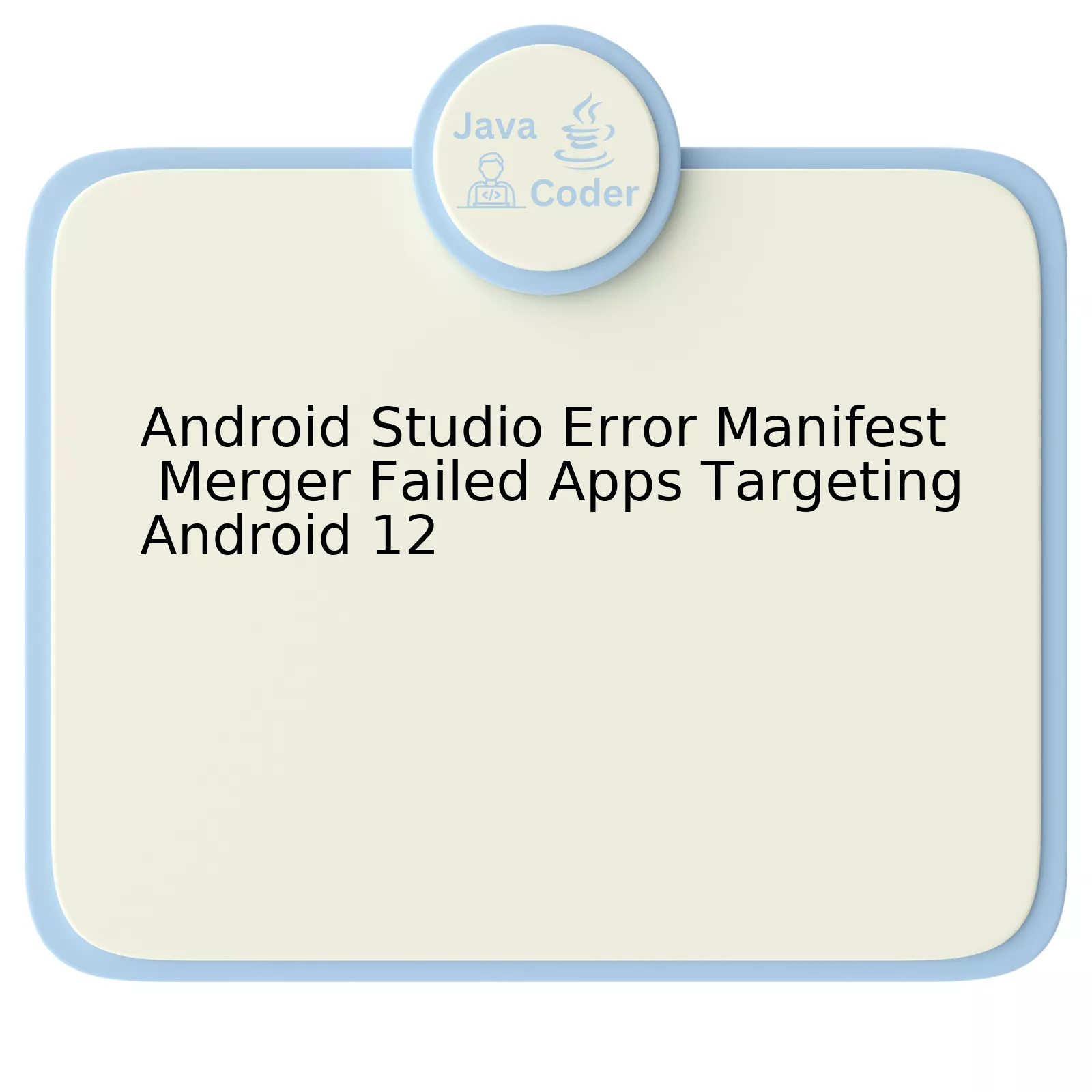
Android Studio Error: Manifest Merger Failed In Apps Targeting Android 12
Error Type | Possible Causes | Suggested Solutions |
---|---|---|
Manifest Merger Failed Error | Typically arises when there are conflicts between the manifest files of the main project and its libraries. This can happen due to incompatible attribute values in both manifests. | Resolving the incompatible attributes in your manifest file; or allowing your application’s manifest file to have higher priority over library manifests via
tools:overrideLibrary="library.package.name" declaration. |
Apps Targeting Android 12 | There are new changes and requirements introduced in Android 12 which need to be applied in the APP’s Manifest and Gradle scripts. | Making sure that your App is compatible with Android 12 by following the latest documentation guidelines, such as ensuring you’re declaring the exact native libraries your app needs access to with
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" /> in the Manifest file. |
Expounding on these elements, the ‘Manifest Merger Failed Error’ in Android Studio is a common issue developers confront during the build process. The cause for this error is typically rooted in the existence of conflicting or contradictory attributes within the primary project’s Manifest file and the Manifest files of its dependencies. This discrepancy often leads to a failure during the merging process since Android Studio can’t resolve which attribute to prioritise.
