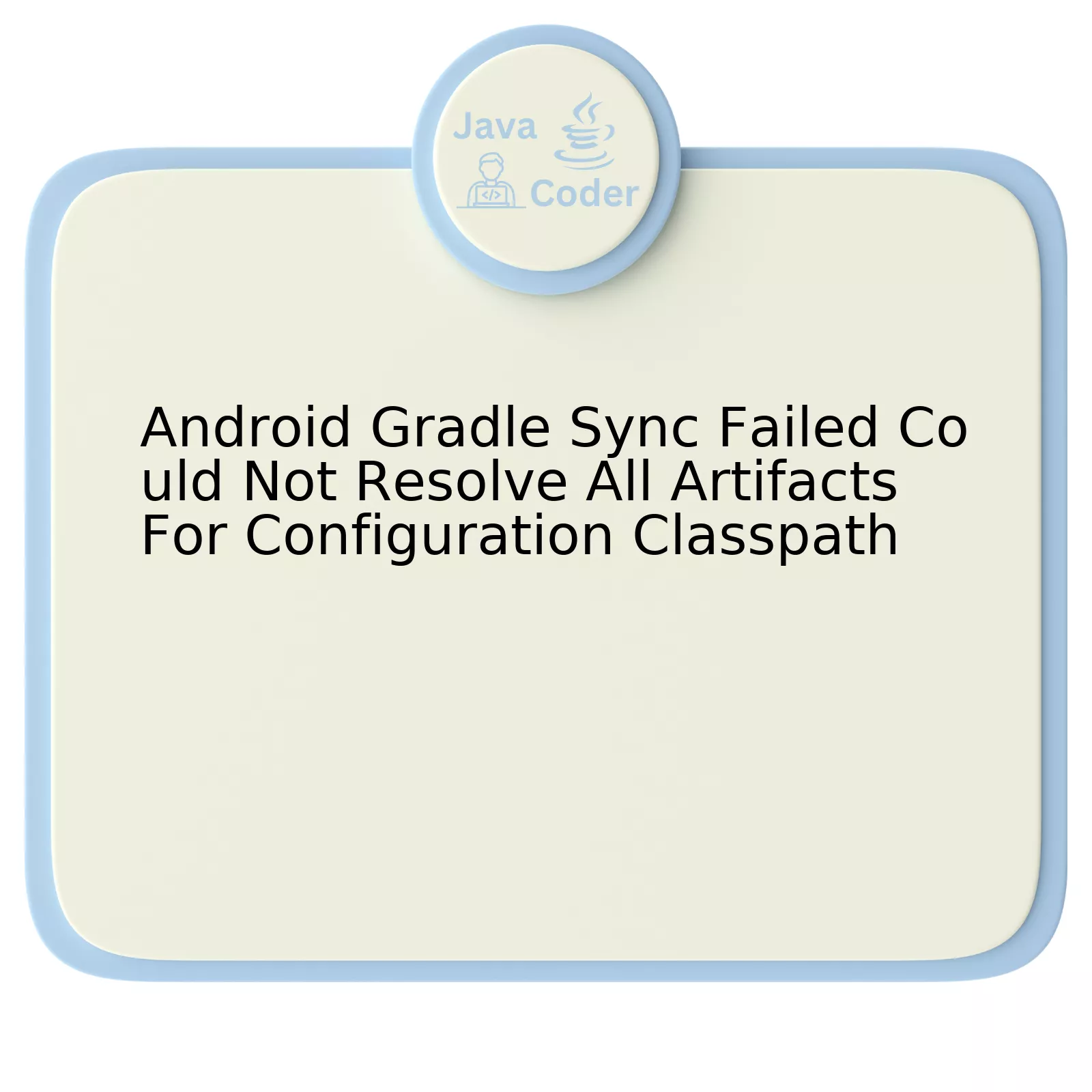
The error message “Android Gradle Sync Failed Could Not Resolve All Artifacts For Configuration Classpath” can happen due to several reasons. It can be incredibly frustrating as it prevents you from syncing your project correctly using Gradle in an Android environment. The following table illustrates the potential causes and solutions for this problem:
Possible Causes | Proposed Solutions |
---|---|
Network Connectivity Issues | Ensure a stable internet connection is available. |
Incorrect Gradle Version | Update or downgrade the Gradle version to match the requirements of the Android Studio version being used. |
Incompatible Plugins | Check for plugin compatibility issues, update or remove incompatible ones. |
Corrupted Gradle Cache | Clear the Gradle cache by deleting the .gradle directory within the project or home directory. |
Unresolved Dependencies | Make sure all dependencies declared in the build.gradle files are available and resolvable. |
These above-mentioned problems and solutions can help you troubleshoot when facing the error: “Android Gradle Sync Failed Could Not Resolve All Artifacts For Configuration Classpath.”
