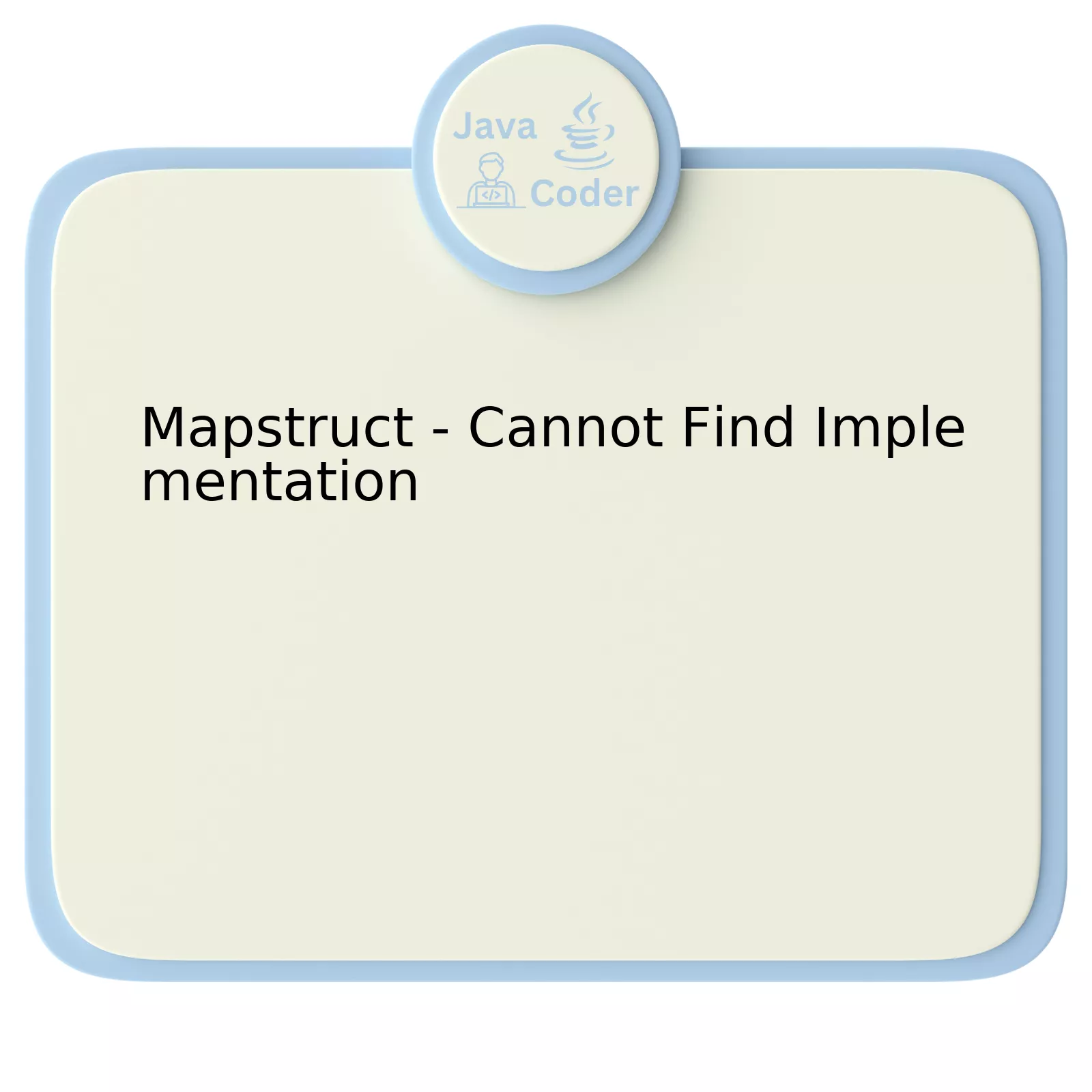
Mapstruct | An open-source code generation tool streamlining the implementation of mappings between Java bean types. |
Implementation Issue | Error or problem that arises when the system fails to locate the generated Mapper implementation class during the project’s compilation phase. |
MapStruct is essentially a code generator that substantially eases the process of implementing mappings between Java bean types which, in real-world application development, are often monotonous and error-prone. The basic concept is to automate the transfer of data between objects (mapping), especially when dealing with similar structural classes, thereby reducing boilerplate coding. MapStruct will – at compile time -generate an implementation based on method signatures in interface or abstract class.
