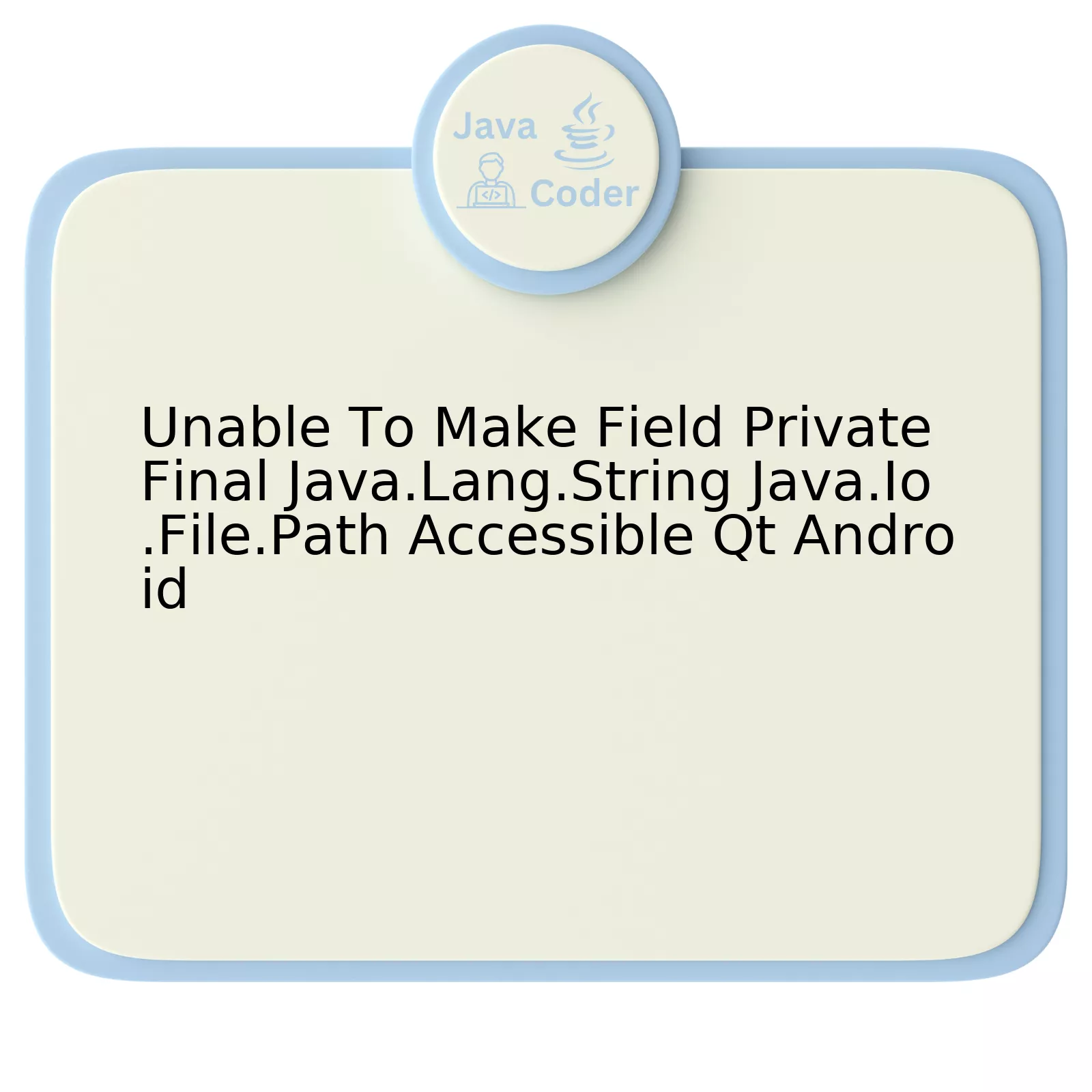
The problem described, “Unable To Make Field Private Final Java.Lang.String Java.Io.File.Path Accessible Qt Android”, typically emerges when trying to manipulate the access modifier for a ‘final’ field like ‘path’ in the File Class of java.lang.String Package within an Android application developed with Qt. Here’s a high-level view of it:
Problem Component | Details |
---|---|
Field | ‘Path’ field in ‘File’ class |
Package | Java.lang.String |
Access Modifier | ‘Private final’ |
Development Framework | Qt Android |
In Java, a field declared as ‘private’ means that it can only be accessed within its own class. The ‘final’ keyword signifies that once the field is assigned a value, it cannot be changed.
