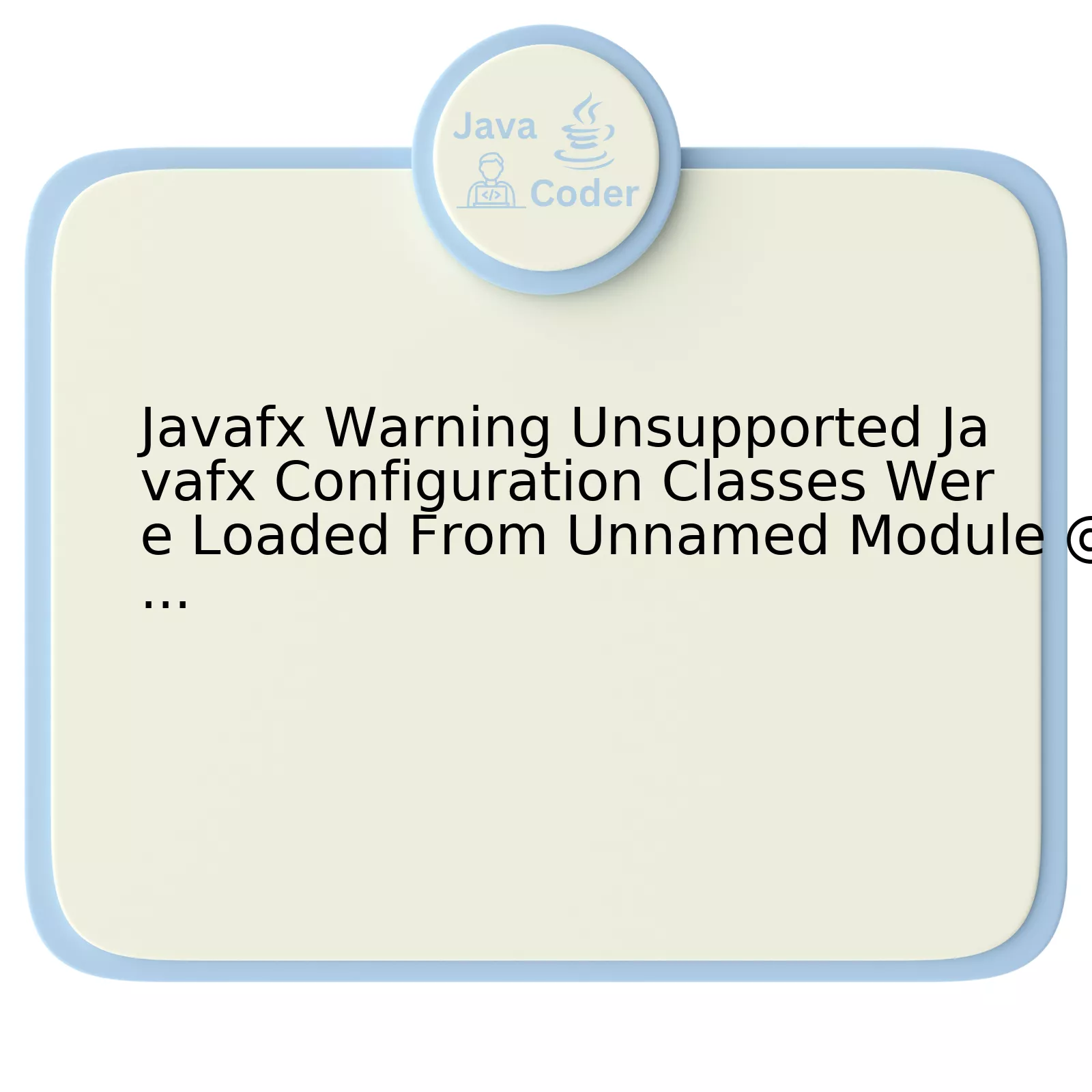
The warning message “Unsupported JavaFX Configuration: Classes were loaded from unnamed module @…” denotes a circumstance where JavaFX classes and the application itself are being loaded from different modules, resulting in an unsupported configuration. This commonly occurs when using JavaFX with Java 11 or above, as JavaFX was decoupled starting with Java 11.
Below is a detailed breakdown of the components involved:
Component | Description |
JavaFX | This is a software platform used for creating and delivering desktop applications. From Java version 11, it’s no longer included in the JDK. |
Classes | Blueprints that define object properties (attributes) and actions (methods). In context, this refers to JavaFX’s architectural elements. |
Loading | A stage in the lifecycle of a class involving fetching its bytecode for execution. The warning message suggests that loading has been performed in different modules. |
Unnamed Module | A type of module introduced in Java 9. If an application is not modularized, it operates within an implicit ‘unnamed’ module during runtime to maintain compatibility. |
@… | This represents the hashcode of the object from which the classes are loaded (i.e., unnamed module instance). |
To resolve this issue you must ensure that the runtime system and JavaFX classes belong to the same module. This could be accomplished by running the application as a modular application or ensuring without the module system. Additionally, having both JavaFX and your application on the module-path will help avoid the problem. You can do so by passing relevant command-line arguments to the JVM.
