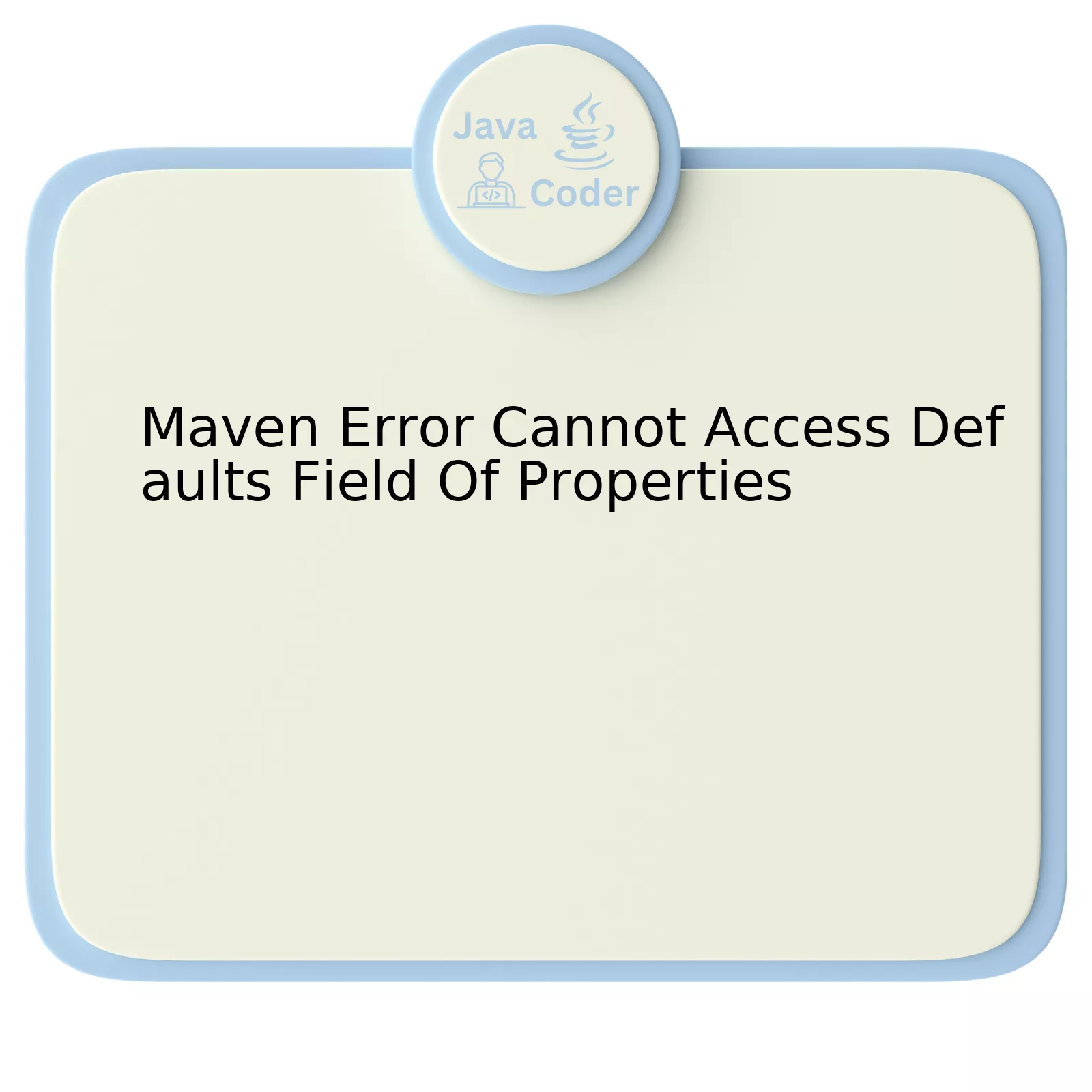
When looking at the issue pertaining to ‘Maven Error: Cannot Access Defaults Field of Properties’, we can understand it through a structured approach, presented as follows:
Error Issue | Common Cause | Proposed Solution |
---|---|---|
Maven Error: Cannot Access Defaults Field of Properties | Certain dependencies in your Maven POM file may not be correctly configured, or there might be an access problem due to version incompatibility between Java and Maven. | Review the configuration of your Maven POM file specifically focusing on properties. If possible, try to update your Java or Maven to a compatible version. |
As seen above, this error is typically caused when there is a mismatch between certain configurations of dependencies specified within your POM file in a Maven project, or there are compatibility issues between the linked Java and Maven versions. Maven, being a powerful project management tool used widely in Java development, has its complexities; and configuration errors like these are quite common.
