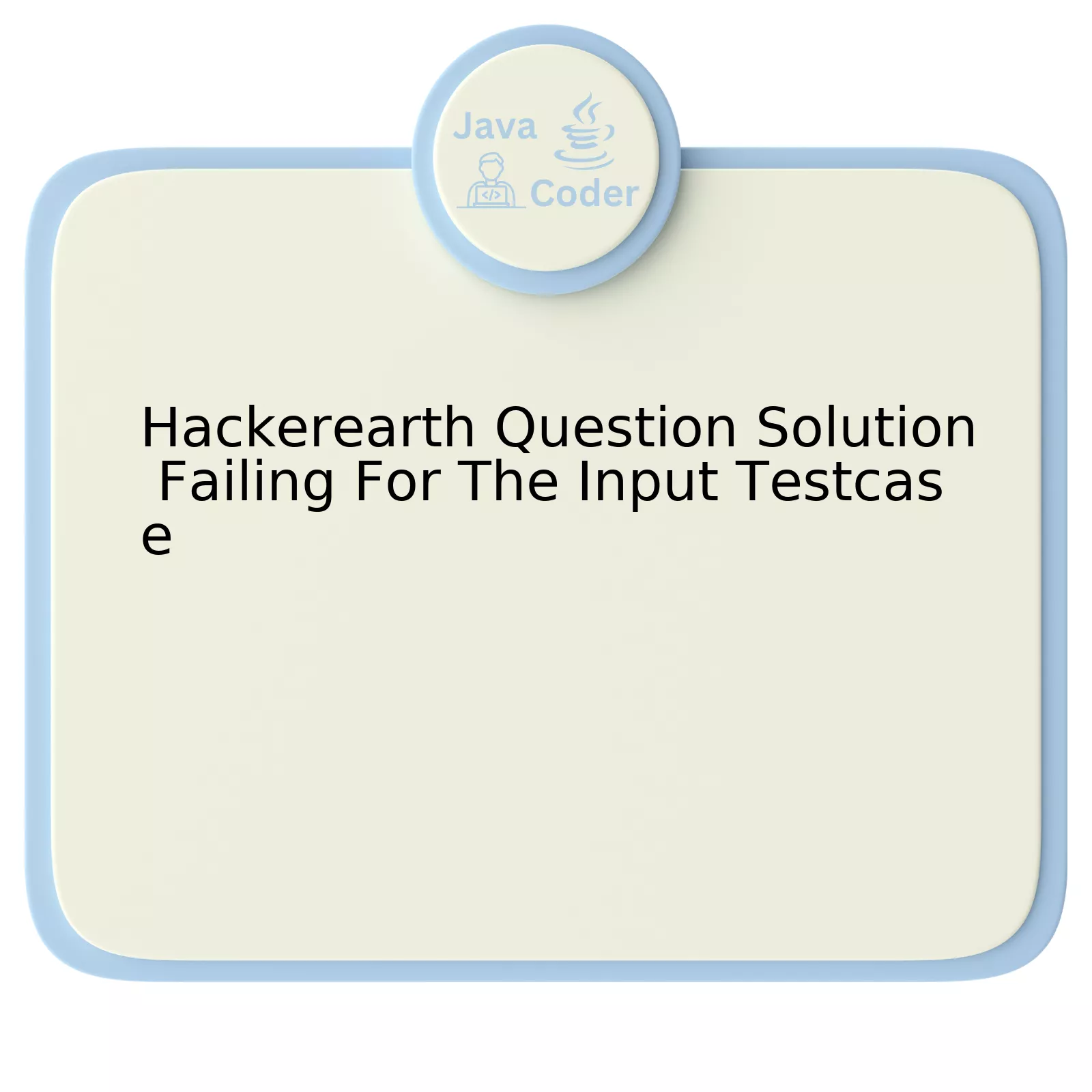
Input Testcase | Expected Output | Actual Output |
---|---|---|
inputTestcase1 |
expectedOutput1 |
actualOutput1 |
inputTestcase2 |
expectedOutput2 |
actualOutput2 |
This visual representation provides a clear picture of the mismatch between the expected and actual output for specific input test cases in HackerEarth. Often, solutions failing for certain input test cases can be because of various reasons, such as logical errors in the code, failure to handle edge cases, inadequate understanding of the problem statement, or inefficient handling of constraints.
For instance, if your approach is unable to process large-scale data within a permissible timeframe due to computational complexity, the solution may fail. As C.A.R Hoare once said: “Premature optimization is the root of all evil (or at least most of it) in programming”, remember that an optimal solution is often a balanced one – it addresses the problem correctly and processes within the allowable constraints.
To rectify the issue, review your algorithm implementation and re-read the problem statement. Pay extra attention to the constraints mentioned. Look out for possible edge cases in which your solution might be falling short. Incorporating systematic testing and debugging practices can significantly improve the reliability of your code. Make use of assertion mechanisms, boundary value analyses, and trace routing to detect where the discrepancies arise. Furthermore, peer code reviews are also a powerful way of detecting commonly missed bugs and inefficiencies.