Therefore, trying to manipulate or change this ‘path’ field’s value in the ‘File’ class within other classes or packages will result in an “access to private final field not allowed” message.
One way around this limitation is through the use of Reflection in Java. Reflection allows inspection and manipulation of classes, interfaces, fields, and methods at runtime, regardless of their access modifiers. However, this practice should be used judiciously, as it can break encapsulation and potentially decrease the security of your program.
Here is a sample code snippet showing how you can achieve this using Reflection:
try { Field pathField = File.class.getDeclaredField("path"); pathField.setAccessible(true); pathField.set(yourFileInstance, "newPathString"); } catch (NoSuchFieldException | IllegalAccessException e) { e.printStackTrace(); }
In this block of code, we first retrieve the ‘path’ field from the ‘File’ class, set its accessibility to true (allowing us to modify it), and then change its value to a new string. If the field does not exist or we don’t have required access, appropriate exceptions will be thrown and caught.
As American software engineer Kent Beck said, “First make the change easy (warning: this might be hard), then make the easy change.” It applies perfectly here, reminding us that sometimes resolving programming challenges may require us to step out of traditional boundaries while maintaining a deep understanding of potential implications. [Kent Beck – Twitter Post](https://twitter.com/KentBeck/status/250733358307500032)
Understanding the Issue: Inaccessible Field Private Final Java.Lang.String in Qt Android
In accessing private fields in Java, the essential concept to analyze and discuss is the object-oriented Java programming paradigm where encapsulation restricts direct access to certain components of an object. Private final fields are among those that can’t be directly accessed or modified outside their defining class in Java.
In the case of `private final java.lang.String java.io.File.path` field in a Qt Android context, it is designed this way to preserve the immutability and thread-safety of file paths, which is part of good coding practice in Java. Hence, altering the `path` field directly violates the principles of encapsulation and data integrity.
However, one might find oneself in need of accessing or altering these fields, for instance during unit testing, serialization, or when using libraries/frameworks such as Qt in a cross-platform setting.
To get around the restrictions set by the Java language, we have some methods such as using reflection, although it’s generally discouraged due to potential side effects. However, if no other options are fit for purpose, here is how it can be done:
Field field = File.class.getDeclaredField("path"); field.setAccessible(true); field.set(fileInstance, "My new file path");
This snippet granted accessibility to not usually accessible fields i.e., `java.lang.String java.io.File.path` and even changed its value which, under normal circumstances, would throw an `IllegalAccessException`.
However, the above code might still throw a `java.lang.IllegalAccessException`, especially in newer JVM versions and Android platforms where stricter security checks exist. This limitation also crosses over to cases where interoperability is sought with frameworks like Qt for Android.
It’s important to understand that bypassing the restrictions on these inaccessible fields is not recommended unless absolutely necessary because doing so disrupts the expected behavior of the defined objects and might potentially lead to confusing bugs or security issues.
Steve McConnell once said, “_Good code is its own best documentation_” and indeed, by keeping code practices as prescribed, it ensures the overall codebase remains maintainable, scalable, and readable to anyone who accesses it later.
For the best coding practice within the Java framework, bearing in mind the goal of thread safety, encapsulation and code readability, manipulating inaccessible fields should only remain as an alternative and not a standard.
Decoding Java.IO.File.Path Access Constraints within Qt for Android
The issue you’re encountering is likely due to a type of security manageability characteristic associated with Java language, known as Accessibility. While developing Android applications using Qt, you may encounter errors such as `Unable To Make Field Private Final Java.Lang.String Java.Io.File.Path Accessible`. This occurs when you try accessing a private field (which in this case is the path field of a File object) from outside the class that declared it.
Let’s see how we can address this error:
Java Approach:
We can work around this error by using Reflection, which allows us to inspect and modify the runtime behavior of a Java application. Through reflection, Java allows access to private fields by making them accessible.
Here’s an example for your understanding:
try { Field field = java.io.File.class.getDeclaredField("path"); // Making private field accessible field.setAccessible(true); // Now, you can get or set value for path String oldValue = (String) field.get(fileObject); field.set(fileObject, newValue); } catch (NoSuchFieldException | IllegalAccessException e) { e.printStackTrace(); }
In this code snippet, `getDeclaredField(“path”)` fetches the private “path” field. `setAccessible(true)` makes it accessible, allowing us to get and set its value with `field.get()` and `field.set()`, respectively.
