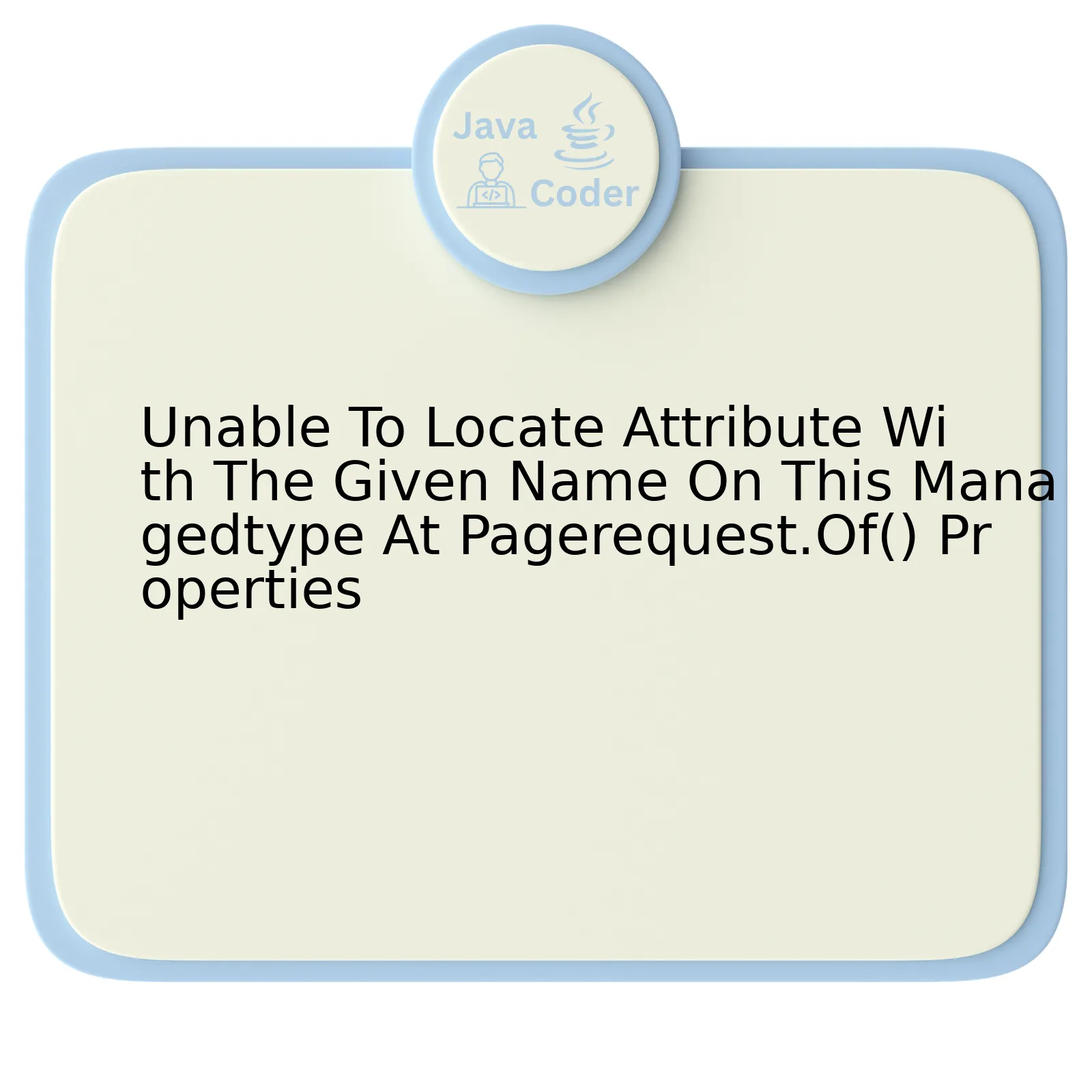
The issue with the “Unable To Locate Attribute With The Given Name On This ManagedType At PageRequest.Of() Properties” mostly arises from invalid references to attributes in JPA (Java Persistence API) queries within a Java application. The following table illustrates the various elements pertinent to this problem:
Problem Element | Description |
---|---|
Error Message | “Unable To Locate Attribute With The Given Name On This ManagedType” |
Cause of Error | Typically caused by misspelling an entity attribute or referencing a non-existent attribute in a filter or sort specification in PageRequest.of() method. |
Impacted Area | JPA Query, PageRequest.of() properties |
Solution | Check the entities’ attributes being referred to in queries and ensure their validity. Refactoring might be required if the attribute does not exist. |
The error message indicates that the attribute specified in the JPQL (Java Persistence Query Language) cannot be found on the ManagedType (the JPA representation of the entity). This could occur in the usage of
PageRequest.of()
method where an invalid attribute is specified for sorting data.
The primary cause of the problem usually lies in the typing errors when writing attribute references within the query or the reference to a non-existing attribute. Precisely, the error surfaces during the referencing of attributes in the fields of the
PageRequest.of()
method’s parameters for filtering or sorting the data.