To solve this issue, firstly you should go over your Project Object Model (POM) file, where you have declared your project’s dependencies, to make sure every component is correctly defined. Here’s a simple example of how a
pom.xml
looks like:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mycompany.app</groupId> <artifactId>my-app</artifactId> <version>1</version> </project>
Secondly, an update to the latest stable version of both Maven and Java would likely resolve any version compatibility problems restricting access to default fields of properties. Remember to verify that the updated versions are mutually compatible, as specific versions of Java work best with corresponding releases of Maven.
As Linus Torvalds, creator of Linux and Git, once put thus: “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” So, don’t let such errors deter you from the joy of coding. Keep exploring and digging deeper into concepts to solve them swiftly!
Lastly, while solving the problem, consider referring to leading online platforms for developers to draw alternative solutions and learn from others’ experience handling similar issues. Remember, every error encountered is just another opportunity to enhance your knowledge base.
Understanding the Maven Error: Cannot Access Defaults Field of Properties
The Maven error, “Cannot Access Defaults Field of Properties”, is typically encountered when you’re working on a Java application using certain versions of Apache Maven, especially older ones. This error signals that there’s a problem accessing default fields from the properties file.
To understand this error and its causes, we’ll review the following aspects: the role of Maven in Java development, the concept of defaults in properties files, and finally, common strategies to resolve the error.
- Understanding the Role of Maven in Java Development
Apache Maven is a powerful project management tool used by Java developers. Maven simplifies project builds, ensures consistency across multiple projects, and aids dependency management. It uses an XML file—pom.xml—for configuration, describing the project structure, dependencies, plugins, goals, etc. However, mismatches between system configurations and prespecified build settings can lead to errors like “Cannot Access Defaults Field of Properties”.
What does ‘Cannot Access Defaults Field of Properties’ mean? |
---|
maven-error |
For instance, this error might crop up if your system is running a version of Java that’s incompatible with the version Maven was built with, making access to default property fields unsuccessful.
- Deciphering Defaults in Properties files
In Java, properties files are mainly used for storing configurable parameters of an application, including default settings. These can be modified without altering the core codebase; instead, any changes take effect when the application restarts.
When Maven encounters the “Cannot Access Defaults Field of Properties” error, it informs you that while trying to read these default settings from your properties file, it came across issues—in other words, it couldn’t access the fields designated as ‘default.’
- Addressing the Error: Cannot Access Defaults Field of Properties
Here are several potential solutions:
- Review your pom.xml file configuration – Often, minor misconfigurations in the pom.xml file can cause this error.
<build> <finalName>${project.artifactId}</finalName> </build>
Ensure that any properties used in pom.xml are correctly defined.
- Verify your Maven and JDK Version – Make sure that your Maven version is compatible with the JDK version installed on your system.
- Update Artifact Versions in pom.xml – If some of your project’s dependencies have been updated recently, ensure that their newer versions are reflected correctly in your pom.xml file. If not, update accordingly.
In the words of Edsger Dijkstra, a luminary in the field of computer science- “If debugging is the process of removing software bugs, then programming must be the process of putting them in.” In spite of all its benefits, Maven, much like any software tool, isn’t devoid of occasional glitches. But with an understanding of its inner workings- as well as its potential hiccups- you’re much better equipped to resolve them promptly. Just remember that most errors, including “Cannot Access Defaults Field of Properties”, are usually due to some sort of mismatch- in configuration, in versions, etc. Your first point of investigation should always be identifying what this inconsistency might be.