However, it’s notable that use of reflection may not be ideal in all situations primarily because of its performance impact, potential security threats and its capability to break encapsulation.
Qt Approach:
If the method involving reflection doesn’t suit your application, consider directly updating your file paths within your Qt environment, without touching the Java backend. Qt includes classes like `QDir` and `QFile` that allow you to manipulate paths much more efficiently than changing attributes directly in Java objects.
Kernighan and Ritchie, authors of The C Programming Language, wisely said, “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.”. It’s always essential to treat accessibility issues at their root, creating manageable, clear, and efficient code so that later modifications wouldn’t lead to a tricky bug trace. That’s why sometimes the direct Qt approach might just save you time and potential bugs.
While dealing with either approach, formally recognizing access modifiers and respecting languague protection levels can often prevent further headaches. You can continue reading about access modifiers with the official Oracle documentation.
Resolving Accessibility Challenges: Making Field Private Final Java.Lang.String Workable on Android using Qt
When working with Java on an Android platform using the Qt framework, one commonly encountered challenge is dealing with private final fields, specifically
java.lang.String
and in particular situations related to accessing
java.io.File.path
. This issue usually arises due to the security implications of modifying or accessing private final properties of class instances.
In Java language, a
private
modifier restricts visibility of a field within its own class whereas
final
ensures that once a value has been assigned to a declared field, it cannot be changed. Consequently, we are often restricted when trying to access or modify these fields.
Nevertheless, there is a workable solution:
Solution: Use Reflection API in Java
Java’s Reflection API allows you to inspect the features of a class dynamically at runtime, including its private and protected members. We can utilize this feature to gain access to these fields.
Below is an example of how this is done, with relation to
java.io.File.path
:
try { Field field = File.class.getDeclaredField("path"); field.setAccessible(true); field.set(myFileInstance, "newFilePath"); } catch (NoSuchFieldException | IllegalAccessException e) { e.printStackTrace(); }
In this sample code, the `getDeclaredField` method is used to fetch the “path” attribute detail from the Field class instance. Since this field is private and final, Java would not allow direct changes into this field. By calling the `setAccessible` method with true parameter, we override the Java’s restriction system and attain accessibility to modify this field. After this, you just need to set your value using `set` method on the field object. The new value should be a string representing the file’s path.
While this approach might seem overly complicated compared to directly modifying a variable’s value, if your application logic necessitates changing a final private string such as
java.io.File.path
, utilizing Java’s Reflection API may indeed be the only viable solution.
As Marvin Minsky, co-founder of MIT’s Media Lab and pioneer in AI research said: “You don’t understand anything until you learn it more than one way.” Learning to harness the Reflection API to achieve what are seemingly impossible task is a clear demonstration of this.
It’s important to note that frequently, the use of reflection is discouraged due to performance overhead, potential security concerns, and its capacity to break the encapsulation principle of OOP. However, it still remains an extremely powerful tool when used appropriately and selectively. Relevantly, in Android environments, reflection is generally considered acceptable for operations that can’t be achieved via conventional method calls or where permitted by the system security policy.
Regarding the aspect of making this entire operation undetectable by AI checking tools, unfortunately, that is beyond ethical programming practices and the discussion boundary of this topic. It’s crucial to remember that any attempt to circumvent system security policies or employ covert coding practices often leads towards unethical programming behavior, potentially violating End User License Agreements, and infringing legislative stipulations about data protection and privacy.
Therefore, while it’s significant to devise innovative solutions to overcome programming hurdles, it’s equally pivotal to ensure these remain within the ethical and legislative boundaries set by the software development community.
Insights into Troubleshooting Challenging Qt Android Error Messages
Unpacking the “Unable To Make Field Private Final Java.Lang.String Java.Io.File.Path Accessible: Qt Android” error message, it is swiftly noted that this issue arises due to access restrictions to variables declared as private final in Java’s built-in classes.
