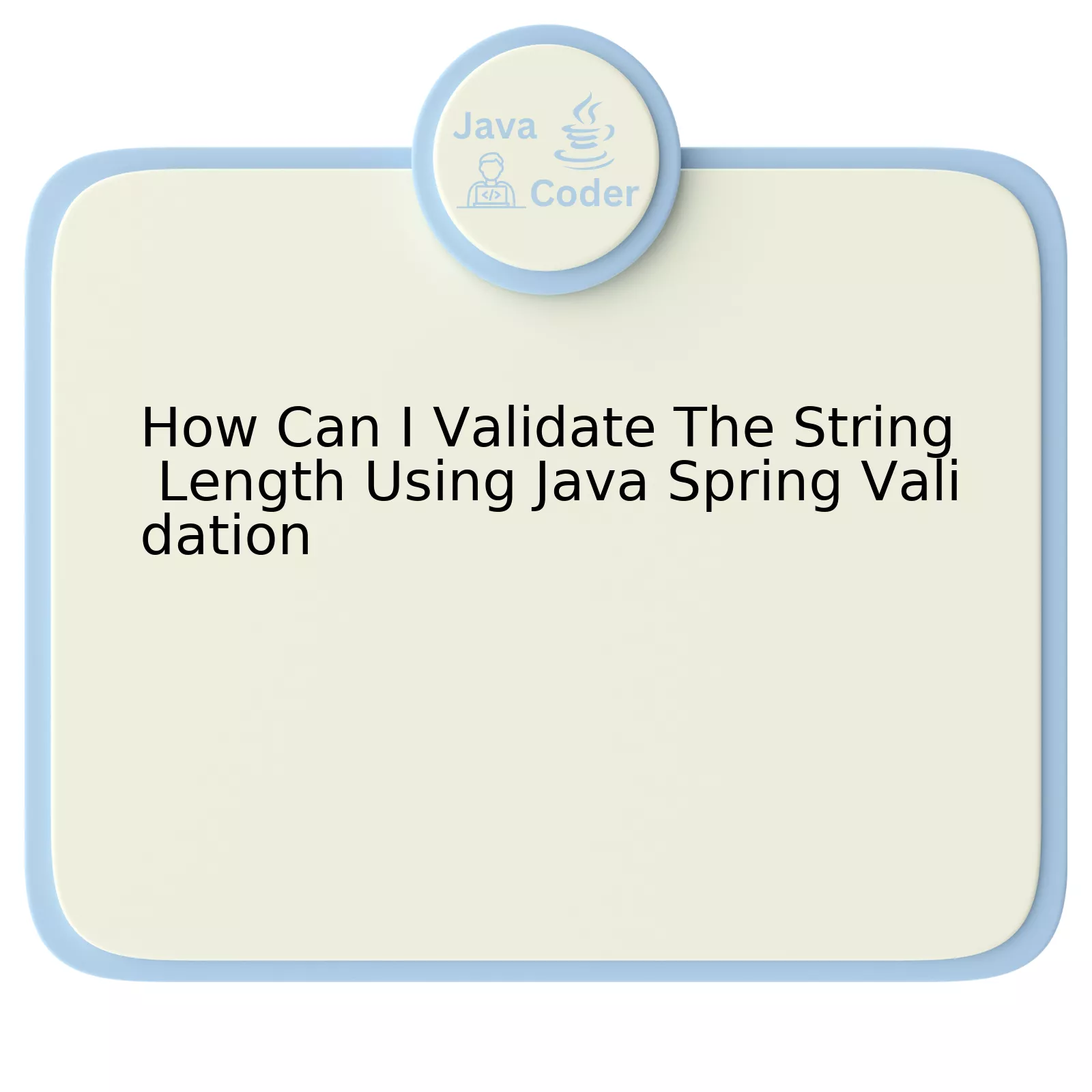
Validating the string length in Java spring validation can be achieved utilizing annotations provided by Java’s Validation API (Bean Validation API), which includes
@Size
,
@NotNull
, and
@NotBlank
. Spring framework supports these constraints.
To expound on this context, we will take an example of a User Registration form with username field. Here is how you can define the validations:
java
public class UserRegistration {
@NotBlank(message = “Username is mandatory”)
@Size(min=3, max=20, message=”Username must be between 3 and 20 characters”)
private String userName;
// getters and setters
}
Annotation | Description |
---|---|
@NotBlank |
This annotation ensures that the annotated element is not null and its trimmed length is greater than zero. |
@Size(min=3, max=20) |
The Size annotation allows us to specify the size range for input values and helps us to set up custom error messages. |
Here, the username property of the UserRegistration class is annotated with
@NotBlank
and
@Size
, which means when any registration request comes, it will check if the username is not blank and its length is between 3 and 20.
