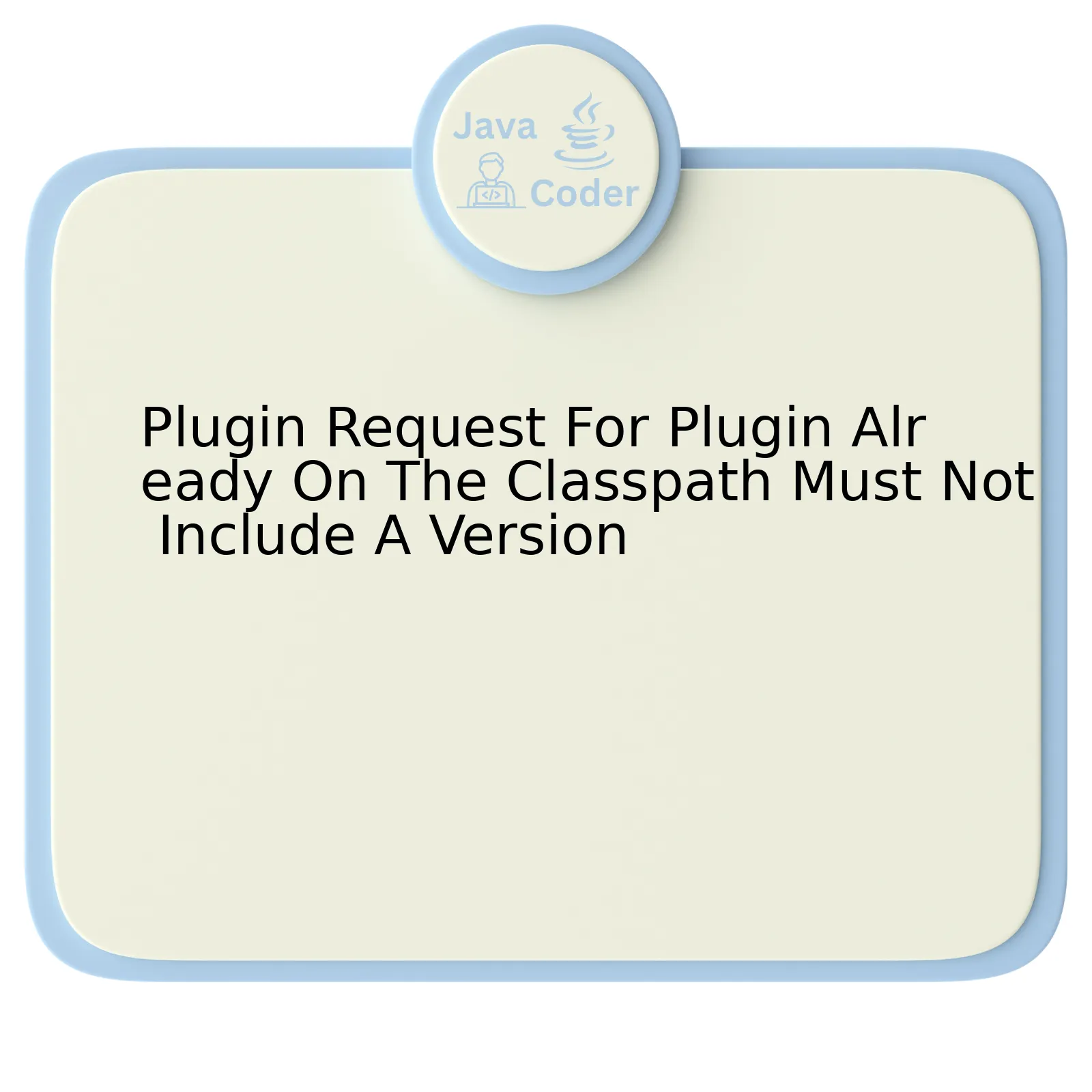
Key Terms | Explanation |
---|---|
Plugin Request | In the context of Java programming, a plugin request is a call made to an external software component – in other words, a plugin – to perform specific functionalities. These plugins enhance or extend your application’s capabilities without altering the main program. |
Plugin on Classpath | A plugin resides ‘on the classpath’ if it has been included in your project’s build path. This informs the Java Virtual Machine (JVM) where to locate and load required classes and resources from. |
No Version | When a plugin is on the classpath, it must not include a version in the specification. The reason is that the project’s build system, such as Maven or Gradle, dynamically resolves the plugin version based on what is available on the classpath. Explicitly providing a version can lead to conflicts and inconsistencies. |
To further understand this concept, consider the scenario where multiple versions of the same plugin reside on the classpath. If you specify a particular version for the plugin request, it could create havoc during the compile or runtime phases of the project.
An instance with the Gradle plugin setup can be a perfect example. Here’s how you typically declare a plugin in your
build.gradle
file:
plugins { id 'com.example.plugin' }
The above declaration does not include a version because Gradle will resolve the plugin version automatically based on what’s available on the classpath. On specifying a version, the build tool could fail when encountering different conflicting versions.
Remember:
“JVM does not care about what version of bytecode it runs, nor from where it was loaded.” – James Gosling, creator of Java.
