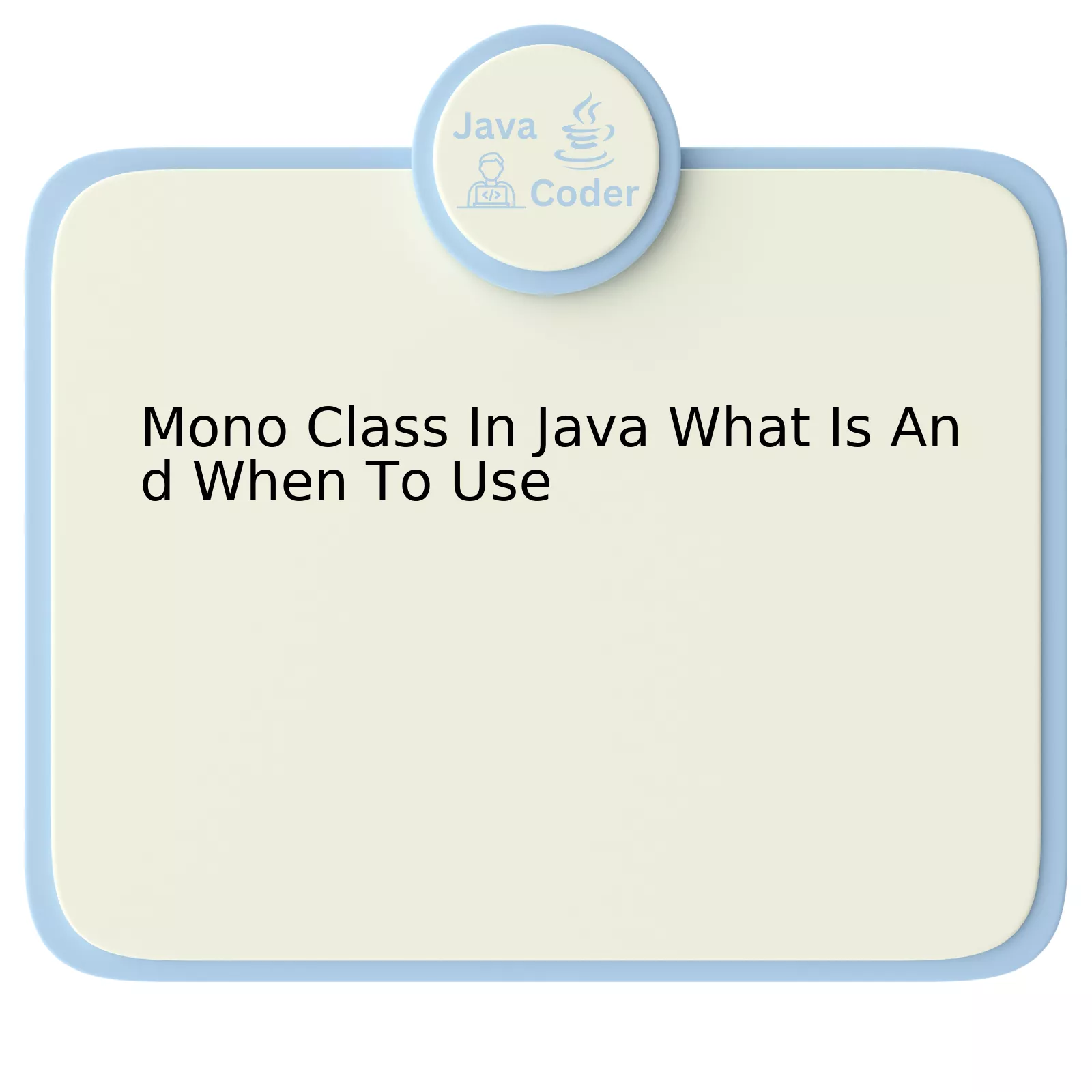
A deeper understanding of Mono class in Java and its various usages can be illustrated through an informative table:
Mono Class Feature | Description |
---|---|
Definition | Mono is a class in Java that belongs to the Reactive Programming Paradigm. It allows developers to work with asynchronous data streams. |
Usage | This class is effectively used when you are dealing with asynchronous processing and you expect no more than one item to be returned by the operation. |
Purpose | The leading objective of this class is to handle asynchronous operations more efficiently, like networking activities or file input/output processes, by managing everything under single threads or events. |
Implementations | It has been implemented successfully in creating non-blocking applications and services in micro-services architecture. Moreover, it finds itself very relevant in Spring WebFlux. |
Mono class, as defined above, plays an integral role in reactive programming in Java with its unique ability to deal with asynchronous data streams efficiently. Its implementation focuses on the idea of handling potentially lengthy operations such as network activities or file I/O by managing them under a unified thread or event. This functionality makes Mono an excellent tool for the development of non-blocking applications.
