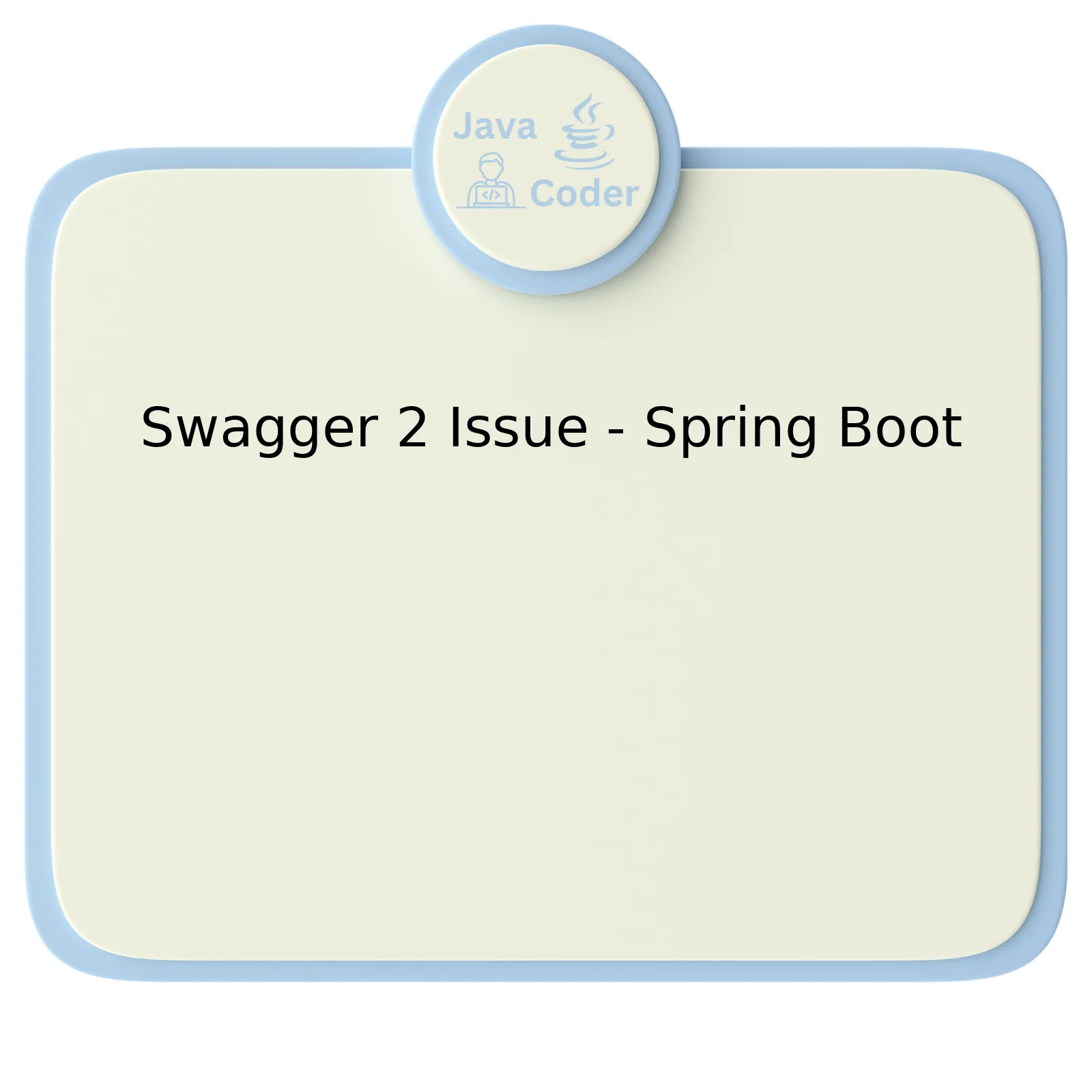
The following table provides an overview of the main details associated with Swagger 2 issues specifically dealing in the context of Spring Boot:
Title | Description |
---|---|
Issue | The code encounters problems when integrating Swagger 2 with a Spring Boot project due to discrepancies in the default conveying configurations. |
Root Cause | These complications often stem from an incorrect configuration or incompatible versions of Swagger and Spring Boot. |
Possible Solution | Reconfiguring Swagger or updating it, if the current version seems incompatible with the existing Spring Boot version, may be feasible solutions. |
Prospects for Resolution | With diligent test-driven development and careful update management, avoiding these issues becomes significantly easier. |
Broadly talking about the issue, when integrating Swagger 2 into Spring Boot application, developers can occasionally encounter various problems. These can range from simple misconfiguration issues to more complex ones, such as incompatibility between different software package versions. Take the scenario where an application built on an older version of Spring Boot presents trouble while integrating with the latest version of Swagger; this is potentially attributed to an incompatibility issue.