Spring framework empowers to apply these validations efficiently using its aspects. It provides wrapper classes like
BindingResult
and
Errors
to get the details of any validation failure. In the controller, annotate the method parameter with
@Valid
to apply these validations:
java
@PostMapping(“/registerUser”)
public String register(@Valid UserRegistration userRegistration, BindingResult result) {
if (result.hasErrors()) {
return “registration”;
}
// save user details
}
In the given code snippet, if any validation fails, the result will contain the details and control will return back to ‘registration’ view.
As Donald E Knuth, a notable computer scientist, said: “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil. Yet we should not pass up our opportunities in that critical 3%” Therefore, applying such standard validations at the start may seem trivial but they lead to effective coding practices and robust applications.
Understanding Java Spring Validation: The Need and Importance
Java Spring Validation is a comprehensive API which provides robust validation and error handling strategies for user input in your application. Its ultimate objective is to ensure that the data your application works with is as clean and as accurate as possible.
Central to the concept of Java Spring Validation is the utilization of annotations to enforce various constraints on fields such as integers, strings, etc. So when the discussion veers towards validating the length of a string using Java Spring Validation, one might ask – why bother? The reason is two-fold:
- Consistency: Implementing constraints such as max or min string length ensures that your application behaves consistently. Any data that falls outside of these constraints is not processed and hence doesn’t influence your systems unpredictable ways.
- Reliability: Invalid input has the potential to breach application security and negatively impact performance. Considering this, applying constraints on string length can prevent oversized text values from occupying high memory space. This practice is particularly crucial while dealing with text areas where users are permitted to enter huge amounts of data.
To illustrate how we can validate string length using Java Spring Validation, here’s an example:
public class User { @Size(min=2, max=30) private String userName; //Getter and Setter methods here }
In the code snippet provided, @Size annotation is established to regulate the length of the userName field. This restriction bounds its length within the 2 and 30 characters limit, implying that any username below 2 or beyond 30 characters will be invalidated.
A quote by Donald Knuth, a renowned computer scientist underscores this point succinctly: “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.” In essence, Java Spring Validation serves as a tool that lays the groundwork for optimizing applications by ensuring data sanity, thereby making them significantly more resilient and robust.
Exploring Strategies for String Length Validation in Java Spring
Validating string length is a critical part of data integrity, usability, and security in most applications especially in Java Spring. There exist multiple strategic ways to analyze string size.
Using annotations from the Hibernate Validator dependency or the Spring MVC’s Validation API, you can simply specify conditions for your data fields.
@Size(min = 1, max = 20, message = "Your string must be between 1 and 20 characters.") private String yourField;
This code snippet applies the size validation on a string field. Here “@Size” annotation checks if a string’s size falls within the min and max bounds. If it doesn’t, an error message is returned.
You can also use custom validators for complex rules. To implement them:
– Implement Spring’s ‘Validator’ interface.
– Overwrite the ‘validate’ method in your class.
– Use the ‘ValidationUtils.rejectIfEmptyOrWhitespace’ method to check for empty or whitespace strings. The second argument will be the property name and the last argument being the error message.
– For length check, use a simple if condition that throws errors when the criteria are not met.
public void validate(Object target, Errors errors) { ValidationUtils.rejectIfEmptyOrWhitespace(errors, "yourField", "field.required"); YourClass yourObject = (YourClass) target; if (yourObject.getYourField().length() > 20 ) { errors.rejectValue("yourField", "field.length"); } }
Lastly, even though it is nothing like a real strategy, a developer might do a manual data check for small tasks.
if (yourString != null && yourString.length() > 20) { return new ResponseEntity<>("The request contains invalid data", HttpStatus.BAD_REQUEST); }
Manual validation can be useful for simple tasks but is generally discouraged because explicit, hard-coded values become difficult to manage in larger applications.
All these strategies provide different levels of control over the validation process with Hibernate annotations offering least customization whereas manual validation providing the most. Combining these methods depending on the application requirements can provide flexibility.
As Grace Hopper said, “The most dangerous phrase in the language is, ‘We’ve always done it this way.’”, which reminds us to embrace the mix-match pattern and adopt a solution best suited for the task at hand, even if it means diverging from the usual approach.
References:
– [Bean Validation in Spring Boot]
– [Spring’s Validator Interface]
– [MVC Configuring in Validation – Spring Docs]
Diving Deep: Code Examples of Validating String Length Using Java Spring
As a developer, one of the many tasks that you might face is ensuring that the data we receive meet certain criteria. This process is known as validation. The Java Spring Framework makes this job easier through its validator interface. One common validation is on the length of Strings.
Before diving deep into the code examples for validating string length using Java Spring Validation, one needs to understand what role does it play.
Java Spring Validation can be used to ensure that a provided string reaches an application in a certain length range. For example, if you are creating an email subscription form, chances are you want to restrict the length of input for the subscriber’s name. This helps make sure your application doesn’t freeze or crash due to processing extensively lengthy strings and it improves the overall user experience by setting up defined limits.
