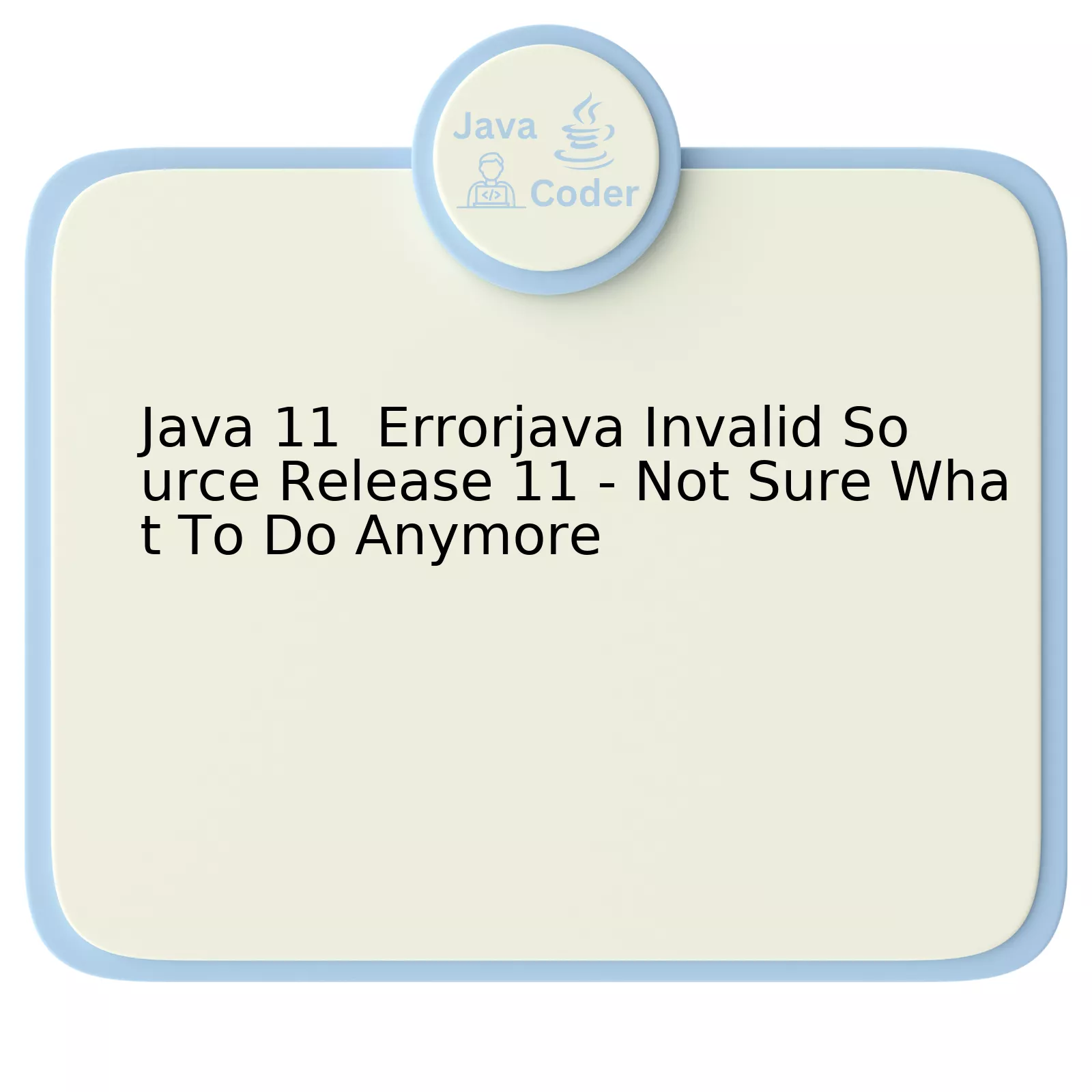
Java 11 Error – Invalid Source Release 11 is a common issue that users may encounter when trying to compile their Java files with a lower version of Java while having set a higher version like Java 11 in the source and target of their maven or Gradle build files. This error message indicates that the system does not support the specified source release.