For a robust solution, the enlist procedure could be adhered to: checking the compatibility beforehand, ensuring that we configure Swagger correctly, and validating whether both Swagger and Spring Boot are up-to-date. Version conflicts can be amicably resolved by the upgrade or downgrade of the problematic packages, depending on the circumstances.
As Martin Fowler quotes, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Being meticulous about the configurations and concise about the dependencies keep our code understandable and thereby decreases the likelihood of similar issues arising.
Following this understanding provides a proactive way of managing potential issues that might arise during the integration process.
Understanding Swagger 2 in Spring Boot
Understanding Swagger 2 in Spring Boot, especially in relation to some potential issues, requires a deep dive into its functionalities and the common problems developers may encounter.
Swagger is an open-source framework that aids in the development, design, and documentation of REST APIs. It provides tools for understanding API endpoints without needing to dig into the code itself. Among its benefits are self-documenting RESTful services environment creation and enhanced testing efficacy.
In the context of the Spring Boot environment, integrating Swagger can seem straightforward, due to the presence of ‘springfox-swagger2’ as a dependency. However, there are times when issues like not displaying or recognizing the API endpoints after setting up Swagger can surface.
To illustrate this, one might define a new Spring Boot project and add Swagger dependencies:
<dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.9.2</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.9.2</version> </dependency>
Despite correct setup, perhaps including a configuration class for Swagger:
@Configuration @EnableSwagger2 public class SwaggerConfig { @Bean public Docket api() { return new Docket(DocumentationType.SWAGGER_2) .select() .apis(RequestHandlerSelectors.any()) .paths(PathSelectors.any()) .build(); } }
One might find that the API endpoints do not appear on the Swagger-UI page (usually accessed at ‘/swagger-ui.html’). It could be mistaken as a Swagger 2 issue, but it’s usually caused by Spring Security configurations, i.e., restrictions not allowing Swagger to scan for controllers.
If you face such an issue, ensure to consider two factors:
1. Security Configurations: Check your security configurations. If they don’t allow Springfox’s handlers to bypass them, Swagger won’t be able to scan your controllers. Test by temporarily disabling Spring Security and see if Swagger works.
2. Controller Mapping: The controller’s package might not be correctly set for ComponentScan in SpringBootApplication, causing Swagger to fail mapping it properly. Check if your controllers reside under the directory (or sub-directory) of your main Spring Boot Application file.
Adaptations may need to be made to security config classes or component scan configurations to rectify the issue.
As Martin Fowler eloquently put, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” By adequately understanding, implementing, and troubleshooting Swagger 2 in Spring Boot, we enhance human comprehension, making our APIs accessible and manageable.
References:
TutorialsPoint – Enabling Swagger2
Baeldung – Documentation For Spring REST API
Identifying Common Issues in Swagger 2 with Spring Boot
Swagger 2 is a widely used tool for designing APIs, and it can pose some challenges when integrated with Spring Boot. Here, we aim to detect and resolve common issues that can arise during this integration.
