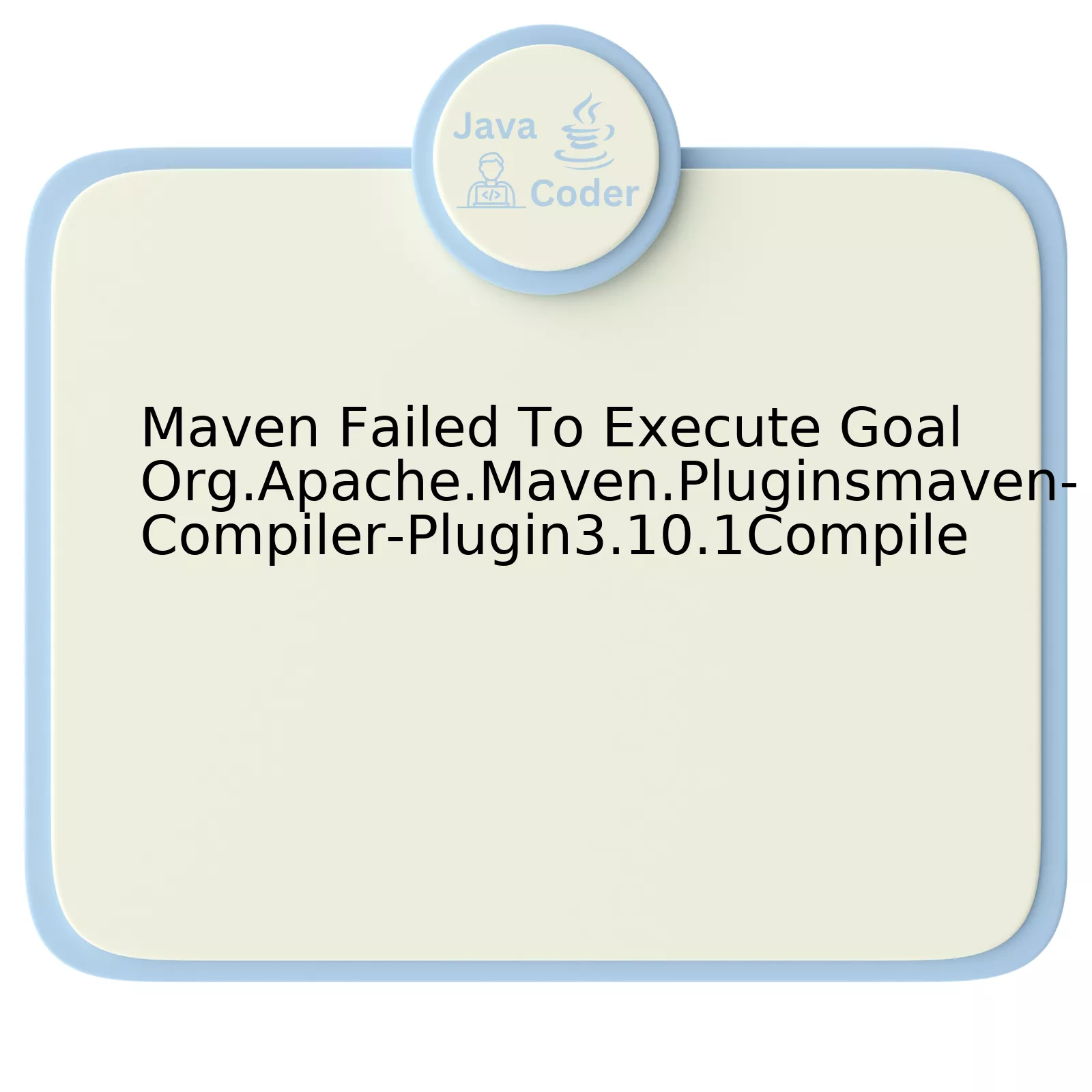
For a comprehensive understanding, let’s delve into these points:
Regarding the issue of Maven failing to execute a goal, it commonly unfolds due to incorrect or incomplete software configurations. One might overlook the crucial aspect of specifying proper environment variables. It is important to correctly set up Maven’s environment variables such as PATH and particularly JAVA_HOME.
The maven-Compiler-Plugin:3.1 compile error often occurs when using support-lacking Java versions or incompatible libraries. Developers should employ only those Java versions that the plugin supports and certify libraries’ compatibility in concurrence with the project requirements.
