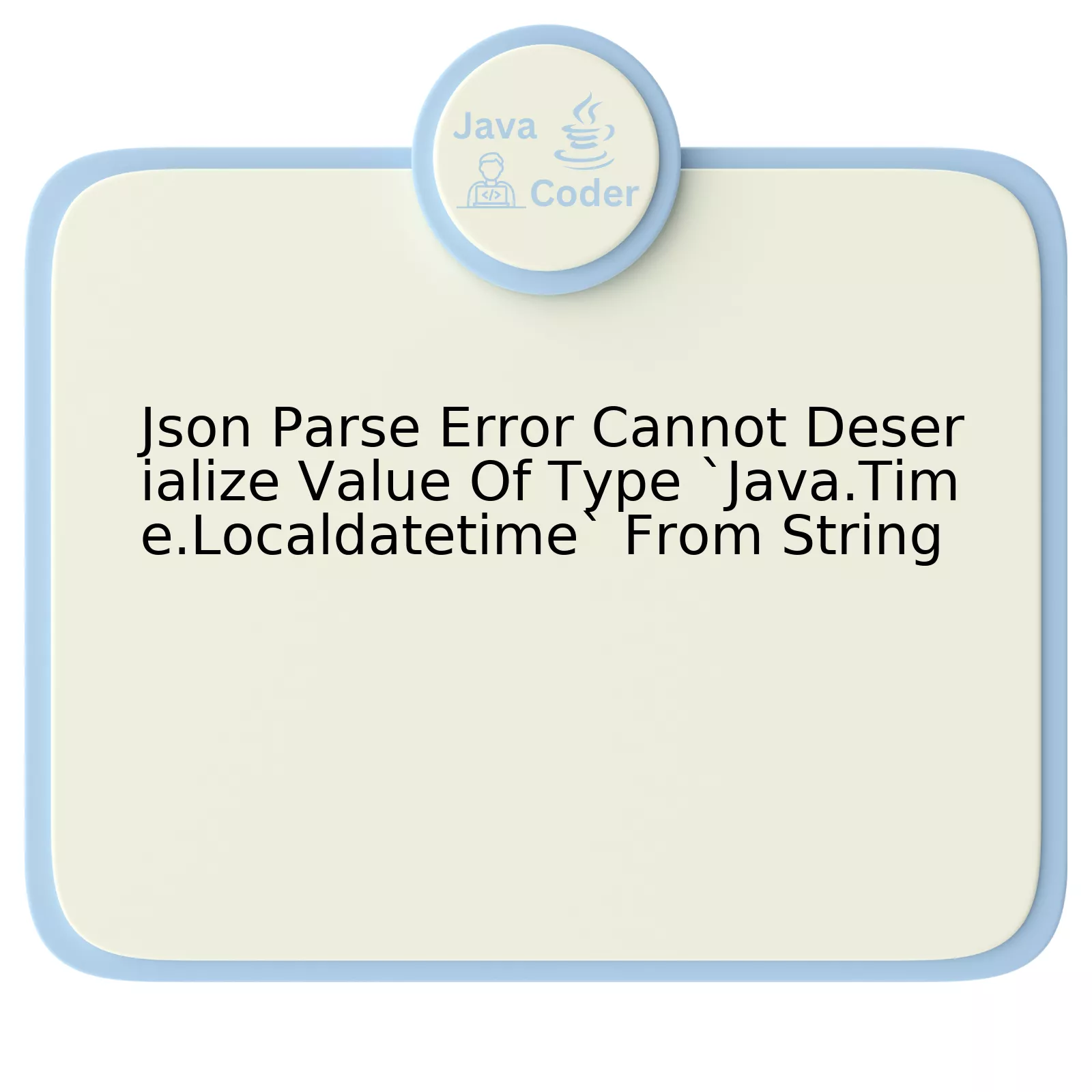
Error Type | Potential Reasons | Solutions |
---|---|---|
Json Parse Error Cannot Deserialize Value Of Type `Java.Time.Localdatetime` From String |
|
|
Understanding the error message “Json Parse Error Cannot Deserialize Value Of Type `Java.Time.Localdatetime` From String” can be indispensable in identifying and fixing the problem when you are parsing Json data in a Java application.