For instance, if the problem is network connectivity, making sure that your machine has stable Internet access is crucial. Gradle needs to download many dependencies and other essential resources, especially during initial builds.
When it comes to an incorrect Gradle version, it’s important to know that every version of Android Studio works best with a specific Gradle version. You might need to downgrade or upgrade your Gradle version to ensure compatibility.
Sometimes, plugins can be incompatible with either the Gradle version, Android Studio version, or even with one another. Keeping your plugins updated, removing unnecessary plugins, and checking against known plugin incompatibilities can help fix this issue.
Furthermore, the Gradle local cache can become corrupted leading to this synchronization failure. Deleting the .gradle folder in your user home directory or project directory will clear this cache, forcing Gradle to redownload its necessary components, potentially fixing any corruptions.
Next, unresolved dependencies can often cause this issue. Double-checking the specified dependencies in your build.gradle files can solve unresolvable artifact errors.
For a more detailed analysis of each problem and its solution, consider referring to the official Android Developers documentation.
As Frederick P. Brooks famous quote goes, “The bearing of a child takes nine months, no matter how many women are assigned. Many software tasks have this characteristic because of the sequential nature of debugging.” So, the same applies to figuring out such Gradle issues – it requires patience and a systematic approach to debugging.
Understanding the Causes of Android Gradle Sync Failure
The Android Gradle Sync Failure is an error that developers often encounter during their Android development journey. Specifically, when the reported error manifests as “Android Gradle Sync Failed Could Not Resolve All Artifacts For Configuration Classpath”, it indicates that Gradle, Android’s build system, experiences difficulties in obtaining necessary dependencies for your project.
There are several potential causes explaining why Gradle cannot resolve all artifacts for configuration classpath:
– Dependency Versions Mismatch: This issue could stem from mismatched or incompatible versions of the dependencies in your app’s
build.gradle
file. It’s crucial to ensure that all library and plugin versions are compatible with each other and the version of Android Gradle Plugin (AGP) you are using.
– Corrupted Cache: Gradle maintains caches primarily to improve build speed. However, should these caches get corrupted, they can cause Gradle sync to fail. Cleaning the cache can help fix this issue. Simply open the terminal and run the following command inside your project directory:
./gradlew cleanBuildCache
– Broken or Unavailable Internet Connection: Gradle requires internet access to download dependencies. An unstable or broken internet connection would prevent this process, leading to sync failure.
– Inconsistent Gradle and JDK Versions :Java Development Kit(JDK) version must be compatible with the Gradle version; else it leads to sync failure
With the recognition of aforementioned common causes, one quote by Brian Foote seems particularly apropos here: “If a program is useless, it will have to be changed. If a program becomes useful, it will have to be changed.” This simply reinforces the changing and evolving nature of software and codebases. Samsung themselves stated that “software development is more than just writing code.”[source] But rather, it permeates into understanding its tooling infrastructure, debugging and resolving errors which include understanding issues like Gradle Sync Failures.