Issues related to misconfigured or missing annotations
Often, developers may encounter problems in Swagger 2 due to missing or misconfigured Swagger 2 annotations on API endpoints. This often leads to incomplete or wrong documentation of the API endpoints in Swagger UI.
For example:
&i#64;ApiOperation(value = "Get user by ID", response = User.class) public User getUser(@ApiParam(value = "ID value for the user you need to retrieve") @PathVariable Integer id) { //Method implementation }
This snippet indicates important information such as operation description, response model, or parameter information in the generated Swagger UI, but if not written properly, it might lead to incomplete API endpoints documentation.
Mismatched Content Type Issue
The mismatch in the content type may result in an error message similar to “no response from server” or a problem while trying to invoke the REST API using Swagger UI. This issue stems from the aspect that Spring Boot’s default data transfer format is JSON, and any divergence requires explicit configuration.
Incomplete Documentation
This is one major shortcoming affecting Swagger2-Spring Boot integration where the API documentation provided is not comprehensive enough. Explicitly documenting the models and responses helps make it more descriptive and easy to understand. Below is an example of how certain entities are annotated for better representation:
&i#64;ApiModel(description = "Details about the user") public class User { //class attributes and methods }
As Marc Andreessen articulated aptly, “Software is eating the world.” Nevertheless, even the most popular and powerful software like Swagger2 has its weaknesses. Addressing these challenges should thereby be part and parcel of every developer’s work itinerary especially when integrating Swagger2 with frameworks such as Spring Boot.
More insights about common issues and their fixes during Swagger 2 and Spring Boot integration can be found here.
Resolving Swagger 2 Errors in Your Spring Boot Application
Swagger 2, essential in building robust APIs in a Spring Boot application, could at times present errors that may halt the software development process. One common issue includes Swagger API not displaying any endpoints. To diagnose, fix such problems comprehensively, our discussion will encompass:
1. Configuring Swagger,
2. Fixing Swagger configuration issues,
3. Fixing absence of Swagger UI,
4. Resolving missing endpoints.
Let’s launch into the nitty-gritty.
Configuring Swagger
The first step when working with Swagger is to ensure correct and robust configuration. In a Spring Boot application, your Swagger Configuration file might look something like below:
@Bean public Docket api() { return new Docket(DocumentationType.SWAGGER_2) .select() .apis(RequestHandlerSelectors.any()) .paths(PathSelectors.any()) .build(); }
If appropriately set, this configuration should help Swagger identify the controllers and map them to corresponding paths for appropriate HTTP methods.
Fixing Swagger Configuration Issues
The following are solutions to common Swagger configuration problems:
– If Swagger isn’t configured correctly, it’s advisable to debug the SwaggerConfig.java or check for errors regarding the same in console logs.
– Remember to update the pom.xml file by adding dependencies required for Swagger like ‘springfox-swagger-ui’ and ‘springfox-swagger2’.
– Ensure that all necessary annotations (@EnableSwagger2, @ComponentScan) are included inside the main application class.
Fixing Absence of Swagger UI
Assuming the Swagger UI does not appear, the prime suspect would be the version of Swagger in use. Note that ‘springfox-swagger-ui’ version 3.x has altered the default URL from localhost:8080/swagger-ui.html to localhost:8080/swagger-ui/. Therefore, it’s advised to check the updated URL or switch back to an earlier version if needed.
Resolving Missing Endpoints
If controllers aren’t showing up as expected on the Swagger UI, the probable causes could be:
– The controller lacks relevant swagger notation, e.g., @RestController, @RequestMapping.
– The APIs in question might be beyond the Docket paths() scope. Make sure the API paths align perfectly with what’s defined in the Docket bean in your Swagger configuration.
As Bill Gates once aptly stated, “The computer was born to solve problems that did not exist before.” As coders, we thrive on problem-solving; therefore, encountering and surmounting challenges is part and parcel of the journey. Happy coding!