Solutions for the Maven Error on Property Access
The Maven error “Cannot Access Defaults Field Of Properties” is a relatively common issue seen in Java development, largely due to misconfigurations or incorrect set-up of your Maven project. To navigate around this difficulty, several steps are required.
Let’s first take a closer look at why this error might be occurring:
- Incorrect POM (Project Object Model) File Configuration: This problem can arise from a wrongly constructed POM file. It’s pertinent to remember that Maven is highly dependent on the accuracy of the configuration in the pom.xml files.
- Mismatched Versions: An inconsistency between the Maven version and the Java compiler version can result in such an error. It’s critical to use compatible versions to ensure seamless operation.
- Inadequate Access Privileges: The lack of sufficient access permissions to the directories can cause this issue. It’s crucial to have the necessary access privileges to facilitate uninterrupted and smooth project building.
Now, delving into a few potential solutions for this error:
- Revise the POM.xml File: Double-check your pom.xml file to ensure there are no errors or omissions. This can range from verifying the syntax to assuring that all dependencies and plugins are correctly declared. For example:
<project> ... <dependencies> <dependency> ... </dependency> </dependencies> ... </project>
- Maven and Compiler Version Compatibility: Ensure that you’re employing a Java compiler version that’s compatible with your version of Maven. Remember, often Maven won’t work properly if you’re using an incompatible Java compiler.
An evergreen piece of advice by Edsger W. Dijkstra is: “If you want more effective programmers, you will discover that they should not waste their time debugging, they should not introduce the bugs to start with.” This rings particularly true in this case. In the software world, ensuring compatibility from the onset saves much time and effort down the road. - Review Access Permissions: Make sure the account running Maven has full access permissions to the necessary directories and files. You might need to modify the access control settings or run the process as an administrator if it’s being blocked because of insufficient permissions.
Lastly, don’t forget to clean and build your project post making these adjustments. Utilize the Maven Clean plugin to clean your project before re-building it. Running
mvn clean install
might resolve your issue, assuming one of the aforementioned aspects were causing the problem.
For additional insights, consider referring to various comprehensive online forums such as StackOverflow, official documentation such as Maven Dependency Mechanism, and other digital resources at your disposal.
Errors in Code: How they Lead to Maven Inaccessibility Issue
In understanding how errors in code contribute to Maven inaccessibility issues, particularly the “Maven Error: Cannot Access Defaults Field of Properties,” we need a comprehensive understanding of what Maven is, its expected behavior, and what happens when an anomaly like an error occurs.
Aspect | Description |
---|---|
What is Maven? | Maven is a powerful project management tool primarily used in Java programming. It facilitates build automation, dependency management, and project setups.(source) |
Role in Error Handling | Maven helps identify, isolate and hence resolve dependencies which can potentially be sources of bugs or unforeseen errors in a program. |
Errors in code, termed as “bugs,” are unexpected behaviors that obstruct normal program execution. In the context of Maven, these unexpected behaviors may lead to certain components becoming inaccessible. For instance, when an uninitialized field is accessed, this leads to the “Cannot Access Defaults Field of Properties” Maven error.
Diving Deeper: The Maven Error
This error typically arises from improper use of the defaults field in the properties file. Erroneous manipulations within the properties file might distort the instructions sent to the Maven Project Object Model (POM), resulting in this error.
For example,
//Incorrect code Properties prop = new Properties(); prop.load(new FileInputStream("config.properties")); String property1=defaults.getProperty("Property1");
To correct this error and ensure Maven accessibility isn’t compromised, one needs to correctly access the defaults field as shown,
//Correct code Properties prop = new Properties(); prop.load(new FileInputStream("config.properties")); String property1=prop.getProperty("Property1");
The inability to access the defaults field often results from either a lack of knowledge of Maven’s structure or simple spelling mistakes. To prevent such “Cannot Access Default Field of Properties” errors and subsequently foster Maven accessibility:
- Consistency and correctness: Ensure there’s no divergence from Maven’s expected format while constructing the `properties` field. Typos or syntax irregularities can result in inadvertent errors.
