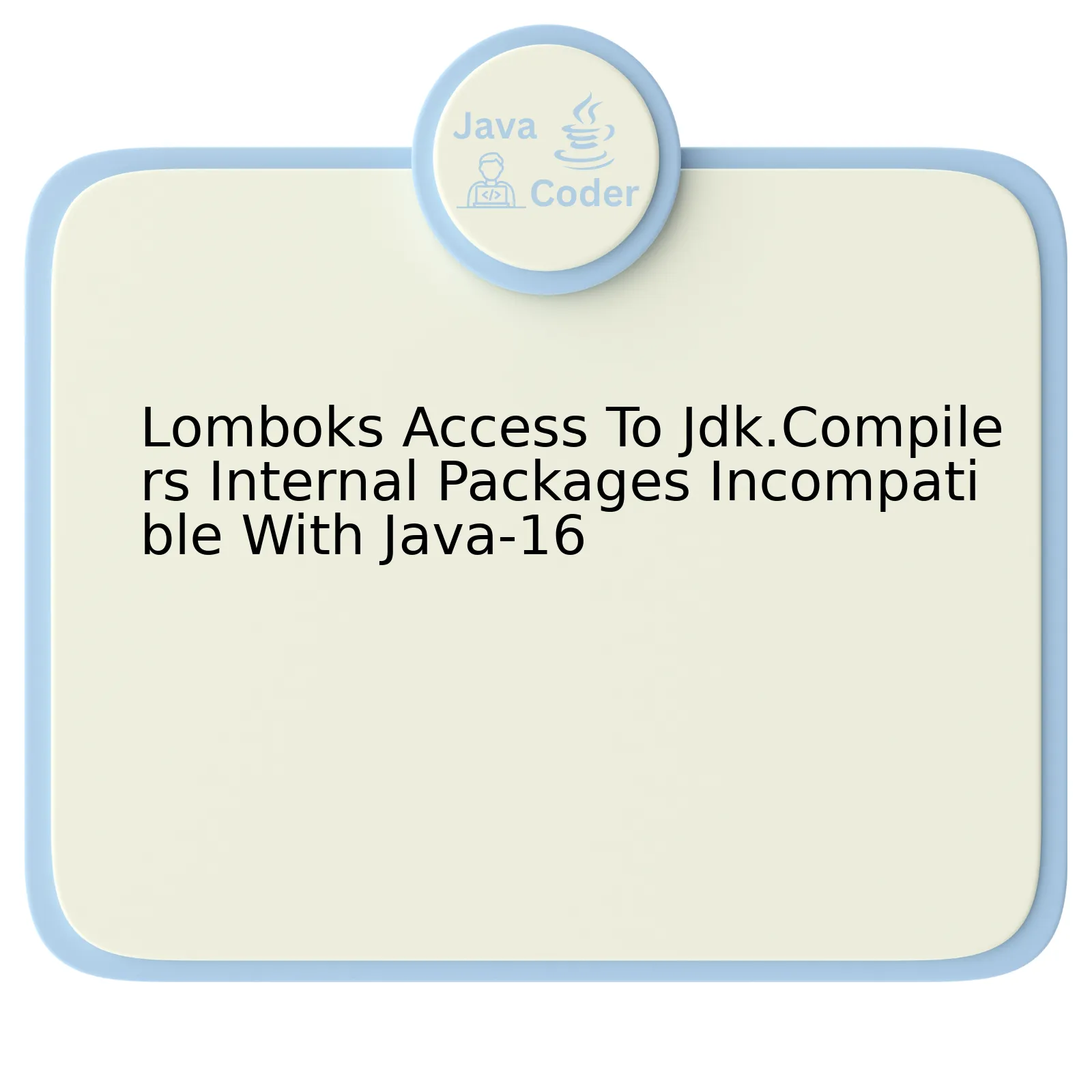
Lombok | JDK Compilers | Java 16 |
---|---|---|
Lombok is a Java library that plugs into your editor, eliminating the need for getters, setters, and other mundane code. | The JDK (Java Development Kit) compilers are essential tools for developers to compile and run their Java applications. | Java 16 is one of the latest stable version that brings several enhancements over its predecessors. |
Currently, Lombok faces a significant challenge regarding its access to JDK compilers’ internal packages. With the introduction of Java 16, the compatibility issues have emerged, mainly due to the encapsulated internals implemented in this Java version.