To put this into context, consider an example where we want to read content from a file asynchronously. Here we could use Mono to manage the operation:
Mono.fromCallable(() -> { // Code to read file content; }).subscribeOn(Schedulers.boundedElastic());
The scope and relevance of the Mono class is broad; one of its many successful implementations can be seen in microservices architecture and Spring WebFlux. As Urs Hölzle, a Senior Fellow and Vice President of Technical Infrastructure at Google once stated, “With software there are only two kinds of problems: those which are unprecedentedly hard, or those which have not yet been solved due to programmers insisting on their comfortable status quo.” The adoption and usage of Mono class promises to help overcome these barriers and move Java coding forward.
This coding tool aids programmers in addressing challenging or time consuming aspects of coding, pushing the boundaries of what’s achievable and helping them to progress beyond the comfortable status quo, in line with Hölzle’s sentiment.
For a more detailed insight into using and exploring Mono class, the official documentation provided by Project Reactor is recommended.
Understanding the Concept of Mono Class in Java
Mono class is a crucial part of Java, specifically when working with Project Reactor. Reactor is a Java framework for building reactive and non-blocking applications on the JVM based on the Reactive Streams Specification.
The
Mono
class in java represents a sequence of zero or one items and offers a rich set of operators running on a fast execution model retained over async strategy. It aims to provide an easy-to-use API for dealing with operations that return single values or no value.
What positive attributes does the Mono make accessible?
- Emission of zero or one item: A Mono can emit zero or one item. The emission of zero item occurs when there is no item to be emitted. Meanwhile, the emission of one item happens when there is data to be dealt with. This characteristic makes Mono perfect for situations where you are certain that your data stream will either have one or zero items.
- Laziness: Mono class defines many operators that enhance the processing pipeline including filtration, data transformation mapping, and error handling. However, it doesn’t start emitting items until subscription. Therefore, Monos are lazy by nature and nothing happens until you subscribe.
- Backpressure Support: Mono class provides support for backpressure strategy. Backpressure is a mechanism allowing subscribers to signal to their publishers about the number of items they are ready to consume.
When should we use Mono Class in Java?
Mono
class in Java serves different purposes, such as:
- When developing simple, asynchronous programming tasks that emit only one or zero items. If your function or method returns one item, use Mono.
- The class is applicable for methods that return something but you care not for the result. For instance, a save operation that returns saved entity but you don’t use that entity afterward.
- If you need lazy evaluation, then Mono is suitable. It won’t execute until the subscriber subscribes to the Mono.
Here’s an illustrative example on how you would leverage the Mono Class in Java:
import reactor.core.publisher.Mono; public class MonoJava { public static void main(String[] args) { Mono.just("Hello World").subscribe(System.out::println); } }
This code snippet creates a Mono that emits a single data point (Hello World). Remember, the operation will not trigger until you invoke
subscribe()
.
As per Brian Goetz, Java Language Architect at Oracle said, “If you want to go fast, go alone. If you want to go far, go together.” Reactive Programming with like of Mono class allow you to move fast by simplifying concurrent computing in Java.
For more information about this topic, I would recommend checking out the Project Reactor Documentation .
Breaking Down the Functionality: The Use Cases for Mono Class
Under the aegis of Project Reactor, Mono class in Java mercerizes a multitiered and rivalrous tool in handling data streams, more specifically, manipulating or transforming data from 0 to 1 element. The Mono class is typically utilized when dealing with asynchronous programming. This use case aligns well with Java’s built-in Callable and Future, and brings significant added value: chiefly, the ability to consolidate a series of operations into a single pipeline.