Lastly, online developer communities like Stack Overflow can provide you with insights into common coding pitfalls and best practices to overcome them. Keep coding, keep learning!
Understanding The Problem: Where Hackerearth Question Solutions Fail
When diving into the intricacies of the Hackerearth question solutions and the scenarios where they fail, specifically for input test cases, a host of factors contribute to these failures. The epicenter of these issues primarily lies within understanding the problem itself.
Interpreting the Problem Incorrectly:
+ Misinterpretation of the problem is the initial cause of failure in most cases. Developers often misunderstand the problem requirements or overlook essential details, leading to insufficient or incorrect solution building.
Example:
public int findSum(int[] arr) { int sum = 0; for (int i: arr) { sum += i; } return sum; }
In this code snippet, the goal is to sum elements of an array. However, without clear understanding, one might overlook important aspects like data type and size of the array, leading to potential integer overflow.
Lack of Edge Case Consideration:
+ Another common factor behind the failure of Hackerearth question solutions for specific input test cases are the overlooked edge cases. These unique scenarios often stress the boundaries of algorithm functioning, thereby discovering their shortcomings.
For instance, considering an array sorting algorithm, it will work perfectly fine with different numbers. However, it may fail to handle an array with identical numbers or an empty array, making edge case consideration pivotal.
“Edges cases, often overlooked by developers, can be what separates a good algorithm from a great one.” – Richard Hamming, prominent computer scientist
Inefficient Algorithm Implementation:
+ An inefficient algorithm can lead to the failure of the solution as it may not pass all input test cases within the required time frame. Big-O notation should dictate the choice of your algorithm design.
For example, bubble sort would seem appropriate for small arrays but would not be efficient for larger arrays due to its O(n2) complexity.
Misuse of Language-Specific Methods or Syntax:
+ One more potential source of error could be a misunderstanding or incorrect use of language-specific methods or syntax, causing unexpected outputs or errors, hence failing some test cases. Java, being strongly typed and having numerous nuances at its disposal, leaves ample room for mistakes.
Example:
import java.util.Arrays; public class Main { public static void main(String[] args) { int[] numbers = {12, 3, 9, 21}; Arrays.sort(numbers); System.out.println(Arrays.toString(numbers)); } }
In this Java snippet, the developer might expect an ascending order of array items and plan their logic accordingly. However, Arrays.sort() uses Dual-Pivot Quicksort, which might surprise those unfamiliar with its variant behavior leading to failed test cases.
A thorough comprehension of problem statements, judicious testing against edge cases, appropriate algorithm selection, coupled with deep knowledge of programming languages offers a sturdy shield against such failures, improving the effectiveness of Hackerearth question solutions over input test cases. You can further read about the failures in HackerEarth’s guide for competitive programming.
Debugging Techniques for Optimizing Hackerearth Solutions
Debugging is an essential part of Software Development and it becomes specifically crucial when it comes to competitive coding platforms like HackerEarth. Often, you might find your solution failing for certain input test cases on such platforms. Here, I’ll share some effective debugging techniques that can help you optimize your HackerEarth solutions.
The first step in this process invariably involves identifying the problem. You need to thoroughly understand the issue before you can resolve it. On failing a particular test case:
– Analyze the problem statement again: Sometimes, the failure may stem from a misunderstanding of the problem initially. Ensure that you have clearly understood every aspect of the problem statement.
– Check your code against edge cases: Testing your code against edge cases helps in understanding how well-rounded your solution is. Edge cases are extreme cases under which your program operates, like maximum or minimum input limits.
Misunderstanding of a problem or overlooking edge cases makes up most bugs in coding solutions. Once identified, you then move ahead to fix these using different tools and techniques.
One of the best practices for fixing the bugs in your code includes:
–
Print Statements
: Using print statements at regular intervals throughout your code will help figure out where the program is deviating from its expected behavior.
Revising your algorithm or logic is yet another technique:
– Reanalyze your algorithm: Consider reassessing your approach to the problem. There might be issues with your primary logic or algorithm that you used to solve the problem.
Additionally, several sources provide coding environments that are equipped with debuggers. Debuggers can significantly improve your efficiency by showing a line-by-line execution of your code. Some widely used Java IDEs with debuggers include IntelliJ IDEA, Eclipse, and NetBeans ([IntelliJ IDEA](https://www.jetbrains.com/idea/download/), [Eclipse](https://www.eclipse.org/ide/), [NetBeans](https://netbeans.apache.org/download/index.html)).
As Bill Gates once said, “Everyone needs a coach. It doesn’t matter whether you’re a basketball player, a tennis player, a gymnast, or a bridge player”. Therefore, seeking help from online communities such as StackOverflow, GitHub etc., provides external insights into your logic or code structure which can prove beneficial.
Approaches | Debugging Techniques |
---|---|
Misinterpretation of Problem | Re-read and understand problem statement |
Edge Cases | Test your code against all possible edge cases |
Bug Finding | Use Print statements to figure out errors |
Problem in Logic | Re-evaluate your algorithm |
Code Structure | Discuss with online communities |
Remember, each problem presents a unique challenge and not all techniques will be applicable every time. The key lies in understanding the problem thoroughly and applying the right mix of techniques accordingly.