The foundation of the problem lies within JDK’s move towards more encapsulation. This decision limits the reachability of non-exported packages and hence obstructs frameworks like Lombok that were previously accessing them. Specifically, Lombok accesses “jdk.compiler”, an internal package of the modular system in JDK, which is no longer permissible with Java 16 compliance.
To resolve these issues with Java 16, it has been suggested to apply command-line flags like “–add-opens” or “–add-exports”. Unfortunately, this approach can be cumbersome for developers since they might need to mix different versions of Java in the same environment, given Lombok’s wide usage across numerous projects.
“Always code as if the guy who ends up maintaining your code will be a violent psychopath who knows where you live.” – John Woods
Migrating from older versions of Java to Java 16 requires careful planning and consideration of impacted libraries and frameworks. It’s important to keep updated with how such crucial frameworks like Lombok evolve their API adherence related to the stricter encapsulation introduced by newer Java versions.
It remains to be seen how Project Lombok adapts to fit into this new encapsulated world brought in by Java 16 whilst aiming not to dilute the developer experience it significantly enhances. For now, developers could stick to using older versions of Java for their Lombok-based implementations until a viable solution emerges.
Understanding the JDK Compiler’s Internal Packages: An Overview
Delving into the JDK Compiler’s internal packages with a focus on the compatibility issues between Lombok and Java-16 can provide valuable insight. The JDK (Java Development Kit) Compiler, widely adopted for converting Java code into bytecode that JVM (Java Virtual Machine) can understand, consists of different internal packages that are instrumental in various stages of code compilation.
To start off, one needs to appreciate that
jdk.compiler
and its sub-packages contain interfaces, classes, enums, and exceptions consummated in the Java programming language, facilitating it the capacity to perform source file(s) to bytecode transformations.
The internal package
com.sun.tools.javac.main.JavacOption.Option
, for example, is crucially involved with command-line options. Other known classes like
com.sun.tools.javac.main.OptionHelper.GrumpyHelper
and
com.sun.tools.javac.main.OptionHelper.DefaultHelper
aid programmers in handling diverse situations by introducing adaptability in various conditions during compilation.
Lombok and JDK Compiler’s Internal Packages Compatibility Issues
Though helpful tools such as Lombok help to get rid of boilerplate code in Java, they struggle with accessing JDK compiler’s internal packages—particularly visible with Java-16. The main reason stems from Project Jigsaw’s introduction when it launched with Java-9. It introduced a modular system that encapsulates internal APIs, making them inaccessible by default. While this helps to enhance platform integrity and maintainability, it also restricts the visibility of certain JDK internal APIs.
Herein, we face the issue—an incompatibility problem between Lombok and the JDK compiler’s internal packages in Java-16 due to strong encapsulation of these internal APIs. No longer are coding frameworks free to interact with
jdk.compiler
. The mandatory restrictions require explicit commands to allow interaction, which ultimately introduces barriers and compatibility issues.
Overcoming Limitations
Fortunately, Java provides an escape route. To access the internal APIs, flags such as
--add-exports java.compiler/jdk.compiler.com.sun.source.tree=ALL-UNNAMED
and
--add-opens java.compiler/jdk.compiler.com.sun.source.util=ALL-UNNAMED
can be used. However, these commands carry long-term maintenance implications and risks.
I would like to conclude with a quote from the renowned software engineer Uncle Bob Martin: “The best code is no code at all”. In the context of this discussion about JDK compiler’s internal packages, Lombok and Java-16 compatibility issues, it serves as a gentle reminder to strike the balance between ease of development and enduring application performance and maintainability rather than hacking around limitations. (source)
Exploring Lombok’s Interaction with JDK.Compiler’s Internal Packages
Project Lombok is a Java library tool that is used to reduce the boilerplate code and increase productivity by simplifying the Java coding. It facilitates various annotations to implementing tedious or repetitive tasks such as creating getters, setters, and toString methods automatically.