Possible issues in the “implementation not found” scenario occur, predominantly, when the system, for various reasons, cannot find the Mapper implementation that was supposed to be automatically generated during the build stage. This issue might manifest if there’s a project configuration problem or if the build tool isn’t appropriately configured. Also, it’s common when the classpath lacks certain dependencies required for automatic Mapper creation, or simply if MapStruct isn’t properly integrated within the project’s build lifecycle.
To overcome this, ensuring the right setup is crucial:
- Firstly, always verify your build setup; Maven or Gradle should be correctly installed, and its respective plugin for MapStruct configured as per the official documentation.
- Secondly, double-check the classpath details where the Mappers are supposed to be located, and confirm those match with where the system is searching.
- Furthermore, be proactive about managing and updating dependencies in your project configuration file (such as pom.xml in Maven). For instance, ensure to include MapStruct’s processor package
- Lastly, since MapStruct has different versions, compatibility could play a role. Always make sure you’re using corresponding compatible versions of both, MapStruct and your chosen build tool.
For example:
Your Maven/Gradle configuration may look like this:
<dependencies>
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct</artifactId>
<version>1.4.2.Final</version>
</dependency>
<!-- if using Spring ... -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
</dependencies>
As Bill Gates once said, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency.” Herein, MapStruct exemplifies this maxim by automating the ‘mapping’ task for improved programming efficiency.
Unpacking the “Mapstruct – Cannot Find Implementation” Error
Unraveling the “MapStruct – Cannot Find Implementation” error requires an understanding of MapStruct’s fundamental operations. Primarily, MapStruct is a code generator simplifying data mapping between Java beans. It does this by generating Mapper classes during compile time. However, instances when it can’t locate these Mapper implementations trigger the “Cannot Find Implementation” error.
The Root Cause: Annotations and Configuration
Before delving into possible solutions, pinpointing the root causes of the ‘MapStruct – Cannot Find Implementation’ error is instrumental.
-
@Mapper
Annotation Absence – MapStruct uses the
@Mapper
annotation to generate mapper implementation at compile time. If no class carries this annotation, MapStruct won’t generate the required implementation triggering the error.
- Maven Configuration – Sometimes, your Maven not properly configured can cause this error.
Potential Solutions
Strategies for resolving this issue include:
Implement the
@Mapper
Annotation
In your Mapper Interface, implement the
@Mapper
annotation. This directs MapStruct to enact an implementation of this interface.
For instance,
@Mapper public interface CarsMapper { CarsMapper INSTANCE = Mappers.getMapper( CarsMapper.class ); @Mapping(source = "numberOfSeats", target = "seatCount") CarDto carToCarDto(Car car); }
You’re cuing MapStruct to generate a Mapper implementation named “CarsMapperImpl”.
Include the
mapstruct-processor
within your Maven Plugins
A common cause of this issue is incorrect or inadequate Maven configuration. Steps to resolution are:
- Incorrect or missing annotations
- Inappropriate build configuration
- Ambiguous mapping methods
- Detection of potential runtime errors during compilation
-
Include
mapstruct-processor
in the dependency module.
Include
mapstruct-processor
in the plugins module.
Worked Example:
<dependencies> <dependency> <groupId>org.mapstruct</groupId> <artifactId>mapstruct</artifactId> <version>${org.mapstruct.version}</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.8</source> <target>1.8</target> <annotationProcessorPaths> <path> <groupId>org.mapstruct</groupId> <artifactId>mapstruct-processor</artifactId> <version>${org.mapstruct.version}</version> </path> </annotationProcessorPaths> </configuration> </plugin> </plugins> </build>
However, per software engineer Rebecca Parsons, keeping your code clean by regularly testing and fixing bugs increases the ease of maintaining it, so keep these practices in check to avoid such errors.