On the topic of :compile goal failure, it often stems from incomplete or even incorrect dependencies. A meticulous verification of the project’s pom.xml configuration will prove beneficial, ensuring no dependencies are missing or incorrect while updating the necessary ones where required.
According to Edsger Dijkstra, a prominent figure in the field of computer science, “If debugging is the process of removing bugs, then programming must be the process of putting them in.” This quote aptly suits the scenario. We are often bound to run into hiccups in our coding journey, like this Maven compilation error. But recognising and navigating through them is what makes us better, more adept developers.
Understanding the Maven Compiler Plugin Error
The Maven Compiler Plugin, typically used in a Java project, is an essential feature in Maven that allows for the compilation of source codes. Understanding why you are encountering the error “Maven Failed To Execute Goal Org.Apache.Maven.Pluginsmaven-Compiler-Plugin3.10.1Compile” requires diving into possible scenarios or reasons.
By default, Maven Compiler Plugin uses the version of your JDK to compile your source code which might lead to compatibility issues if your source code and JDK version mismatch. This could be one reason behind the issue.
Possible Reason 1: JDK version mismatch
Check if your JDK version matches with the source code version. The default source setting is 1.5, and if your JDK version is older, this can cause problems.
To resolve this:
Implement these adjustments directly to your
pom.xml
file:
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.10.1</version> <configuration> <source>1.8</source> <target>1.8</target> </configuration> <plugin> </plugins> </build>
Where ‘source’ and ‘target’ values must be compatible with your JDK version.
Possible Reason 2: Dependencies Issues
Sometimes, the error may not reside within the Maven Compiler Plugin itself but elsewhere – such as inside the various other plugins or dependencies that Maven is coordinated with.
Validate your project’s dependencies and plugins. In some cases, missing or misconfigured project metadata, including dependencies or parent POMs, could result in this error.
Also, ensure your POM file is not corrupted or poorly formatted.
Lastly, it’s worth noting an interesting perspective from Linus Torvalds, creator of Linux and Git, who said “Given enough eyeballs, all bugs are shallow”. So always try to revisit your code, iteratively, until you flush out where exactly the problem lies.
Diving into Debugging Strategies for Maven Failures
For developers, encountering Maven compilation failure isn’t a rarity. Sometimes while working with Maven, one might encounter the compilation error “
Failed to execute goal org.apache.maven.plugins:maven-compiler-plugin:3.10.1:compile
“. Understanding how to tackle these Maven failures effectively is as crucial as being able to write code in isolation.
The first and foremost thing to do when you encounter this kind of problem is to check your
pom.xml
file. It could be that the
maven-compiler-plugin
hasn’t been explicitly defined here, resulting in Maven making assumptions based on its defaults which may not match your environment requirements.
Here’s an example of how to specify the maven compiler plugin in your
pom.xml
:
…
In the context of your question, another troubleshooting approach is looking into the compatibility of your Java versions with the
maven-compiler-plugin
. The 3.10.1 version of this plugin doesn’t exist, so it might be a typo. The latest version available (as of November 2021) is 3.8.0.
Ultimately, understanding that debugging is a process of elimination often leads us to resolution. As Brian Kernighan, a pioneer in the field of software development once said, “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” Its significance cannot be understated.
It further underlines the point that creating an environment internally that allows for ease of debugging can go a long way in helping resolve issues such as these. Periodic code reviews, thorough testing, and maintaining clean and understandable project structure all contribute towards easier debugging and ultimately smoother development experience.
Remember, each Maven error can be solved step-by-step. Don’t hesitate to look into the online [Maven Documentation](https://maven.apache.org/guides/index.html) or [Maven Compiler Plugin documentation](https://maven.apache.org/plugins/maven-compiler-plugin/) for exploring more information regarding potential solutions. Surrounding yourself with resources such as stack overflow, relevant blogs, and fellow developer insights, upon facing any coding challenges, will only create avenues for successful debbuging.
Effective Solutions to Overcome Apache Maven’s Compilation Issues
Overcoming Apache Maven’s compilation issues, particularly those related to the Maven-Compiler-Plugin3.10.1Compile goal, can often be difficult. However, there are a few effective solutions to these problems that commonly work:
– Updating Maven: Often, Maven Compiler Plugin errors can be resolved by running an update. A new version of Maven may have the necessary patches or features to solve these issues. You can easily update Maven by downloading the latest version from the official Maven site.