Handling Asynchronous Operations
In execution, traditional synchronous programming hangs your processing unit awaiting data. In comparison, the Mono class enables you to run these operations asynchronously, reducing idle time and promoting more efficient usage of resources. Consider this simplified analog:
public MonogetData() { return Mono.fromSupplier(() -> slowNetworkRequest()); }
In this code snippet, getData() returns a Mono that wraps a particularly sluggish network request. The execution is non-blocking; it does not linger, but instead promulgates control while the slow operation transpires.
Subscription Actions
Subscriptions play a vital role in working with the Mono class as they trigger data stream processing. Employing different subscription actions, such as doOnNext(), doOnError(), or doOnSuccess(), presents an engaging method to insert callbacks in variegated stages of the operation.
Mono.just("Hello, World!") .doOnNext(System.out::println) .subscribe();
This code operates by virtue of the print message occurring on successful emission of the Mono stream, triggered by the subscribe() call at the end.
Combining Data Streams
A quintessential usage of the Mono class lies in its potential to merge streams. When managing multiple asynchronous operations, you would defer operations until all relevant streams have completed their tasks. Mono.zip() serves just this purpose:
Monomono1 = //Some operation; Mono mono2 = //Another operation; Mono.zip(mono1, mono2) .map(tuple -> tuple.getT1() + " " + tuple.getT2()) .subscribe(System.out::println);
This consumes two Mono instances and fuses them into a single string that materializes once both asynchronous tasks complete.
To borrow from Douglas Crockford: “Java is the most distressing thing to hit computing since MS-DOS.” And yet, tools such as the Mono class bring a modern touch to what some may deem outmoded technology. It leverages the power of reactive programming, encouraging developers to create robust, high-performing systems that take full advantage of available resources while simultaneously shoring up usability.
For a deeper understanding of how Mono works within the concept of Reactive Programming, this article might help create a broader perspective.
Please remember that programming languages are tools and like every tool, they come with their unique strengths and weaknesses. Understanding the requirements, scoping the problems, considering the available resources are the keys to choose the right tool for the task. Don’t become attached to tools, be focused on solutions!
Exploring Real-World Examples of Mono Class Application in Java
The Mono class in Reactor Core library (widely used in Spring WebFlux – an integral part of the Spring 5 release) plays a key role in Java reactive programming. It’s capable of emitting only a single value or none at all, rendering it optimal for transforming web requests into responses. Now, we shall delve deeper into its real-world applications.
Real-World Scenarios Of Mono Class In Reactive Programming
In practical Java development scenarios, you may encounter several uses of Mono. Let’s highlight the primary instances wherein utilizing Mono would be particularly beneficial:
Asynchronous API Calls:
Due to its ability to encapsulate a singly returned value from a method call (typically across network boundaries), Mono is utilized for asynchronous API calls.
Let’s consider an example where we call another service in a REST API as follows:
Monoresponse = webClient.get() .uri("/customers/{id}", id) .retrieve() .bodyToMono(Customer.class);
Here,
bodyToMono(Class)
is a WebClient method that materializes body data as a Mono.
Error Handling:
If we expect any error that can occur during runtime such as errors due to API endpoint down, invalid request etc., we handle these scenarios using Mono.
Monocustomer= findCustomer(id).onErrorReturn(new Customer());
Where findCustomer(id) is a method that can return a Mono of type ‘Customer’.
Unit Testing:
In the space of unit testing, particularly when testing methods that involve non-blocking coding patterns, Mono can be valuable. The reason is that it promotes writing cleaner code which then facilitates the testing process.
Consider the following test case as an illustration:
@Test public void checkMono(){ Mono justMono = Mono.just("A"); StepVerifier.create(justMono.log()).expectNext("A").verifyComplete(); }
This Java code snippet demonstrates how StepVerifier, a Reactor Core component, can be applied in tandem with Mono to verify an expected emission in unit testing.
At final notes, the usage of Mono represents one of the various strategies Java developers have at their disposal to manage singular results in an asynchronous manner, thereby enhancing application responsiveness and concurrency capabilities. Even though traditional models of programming may seem more simple at the initial stages, reactive programming provides effective solutions for large-scale systems which are predominantly data-driven and encounter high levels of traffic.
“Learning without thinking is a waste. Thinking without learning is dangerous” – Confucius. This bit of wisdom relates well to the topic on hand because understanding when and why to use Mono can significantly improve your effectiveness as a Java developer.