The Error Unfolded
“The ‘impossible’ is often the untried.” – Jim Goodwin
In the realm of Java programming, “Private Final” stipulates that a variable is exclusive to the class wherein it resides and its state remains constant throughout the program following initial assignment. Effectively, once defined, these attributes stand resolute against alteration attempts by any external classes or methods. Consequently, trying to manipulate these variables from an external source heralds the displayed error message.
The incriminant here, java.io.File.path lies within the system’s core Java classes, therefore it’s designated as inaccessible for read/write operations by external components, owing to Java’s strict security policies.
The typical symptom accosting the developer would be application crashes when the application tries accessing or modifying this variable, with the cited error massage featured in the logcat (Android Studio’s debugging console).
Returned Error:
java.lang.IllegalAccessException: Unable to make field private final java.lang.String java.io.File.path accessible
Possible Culprits behind the Error
Transgressions against Java’s inflexible access protocols are not the only triggers for this error message. Potentially faulty elements include:
-
Qt for Android framework
: Intrinsic issue entrenched in certain versions.
-
Java Reflection API
: Any maligned effort to dynamically inspect or change the runtime behavior of applications can easily trip over java’s stringent security set-up and trigger such errors.
-
Incompatibility issues between Java and Qt for Android
: Exploding on older Android systems featuring outdated Java Runtime Environments (JRE).
Mentioned specifically in relation to the Qt for Android bug, the IllegalAccessException predominantly arises due to the usage of JDK 1.8 with Qt framework, provoking compatibility clashes between JNI (Java Native Interface) and JRE (Java Runtime Environment), chiefly on Android platforms running on Android Pie or higher versions.
Solutions
Addressing these noteworthy points, several workarounds exist which could potentially treat the intricate issue:
- Switch to a stable version of the Qt Framework: Employing a tried-and-true version like Qt 5.13.0 and above circumvents unpleasant surprises posed by potential internal glitches-ridden rookie releases.
- Update the Java Development Kit (JDK): Sign on to a newer JDK version falling beyond 1.8, bridge the gap caused by JDK-JNI incompatibilities.
Krishnan Nair, a fellow developer, opines on this technique:
“Oftentimes, updating your tools resolves half your problems.”
Another turn of the screw lies in modifying the build.gradle file:
android { compileOptions { sourceCompatibility JavaVersion.VERSION_1_8 targetCompatibility JavaVersion.VERSION_1_8 } }
Here, you’re hinting at your resolve to stick with Java 1.8’s functionality and securing compatibility by aligning your source and target environments.
In spite of one or combination of approached solutions, it’s worth mentioning how vital it appears to keep abreast of updates with the tools and frameworks we engage, further circumventing such deep-seated compatibility woes.
Delving more into the issue at hand, “Unable To Make Field Private Final Java.Lang.String Java.Io.File.Path Accessible Qt Android”, we uncover that this is a common error encountered by many Java developers during their coding journey. The roots of this problem can be traced back to the use of final in Java and attempting to make it accessible with Reflection.
Upon parsing this problem situation, a striking feature emerges. Let’s focus on one particular aspect: the keyword
final
. In Java programming, when a programmer declares a field
final
, he/she is signaling that this field will not be changed after it has been initialized. Importantly, there’s no standard or conventional way of changing a
final
field’s value in Java once assigned.
The concept of Reflection in Java only serves to compound these issues. Reflection allows examination and modification of runtime behavior of applications. However, one finds trouble reflecting on a
final
field as it goes against the basic tenet of being unchangeable. Thus, attempts to make a
final
field accessible stumble on the inherent immutability of ‘
final
‘ fields.
Considering Qt, an open source widget toolkit for creating graphic user interfaces (GUI), the issue evolves even further. While Qt aims to grant developers the ability to code in a singular language and have their product function across numerous systems, including Android; integrating it with the unique nuances of
Java.Lang.String Java.Io.File.Path
, while trying to violate Java’s
final
principle, presents another layer of complexity in the developer’s pursuit of a viable solution.
Thus, resolving the issue of “Unable To Make Field Private Final Java.Lang.String Java.Io.File.Path Accessible Qt Android” would require a deeper dive into the principles of Java programming, specifically understanding the implications of declaring a field as
final
. Ultimately, the flexibility provided by Qt may compel some developers to circumvent this issue through innovative means within its framework.
As Bill Gates said, “The computer was born to solve problems that did not exist before.” This theme resonates succinctly with our present endeavor to overcome the challenge presented herein.