- Parameter definition: Always define parameters that are intended for use in the properties files. Hibernate’s validation feature can be an excellent solution for parameter validation.
- Error checking: Regularly perform tests to encounter and fix any error before it escalates to a fault. Automated testing tools, like JUnit and TestNG, integrated with Maven can prove useful.
As Kent Beck said, “Make it work, make it right, make it fast.” No code is without bugs or errors, but diligent debugging, meaningful error handling mechanism, and appropriate practices can significantly mitigate their impacts, keeping Maven accessible and functional.
Troubleshooting and Avoiding Future Occurrences of The Maven Error
The issue of “Maven Error Cannot Access Defaults Field Of Properties” can be quite a setback for Java developers, especially those predominantly using Maven as their project management tool. This is often associated with problems related to the properties file and its access permissions.
Troubleshooting this kind of error involves several systematic steps. Firstly, you should confirm that the Maven version being used supports the Java version in your environment. They should be compatible to prevent conflicts that might trigger errors. For instance, Maven 3.3 requires at least Java 7 or higher.
mvn --version
You’ll receive an output indicating your Maven and Java versions. If these are incompatible, upgrade or downgrade one of them accordingly.
Secondly, check the
pom.xml
file to ensure it’s correctly structured without any syntax errors. The properties field should be appropriately defined. A typical structure should look like:
<project> <properties> ... </properties> ... </project>
Validate the XML file online using available free tools like XML Validation Tool.
Finally – and this step deals directly with the error message – ascertain that the “defaults” field in the properties file is accessible. This might involve ensuring appropriate read/write permissions are set on the properties file itself. You could use standard operating system commands like chmod (for Unix-like systems) or icacls (for Windows).
To avoid future occurrences of this error, consider the following practices:
– Regularly update both your Maven and Java installations to their latest stable versions.
– Use modern Integrated Development Environment (IDE) tools which usually come with built-in syntax highlighting and formatting features for XML files.
– Adopt sound file permission management strategies to ensure important files retain their necessary access rights.
As Tim Berners-Lee, the father of the World Wide Web once said, “We need diversity of thought in the world to face the new challenges.” In the same way, exploring diverse troubleshooting methods and proactive habits will ensure a more streamlined and productive coding experience when dealing with Maven projects.
Maven, a powerful tool utilized widely in the Java industry, occasionally encounters issues that leave developers puzzled. One such issue is the error: “Cannot Access Defaults Field of Properties.” This pertains to Maven’s inability to access the default fields of properties.
Error | “Cannot Access Defaults Field of Properties” |
Assessment | Maven’s struggle to access the default fields of properties. |
It’s crucial to note that any instance where Maven fails to function as expected is generally due to configuration errors. Misconfigured settings.xml or pom.xml files are likely culprits behind this incidious error notice.
<settings xmlns="http://maven.apache.org/SETTINGS/1.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/SETTINGS/1.0.0 https://maven.apache.org/xsd/settings-1.0.0.xsd"> ... <activeProfiles> <activeProfile>myprofile</activeProfile> </activeProfiles> ... </settings>
This error often arises when an attempt is made to access a property that isn’t defined or instantiated – hence defaults not being reachable. In most cases, rectifying these discrepancies by ensuring careful adherence to accepted standards for setting up projects in Maven can help eliminate this error.
A hands-on approach may involve redoing the configuration from scratch to weed out accumulated misconfigurations that tend to go unnoticed over time; however, a more cautious approach would be to methodologically check each line of your configuration files for potential oversights, as mentioned by James Gosling, the Father of Java: “Always code as if the guy who ends up maintaining your code will be a violent psychopath who knows where you live.”
For further guidance, it’s advisable to consult official documentation on Maven’s website and well-trusted online resources like StackOverflow. These sources hold wealths of knowledge and insights from experienced developers tackling similar issues, making them invaluable allies in your quest for problem-solving.
Remember, while facing errors can be frustrating, each one offers a unique opportunity to learn and refine skills that ultimately mold us into better programmers.