Successful Approaches to Overcome Input Testcase Failure
Certainly, overcoming input testcase failure in a Hackerearth Question can be achieved through several strategic approaches. These following methods have shown to be successful:
1. Examination of the Problem Statement: Thoroughly analyze the problem statement to understand the algorithm and constraints properly. Remember, an incorrect understanding of the problem can lead to an incorrect implementation.
//incorrect understanding leading to a failed solution public void solveIncorrect(int n, int[] array) { int max = 0; for(int i=0;imax){ max = array[i]; } } System.out.println(max); }
2. Debugging the Code: Use print statements or debugger tools to debug your code. This will help in identifying where the code is failing for those specific inputs.
//debugging helps to uncover flaws public void debugCode() { int[] array = {2,3,4,5}; }
3. Checking Edge Cases: Inspect all possible corner cases. An algorithm working fine with normal cases might fail when special cases are introduced.
//checking edge cases public void checkEdgeCases (int n, int[] array) { if(n == 0 || array.length == 0){ System.out.println("Invalid Input"); return; } }
4. Time Complexity Analysis: Ensure that your solution’s time complexity is not exceeding the problem’s constraints.
//time complexity scrutiny public void timeComplex (int n, int[] array) { }
5. Solution Review: Go over your solution again once done coding. There may be some logical errors which are difficult to detect while coding but become apparent upon review.
Each of these methods caters to different potential causes of input testcase failures. Thus implementing them collectively increases the chances of success.
The former IBM CEO, Thomas Watson famously inspired us with his words — “Good design is good business”. So, always remember that well-structured, efficient, and readable code plays a crucial role too!
You can find more insights on these methods in this Blog Post. Using these comprehensive techniques could eventually help you ace any coding challenge on platforms like Hackerearth.
Towards Robust Outputs: Correcting Hackerearth Solution Errors
To enhance the robustness and correctness of a HackerEarth solution, it’s pivotal to identify the root cause of the solution failing for an input testcase. Primarily, one needs to understand whether the error pertains to logic, code syntax or certain special edge cases.
Identifying Logic Errors
If your program incorrectly handles data, it might indicate logic errors in your solution. For example, should you be developing a problem based on sorting algorithm and the output returns unorganized lists, this indicates there’s a logical issue.
ListmyList = Arrays.asList(4,2,9,7,5,1); Collections.sort(myList. System.out.println(myList); //Output: [1,2,4,5,7,9]
Hence, if you encounter such errors, it is recommended to meticulously evaluate algorithms and conditional statements. (source)
Syntax Errors
These are mistakes in the Java language rules. Your program won’t even start if it has these kinds of bugs:
public class Test { public static void main(string[] args) { //ERROR: `string` should be `String` System.out.println("Hello, world!"); } }
Here, your integrated development environment (IDE) can aid significantly in identifying and correcting such errors. Keep an eye out for red underlines, they typically indicate a possible syntax error. (source)
Edge Cases
In some instances, your algorithm might work correctly for most inputs but fail at particular ‘edge’ values. Consider simple division operations where attempting to divide by zero would result in an ArithmeticException:
public class DepictArithmeticException { public static void main(String... showDivideByZero) { System.out.println(10 / 0);// Output: Exception in thread "main" java.lang.ArithmeticException: / by zero } }
Therefore, thoroughly testing edge cases is crucial when enhancing the robustness of your solution. Make sure to include edge-cases in your test suite.
The renowned computer scientist Donald Knuth once said, “Beware of bugs in the above code; I have only proved it correct, not tried it.” This statement re-emphasizes the importance of debugging, testing, and correction while creating software solutions. Successful addressing of these concerns would subsequently increase your likelihood of passing those tricky HackerEarth test cases.
HackerEarth
poses programming challenges that require developers to form strategic solutions. Under some circumstances, these solutions might fail for certain input test cases; whether due to an oversight in logic, incompatible variable types, or aberrations in utilized data structures.
Situation | Explanation |
---|---|
Logic Misrepresentation | A flaw in logic design can cause discrepancies between the expected outcome and the actual output. An initial step should be scrutinizing the implementation details closely, correcting those pieces where assumptions don’t meet specifications. |
Variable Type Infringement | In Java, different variable types manage different kinds of data. If the chosen variable type cannot handle the question’s requirements, the result might incur errors. Developers must stay attuned to the varying limitations each variable type imposes. |
Data Structure Conundrum | Each programming problem calls for an appropriate data structure. A simplified example would be utilizing an array when a LinkedList would be more efficient due to frequent insertions and deletions. An understanding of which data structure best serves the problem at hand ensures optimal results. |
To further elude detection by AI checking tools, developers might obfuscate their code. Code obfuscation involves changing the code structure without affecting its functionality, making it harder for AI tools to evaluate.
Unfortunately, this practice is not encouraged as it reduces readability and maintainability, principles of exemplary coding ethics. As Robert C. Martin notes;
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand” – Robert C. Martin.
Hence, aiming to create readable, maintainable, and logically sound solutions provides the most beneficial approach when tackling programming problems on platforms like HackerEarth.