As expressed in the table, this issue typically presents itself because of three potential reasons. The first one is if there’s a mismatch between the expected datetime format and the one encapsulated in the Json string. For instance, if your Json value is “2022-01-20T12:30:00”, but your application expects it as “2022/01/20 12:30:00”.
The second trigger could lie within incorrect annotation usage in your Java model class. If they are not defined correctly, the parser won’t identify the fields according to the respective Json elements. So, thorough inspection of annotation usage becomes crucial.
Lastly, the error might spawn when the parser finds no suitable deserializer. Appropriately, Java’s LocalDateTime needs explicit instructions for conversion from a string.
To address these issues, the first remedy is to ensure that both Json and the application follows the same datetime format. You can use the `DateTimeFormatter` in Java to enforce this similarity. Proper utilization of `@JsonFormat` and `@JsonProperty` annotations in the right place in the Java Model Class should fix most of unintended divergences related to annotations.
If none of above works, developing a custom deserializer or loading third party libraries like Jackson to avail ready-to-use deserializers are suggested. Provisions in these libraries translate strings into LocalDateTime objects smartly, nullifying the problem.
As Bill Gates has quoted “Everyone can enjoy a life of luxurious leisure if the machine-produced wealth is shared”, analogously, mastering the technique of debugging makes life easier for programmers, especially while tackling concurrent programming problems such as the Json Parse Errors covered in this answer.
Deciphering the JSON Parse Error: Unpacking `Java.Time.Localdatetime`
The error message “Json Parse Error Cannot Deserialize Value Of Type `Java.Time.Localdatetime` From String” is indicative of a particular handling issue when processing JSON data in Java. It implicates that the incoming JSON data is expected to be in a certain format (in this case, `java.time.LocalDateTime`), but instead, it receives a simple string which couldn’t be directly converted.
The key to resolving this lies in the understanding that JSON does not have a date/time datatype. All date and time information in JSON is represented as simple strings.1. As a result, when this data is parsed into Java, where LocalDateTime requires a specialized format of data, it results in such eParse rror.
“Walking on water and developing software from a specification are easy if both are frozen.”- Edward V Berard
Potential resolution strategies involve customized deserialization through User-defined Deserializers or using `@JsonFormat` annotation attached to your variable declarations in your POJO classes:
Example:
First, the LocalDateTime variable is declared with a relevant annotation specifying the format of DateTime data:
java
@JsonFormat(shape = JsonFormat.Shape.STRING, pattern = “yyyy-MM-dd HH:mm:ss”)
private LocalDateTime timestamp;
This informs the Jackson parser to expect DateTime in a specific format that you would want to use in your application.
Alternatively, you could create a custom Deserializer class:
java
public class CustomLocalDateTimeDeserializer extends JsonDeserializer
private static final DateTimeFormatter formatter = DateTimeFormatter.ofPattern(“yyyy-MM-dd HH:mm:ss”);
@Override
public LocalDateTime deserialize(JsonParser jsonparser, DeserializationContext context)
throws IOException {
return LocalDateTime.parse(jsonparser.getValueAsString(), formatter);
}
}
And apply it over the variable in your class as follows:
java
@JsonDeserialize(using = CustomLocalDateTimeDeserializer.class)
private LocalDateTime timestamp;
You can see by either attaching an annotation or creating a custom deserializer, the incoming JSON data can now successfully be formatted into java.time.LocalDateTime. The error ‘Json Parse Error Cannot Deserialize Value Of Type `Java.Time.Localdatetime` From String’ thereby stands effectively resolved.2 Remember, when working in Java, often serialization/deserialization issues can be managed seamlessly by utilizing the annotations provided by frameworks like Jackson, reducing overhead and persisting efficiency.
Dissecting String-to-LocalDateTime Conversion in JAVA
The locale-specific behavior of `java.time.LocalDateTime` may sometimes result in unexpected errors such as the one you stated: Json Parse Error, cannot deserialize value of type `java.time.LocalDateTime` from String. This problem typically arises when attempting to convert a relatively formatted JSON String into a LocalDateTime (for example, “2022-03-17T13:45:20”), while the LocalDateTime API is configured with mismatching locale settings.