Lombok’s underlying working mechanism involves accessing Java Development Kit (JDK) compiler’s internal packages for generating bytecode directly. However, such access has been subjected to certain issues in recent JDK versions, specifically with Java 16.
With the strict encapsulation policies implemented in JDK 9 under the Jigsaw Project, significant changes were made leading to encapsulation of internal elements of the JDK. Access to these internal APIs have been increasingly restricted over different versions to ensure integrity and security. When reaching JDK 16, the strong encapsulation was enforced further – making it essentially incompatible with tools like Lombok.
Here is a brief concept of what happens:
// Including Lombok Annotations import lombok.*; @AllArgsConstructor @NoArgsConstructor @Data public class Employee { private String firstName; private String lastName; }
Using this Lombok annotated code, a simple Employee class can be modified at runtime through the use of JDK Compiler’s internal packages to include additional methods such as getters, setters and toString.
However, with JDK 16 encapsulating its internal packages, Lombok cannot gain unrestricted access to modify the compiled classes. As a result, developers would encounter error messages stating inaccessibility of internal APIs.
To bypass JDk 16’s encapsulation policy without any violation, you may run JVM with the `–illegal-access=warn` or `–illegal-access=permit` option. But it only offers a temporary fix and does not solve the fundamental incompatibility issue between Lombok and JDK 16. With future Java versions, these access options will likely be removed, thus raising a need for an updated strategy for code generation libraries like Lombok.
Steve Jobs once said, “Innovation distinguishes between a leader and a follower”. This clash between Lombok and JDK 16 advances a notable opportunity for innovation: other ways for Lombok to facilitate its features without violating JDK encapsulation. Alternatively, there could be means for JDK’s encapsulation functionality to allow such utilities selectively that facilitate developer productivity- without compromising on security.
It is advisable to keep track of updates from both Lombok and OpenJDK community as solutions are likely to be introduced over time. For now, using earlier JDK versions where Lombok is still compatible might be your best bet if you wish to take full advantage of Lombok’s productivity enhancing features.
Dealing With Incompatibilities Between Lombok and Java-16
Java is an evolving language with constant changes and improvements to each new version. Over the years, many libraries like Project Lombok have emerged to tackle common problems and make the Java programmer’s life easier. However, as Java evolves, certain incompatibilities may pop up between versions of the JDK and some third-party libraries. Here, we will discuss the incompatibilities issues encountered while using Lombok with Java-16.
Lombok is a popular tool that helps reduce boilerplate code in Java by generating getter/setter methods, builders, constructors, and more during compile time. Despite its convenience, using Lombok with Java 16 might encounter issues due to Lombok’s dependency on the now encapsulated internal compiler APIs.
Java 9 onwards, certain packages were restricted under Java Platform Module System (JPMS). As part of JPMS, Java’s internal APIs are no longer freely accessible. As a result, Lombok’s access to `jdk.compiler` internal packages became incompatible, affecting developers using Java 16 or any releases after Java 9.
JDK’s shift towards tighter encapsulation under Project Jigsaw has had impacts on how third-party libraries interact with the core Java libraries.
To deal with this compatibility issue, several measures can be taken:
- Update Lombok: Developers maintaining Lombok continuously work to ensure it’s compatible with the latest Java versions, thereby ensuring compatibility. If you’re facing issues running Lombok with Java 16, updating Lombok to the latest stable version could be a potential solution.
- Use ‘–add-exports’ flag: The
--add-exports
flag can make inaccessible APIs available to specific modules only, which wouldn’t be available otherwise. Using it during compilation can allow Lombok’s access to internal `jdk.compiler` packages. An example would be
--add-exports=jdk.compiler/com.sun.tools.javac.api=ALL-UNNAMED --add-exports=jdk.compiler/com.sun.tools.javac.file=ALL-UNNAMED --add-exports=jdk.compiler/com.sun.tools.javac.parser=ALL-UNNAMED --add-exports=jdk.compiler/com.sun.tools.javac.tree=ALL-UNNAMED --add-exports=jdk.compiler/com.sun.tools.javac.util=ALL-UNNAMED
.