For troubleshooting this error message, analyzing the JPQL query or the parameters of the
PageRequest.of()
is important. Cross-verification of the entity attributes against the ones referred in the methods ensures accuracy and minimizes potential errors.
Resolving this requires rectifying the incorrect attribute references present in the JPQL query or the parameters to match the actual attribute names in the Java entity classes. If the attribute doesn’t exist, refactoring the code would be necessary.
As Kent Beck, a renowned software engineer once said, “Make it work, make it right, then make it fast.” These words resonate particularly well when troubleshooting coding problems. Thorough analysis, step-by-step correction, and constant refinement usually lead to effective code solutions which are free from persistent bugs like these.
Understanding the Error: Unable to Locate Attribute on ManagedType
In the context of developing with Java, understanding an error such as ‘Unable to Locate Attribute on ManagedType’ often proves crucial for developers. Specifically, this issue occurs when a ManagedType cannot identify a particular attribute.
The attributes map to the fields/properties within your Java classes that have been annotated as being part of the persistence layer. This includes annotations like
@Id
,
@Column
, etc. And the ManagedType is the representation of these persistent entities at runtime in the JPA Metamodel.
This error can occur due to several reasons:
– The attribute may not be present in the class or superclass inherited properties.
– The attribute might be private and inaccessible.
– Attribute name passed to the
attribute(…)
method could be wrong.
– The annotation defining the persistence mapping might be incorrect or missed, so JPA does not recognize it.
– Mismatch between names or case sensitivity issues in both Java code and data persistence layer.
To rectify the error ‘unable to locate attribute with the given name on this managedtype at pagerequest.of()’, you should first check if the entity’s attribute is correctly defined in your Java class code. Make sure the attribute is public, or there are relevant getters and setters available. If the attribute is inherited from a superclass, ensure correct visibility and accessibility.
For example, considering below code sample:
Code Sample |
---|
@Entity public class MyEntity { //... @Column(name = "my_attribute") // Matches column name private String myAttribute; // Make sure attribute visibility public String getMyAttribute() { return myAttribute; } public void setMyAttribute(String myAttribute) { this.myAttribute = myAttribute}; } |
In this code, we define an attribute
myAttribute
mapped to a column named
my_attribute
. Additionally, we have a getter and setter for this attribute ensuring its accessibility.
Additionally, cross-check your database schema to verify if the attribute matches with the corresponding field in the database table. Verify if all your entity classes are scanned and correctly loaded by your persistence provider.
As quoted by Robert C. Martin (Uncle Bob), “Truth can only be found in one place: the code”. Therefore, deep-dive into your Java code, scrutinize the SQL queries, and ask critical questions to understand and resolve this error. Following these steps will keep your development process fluid and efficient, delivering high-quality software.
Delving into PageRequest.of() Properties and Their Role in Entity Management
While working as a Java developer, you might’ve come across the
PageRequest.of()
method in Spring Data JPA which is quite helpful for implementing pagination and sorting of data. However, you might also have faced an issue where you’re unable to locate attribute with the given name on this managed type at
PageRequest.of()
properties.
The
PageRequest.of()
function essentially creates a new
PageRequest
with sort direction and properties. The arguments it usually takes include:
– The page number (zero-indexed)
– The size of the page
– The
Sort
instance which determines the direction and properties for sorting
So when the error “unable to locate attribute with the given name on this managed type” occurs, it’s most likely due to incorrectly defining the properties while making the
PageRequest.of()
call.
Here’s how a typical use would look like in your entity management:
Pageusers = userRepository.findAll(PageRequest.of(0, 20, Sort.by("name")));
In this example, you’re asking for the first page of users, with 20 records per page, sorted by ‘name’ field of ‘User’ entity. If the ‘name’ field doesn’t exist in your User entity, this will throw “unable to locate attribute with the given name on this managed type” error.
A way to fix this error is to ensure that the property you’re using for sorting exists in the entity class.
To put it succinctly, always cross-check that your sort properties align correctly with the fields defined in your entity class.
As technology pioneer Tim Berners-Lee rightfully said, “We need diversity of thought in the world to face new challenges.” Hence, embrace such issues as learning opportunities to reinforce your role and skills as a Java developer.
Strategies to Avoid Incorrect Attribute Naming in ManagedType
Navigating the realm of attribute naming in ManagedType without falling victim to incorrect terminology can be a tricky expedition. An encounter with an exception like “Unable to Locate Attribute with the Given Name on This ManagedType at PageRequest.of() Properties” may leave one scratching their heads.
Clear strategic measures are an imperative path towards avoiding such conundrums:
• Adopting Standard Naming Convention: A cornerstone principle in any programming entity is following uniform conventions for attribute naming in ManagedType. Java typically follows the pattern
private DataType attributeName;
. Such standardization helps in reducing errors and maintaining code readability.
• Ensuring Existence: It seems rudimentary, but developers often fall into traps when they reference attributes that don’t exist within the ManagedType. Check if the attribute name in question indeed exists and matches the referred name case-sensitively.