Consider the below example to run through command-line:
java --module-path /path/to/your/javafxSDK/lib --add-modules javafx.controls,javafx.fxml -jar YourApp.jar
Here:
* ‘–module-path’ directive points to the location of your JavaFX SDK library.
* ‘–add-modules’ provides the list of necessary JavaFX modules separated by commas.
* ‘-jar YourApp.jar’ starts the application from your packaged jar file.
“In programming, everything we view as a mistake will lead us to improve it.” – Bjarne Stroustrup. Even warnings like the one discussed are opportunities for developers to better understand and apply technologies like JavaFX.
Poease refer to Oracle’s official JavaFX installation guide for comprehensive instructions.
Understanding the Warning: Unsupported JavaFX Configuration Classes
Understanding the warning “Unsupported JavaFX Configuration Classes” in Java involves digging into the internals of both Java and JavaFX.
The warning, often appearing as follows:
WARNING: Unsupported JavaFX configuration: classes were loaded from 'unnamed module @...'
, typically comes up while executing a JavaFX application with Java 11 or higher. This usually signifies that your application is running on a modular path instead of the classpath which can lead to runtime unpredictabilities.
When you run a JavaFX application with Java 11 or higher, the Java platform module system kicks in. The platform module system, introduced in Java 9, organizes JAR files and classes into modules, each with its own namespace.
In the absence of a defined module-info.java file—which delineates the modules your application depends upon—the system places all uncovered items into an unnamed module, aslamming together every package inside one common namespace. If JavaFX classes, not designed for this particular operation, are loaded from the unnamed module, it leads to said warning.
To deal with this issue, consider these steps:
– Encapsulate your JavaFX code into a proper module by creating a module-info.java file at the root of your source code hierarchy.
– In the module-info.java file, declare your JavaFX dependencies using requires directives. A simplified version looks like this:
module my.module { requires javafx.controls; requires javafx.fxml; }
This tells the JVM about the dependencies between your module and other modules within JDK, hence eliminating the need for the unnamed module.
With the provided context, this quote from Neal Ford seems apposite: “Objects, abstraction, data structures, and modularity are not just central to computer programming. They’re also central ways to represent knowledge.” Understanding how modularity applies to Java applications—a compelling feature of modern Java versions—can be crucial in dealing with warnings such as “Unsupported JavaFX Configuration Classes.”
By following these guidelines, you should be able to understand, manage, and avoid the “Unsupported JavaFX configuration” warning efficiently. Your experience in dealing with JavaFX code will significantly improve, allowing you to create more reliable JavaFX applications.
Resolving JavaFX Configuration Loading Issues
JavaFX configuration loading issues, specifically the one that throws a warning “Unsupported JavaFX Configuration classes were loaded from unnamed module”, can cause a lot of frustration among Java developers as it may interfere with the smooth execution of their program. However, by understanding its roots and applying appropriate solutions, these issues can be easily resolved.
Understanding the Issue
This warning typically appears when the required libraries for configuring JavaFX are loaded from an ‘unnamed’ module. This ‘unamed’ module term refers to the automatic module, a feature introduced in Java 9. The modules that are not explicitly declared as modules are known as automatic modules, hence the term ‘unnamed’.
The primary reason behind this issue is improper encapsulation. Since Java 9, the Java platform has been modularized, which means each part is encased within its own boundaries. Hence, the classes get loaded into the JVM from modules, requiring prerequisites to guide this process correctly.
When these prerequisites aren’t met or are incorrectly calibrated, it can lead to a warning that essentially says: the JavaFX configuration class, rather than being loaded from its dedicated JavaFX module, was loaded from an ‘unnamed’ module.
How to Address this Issue
There are three main steps to address this issue:
Building Modules Explicitly
Since the warning primarily occurs due to the lack of explicit named modules, declaring the modules explicitly might solve the problem. The
module-info.java
file can declare the required modules. Here’s an example:
module com.myapp { requires javafx.controls; exports com.myapp to javafx.graphics; }
In this piece of code, ‘com.myapp’ is your application, which requires the ‘javafx.controls’ in order to function properly. The command ‘exports’ signifies the components that can interact with this module.
Following Proper Encapsulation
Java, post JDK 9, made the encapsulation process smoother. By following the principles of proper encapsulation and keeping the modules self-contained, the JVM can load classes smoother, thus avoiding the issue of unnamed modules.
Adjusting IDE Settings
Depending on the IDE you’re using, there might be settings to adjust how modules (especially automatic ones) are handled. For example, IntelliJ IDEA offers options under Project Settings where one can set modules and dependencies.
Albert Einstein once said, “You cannot solve a problem with the same thinking that led us to this problem.” This quote certainly rings true in this scenario. Understanding JavaFX configuration, its issues, and coming up with the appropriate fixes requires challenging established norms, habitual ways of coding, and embracing new paradigms like modular programming.
For more information on modules in Java, you can refer to Oracle’s official documentation.
Please note, this advice will help quell warnings but if there are errors during runtime caused by misconfigured libraries or other issues, separate debugging may be necessary.
