ic_menu.png drawable/IC_MENU.png
Invalid Characters in Resource Names
Including certain special characters or spaces in resource file names can cause Aaptcompiler to fail. Android only allows alphanumeric characters (a-z, 0-9), underscores, or period in resource names.
Exceeding the Resource Count Limit
There is a maximum limit (65,536) to the number of methods that can be referenced within a single DEX file – the executable file format used in the Android platform. Certain libraries include large numbers of methods, subsequently contributing towards this limit, which on exceeding can trigger a failure in Aaptcompiler.
Let’s consider the statement by Brian Kernighan, one of the developers of C programming language – “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” This suggestion applies to troubleshooting Aaptcompiler failures too. The key is to understand the Android resource structure and use its guidelines efficiently to minimize these occurrences Android Developer Documentation.
When addressing issues related to ‘Can Not Extract Resources From Com.Android.Aaptcompiler,’ focus should be on evaluating any incompatible resources, observing proper naming conventions, ensuring syntax correctness and being mindful about the method count limit. Following these guidelines can significantly reduce the chances of encountering errors related to Aaptcompiler.
Implementing Solutions for Com.Android.Aaptcompiler Problems
The
com.android.aaptcompiler
issue often presents itself when developers attempt to build an Android application and there exists a problem with the resource files. Basically, the Android Asset Packaging Tool (AAPT) handles resources compilation (including any PNG or JPG images, XML files, debug information, etc.) as well as managing the app manifest file creation process. When it fails to compile resources properly or extract them, it returns errors associated with the aaptcompiler.
One possible solution for implementing measures to address issues related to
com.android.aaptcompiler
is through the following steps:
1. Checking Resource Files:
Ensure all your resource files, including images and XML files, follow Android’s naming conventions. Filenames should contain only lowercase letters, numbers, or underscores. For instance, using capitals or special characters in names can trigger these problems.
HTML code example:
Improper Naming | Proper Naming |
---|---|
image-file.PNG |
image_file.png |
[icon].jpg |
icon.jpg |
It’s also essential to make sure that you have not added any unwanted files in the resource folders. If so, remove them immediately.
2. Sync Project with Gradle Files:
If erroneously added or removed dependencies in the Gradle file, synchronizing the project with its Gradle files can help rectify the issue.
You can use the command
File > Sync Project with Gradle Files.
on Android Studio’s top navigation bar.
3. Cleaning and Rebuilding the Project:
Cleaning the project removes all previous build artifacts that might be causing the problem. Then rebuilding the project will create new ones which can potentially eliminate obscure issues:
Use the commands
Build > Clean Project
then afterwards call
Build > Rebuild Project.
Considerations made while tackling the problem of
Cannot extract resources from com.android.aaptcompiler
, will have to revolve around ensuring that all the resources are present and correctly named, syncing versions of the necessary tools with gradle files, and cleaning and rebuilding the project. Both cases require similar solutions as they are closely tied to how AAPT processes and manages resources.
"Good code is its own best documentation."
– Steve McConnell, author of Code Complete. No clearer words could frame the importance of neatness and conformity when it comes to solving such problems.
Delving deeper into the spiral of complexities in the Android development world, a commonly stated conundrum is “Cannot Extract Resource From Com.Android.Aaptcompiler”. The obstacle lies within the Android Asset Packaging Tool (AAPT), an integral component in the Android SDK.
In deciphering the root of this problem/complaint ‘Can Not Extract Resource From Com.Android.Aaptcompiler’, we skirmish with various aspects. This compilation error arises predominantly when resources are improperly configured or inaccessible due to one or more reasons such as:
- Erroneous XML code
- Noisy hard drive
- Inaccessible or deleted resources
- Faulty Gradle configurations
- Comprehensively inspecting your XML files for any syntax errors or misplaced attributes and correcting them.
- Checking hardware status, including the functionality of your hard drive.
- Ensuring all referenced resource files exist and are placed correctly within the project hierarchy, if not, either reinstating them or removing their references from the code entirely.
- Refreshing Gradle by opting for Invalidate cache/restart from the File menu of Android Studio, helping also in optimization scenarios.
And providing a potential solution around these issues could entail:
Advanced debugging techniques may also come into play, scrutinizing logcat messages or availing profilers can aid in resolution of ‘Cannot Extract Resource From Com.Android.Aaptcompiler’. The semantics behind this exception can be derived using android’s built-in debug features or third-party debug tools that enable developers to delve deep into their code.
A quote from Marissa Mayer, former Vice President of Google Search Products and User Experience, says, “We need to maintain a productive tension between viewing code as craft and art – doing things right. And, at the end of the day, shipping product and recognizing that you can’t let your wish for perfection be the enemy of the good.”
. This is well-suited for our context , as it emphasizes that perfection in coding is crucial, but it should not hinder the ultimate goal of delivering a functional product.
I hope tackling this unique challenge turned out to be insightful, magnifying your understanding about the unruly exception we faced today, ‘Can Not Extract Resource From Com.Android.Aaptcompiler’ and its prospective resolutions. May this foster further exploration and discovery on your voyage to master the art of Java and Android Development.