Troubleshooting Techniques for Mapstruct’s Missing Implementation Issue
Mapstruct is a powerful tool for data mapping, but developers can sometimes encounter the “Cannot Find Implementation” issue. This denotes that Mapstruct cannot locate an implementation for the mapper interface declared. Generally, this can be linked to configuration or setup problems within Mapstruct and your Java environment. Below are outlined some techniques that should assist in troubleshooting this problem:
1. Confirm if Annotation Processing is Enabled
Mapstruct operates closely with annotation processing. If the library fails to discover the implementation, there might be a chance that this feature has been turned off in your IDE. To rectify this, turn it on in your settings:
// IntelliJ IDEA: // File → Settings (Ctrl + Alt + S) → Build, Execution, Deployment → Compiler → Annotation Processors // Ensure 'Enable annotation processing' is checked
This process varies slightly for different IDEs, but they most likely have similar options to toggle annotation processing.
2. Check your POM Configuration
Your Maven setup also plays a significant role in determining the implementation discovery of Mapstruct. When utilizing the Mapstruct processor, ensure it is correctly configured within your POM file:
<dependency> <groupId>org.mapstruct</groupId> <artifactId>mapstruct-processor</artifactId> <version>1.4.2.Final</version> <scope>provided</scope> </dependency>
3. Make Sure You’re Using the Right Version
In other circumstances, you might be dealing with compatibility issues. Various integration issues may arise from subtle differences between versions of Mapstruct and JDK, leading to failed implementations detections. Always ensure that you’re using the latest stable version of Mapstruct compatible with your JDK.
4. Rebuild Your Project
Sometimes the issue could be far less sophisticated – a simple solution might be to clean and rebuild your project. As much as it sounds trivial, cleaning out compiled classes and rebuilding the project has saved many developers countless hours of troubleshooting.
Stack Abuse provides in-depth discussion and various examples about troubleshooting common Mapstruct issues.
“Measuring programming progress by lines of code is like measuring aircraft building progress by weight.” – Bill Gates. Remember, every step towards finding a solution matters, no matter how small or seemingly insignificant. Happy coding!
Potential Solutions: Addressing “Cannot Find Implementation” in Mapstruct
When you encounter a “Cannot Find Implementation” error while using Mapstruct, it’s often because Mapstruct is unable to locate the implementation class for the mapper interface. This could be due to a number of factors. Let’s consider the most common scenarios and potential solutions:
1. No component model specified: MapStruct requires a defined component model in order to generate the correct implementation. For Spring-based applications, this is accomplished by annotating the mapper interface with
@Mapper(componentModel="spring")
.
2. Inappropriate scope: If your mapper classes are not in the same or sub-package as your main application class (In spring boot) or as your configuration class (Spring with manual configuration), MapStruct may not be able to identify them.
3. Misconfiguration during project compilation: The error message could also appear if your IDE (like Eclipse, IntelliJ, etc.) doesn’t invoke the annotation processor during compilation.
Let’s illustrate solution sketches to these scenarios.
If you’re dealing with an unspecified component model, simply add the appropriate model to your mapper interface. For instance, in Spring, your code would look something like:
@Mapper(componentModel="spring") public interface YourMapper{ ... }
Encountering an issue due to inappropriate scope? It’s advised to reorganize your code base to ensure mappers are accessible. Ideally, the mapper interface should be in the same package where your Application class exists or in a sub-package.
Lastly, if misconfiguration is at fault, adjusting the settings of your IDE can aid in resolving this issue. Within IntelliJ IDEA, navigate to ‘Build, Execution, Deployment -> Compiler -> Annotation Processors’ and enable annotation processing.
In cases revolving around Eclipse, you’ll need to include MapStruct as a dependency in your Maven or Gradle configuration. Additionally, incorporate the respective plugin in your pom.xml file. Thereafter, rebuild your project and let the autobuild feature handle the rest.
Remember, as Sergey Makarenko once stated, “Programming isn’t about the language you use, it’s about the solutions you’re able to put together”. Don’t feel disheartened when met with errors – they’re just stepping stones towards becoming a better developer.
These measures should help you effectively troubleshoot and resolve the “Cannot Find Implementation” issue seen in Mapstruct, fostering smoother development experience always.