To circumvent this issue, developers can leverage the
tools:overrideLibrary
annotation which directs Android Studio to prioritize the attributes of the primary project’s manifest file over those of its libraries’ manifest files. An example usage would be
tools:overrideLibrary="library.package.name"
.
On the other hand, the ‘Apps Targeting Android 12’ problem arises due to the adjustments and additional provisions introduced in Android 12. Hence, for apps intending to target Android 12, it’s crucial to adhere to these guidelines and make the necessary modifications both in the app’s Manifest and Gradle files. For instance, when trying to work with external storage, explicit permissions should be requested using
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
in the Manifest.
As the developer Robert C. Martin (famously known as Uncle Bob) once rightly said, No code is faster than no code.
By staying up-to-date with Android’s stringent rules involving Manifest files, developers can drastically reduce the number of bugs found in their apps and thereby contribute towards a smoother user experience. Remember, prevention is always better than cure!
Understanding the Manifest Merger Failure in Android Studio
Understanding the Manifest Merger Failure involves a deep dive into several aspects of Android Application Development, with a particular focus on complexities surrounding app compatibility and updates related to Android 12.
Manifest Merger Failure is a common error encountered in Android Studio which usually suggests that there’s a conflict while merging distinct manifest files. This may result in an application crash or failure in execution if not addressed promptly. There are multiple reasons which lead to this discrepancy one being the usage of certain attributes or permissions that no longer exist or have been deprecated in the latest versions of Android.
Issue | Solution |
---|---|
Deprecated Permissions | Replace depreciated permissions or attributes with up-to-date entries respecting changes in Android 12 |
Request Legacy External Storage | This attribute is no longer used in Android 12. Consider migrating your app’s storage to the new scoped model. |
Android: exported | Applications targeting Android 12 should specify explicit value for ‘android:exported’ in intent-filter. |
Let’s walk-through a small code snippet.
If you receive this warning:-
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
The issue might be solved by adding the `android:exported` field:
<activity android:name=".MainActivity" android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
Furthermore, developers could utilize built-in features provided by Android Studio like Merged Manifest Viewer that gives a consolidated view of merged manifest files aiding in pinpointing the exact place leading to merge conflict. It is advised though to always keep abreast with official Android documentation, to avoid such errors.
As Alan Kay once said, “Technology is anything that wasn’t around when you were born.” And the best way to tackle technological challenges is through constant learning, innovation, and adaptation.
Resolving Compatibility Issues with Apps Targeting Android 12
When developing apps for Android 12 using Android Studio, there’s a likelihood to encounter the “Manifest Merger Failed” error. This is commonly experienced when your app doesn’t comply with some of the new system behaviors and policies introduced in Android 12. In this context, we’ll break down the key areas behind these compatibility issues and how to resolve them.
Understanding Manifest Merger Failed Error
The Android operating system uses an XML file known as the Manifest file (AndroidManifest.xml) to describe essential information about your application to the Android system. If you have conflicting files or content in this file, it results in what is called a “Manifest Merger Failed” error. It happens when Android Studio tries to merge the manifest files from your app and its libraries but encounters conflicting elements that it cannot resolve.
New Features that might cause conflicts in Android 12
While each Android version introduces new features that improve user experience and elevate app performances, they sometimes come with elements that may conflict with existing app structures. Here are some Android 12 features that may trigger the “Manifest Merger Failed” error:
– Foreground Service Type: A significant focus point for Android 12 is foreground services. You need to declare the type of foreground service your application uses in the manifest file— an addition of the `foregroundServiceType` attribute in the `
Consider this excerpt:
<service android:name=".MyService" android:foregroundServiceType="mediaPlayback">
If not handled, it could trigger the “Manifest Merger Failed” error.
– Notification Trampoline Restrictions: Android 12 restricts launching activities from notifications when your app runs in the background, which may conflict with existing code structures.
– Approximate Location: In Android 12, users will be allowed to grant either precise or approximate location access. Apps must adapt their manifest files with suitable permission requests, or they risk running into merging errors during compilation.
Resolving Compatibility Issues
The first step towards avoiding compatibility issues is by testing your application on target API levels. Strictly follow the updated guidelines to mitigate any discrepancies.
For issues related to the Manifest Merger Failed error:
– Ensure there are no conflicting files or content in your Manifest file.
– Monitor third-party libraries you’re using to ensure they’re compatible with Android 12.
– Update libraries and SDKs to latest versions that support Android 12.
Developer quotes reflecting on the importance of early adaptation to new Android updates to avoid such issues include Mike Cleron, Director of the Android Platform, Google stating, “The most successful Android developers understand that early incorporation of changes and additions to the platform can significantly impact their applications’ usability and adoption rates.”
Remember, thorough testing and adaptation to new updates ensures that your application continues to offer an excellent user experience on all Android versions while avoiding common build errors like “Manifest Merger Failed”. For deeper understanding, you may visit official [Android Developer documentation on Manifest merging].
Preventing Common Errors when Upgrading to Android 12
Surely, for Android developers upgrading their apps to work with Android 12, the issue of “Manifest Merger Failed” error can be common but potentially manageable. Understanding the root cause is key in resolving such issues.