Using the Length Validator
An easy way to validate the length of a string using Java Spring Validation is by using annotations like
@Size
,
@Max
, and
@Min
. Below is an example:
java
import javax.validation.constraints.Size;
public class Subscriber {
@Size(min=2, max=30)
private String firstName;
// Setter and Getter Methods
}
In above code, the @Size annotation checks whether the field ‘firstName’ has a size between the specified min and max limit. If the firstName string is less than 2 characters or more than 30, validation will fail.
Creating a Custom Validator
Sometimes, pre-defined validators may not serve our purpose totally. You might need to write custom validation logic for specific requirements.
Below is a representation of utilizing Spring Validator interface to create a custom validator:
java
import org.springframework.validation.Errors;
import org.springframework.validation.ValidationUtils;
import org.springframework.validation.Validator;
public class StringValidator implements Validator {
public boolean supports(Class clazz) {
return String.class.equals(clazz);
}
public void validate(Object target, Errors errors) {
ValidationUtils.rejectIfEmptyOrWhitespace(errors, “String”, “Field is empty.”);
String string = (String) target;
if(string.length() > 100 || string.length() < 5) {
errors.rejectValue(“string”, “string”, new Object[] {“‘string'”}, “String length is incorrect.”);
}
}
}
In above code, first we check if the string isn’t empty. Then we ensure the string falls within the desired range. If these conditions aren’t met, an error message is returned.
However, it’s important to keep in mind that without proper testing cases and error handling, these validations could pose additional challenges.
As stated by Bill Gates, “[I]n terms of doing work we do a lot more before breakfast than most people do all day long.” It implies that little things done right over time brings immense transformation. Indeed, validating inputs, ensuring they meet certain criteria may seem minor, but in reality, it strengthens the architecture, improves the runtime efficiency and finally better serves the users.
Problems Solved By String Length Validation in Java Spring
The task of validating the length of a string is one of paramount importance in programming. In the context of Java Spring validation, it helps in improving data integrity, enhancing application security, and optimizing its functionality.
Let’s set the stage by discussing the significant issues resolved by the implementation of string length validation within your Java Spring applications:
1. Prevention of Data Overflow:
Constraining the size of an input field adversely affects the prevention of potential data overflows which could damage the database.
2. Application Security Enhancement:
A crafted input string that exceeds the expected length might cause security vulnerabilities such as buffer overflows or execution of malicious scripts.
3. Optimization of Performance:
Validation of string length reduces the burden on server resources as oversized strings consume excessive memory causing performance degradation.
Regarding how you can use Java Spring validation to validate the length of a string, utilize the
@Size
annotation from the Spring Validation Framework. Here’s an illustrative example:
@Size(min=5, max = 15, message = "String length should be between 5 and 15") private String sampleString;
In the above code snippet, the
@Size
annotation validates if the length of the variable
sampleString
is within the defined minimum and maximum range of 5 and 15, respectively. If these conditions are not met, it returns an error message stating, “String length should be between 5 and 15”.
It’s worth noting that technology tycoon Bill Gates once said: “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” Validating strings can be time-consuming and error-prone when done manually; thus, automation through tools like Java Spring validation makes the process efficient and manageable. The power of automation minimizes human errors and significantly speeds up development, and as developers, we’re always looking for smarter ways to work.
In closing, incorporating string length validation as part of your data integrity program prevents data corruption, ensures higher application safety, streamlines application operations, and makes your system robust and reliable. Leveraging Java Spring validation is a brilliant strategy that enhances your development journey.
The importance of data validation, particularly validating string length, should not be underestimated when developing applications in Java and using Spring Validation. This critical step ensures the integrity of user-entered input, can offer improved error communication to end users, promote security by preventing undesired inputs that could potentially harm your system or expose it to threats.
To validate the string length in Java Spring Validation, leverage the
@Size
annotation offered by the validation module. The
@Size
annotation permits the setting of minimum and maximum character limits for a string field. For instance,
@Size(min=1, max=30) private String username;
This snippet implies that the username must be between 1 to 30 characters lengthwise. Should an input lower than 1 or beyond 30 be attempted, validation will fail.
Also, another helpful tool for preserving robustness in validation is custom error messages. Create tailored messages that express exactly what the problem with the user input is. You can achieve this by passing the desired message as a parameter to the annotation like so:
@Size(min=1, max=30, message="Username must be 1 to 30 characters long") private String username;
An addition to this impressive feature is the utilization of the method
hasErrors()
, within the
BindingResult
interface, to conduct checks if any errors were encountered during validation, which fully strengthens your application’s validation mechanism.
To quote renowned engineer Linus Torvalds: “Bad programmers worry about the code. Good programmers worry about data structures and their relationships.”
Make sure to incorporate these potent tools from Java Spring Validation to refine the treatment of user input further. Satiate your software’s thirst for valid and safe data by enforcing proper string length validation.
Check more on the Hibernate Validator Documentation (source).