Take a look at the following table which explains how this problem generally arises:
Factor | Description |
---|---|
Java Compiler Version (Javac) | The javac tool in your System PATH might not match the JAVA_HOME environment variable. The javac tool used depends on its order in system path, and it’s possible an older version might be getting picked up instead. |
Maven/Gradle Configuration | If using Maven or Gradle for building your project, source and target Java version in the POM.xml or build.gradle file might have been specified as 11, while your system only supports a lower Java version. |
Incorrect JAVA_HOME configuration | Your system’s JAVA_HOME environment variable is linked to incorrect Java version, or hasn’t been set at all. |
To rectify “Invalid Source Release 11” error, you should ensure compatibility between the Java versions of the development environment and the build tools. Don’t forget to update the JAVA_HOME environment variable to point to correct Java Development Kit (JDK) version if you’ve upgraded it.
Let’s examine a solution snippet:
//For UNIX-based systems export JAVA_HOME=/path/to/jdk-11 //For Windows set JAVA_HOME="C:\path\to\jdk-11"
Then, ensure the defined Java version matches what is specified within your Maven or Gradle build configurations. For example,
<!-- pom.xml – For Maven --> <properties> <maven.compiler.source>11</maven.compiler.source> <maven.compiler.target>11</maven.compiler.target> </properties> //build.gradle - For Gradle compileJava { sourceCompatibility = '11' targetCompatibility = '11' }
In the words of Bill Gates, “Software innovation, like almost every other kind of innovation, requires the ability to collaborate and share ideas with other people, and to sit down and talk with customers and get their feedback and understand their needs”. Fixed by taking these steps, it demonstrates that collaboration between software and its user is key to successful problem-solving.
Understanding the “Errorjava Invalid Source Release 11” in Java 11
The “Errorjava Invalid Source Release 11” message typically happens when you’re compiling your Java code with a different JDK version than you initially targeted. This is particularly prevalent in environment settings where multiple Java versions are installed.
When programming with Java 11, the compiler requires accurate source values. The Java compiler translates your program’s source code into bytecodes that can be relayed to the Java Virtual Machine (JVM) for execution. Errors like “Invalid source release 11” suggests an issue with these settings.
Here are steps to address this error:
- Check your Environment Variables
Environment variables are system-wide values that provide crucial information about your operating system and other software to applications. A common mistake may be due to having a PATH variable that points to an out-of-date JDK. Also verify if you set JAVA_HOME correctly.
C:\Users\YourUsername>echo %JAVA_HOME%
C:\Users\YourUsername>echo %PATH%
- Correctly Set Java Version
It would help to check and confirm the Java version associated with javac command via the terminal as follows:
javac -version
If the output reflects a different version, you ought to reconfigure your IDE or build tool (like Maven or Gradle) accordingly.
- Update Your IDE Settings
Most popular Integrated Development Environments (IDEs) like IntelliJ IDEA, Eclipse, or NetBeans will let you specify the JDK for each project. Kindly ensure that the Java version selected matches the one you plan to use.
- Changing the Compiler Compliance Level
Fortunately, most IDEs offer the feature of setting a “Compiler Compliance Level.” If correctly adjusted, it matches the source code with the appropriate Java version, thus preventing such errors.
In addition, Eric Raymond, the well-known open-source advocate and programmer, once observed, “Given enough eyeballs, all bugs are shallow.” Indeed, overcoming such technical issues often just means carefully checking our configurations and ensuring they align with the requirements of the software we’re using. As Java developers, comprehending the meaning behind compilation errors is key to writing effective and efficient code.
For additional perspectives, look at StackOverflow threads addressing similar issues. The community can often offer practical advice based on their experiences. this thread could be particularly valuable on understanding ‘source’ in javac.