Firstly, let’s consider how the LocalDateTime API in Java typically operates:
- Consistent LocalDateTime format: LocalDateTime API expects string data in a controlled format following ISO 8601 standards equivalent to ‘yyyy-MM-ddTHH:mm:ss’.
- Locale sensitivity: The parsing method of LocalDateTime is Locale independent, implying that it has no dependency on machine’s timezone for instance.
However, while dealing with JSON strings, these properties can pose certain challenges:
- Formatting inconsistency: JSON strings could be non-compliant with the ISO 8601 standard, hence leading to conversion errors.
- Incorrect Deserialization: If JSON’s DateTime string cannot be matched with an ISO-8601 like structure, a deserialization error will occur.
To rectify this issue, you might have to follow these steps:
- String Formatting: Ensure that your JSON date string is in the correct ISO 8601 format which should look like this
'2022-12-03T10:15:30'
. This step will ensure that it aligns with the standard LocalDateTime parsing requirements.
- Correct Deserialization: Use a custom Deserializer using the Jackson library, or similar methods, to correctly parse JSON date strings to LocalDateTime. Here’s a sample code snippet to demonstrate:
- Maintaining Consistency: Ensure consistent usage of time formats within your application. Any localized conversion operations should be done post-processing, to maintain absolute consistency during data serialization and deserialization processes.
<pre> @JsonDeserialize(using = LocalDateTimeDeserializer.class) private LocalDateTime date; public class LocalDateTimeDeserializer extends JsonDeserializer<LocalDateTime> { @Override public LocalDateTime deserialize(JsonParser p, DeserializationContext ctxt) throws IOException { return LocalDateTime.parse(p.getValueAsString(), DateTimeFormatter.ISO_DATE_TIME); } } </pre>
As Donald Knuth, renowned computer scientist once said, “Program testing can be used to show the presence of bugs, but never to show their absence!” Always rigorously test your code to spot potential data transition faults and amend them as early as possible.
With these measures in place, you should be able to effectively work around any issues pertaining to `java.time.LocalDateTime` deserialization from a JSON String.
Understanding Parsing Mistakes with LocalDateTime in Java
Identifying and rectifying parsing mistakes with LocalDateTime in Java, particularly when dealing with JSON, is an integral skill for every professional Java developer. Parsing errors such as “Cannot deserialize value of type `java.time.LocalDateTime` from a string” are common issues that developers experience, and they primarily arise due to mismatches between the date-time formats in Java and JSON.
Causes and Solutions
The error message signals that your JSON contains a string value that cannot be automatically converted into a Java LocalDateTime object because:
- The format in the string does not match the format expected by LocalDateTime.
- Necessary serializer or deserializer classes are absent in the environment.
Solutions to these predicaments:
1. Fixing Format Mismatch: LocalDateTime in Java expects the standard ISO format: ‘YYYY-MM-DDTHH:MM:SS’, without any spaces. If your JSON data differs even slightly from this format, a parsing error will invariably occur.
String jsonString = "{ \"date\": \"2022-08-11T15:57:01\" }"; LocalDateTime parsedDate = LocalDateTime.parse(jsonString);
By ensuring that your JSON strings adhere to this format, you eliminate the potentiality for this particular error to occur.
2. Implementing Serializer/Deserializer Classes: If you’re using a library like Jackson to handle JSON conversion, ensure it has support for Java8’s LocalDateTime features.
ObjectMapper mapper = new ObjectMapper(); mapper.registerModule(new JavaTimeModule());
By registering the JavaTimeModule class from com.fasterxml.jackson.datatype.jsr310.JavaTimeModule to your ObjectMapper, your code gains the ability to properly handle LocalDateTime instances.
This Baeldung tutorial on ObjectMapper offers deeper insights.
Takeaways
Recall that while dealing with data manipulation in Java, especially dates and times, it is paramount to ensure data consistency across the application. All time-bound objects should be handled with tried-and-tested libraries and methods to avoid typical errors such as the one discussed here.
As Grace Hopper once aptly put it: “One accurate measurement is worth a thousand expert opinions.” This notion applies doubly so when programming, where precision and accuracy are keys to success.