If Lombok’s compatibility issue still persists,
- Using alternatives: As mitigating dependencies can sometimes be complex, consider using alternative tools such as AutoValue and Immutables until issues are resolved.
In hindsight, it is vital to understand that dependency on internal APIs and non-standard features is always tricky as they are subject to change, and their usage can result in unexpected behaviors when upgrading to newer versions. A good practice is to stay updated with the latest stable releases of both the JDK and the libraries one depends upon, weighing the need against potential future compatibility concerns.
Project Lombok and Java continue to evolve, and library maintainers strive to ensure their resources stay relevant and compatible across all changing environments. Understanding these changes and adjusting code accordingly ensures continued efficient coding practices.
Navigating Fixes for Access Issues in Lombok Towards JDK.Compilers
The recent changes in JDK’s encapsulation policies have affected a myriad of frameworks and libraries, Lombok being a notable example among them. The access restrictions on JDK’s internal packages in Java 16 have drawn a line dividing compatibility with previous versions throwing the gauntlet down for developers working with Lombok.
In order to navigate fixes for access issues in Lombok towards JDK compilers, a number of measures can be discussed:
1. One strategy would be
Upgrading Lombok
. With the issue in hand acknowledged, an upgraded version of Lombok addressing this problem has been released. Hence, it becomes particularly relevant to check and update Lombok’s version regularly.
2. A secondary measure involves
--add-opens
or
--add-exports
. These command-line options can be used to control access to JDK’s internal APIs as they enable certain internal packages of java modules. It helps to break down the barriers that are causing the incompatibility.
Here is an example how you use
--add-opens
java --add-opens java.base/java.lang=ALL-UNNAMED -jar your_file.jar
3. Adding Maven Compiler plugin exemptions can also be a useful solution. When we can’t update our Lombok library, or if new releases do not fix the issues at hand, changes can be made manually by updating the pom.xml file with a maven compiler argument, bypassing the module boundaries set up by JDK16.
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.9.0</version> <configuration> <compilerArgs> <arg>--add-opens java.base/java.lang=ALL-UNNAMED</arg> </compilerArgs> </configuration> </plugin> </plugins> </build>
An understanding of these solutions would help navigate compatibility issues between Lombok and JDK compilers and ensure a smoother development process. As Brian Kernighan famously said, “Debugging is twice as hard as writing the code in the first place.” Keeping that in mind, vigilance in staying updated and adapting to evolutionary changes remains a key principle in programming.
For deeper insights and more professional advice, you may refer to the official OpenJDK details regarding this particular change in encapsulation policies.
Diving deep into understanding the compatibility issues that have arisen with Lombok’s access to JDK Compilers internal packages and Java 16, we can ascertain several critical points. Despite potent functionality offered by Lombok for reduced boilerplate code, one cannot overlook the surfacing issues it has undergone with Java 16 due to changes in encapsulation policies.
Java Version | Lombok’s Compatibility Issues |
---|---|
Java 16 | Access problems to JDK Compilers internal packages due to enhanced encapsulation |
Underlining the problem root, Java 16 makes substantial strides in encapsulation within its internal package
jdk.compiler
, which disrupts Lombok’s functioning. This is attributable to Lombok’s under the hood mechanism needing penetration through encapsulation barriers to wield its magic of boilerplate code reduction.
Discerning developers’ plight over this, a refactoring of Lombok or adjusting to the new encapsulation model of Java 16 becomes necessary workarounds. While there are solicitations to make Lombok extensions as part of the Java compiler, until it materializes, alternative pathways like delombok or explicit coding practices might need adoption.
Naturally, these changes demand an upward curve in learning and adaptation from developers before they can reap benefits from both Java 16 and Lombok. Delving into official documentation and forums is suggested to remain updated on this progress and potential solutions[1].
Blaise Pascal’s quote resonates well here, “Our achievements of today are but the sum total of our thoughts of yesterday.” Precisely so, it is insightful how robust discussion and collaboration among developer communities pose potential to tackle such complexity and pave the path toward improved software tools and practices.