Best Practices for Using Swagger 2 with Spring Boot
Swagger 2 Best Practices with Spring Boot
Integrating Swagger 2 into your Spring Boot application streamlines API documentation, enhances UI interaction, and simplifies testing. Remember to include the necessary dependencies in your
pom.xml
or
build.gradle
file.
<dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.9.2</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.9.2</version> </dependency>
Spring will now automatically configure Swagger.
Do not neglect method annotations as these offer useful insights about the operation of specific APIs. For instance, use
@ApiOperation
along with value parameter for all HTTP methods for descriptive information. Include the response models using the
@ApiResponse
annotation.
It presents a prime example of the power of shared open-source work. Google’s Director of Open Source, Chris DiBona was quoted, saying “Open source is good for everyone”which extends to the open standards set by Swagger 2.
If you are dealing with incorrect rendering of Swagger UI in a Spring Boot application, the likely culprit is mismatched SpringFox libraries version. Ensure that both springfox-swagger2 and springfox-swagger-ui dependencies have the same version.
Swagger 2 might not display correctly on Spring Boot apps due to misconfigured resource handlers. To resolve this, explicitly configure a resource handler that mappings to “/swagger-ui.html”.
@Override public void addResourceHandlers(ResourceHandlerRegistry registry) { registry.addResourceHandler("swagger-ui.html") .addResourceLocations("classpath:/META-INF/resources/"); }
Mindful of security, apply OAuth or implement other security measures to regulate access control. Work closely with the
@ApiIgnore
annotation to hide specific operations from the final documentation if not relevant to the user.
The Docket bean can be tuned and customized to craft unique API descriptions. Configure policies based on custom defined Response Messages, Ignored Paths, Alternate Type Rules among others.
@Configuration @EnableSwagger2 public class SwaggerConfig { @Bean public Docket api() { return new Docket(DocumentationType.SWAGGER_2) .select() .apis(RequestHandlerSelectors.any()) .paths(PathSelectors.any()) .build(); } }
For more details, see the official SpringFox documentation. By adhering to recommended Swagger 2 best practices, Spring Boot applications can enjoy an interactive application interface, seamless integration, and a smoother development process.
As the issue addressed, Swagger 2, in conjunction with Spring Boot, exhibits particular challenges that may hinder smooth application operations.
One perspective to ponder on is about specific compatibility issues between Swagger and Spring Boot versions. If the two aren’t properly aligned from a version standpoint, it’s possible this can cause application failures in deployment or operation.
< dependency > < groupId >io.springfox< /groupId > < artifactId >springfox-swagger2< /artifactId > < version >${swagger.version}< /version > < /dependency > < dependency > < groupId >io.springfox< /groupId > < artifactId >springfox-swagger-ui< /artifactId > < version >${swagger.version}< /version > < /dependency >
Another aspect is related to the configuration of Swagger 2. Neglecting necessary Swagger settings when integrating it into your Spring Boot application might also lead to complications. Check out this sample Swagger configuration code:
@Configuration @EnableSwagger2 public class SwaggerConfig { @Bean public Docket api() { return new Docket(DocumentationType.SWAGGER_2).select() .apis(RequestHandlerSelectors.any()) .paths(PathSelectors.any()) .build(); } }
Consider troubleshooting techniques such as revisiting, reviewing, and refining your project’s dependencies and configurations.
Remember what Edsger W.Dijkstra said: “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” This quote implies the need for keen observation and diligence when setting up Swagger 2 with Spring Boot.
To wrap up, resolving problems associated with Swagger 2 setup in a Spring Boot environment does not just involve pinpointing the error and working towards fixing it but also asks for further learning and understanding about integrating these technologies. A thorough study of the Spring Boot documentation, other relevant software documentations and online resources like the Official Spring Boot site can guide developers to find effective solutions.