Analyzing Potential Causes for “Errorjava Invalid Source Release 11”
Understanding the “Errorjava Invalid Source Release 11” in the context of Java 11 requires a deep dive into certain technical aspects of Java.
This error typically appears due to multiple potential causes such as JDK (Java Development Kit) misconfiguration, build tool issues, or IDE (Integrated Development Environment) compatibility problems. Below are some explanations and solutions to look into:
Incorrect JDK Configuration
The error could be related to the version of JDK installed on your machine against which you’re trying to compile your code. If you’re targeting a higher release than what’s available on your system, this error might surface. For instance, if you have JDK 10 installed but writing code with features specific to Java 11, the compiler would throw an “invalid source release” error. Hence, always ensure to check that your JDK configuration is accurate.
To verify your JDK configuration, use the command-line interface and type:
javac -version
In case you find discrepancy, download the appropriate JDK version from the official Oracle website.
Build Tool Misconfigurations
If you’re using a build automation tool like Maven or Gradle, ensure it’s pointing at the correct Java version. They generally rely on JAVA_HOME environment variable specifying the path of JDK installation. The pom.xml (for Maven) or the build.gradle (for Gradle) files should be configured correctly to target the Java version being used.
For Maven, modify the
maven.compiler.source
and
maven.compiler.target
parameters in the pom.xml file to match your JDK version. For example:
<properties>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
Similarly, for Gradle, change the sourceCompatibility and targetCompatibility options in the build.gradle:
sourceCompatibility = '11'
targetCompatibility = '11'
IDE Compatibility Issues
Your IDE, whether it’s IntelliJ IDEA, Eclipse, or NetBeans, may not be compatible with Java 11. Check if you’re running the updated IDE version that supports Java 11. In IntelliJ, you can fix it by going to Project Settings > Project > Project language level and selecting the appropriate SDK version.
Remember, as Tim Berners-Lee, inventor of the World Wide Web, said: “We need diversity of thought in the world to face the new challenges.” Similarly, navigating through such errors requires you to think from different perspectives, whether it’s checking the JDK version, verifying the build tool configurations or the IDE compatibility.
Practical Troubleshooting Techniques for “Errorjava Invalid Source Release 11”
The “Errorjava Invalid Source Release 11” typically emerges when your system doesn’t recognize Java 11 as the default environment. This error could be caused by several factors such as using a different version of JDK (Java Development Kit) than JRE (Java Runtime Environment), or your IDE not recognizing Java 11.
If you’re dealing with this issue, don’t lose hope. The following troubleshooting techniques can assist in resolving this frustrating error:
Making sure you have JDK 11:
The first step is to validate that your development environment has JDK 11 installed. If unsure, you can do so with the following command in your terminal:
javac --version
If this command outputs a version other than 11, consider installing JDK 11 and re-run the command to ensure installation.
Check Java Home Path:
Proper setting of JAVA_HOME path is crucial. An incorrect path can create conflicts between various versions of Java on your machine leading to instances of “Errorjava Invalid Source Release 11.” To verify your JAVA_HOME, use:
echo $JAVA_HOME
Ensure it aligns with your actual JDK 11 directory path.
Update Your IDE:
Sometimes, an outdated Integrated Development Environment (IDE), may not fully support JDK 11 leading to this error. Updating your IDE might fix the problem.
Manually Configure Your IDE:
You would need to manually set your IDE to use JDK 11. Because each IDE is structured differently, refer to its specific user manual for detailed guidance.
Ensure You Are Using Correct Build Tools:
Tools like Maven or Gradle often come entrenched in predefined settings. Confirm your build tools are also using JDK 11.
According to James Gosling, the creator of Java,
“Most programming languages have their little areas where they’re particularly strong… But Java has been incredibly useful just about everywhere. I’ve never seen a single computer architecture that couldn’t benefit heavily from Java.”
So let’s follow his advice, make optimal use of Java by resolving these common errors.