Exploring Solutions for ‘Could Not Resolve All Artifacts For Configuration Classpath’ Issue
When dealing with the “Android Gradle Sync Failed, Could Not Resolve All Artifacts for Configuration Classpath” issue, there’s an array of targeted solutions you can consider. Remember, a symptom-like failure to resolve artifacts usually surfaces due to discrepancies in dependency versions, project configuration issues, or a flawed cache state that could be wreaking havoc in your development environment.
Adjustments to the Dependencies:
The first tip would involve revisiting your dependencies defined inside the
build.gradle
(app level). The problem might root from usage of outdated or incompatible libraries and SDK versions. If you have used ‘+’ symbol to fetch the latest version of a library, replace it with specific library versions, especially if it’s a SNAPSHOT version.
Example:
dependencies { implementation 'com.google.firebase:firebase-core:16.0.9' classpath 'com.android.tools.build:gradle:3.5.4' }
Invalidate Cache and Restart:
Another approach is to invalidate cache and restart. Since Android Studio utilizes caches to speed up repetitive tasks, the caches may get out of date, corrupted, or synced incorrectly, causing problems like this.
Access the option through:
File -> Invalidate Caches / Restart -> Invalidate and Restart
Change Offline Mode :
If the error persists, ensure that the offline work option under Gradle settings isn’t enabled. Working in offline mode might lead to this error as Gradle cannot download necessary artifacts.
Path for this setting is:
File -> Settings -> Build, Execution, Deployment -> Gradle -> untick ‘Offline work’ box.
Check Your Internet Connection:
This issue might occur because of network restrictions blocking certain URLs or unreliable internet connections. Changing to a stable network or disabling network restrictions could certainly help.
As Robert C. Martin, a renowned software engineer and author eloquently puts ‘”‘Truth can only be found in one place: the code.”‘ To solve complex challenges developers often face, diving deep into the codebase and understanding it truly matters. It’s safe to say then, that in overcoming obstacles like these, adopting a detailed and analytical approach towards code verification will undoubtedly pay off in the end.
These solutions act as key starting points for resolving the error on Android Studio. The recommended actions cater to general causes of the problem, and they should enfuse your Android project back on track. For more details on troubleshooting Gradle artifact issues, visiting the official update guide for Android Studio is encouraged.
Effective Techniques to Troubleshoot Gradle Sync Failures in Android Studio
Gradle Sync failures in Android Studio can pose quite a challenge, especially for beginners. Specifically when the issue is “Android Gradle Sync Failed: Could Not Resolve All Artifacts For Configuration Classpath”, a number of distinct techniques can be utilised to rectify this situation.
dependencies { classpath 'com.android.tools.build:gradle:4.0.1' }
The above code snippet demonstrates how an issue with dependencies could lead to Gradle Sync Failure. The version of Gradle mentioned, might not be compatible with the SDK version you have installed or there may possibly be an issue in accessing the specified URL for fetching the correct version.
Error Indicators | Potential Solution |
---|---|
Internet Connectivity Issues | Ensure that you have an active and stable internet connection as Gradle needs to download dependencies. |
Dependency Versions | Check your dependency versions. Incompatibility between dependency versions often leads to Gradle sync errors. |
Inadequate Resources | Gradle requires sufficient resources (RAM/CPU). Monitor these specifications on your machine, and upgrade hardware, if required. |
Corrupted Cache | Invalid cache entries could cause Gradle to fail. Use the ‘Invalidate Caches / Restart…’ option under File menu of Android Studio, which will clear all caches and restart IDE. |
What’s more satisfying than fixing a problem? Fixing it permanently! Automating the process of detecting commonly occurring issues with given conditions can provide longer term solutions:
allprojects { repositories { mavenLocal() google() jcenter() maven { // URL for dependency } } }
Including default repositories in the project level
build.gradle
file ensures synchronized build configurations across all project modules.
Additionally, implementing Gradle Wrapper could also be considered advantageous. This enables automatic set up of the required Gradle version on every build, without manual intervention.
Remember, as Bill Gates once said, “Software is a great combination between artistry and engineering.” Debugging Gradle Sync Failures tends to appeal more to the engineering side, but remember to keep innovating while you code, adding an artistic touch to your professional approach.