This explains why Plugin Request For Plugin Already On The Classpath Must Not Include A Version. It gears towards maintaining consistency and reducing potential conflicts, thus providing a smooth, functional development environment.
Understanding The Concept: Plugins on the Classpath
To delve into the topic of understanding plugins on the classpath, it’s critical to elaborate on how the Java Classpath works. The Java Classpath essentially serves as a parameter that informs the Java Virtual Machine (JVM) and other Java applications where to hunt for user-defined classes. (source). It loads the necessary classes and libraries to ensure your code is executed correctly.
Role of Plugins on the Classpath
Relevant plugins in Java development generally refer to libraries or tools that are used to carry out specific functions or tasks. They can range from those designed for unit testing such as Junit, code coverage tools like Cobertura, to build tools such as Maven or Gradle. These plugins enhance application functionality without interfering with the base application logic.
The mechanism that allows plugins to be recognized and utilized by the JVM is the classpath. When a plugin resides in the classpath, it becomes readily available to your Java code.
Plugins present an interesting dimension to the way dependency management in Java works. With plugins on the classpath:
- One could run different versions of the same library without any conflicts.
- The widespread ‘Jar Hell’ problem common in Java development is notably alleviated.
- Ease of swapping implementations at runtime is possible thanks to dependency inversion.
Concern About Plugin Request For Plugin Already On The Classpath Must Not Include A Version
When a plugin is found on the classpath, it implies that the JVM is already aware of its existence and is capable of using said plugin when required. Including a version number during a subsequent request for the same plugin may result in unintended behavior. This is especially true when the specified version number does not correspond to the one currently existing on the classpath.
Why might there be concerns about requesting a “versioned” plugin that’s already on the classpath? Here are a few reasons:
- Potential for Conflicts: Different versions of the same plugin might have variations in class definitions and methods, which might cause conflicts during runtime.
- Unnecessary Redundancy: Requesting a particular version of a plugin already on the classpath could lead to unnecessary duplications on the classpath, consuming system resources.
- Scope of Errors: If the requested version doesn’t match the classpath version, the risk of NoSuchMethod errors or ClassDefNotFound errors can increase.
To circumvent this, the best practice would be to avoid specifying version numbers for plugins that are already existent on the classpath. In other words, once a plugin is on the classpath, future requests for the same plugin need to be generic, not version-specific.
In the words of Bill Gates – “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” (source) This quote resonates well with this concept; the smoother we can make these processes, the more seamless and efficient our coding lives become!
Avoiding Version Inclusion in Plugin Request
Managing Dependency Resolutions With Maven
Maven, an essential tool in Java Development, excels in project management and understanding software dependencies. When tackling codebases that necessitate multiple interdependent software components, Maven comes into its own for efficient package handling and implementing build automation.
However, one pertinent issue that developers often come across while utilizing Maven is the inclusion of version details in plugin requests, even when a plugin is already on the classpath.
Avoiding Version Details In Plugin Requests
Your typical Maven plugin request includes three components:
<groupId>
,
<artifactId>
, and
<version>
. The last component can prove unnecessary when the plugin mentioned is already existing on the classpath. Ideally, if a plugin already exists on the classpath, there shouldn’t be any need to include a version during its request.
A little insight into Maven’s dependency resolution would highlight why this is an area where efficiency can be improved. Whenever a project references a dependency without specifying a correct version, Maven makes repeated calls to the metadata to determine the right version of the particular artifact, hence leading to slow builds or runtime issues if resolved to incompatible versions.
Optimizing your workflow by excluding the version information in the plugin request enhances Maven’s efficiency in such cases because it wouldn’t have to make additional calls. This functionality aids in maintaining cleaner code, reducing redundancy, and streamlined dependency management.
To avoid version details in plugin requests, ensure to declare the plugin version in the
<pluginManagement>
section of the parent POM(object model). Here, you define the version along with other information in Parent `POM.xml`.
Here’s how it looks:
<pluginManagement> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> </plugin> </plugins> </pluginManagement>
With this arrangement, any reference to the maven-compiler-plugin in subsequent POMs won’t require a specific version as long as they’re within the same hierarchal structure next level.
As Kent Beck, famous for creating extreme programming, once said, “I’m not a great programmer; I’m just a good programmer with great habits.” These beneficial practices of avoiding redundant information in your Maven plugin requests fall under those ‘great habits’ every developer should aspire to nurture.
How to Correctly Request for Plugins Already On Classpath
If you’re using Maven, it’s important to ensure that your plugins are correctly requested from the classpath. In some situations, you might find that a plugin is already available on the classpath. In such cases, you should not include a version when making your request.
Let’s delve deeper into how this can be done:
Maven: Usage of Plugins Without Specifying Version
In situations where the plugin is already on the classpath, it is advisable to omit the version as it will help in avoiding conflicts. A typical situation is when we have an enterprise-level project with multiple child modules. Each module may depend on different versions of the same plugin making version management a cumbersome activity.
“With a good IDE, this discrepancy can be easily managed … provided you leave out version numbers for all plugins in your POM files.” — Daryl Marsden
com.example my-plugin provided
Inheritance and The Maven Super POM
The Maven Super POM is essential to this solution as it contains plugin definitions that are inherited by default. Because these plugins are already defined, they can be executed without specifying their respective versions.