– Checking JAVA_HOME variables: Incorrectly set JAVA_HOME variables can also cause execution failure. You need to make sure that your JAVA_HOME environment variable is correctly set and aligned with the JDK that the Maven Compiler Plugin is using.
Example:
export JAVA_HOME=/usr/lib/jvm/java-8-openjdk
– Adjusting the POM.xml file: You might find some incorrectly configured parameters in the POM.xml file. Namely, it’s recommended to verify whether the plugin declaration is accurate or not. It must reflect the appropriate version, like 3.10.1 for example, in the `
Example:
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.10.1</version> </plugin> </plugins> </build>
– Selecting Correct Dependencies: Make sure all the dependencies you included in the POM.xml file are correct. Incompatible or incorrect dependencies can lead to Maven Compilation failure.
_Larry Wall, the creator of Perl programming language, once said, “The three chief virtues of a programmer are: Laziness, Impatience and Hubris.”_ This quote emphasizes the importance and value of efficient coding practices, which include effective troubleshooting methods. Handling Maven’s compilation issues indeed demands patience and attention, but overcoming them allows developers to return back to what they do best – creating quality software.
Insightful Tips to Optimize Your Use of Org.Apache.Maven.Plugins
Optimizing the use of `org.apache.maven.plugins`, more specifically the `maven-compiler-plugin` error, involves a multidimensional approach focusing on different aspects. The objective is to maximize efficiency and address any errors, such as “Maven Failed To Execute Goal Org.Apache.Maven.Plugins:maven-Compiler-Plugin:3.10.1:Compile”.
Insightful Tips | Description |
---|---|
Check POM File Settings | Your Project Object Model (POM) file should correctly define the maven-compiler-plugin with the correct version (
<version>3.10.1</version> ) under plugins. |
Analyze JDK Version Compatibility | Verify if your Java Development Kit (JDK) version aligns with the requirements of the plugin version you are using. For instance, JDK 1.8 may be suitable for maven-compiler-plugin:3.10.1. |
Ensure Correct Plugin Configuration | In your POM file, ensure that the source and target configurations align with your project’s Java version. For example:
<configuration> |
Addition of Dependencies | Missing dependencies can cause failure to execute goals. Ensure all dependencies related to the compilation are added in your POM file. |
Gauge Plugin Scope | Carefully consider the scope of the plugin. If it’s defined at parent level, child modules might inherit causing conflicts. |
As noted by Jason van Zyl, founder of Apache Maven, “You need reproducible builds… Apache Maven brings you that,” a reflection on how vital attaining optimal configuration is in ensuring proper functionality.
In case these tips don’t resolve the issue, other potential solutions could include checking for incompatible plugins or corrupt local repository. Additionally, stack trace analysis might provide clues about ambiguous or conflicting classes. Consult the official Maven Compiler Plugin documentation for an in-depth understanding.
If you’ve encountered a “Maven Failed To Execute Goal Org.Apache.Maven.Pluginsmaven-Compiler-Plugin3.10:1Compile” error, it is often an indication that there are possible complications during the compilation phase of your Maven build. Let’s explore some potential reasons behind this issue and how to resolve them.
<!-- An example of a problematic configuration --> <project> ... <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.10.1</version> ... </plugin> </plugins> </build> ... </project>
In relation to the “Maven Failed to Execute Goal” issue, a common theme is java versions problems or conflicts. Usually, these arise due to a mismatch between required Java version by the project and the version installed on your machine. You can verify and align this by specifying the correct JDK version in your
pom.xml
file like this:
<properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties>
Another common cause for this issue could be related to dependencies missing or being unreachable. Ensuring all dependencies have been defined correctly in your pom.xml and are accessible from the Maven repository usually resolves this problem.
Unresolved plugin versions can also lead to Maven struggling to achieve its compile goal. Try defining the version explicitly or using the latest version available.
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> </plugin>
Keep in mind that in most cases, refreshing/rebuilding your project after updating your POM.xml helps in resyncing everything back up when Maven struggles with any sort of goals.
Lastly, environment variables need to be properly set for maven to function optimally. Check your system’s JAVA_HOME, M2_HOME, and PATH settings.
To paraphrase Linus Torvalds on the matter, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” Understanding and overcoming errors, such as the one represented here, form part of this fun challenge inherent in programming.
As developers, we ought to remember that troubleshooting maven goals often requires a multifaceted approach – Java version alignment, dependency checks, explicit plugin versions, and proper environment settings. By keeping these aspects under control, goal execution within the Apache Maven framework should ultimately run smoother.