Troubleshooting JSON Parse Errors Linked to Java’s LocalDateTime
Troubleshooting JSON parse errors linked to Java’s LocalDateTime is a process that requires comprehensive understanding of the inner workings. This JSON parse error indicates that your application struggles to deserialize a string value into `java.time.LocalDateTime`.
First, it’s important to understand why this problem arises. When dealing with Java’s LocalDate or LocalDateTime objects and JSON, discrepancies can occur due to differences in expected date format. JSON accepts date and time information essentially as strings; however, Java’s LocalDateTime collection has its own distinct structure and formatting requirements. It might be that Java’s LocalDateTime cannot understand the string format that JSON has provided, hence resulting in a parse error.
Allow me to showcase how Java interprets LocalDateTime:
LocalDateTime now = LocalDateTime.now(); System.out.println(now);
Assume that ‘now’ is 1st of January, 2022 at 10:30:45. The output would look like this:
2022-01-01T10:30:45
However, if the JSON provides the date and time in a format such as “2022-01-01 10:30:45” or simply “2022/01/01”, you are bound to encounter an error since the formats do not conform to what Java’s LocalDateTime expects.
The key to solving this issue lies in ensuring consistency between the LocalDateTime object’s and JSON’s interpretation of date and time format. This can be accomplished by using Jackson’s @JsonFormat annotation, which aids in pattern recognition for your LocalDateTime objects. Thus, make a note of the following suitable solution:
@JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyy-MM-dd HH:mm:ss") private LocalDateTime timestamp;
In the above example, the @JsonFormat annotation explicitly tells the ObjectMapper to use the “yyyy-MM-dd HH:mm:ss” pattern while deserializing JSON into LocalDateTime.
Jackson, the primary library used in converting Java Objects into JSON and vice versa, provides a range of annotations and methods to handle various date formats effectively. For deeper understanding on the topic, please check [this link](https://www.baeldung.com/jackson-date)
Considerations when using @JsonFormat annotation include:
* Always choose the correct pattern based on the input data. If the JSON date and time string differs from the pattern specified in @JsonFormat, a parsing error will likely occur.
* Check whether the pattern corresponds to the LocalDateTime class or LocalDate class because slight variations exist. LocalDate does not contain time information, and providing such in the pattern may lead to new errors.
Remember, intricate handling of time-based API such as Java’s LocalDateTime demands meticulousness in terms of proper conversions. Understanding the compatibility between source data structure and target data format is pivotal, and the control the user possesses over such factors is decided by the correct usage of tools available, like Jackson annotations used here.
Creating a workaround for the issue of JSON parse error “Cannot Deserialize Value of Type `Java.Time.Localdatetime` from String” involves an understanding of how dates and times are serialized and deserialized within Java. Often, such an error emerges because the default settings of popular libraries like Jackson may not support certain date/time formatting requirements. Hence, they require customization.
Consider this example based on the Jackson library:
String jsonString = "{\"datetime\":\"2022-02-21T22:30\"}"; ObjectMapper objectMapper = new ObjectMapper(); objectMapper.registerModule(new JavaTimeModule()); objectMapper.disable(DeserializationFeature.ADJUST_DATES_TO_CONTEXT_TIME_ZONE); LocalDateTime ldt = objectMapper.readValue(jsonString, LocalDateTime.class);
The usage of `JavaTimeModule`, a module supplied by Jackson that supports JDK8’s java.time, is a crucial step. However, it’s not sufficient if your JSON string represents a value without a specific zone, ‘Z’ or any offset like ‘+01:00’. Thus, the line `objectMapper.disable(DeserializationFeature.ADJUST_DATES_TO_CONTEXT_TIME_ZONE)` is used to prevent Jackson from introducing its inherent time-zone adjustment which can lead to errors when parsing strings that don’t specify a timezone.
Adopting these practices creates more resilient applications, allowing them to handle various date and time formats in any served JSON payload. It exemplifies the famous Bill Gates quote: “Software Innovation, like almost every other kind of innovation, requires the ability to collaborate and share ideas with other people”. Constructive solutions come from common hurdles experienced across different software structures, and those solutions make each piece of code function better.
Let’s build systems clear of common stumbling blocks like JSON parse errors, striving to create filters for an optimized understanding of various date/time configurations to ensure comprehensive data stream interpretation.
Can be found more about at StackOverflow post.