“It is generally accepted that Maven’s system of inheritance and its Super POM can help to scrub a lot of the dirty work … out of your project setup and build phase.” — Kay Ewbank
The Pitfalls of Not Providing a Version
However, it is essential to keep in mind the pitfalls of not providing a version:
- Dependency issues: Not specifying a version could potentially lead to dependency-related errors.
- Build Reproducibility: For build reproducibility, it is often recommended to lock down specific versions of dependencies and plugins alike.
When used judiciously, leaving out the version number can be handy during drafting or prototyping stages. For more robust solutions, it is advised to specify the exact versions of your dependencies and plugins. You can read more about maven plugins in the official Maven documentation.
The Impact of Incorrect Plugin Requests on SEO Performance
The world of SEO (Search Engine Optimization) is closely intertwined with the specifications of your Java code, particularly when dealing with plugins. A recurring issue experienced by Java developers is having to deal with incorrect plugin requests which can significantly affect the overall performance of their SEO-optimized applications. This comes into play prominently in the context of the request for a plugin that is already on the classpath but includes an unnecessary version specification.
Dependency Conflicts due to Incorrect Plugin Requests
First off, making a request for a plugin that is already on the classpath can cause dependency conflicts. When this occurs, it often results in unpredictable behavior with components not functioning as expected.
“Every great developer you know got there by solving problems they were unqualified to solve until they did it.” – Patrick McKenzie
The versions of the plugin requested and the one on the classpath may differ, leading to the use of different APIs or methods within the same codebase. This could potentially create complications during runtime and disrupt the smooth operation of your website/platform, negatively affecting its SEO ranking as user experience remains a significant factor in optimization.
Duplication and Performance Degradation
In the same vein, requesting a plugin that’s already on the classpath but unnecessarily including the version could inadvertently lead to duplication. The system might erroneously import both versions, erroneously believing they are distinct because of the included version number. Given that search engines like Google penalize duplicate content, this scenario does not bode well for your platform’s SEO performance.
Moreover, performance degradation is another likely result. Ineffectively managing resources (maintaining two versions of the same plugin) can slow your site’s load time, alienating users and dropping your search rankings.
Proposed Solution: Correct Plugin Request Management
Addressing this issue is predominantly based upon correct management of plugin requests within your Java-based application. One strategy involves adopting the Maven Enforcer plugin [Maven Enforcer](https://maven.apache.org/enforcer/maven-enforcer-plugin/) to ensure the right version of each plugin is loaded, successfully identifying and combating any instances of incorrect plugin requests. As seen from the snippet below:
<project> ... <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-enforcer-plugin</artifactId> <version>3.0.0-M3</version> <executions> <execution> <id>enforce-no-requests</id> <goals> <goal>enforce</goal> </goals> <configuration> <rules> ... </rules> </configuration> </execution> </executions> </plugin> </plugins> </build> ... </project>
This implementation aims at enforcing rules for your project phases and aiding in steering clear from incorrect requests harming your SEO performance. It encourages the best practice of explicitly declaring plugin versions in your Project Object Model (POM) file, eliminating the risk of unexpected version updates disrupting your project’s stability. Thus, enhancing your site’s reliability and ease of use bolsters its favorable standing with search engines too.
Taking such measures ensures that conflicting or unnecessary versions do not sneak into the classpath, preserving SEO ranking, providing a more consistent user experience, and maintaining optimal performance.
.
In the context of software development, particularly Java, the topic revolves around the principle of “Plugin Request For Plugin Already On The Classpath Must Not Include A Version”. This principle is a key aspect of dependency management in Maven, a build automation tool used predominantly for Java projects.
The objective for developers when configuring plugins for their project’s classpath is to avoid specifying a version for plugins that originate from the same classpath. Here’s why:
Clarity and Consistency
When multiple versions of the same plugin exist on the classpath, it introduces an unnecessary level of complexity. By not specifying a version, it aids in maintaining clarity and consistency across the project, saving developers time and avoiding potential confusion.
Plugin Declaration with Version (Not recommended) | Plugin Declaration without Version (Recommended) |
---|---|
|
|
Avoiding Conflicts and Bugs
Problems can occur when a version is specified for a plugin already on the classpath. Multiple versions of a plugin might conflict with each other causing bugs or unexpected behavior. It’s simpler to default to the plugin version already established within the project environment.
File Size Optimization
Java projects, especially at enterprise level, may contain massive codebases and numerous dependencies. Specifying versions for every plugin could potentially increase the size of your project configuration files. Streamlining this process by excluding versions for the plugins on the classpath therefore contributes to file size optimization.
As a respected figure in the software industry, Robert C. Martin, colloquially known as “Uncle Bob”, states, “Clean code always looks like it was written by someone who cares.”
An important part of demonstrating this care is understanding and applying principles as seemingly niche as ensuring a plugin request for a plugin already on the classpath does not include a version. Details such as this, while they may appear minute, are critical in crafting projects that are not only high-performing, but also maintainable, consistent, and clean.