Insights into Preventive Steps: Avoiding Future Gradle Sync Failures
Gradle Sync Failures are common issues that developers run into while developing Android applications. These failures often pose a challenge in compiling and running the application effectively, which might adversely affect the performance of the application. Most commonly, the root cause behind such failures is problems associated with the configuration classpath.
The first preventive measure suggested for avoiding future Gradle sync failures is ensuring a proper internet connection. Gradle needs to download many dependencies from various repositories on the Internet. Therefore, a stable and robust internet connection is crucial.
apply plugin: 'com.android.application' repositories { mavenCentral() } dependencies { classpath 'com.android.tools.build:gradle:3.2.1' }
A second approach revolves around maintaining accurate configurations in the build.gradle files. Developers must validate all specified libraries in the dependencies section using the correct versioning – established through Maven Central or whatever repository used.
An additional technique consists of utilizing the latest versions of Android Studio and Gradle. It is essential for developers to keep their development environment up-to-date because these updates often come with bug fixes and feature enhancements that preemptively remedy typical errors or glitches faced by developers.
Proper familiarity with Proxy Settings when working behind a firewall is also pivotal in preventing Gradle Sync Failures. There are times when developers operate within an organizational network limiting some websites. If Gradle is unable to reach required sites to fetch dependencies, failures ensue. Thus it’s important to verify proxy settings.
Another dominating consideration – not forgetting the power of Stack Trace. These text-based logs help determine the source of the problem when an issue arises. In cases where there is a Gradle Sync Failure, appraising the stack trace allows one to discern whether the failure originates from network issues, lack of system resources, inaccuracies in build.gradle files, among others.
gradlew assembleDebug --stacktrace
Lastly, keeping track of deprecated codes or libraries is beneficial too. As technology advances rapidly, certain codes or libraries often become obsolete. Consequently, incorporating outdated elements can trigger different technical issues like Gradle Sync Failure. Regular reviewing of project libraries and codes helps safeguard against obsolete ones.
Regarding the famous quote from Bjarne Stroustrup, developer of C++ language, “Our civilization runs on software. Understanding how to write and maintain programs is particularly important today…”, these steps represent the importance of preventive measures to ensure that our programs run smoothly and efficiently even in the face of challenges like Android Gradle Sync Failed Could Not Resolve All Artifacts For Configuration Classpath.
Visit [Android Developers](https://developer.android.com/studio/build/dependencies) site for more detailed insights about managing dependency configurations.
As we delve deep into the issue of Android Gradle sync failing with an inability to resolve all artifacts for configuration classpath, it becomes evident that this problem brings work to a halt, derailing the workflow of a java developer.
Several elements come into play when discussing about the causes that trigger this error such as:
- Gradle offline mode: The work environment might be in offline mode hence hindering the download of essential dependencies.
- Cache Issue: There could be critical problems linked with cache which needs proper cleaning and restoration.
- Internet Connectivity: Faint or inconsistent internet connectivity is also a crucial aspect contributing towards this failure.
- Incorrectly Formed build.gradle files: Sometimes the culprit behind this trouble is incorrectly formed build.gradle files causing disruption during the sync.
An understanding of these errors leads us to various solutions – turning off the offline mode, clearing the cache, accurate internet connection and thorough verification of build.gradle files can act as keys to address this concern.
To illustrate the correction of Gradle files, ensure the correct placement of dependencies. Here is the representative code sample:
dependencies { classpath 'com.android.tools.build:gradle:3.5.0' }
And finally, keep all your plugins updated according to the latest version of Android studio you are using.
With further consideration, as Bill Gates once said, “The computer was born to solve problems that did not exist before.” Thus, consider each challenge as an opportunity for growth and understand how the components of Android Gradle work in unison. You might just become well-versed in finding effective solutions to similar issues in the process.
Consider surfing through stack overflow discussions or Android Developer’s official documentation for case-specific guidance and a better grip on the subject matter.