The Advantages and Possible Limitations of Utilizing Mono Class in Java
The Mono class in Java, commonly used alongside the Reactor Project and Spring’s WebFlux API for reactive programming, offers considerable advantages but is not without its possible limitations. To keep this focused on the relevance of its use, let’s delve into its primary advantages and potential hurdles.
Advancements Offered by Mono Class:
- Non-blocking applications:
Mono
is designed to work with non-blocking back pressure, an essential feature of Reactive Streams. This leads to efficient system resources usage as it minimizes idle waiting time during the execution of requests.
- Error Recovery: Another favorable offering is that Mono provides a streamlined error recovery mechanism. Thanks to its `onErrorReturn` and `onErrorResume` methods, developers can sidestep potential bottlenecks effortlessly.
- Composition of Asynchronous Operations: Bold steps in composite operations are made possible with `Mono::then` method. For combined tasks completion, single responses are more convenient than if you were to deal with multiple publishers.
- Transformative Functional Style: Mono accommodates a functional style of coding with transforming operators like map, flatMap, filter, etc., promotes readability and tight code.
“Good code is its own best documentation.” – Steve McConnell
Potential Limitations of Utilizing Mono Class:
- Steep Learning Curve: Your expertise in conventional procedural or object-oriented programming might not rescue you here. Developers need a paradigm shift since reactive programming requires a different learning curve that initially may seem daunting.
- Debugging Difficulty: Debugging asynchronous code could prove to be somewhat of a challenge due to ‘stack trace hell’. The chain of events in your log file can become strenuously long and difficult to walk through.
- Increased Complexity: Irrespective of how promising it seems, introducing Mono into an existing blocking application potentially increases the complexity of your codebase with architectural changes and can lead to unforeseeable issues.
- Dealing with Errors: Mono tends to swallow exceptions and handle errors by calling alternative paths. Although recoverability is lauded, it sometimes becomes tough to measure how your system behaves in case of failures.
Below is a simple example of Mono class usage:
import reactor.core.publisher.Mono; public class MonoExample { public static void main(String[] args) { Mono.just("Hello Reactive World") .map(msg -> msg.toUpperCase()) .subscribe(System.out::println); } }
This example states the creation of a Mono instance that emits the string “Hello Reactive World”. With the employment of `.just()`, `.map()`, and `.subscribe()` methods, the application capitalizes Mono’s potential, enabling you to envisage what operations could be performed using it.
In an ever-evolving tech world, the advent of reactive programming brought about by the likes of Mono class signifies a significant leap. Yet, as with all tools, the mantra remains to wield them judiciously – intelligently leveraging their strengths while effectively managing limitations.
Let’s delve into the topic of Mono Class in Java and examine its primary role and utility within a development context. Fundamentally,
Mono
is an entity belonging to the Project Reactor universe, functioning as a carrier for single data/element pipeline or error.
Absolute utilization of the
Mono
class comes into play when your code requires handling a single result, which could either be a solitary, encapsulated element or nothing at all – precisely the raison d’etre for
Mono.empty()
. This
Mono.empty()
instance effectively declares a situation of ‘no data’, making it quite the useful tool.
Allow me to illustrate this concept with a simple coding example:
Mono<String> noData = Mono.empty();
From an SEO perspective, consider this: If you’re targeting keywords like “Java”, “Mono Class”, and “Project Reactor”, scattered within the rich tapestry of your textual content, algorithms will likely mark these segments due to their relevance and frequency, thereby accelerating your journey up the SERPs (Search Engine Results Pages).
Here’s a thought to mull over from Marc Andreesen: “In the future, there will be two types of businesses – those on the Internet and those out of business”. Whether it’s utilizing the Mono class in Java or any other aspect of programming, remaining updated and adapting to advancements is the key to survival in today’s digital space.
The shroud regarding Mono class in Java should be lifting now, paving the way for understanding not just its definition but also its deployment within various conceivable contexts. You’d find it particularly valuable when your programs necessitate single-result-based operations, expanding your repertoire of optimized coding techniques in Java.Project Reactor
This insight into
Mono
, part of the larger Project Reactor universe, should equip you with greater understanding and prime you for efficient use of this singular resource in your Java adventures.