You can find more information on how Java expect certain commands to run in Java 11 here.
In all, the “Errorjava Invalid Source Release 11” error message is resolvable with some keen troubleshooting steps including validating JDK 11 installation, verifying the JAVA_HOME path, ensuring your IDE supports Java 11, configuring your IDE, and making sure right build tools are being used.
Future-Proof Solutions and Prevention Methods for “Errorjava Invalid Source Release 11”
When you face a situation that states that “error java invalid source release 11” is displayed, there are several avenues to calibrate the situation. This error typically comes up when your Java compiler doesn’t support or can’t comprehend the Java version specified in your project setup.
Error Analysis
The phrase “Invalid Source Release 11” indicates that the targeted Java version in the project configuration surpasses what the current Java environment supports. It could be because the Java Development Kit (JDK) of an earlier version is trying to compile a program written in a subsequent version, Java 11 in this case.
Using JDK 11 to compile will eradicate this warning. If the environment demands that you carry on using an older version of JDK, then shifting source compatibility of your project to correspond with the lower JDK version being utilized could also rectify this.
Solution Paths
Update Project Configuration
Check your project setup and confirm the JDK version it’s set up to use. Altering the source compatibility level inside the build configuration tool—like
Maven
or
Gradle
—to liken it to the installed JDK version should get rid of the error. For instance, in Maven, you should specify the maven.compiler.source property.
For Maven:
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
Upgrade JDK Version
If possible, upgrade the JDK in your environment. You can accomplish this by downloading the new JDK from the Oracle website. After installing the new version, add it to the PATH environment variable to reflect the change in your terminal window.
Remember that Java is backward compatible. Hence, if you have upgraded the JDK to 11, it can still run applications compiled with a previous version.
Prevention Methods
Stay current
Having the most recent JDK would prevent occurrences of such errors in the future. The art of staying ahead involves being at par with the newest software development tools, especially those around the programming language you focus on.
Continuous Assessment of Environments
A regular check on the configured JDK against the required JDK version for each project aids in maintaining consistency. These checks include understanding the updates about depreciated features and migrations of data types, etc.
Practice Compatibility Testing
Before deploying a piece of code, conduct compatibility testing. Perform tests across multiple environments which represent different versions of your SDK.
As Ken Thompson rightly said: “You cannot trust code which you did not entirely create yourself.” Thus, taking preventive actions and understanding your codebase would always pay dividends in the long run.
Dealing with Java 11 Errorjava Invalid Source Release 11 can at first seem overwhelming for any java developer. However, adopting a few strategies will drastically reduce its occurrence and improve your java development experience.
The Errorjava Invalid Source Release 11 is primarily triggered when your system is trying to compile a code with a version of Java that it doesn’t recognize. This usually happens when the wrong JAVA_HOME variable has been set, specifically if the project level JDK differs from the system environmental JDK. Making both JDK versions similar might serve as a fix for this issue.
Furthermore, IDE settings play a crucial role as well. A specific version of Java might be selected in your Integrated Development Environment (IDE) while you’re using a different version for your project. Synchronizing these versions could result in fixing the error.
Additionally, continuously updating your software to the latest version of Java is critical. In the case you encounter an unrecognized source error following a Java update, it may be due to the older ‘javac’ compiler trying to build the newer Java files. To resolve this situation, ensure the path is correctly set, pointing to the latest JDK bin directory.
Here’s an example illustrating how to set the JAVA_HOME:
html
export JAVA_HOME=/path/to/your/jdk export PATH=$JAVA_HOME/bin:$PATH
In the above code piece, /path/to/your/jdk should be replaced with the actual location where the new JDK version is installed.
Remember, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler. One key element of resolving such errors lies in thoroughly understanding what each line of your code does, especially when dealing with Java versions and environments.
Finally, always check out the official Java 11 documentation to better understand common errors and get solutions directly from the official source. Applying these practices should make the task of handling the ErrorJava Invalid Source Release 11 much more manageable.