Implications of Unnamed Module in JavaFX
JAVA FX Warning color=”#C00″>”Unsupported JavaFX Configuration: Classes were loaded from unnamed module @….” is a warning that developers commonly encounter when working with JavaFX, particularly in regards to the implications of the unnamed module.
Firstly, we need to understand what unnamed modules in Java refer to. In Java 9, the Java platform was modularized, meaning that the JDK was divided into a set of standard modules. This feature allows developers to include only necessary modules, reducing application size and increasing security and performance. Since not all classes belong to a named module (defined module) – those which don’t are categorized under
unnamed modules
by default.
“Modularity is a general system concept where concerns are separated such that modules can be developed, tested, optimized, scaled, and refactored independently” – Samuel A. Ajila, expert on software modularity
This warning message typically arises when some classes in the application run on unnamed modules but make use of JavaFX features intended for named modules. It’s essentially a reminder that you’re using an unsupported configuration. Although this doesn’t necessarily prevent your application from running, it may limit your ability to leverage certain benefits of modularity. It could also potentially cause issues in future versions of Java if changes are made regarding how unnamed modules are handled.
Here are the most important implications:
To eliminate this warning, consider transitioning your code to a proper modular form. You can specify your application as a named module that requires javafx.base, javafx.graphics, etc., as needed by your program. A sample ‘module-info.java’ file would look something like:
code
module com.myapp {
requires javafx.controls;
requires javafx.fxml;
//include any other necessary modules here.
}
The modular form offers several benefits including enhanced security and maintainabilitysource.
Strategies for Effective Management of Unsupported JavaFX Configurations
Managing unsupported JavaFX configurations can be a challenging task for many Java developers. Specifically in the issue you mentioned i.e.,
Javafx Warning Unsupported JavaFX Configuration Classes were loaded from unnamed module @....
, arises as the IDE fails to place the resources or .fxml files in the correct location or package. Thus, it leads to a number of undesired problems including failed application launches or even corrupt applications.
Here are some strategies that could potentially remedy these obstacles:
- Meticulous attention to classpaths
HTML content should regularly check and ensure that their JavaFX library file locations correspond correctly with the relevant classpath within their IDE. Modules are only unnamed when they come from the classpath instead of the module-path. Hence, ensuring an accurate classpath is indeed a vital step.
- Configuration File Checking
Checking all configuration file paths involved in your project is vital. There are instances where, even with valid file path entries in the IDE settings, the JavaFX resource files might still be placed incorrectly. Double-checking the configuration files help detecting these inaccuracies.
- Adequate management of IDE versions
It is essential to remember that different IDEs have separate rudimentary requirements for successfully running JavaFX projects. Your JavaFX configuration classes may turn unsupported due to lags in software updates or version mismatches.
- Taking assistance from Community Help
Online tech support communities play a major role in highlighting common problems that arise while working with JavaFX. For instance, platforms such as StackOverflow and other coding forums might provide valuable insights into similar problems faced by others and their solutions.
Following the above-mentioned strategies will give the developer more control over the operational flows and reduce the risk of errors cropping up as a result of unsupported JavaFX configurations. However, there is no definitive solution because it’s dependent on project specific issues. Case-by-case troubleshooting will always be a key component.
As Bill Gates quoted, “Everyone needs a coach. It doesn’t matter whether you’re a basketball player, a tennis player, a gymnast or a bridge player.” This quote is pertinent to developers too, seeking external help when needed can aid in navigating through technical challenges.
The warning you’re encountering regarding Unsupported JavaFX configuration classes loaded from unnamed module often arises due to compatibility issues between different Java versions, particularly during the transition from JDK 8 to JDK 11. This discrepancy often manifests itself due to the modularity introduced by JDK 9, resulting in binding inconsistencies.
Here’s a quick rundown of aspects related to this issue:
• Unnamed Modules: In the pre-JDK 9 era, every class loaded from the classpath belonged to the default package. As per JDK 9 and onwards, such classes are assigned to an intrinsic entity, termed as ‘Unnamed Module’.
• Unsupported Configuration: The warning signifies that certain JavaFX properties may not behave as expected due to inconsistent loading of these classes. This is attributed to the fact that crucial JavaFX classes are housed in named modules while your application resides elsewhere.
One plausible solution to mitigate this concern is to ensure your application classes reside within named modules similar to JavaFX classes. This essentially mandates a modular approach to Java application development.
java
module com.example.application {
requires javafx.controls;
… // other dependencies
}
However, this process might prove cumbersome; particularly when grappling with monolithic code-bases or dealing with a multitude of third-party libraries.
Alternatively, consider using build tools, such as Maven or Gradle, which offer plugins to assist with the transition. These plugins wrap up non-modularized jars as modular ones before assembling your final application.
Noted software architect “Robert C. Martin” encapsulates this notion perfectly by quoting:
> “a good architecture allows major decisions to be deferred.”
Which correlates impeccably in this context: deferring the choice of transitioning to a fully modular structure until it becomes indispensable paves way for uninterrupted development cycles.
For deeper insights and further exploration, refer to this excellent resource titled Project Jigsaw Quick Start guide.
Making necessary modifications in accordance to the evolving Java ecosystem mitigates these warnings ensuring optimized functionality of your JavaFX applications.