Case Study Analysis: Resolving “Cannot Find Implementation” Error in Mapstruct
The “Cannot Find Implementation” error in Mapstruct is more frequent than we’d like. The issue arises when the application fails to locate the mapper implementation at compile time, crippling your pursuit of efficient object translation.
MapStruct relies on annotations during compilation to generate implementation classes for defined interfaces or abstract classes. Any hiccups in this process result in a ‘Cannot Resolve Symbol’ or ‘Cannot Find Implementation’ error.
Please find below key reasons responsible for this scenario:
“Make it work, make it right, make it fast.” – Kent Beck
1. Incorrect Configuration in IDE
Improper setup in IntelliJ or Eclipse could hinder the auto-generation of implementation during build time. For instance, you may face issues if the annotation processor is not enabled in IntelliJ IDEA configuration settings, or the ‘org.mapstruct:mapstruct-processor’ dependency is missing.
2. Erroneous Annotations
Failure to correctly annotate the interface/abstract class or properties can disrupt MapStruct’s functionality. Pay special attention to @Mapper or @MapperComponentModel annotations as these are critical to MapStruct’s success.
An example of the correct use of the @Mapper annotation is shown below:
@Mapper public interface EmployeeMapper { EmployeeDto employeeToEmployeeDto(Employee employee); }
3. Incompatible Methods
MapStruct might struggle with generating implementation if the source and target methods have incompatible return types or parameters. A best practice is to ensure that for every source field, there exists an equivalently named field in the target class.
Solutions
Understanding the potential pitfalls helps in formulating effective solutions. Let’s look at strategies available to rectify this problem:
1. Verify and Correct the Configuration
Check your IDE settings to ascertain annotation processing is on, and the required dependencies are present.
For IntelliJ IDEA, enable ‘Annotation Processing’ in configuration settings and add the ‘mapstruct-processor’ dependency:
\
…
\
\
\
\
\
…
\
2. Add Missing or Correct All Essential Annotations
Ensure that the Mapper interfaces or abstract classes have the @Mapper annotation. Properties should have apt annotations such as @Mapping, @Mappings etc.
3. Sync Method Parameters and Return Types
Both source and target properties need identical namings for proper mapping. If necessary, override the existing naming strategy completely or on a per-field basis by using the @Mapping annotation.
@Mapper public interface EmployeeMapper { @Mapping(source = "fullName", target = "name") EmployeeDto employeeToEmployeeDto(Employee employee); }
Remember, with the right configurations, proper annotations and compatible methods, you can effectively leverage MapStruct’s capability to simplify translations between objects. Keep calm and let MapStruct map.
The “Cannot Find Implementation” error is among the commonly encountered issues in working with MapStruct, a type-checking library for Java programs.
MapStruct operates by generating implementation classes of mapper interfaces during the compile time. Primarily, the cause of the “Cannot Find Implementation” problem can be traced back to one of four scenarios:
In the context of
@Mapper
or
@MapperComponentModel
annotations (in situations where inadequate or incorrectly formed annotations are used), it may lead to an issue where generated implementation classes fail to be identified at a later stage of the coding process.
Substantial intricacies may likewise be related to a developer’s build setup. For instance, you need to assure that the compiler plugin is accurately declared within your Maven or Gradle configuration, and the annotation processor path is defined correctly too. This oversight might potentially hinder MapStruct from carrying out necessary operations throughout the compilation phase.
Similarly, ambiguous mapping methods could also provoke these issues. In other instances where several eligible mapping methods are present, some form of conflict may arise consequently causing problems identifying the correct implementation class.
Lastly, but certainly significant, if MapStruct encounters conditions which it deliberates could give rise to runtime errors, it will refuse to generate the implementation class – triggering the error ‘cannot find implementation’.
To effectively handle this obstacle, developers need to further explore and understand the root cause of such error messages. Some hints could be obtained from examining the stack trace in detail, checking mapper interfaces, validating project configurations, or resorting to comprehensive debugging methods.
Remember that Java errors like these aren’t necessarily detrimental – they often serve as valuable feedback mechanisms that help us improve our coding skills. As Martin Fowler eloquently said: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Be that good programmer, constantly learning by untying knotted lines of codes.
Reference: MapStruct Official Documentation