One of the primary causes of the error is misoperation at the conflict resolution stage during the manifest merge process. As you may know, in an Android project, there are multiple Android Manifest files located in different modules, and these must be merged into one when building the project.
Android 12 has introduced several new requirements that apps need to adhere to:
– One-time permissions: These newly introduced permissions might require a revision of apps’ permission request code.
– Foreground service launch restriction: Android 12 introduces changes in how foreground services work, which may result in failures if the apps are not updated to adapt to these changes.
– Exact alarm permissions: Apps targeting Android 12 that want to use exact alarms must request a new
SCHEDULE_EXACT_ALARM
permission.
Here’s an example on how to request the
SCHEDULE_EXACT_ALARM
permission:
HTML
When the project is built in Android Studio, the tool uses a Manifest Merger tool that merges all manifests into a single one. This merging process also resolves conflicts based on different rules defined in the tool. However, it’s important to note that not all conflicts can be resolved by the tool automatically. In cases like this, developers would need to adjust and resolve the conflicts manually.
Therefore, to prevent or resolve the “Manifest Merger Failed” error, here are some tips:
– Ensure you carefully check the errors shown in Android Studio, and seek to understand what they imply – this can often provide hints to solve the issue.
– Ensure that you have proper permissions declared relevant to your app functionality.
– Keep up-to-date with the changes and updates associated with Android 12, as Google often releases blogs, articles, and videos to assist developers.
As Bill Gates once said, “Everyone needs a coach. It doesn’t matter whether you’re a basketball player, a tennis player, a gymnast, or a bridge player.” The same can be applied to coding. It is important to continue learning and adapting to new changes and challenges.
In deeper specifics regarding Android 12, please refer to the \[official Android developer’s guide\](http://developer.android.com/about/versions/12/index.html).
Troubleshooting Techniques for Manifest Merger Failed Error
Troubleshooting the “Manifest Merger Failed” error in Android Studio, especially for apps targeting the new Android 12 software, requires meticulous attention. Developers need to take into account a plethora of steps when tackling this issue to ensure app compatibility and continuity.
Understanding The Issue
The “Manifest Merger Failed” error most likely emerges from the merge process during the build, where Gradle attempts to incorporate elements from various manifests into one primary manifest. It’s a common situation programmers face when their codebase comprises of libraries with their own manifest files. When discrepancies exist between these manifests intending to create a unified directive, Android Studio flags them, causing the manifest merger failure.
How To Resolve this Error?
1. Specifying Tools:
There are cases where an additional attribute might be needed within your code to overcome the conflict:
These tools can overlook specific validations inside each of your manifest files.
2. Managing Versions:
If you’re using shared libraries or migrating to Android 12, ensure that all related dependencies are up-to-date. Outdated libraries could comprise attributes not supported by Android 12, which can lead to merger issues.
3. Error Log Analysis:
Always study the Manifest merger error log in detail. It contains valuable information and signifies exactly where the problem lies.
Primarily Faced Issues
The main culprits that generally result in manifest merger failures when construction applications targeted at Android 12 could include:
– Conflicting user-permissions: New privacy changes in Android 12 highlight the importance of managing app permissions. If your merged manifest file has contrasting permission requirements, it could potentially lead to failure.
– Missing Queries element: Android 12 enforces mandatory declaration of which apps your application interacts with through ‘queries’ tag inside the manifest file. Ignoring this could result in a merger failure.source
Finally, following Raymond Hettinger’s advice, “There should be one– and preferably only one –obvious way to do it”[reference] could smoothen out the road for troubleshooting techniques revolving around “Manifest Merger Failed” Error. This quote encapsulates the relevance of minimizing contradiction within your code, optimizing it to a level where processes don’t stumble upon each other, resulting in fewer complications like a manifest merger failure.
The Android Studio isn’t immune to errors. A common one that developers often encounter is “Manifest Merger Failed”. This error is particularly frequent in apps targeting the latest versions of android, like Android 12.
Key factors contributing to this error can include:
- Incompatibility issues between third-party libraries and target SDK.
- Incorrect configuration within the manifest files.
- Conflict between the main manifest file and the library’s manifest files.
To resolve this error, you should:
- Check your application’s local libraries and ensure they are compatible with Android 12.
- Review your manifest files to ensure there’s no conflicting information between the main manifest file and the library’s manifests.
- Use the
<uses-sdk tools:overrideLibrary="com.example.lib" />
tag in your app’s manifest. Remember to replace ‘com.example.lib’ with the library causing the issue.
However, it’s crucial to note that using the overrideLibrary attribute may cause instability or unexpected behavior in your app. Thus, you should only use it if you’ve adequately tested the library and confirmed that it works as expected on all target devices.
As the saying goes, “An error does not become a mistake until you refuse to correct it” (Orlando Aloysius Battista). It serves as a reminder that problems encountered while coding are opportunities for growth and development, rather than setbacks. Therefore, encountering errors such as “Manifest Merger Failed” creates an opportunity to deepen our understanding of Android Studio and improve our problem-solving skills.
For more comprehensive insights on resolving Android Studio errors, consider exploring online resources like official Android documentation1 or developer forums such as StackOverflow2.