• Adherence to Language Syntax: If adopting Java’s ManagedType, it’s paramount to stay within the bounds of the language’s syntax rules, paying close attention to variable naming (attributes should start with lower-case letters and camel-case for other words) to avoid trouble.
• Checking Values at Runtime: In Java, the
Attribute super X, ?> attr = metamodel.attribute(name);
method tries to locate an attribute based on its name. An error will be triggered if there’s no attribute with that name. To counter this, use the
hasAttribute(String name)
method to verify the attribute’s existence before calling it.
An insightful quote by Linus Torvalds amplifies the importance of attention to detail in any code writing activity:
*”Talk is cheap. Show me the code.”*
Whether you’re dexterously navigating the challenges of attribute identification in ManagedType or any other programming hurdle, your code speaks volumes about your clarity and strategy. Hence, placing mindful focus on attribute naming from the get-go could save development hours that might be otherwise lost debugging elusive Not Found exceptions.
You can further refer to GeeksforGeeks article on Java’s naming conventions and look out for more insights from Oracle Documentation.
Deep Dive into Attribute Handling within a Managed Type
Managed types in Java refer to the classes that are managed by a persistence context. The problem stated is related to an instance where an attribute within a Managed Type cannot be found through the `PageRequest.of()` properties. This could occur if the specified attribute does not exist or is not accessible in your class. To understand this further, let’s delve deeply into Attribute Handling within Managed Types and how it can be linked to the error presented.
Primary reasons for the inability to locate an attribute could be due to:
- Attribute Misnaming: Here, you might have specified an attribute name that does not match with any of those present in your Managed Type. A code snippet sample of a correct naming practice is as follows:
// Correct way to denote an attribute public class YourClass { // Attribute Declaration private String attributeName; }
- Inaccessible Attribute: If your attribute is not public or accessible due to its scope like being defined in a private inner class or packaged private class, then this issue could arise.
For instance,
// Unrecommended attribute declaration private class YourNestedClass { private String inaccessibleAttribute; }
Hence, make sure your attribute is accessible or has necessary getter methods available for proper access.
Secondly, taking heed of providing explicit mapping of your attributes with JPA columns using Annotations (like `@Column`, etc.) can assist in the resolution.
The `PageRequest.of()` method creates a new PageRequest object which indicates information about sorting, pagination parameters, etc., expected from your database request. In case the attribute does not exist or isn’t handled properly, it throws an error.
As per James Gosling, creator of Java, “Doing software updates is always a balance among various things, including maintaining backward compatibility, necessity for improvement, crystal ball gaze into the future, and resources at hand.”
Although seemingly unrelated, this quote rings true for this situation. As developers, we should constantly make sure our code is bug-free, maintainable, while striving for continuous improvement, especially when dealing with Managed Types in Java. This deep understanding and careful handling of attributes within a Managed Type will resolve the issue at hand and aid in optimizing your application performance.
The situation of being “unable to locate attribute with the given name on this ManagedType” at
PageRequest.of()
properties in Java hints towards the misalignment between the underlying database schema and the Java entities set up via JPA and Hibernate. These pesky issues could stem from various reasons, within which, I will walk you through.
“Remember that all models are wrong; the practical question is how wrong do they have to be to not be useful.” – George E. P. Box
- Misspelled Attribute Names: Cross verifying your entity declaration in Java Business Object against the actual field name in the database could reveal spelling mismatches.
- Case Sensitivity: Coding languages treat UpperCamelCase and lowerCamelCase as separate attributes. A small oversight here could cause the said error.
- Deployment Issues: The application may use an outdated deployment descriptor or an old version of the java persistence annotations.
- JPA/Hibernate Integration: The aforementioned problem sometimes is a product of incorrect configuration of the JPA provider or misuse of Hibernate.
To troubleshoot this issue, you should first check if any of the potential problems above hold true for your case. If they do, adjusting your code or settings to realign with the expected setup should eliminate the error. Notably, you need to make sure that the attribute names and their usages, both direct and indirect (like in derived queries), should map exactly with what has been declared in your Java objects or database schema.
Here’s a basic example of troubleshooting:
//Check if database column name matches the JPA entity attribute. class User { @Id private long id; @Column(name = "username") //The column name must match exactly in case-sensitive manner. private String userName; }
Above snippet demonstrates a simple validation approach where you compare your entity attribute (userName) with its counterpart in the database. A typical mismatched case scenario would be having
"USERNAME"
or
"UserName"
as the column name in the database instead of
"username"
. This would result in the said error.
If the issue extends beyond simple fixes, consider digging deeper into the integration setup for your JPA provider or ORM framework. Moreover, upgrading to more recent versions might solve the issue if it was caused by bugs inherent to older versions of the software.
Being equipped with the understanding of causes and resolution to such prevalent intricacies will enable you to overcome these hurdles with poise. And thus ensuring the smooth implementation of the data access layer in your applications.