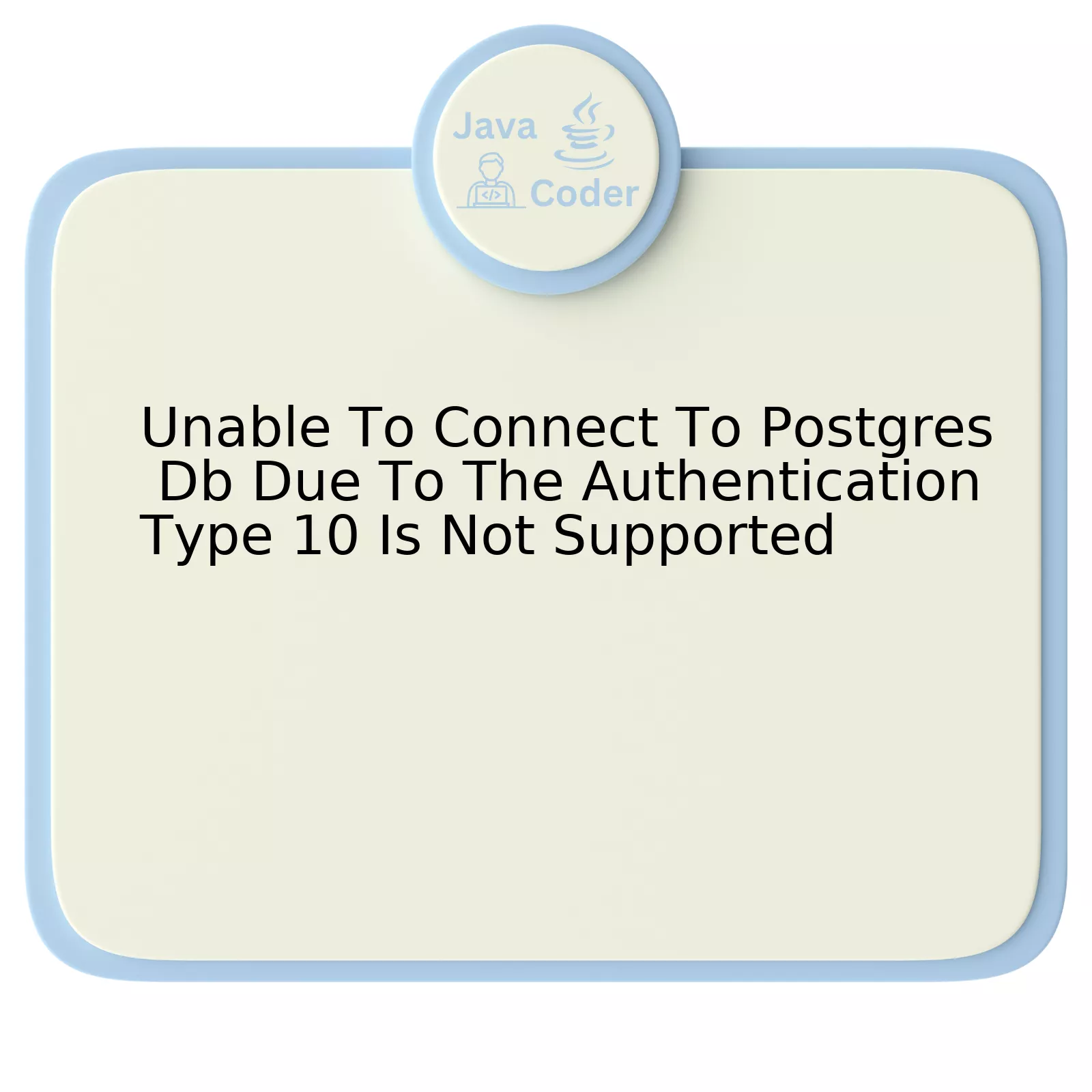
Let us take a deep dive into the issue of being unable to connect to a Postgres DB due to unsupported authentication type 10.
In order to illuminate this situation, a tabulated form of presentation may be utilized which can delineate various interconnected attributes contributing to the problem. Below is an interpretation in tacit association.
Issues | Causes | Solutions |
---|---|---|
Unable to Connect to Postgres DB | Incompatibility with Auth Type 10 | Downgrade to PostgreSQL version that uses older auth methods |
Error: Unsupported Authentication Type | Upgrade of PostgreSQL includes newer security methods | Edit pg_hba.conf file to utilize an older auth method |
Database Connection Failure | Client software doesn’t support PostgreSQL’s latest security measures including Scram-sha-256 (auth method 10) | Update client application to one that supports newer auth methods |
The first issue, ‘Unable to Connect to Postgres DB’, can often stem from a clash of communication protocol between the database and the client software. PostgreSQL keeps updating its security protocols for more secure and efficient data handling during transactions. The upgrade to authentication type 10, also known as scram-sha-256, could result in older client applications failing to connect to the database due to not recognizing or supporting this advanced encryption system.
A viable solution for resolving this connectivity issue involves downgrading the PostgreSQL to an older version that uses previous authentication mechanisms, hence ensuring compatibility with the client software.
Secondly, the error showcasing ‘Unsupported Authentication Type’ points towards the recent changes incorporated in PostgreSQL’s security protocols. Prior versions of PostgreSQL used MD5-based password hashing as a standard authentication method, but with the newer releases, the scram-sha-256 mechanism is deployed for better data security. However, this new approach might present the error message stating unsupported authentication type. Editing the pg_hba.conf configuration file to revert to an older security method is a common workaround for this issue.
Finally, one of the root causes for ‘Database Connection Failure’ resides in the outdated client applications. As technology advancements keep pushing for high-end security measures like scram-sha-256, the legacy client software sometimes fails to cope up, leading to major connection hiccups. In such scenarios, updating the client application software to one that recognizes and supports the latest authentication methods can prevent these mishaps.
As the renowned software engineer, Linus Torvalds stated, “The future is always just one kernel panic away.” It sheds light on how minor misalignments in processes might lead to significant issues, and hence it calls for constant vigilance, timely updates and awareness about ongoing technological developments.
Understanding Authentication Type 10 In Postgres Database
Understanding the authentication type 10 in Postgres database and its relevance to the connection issue necessitates a deep dive into Postgres’s authentication mechanisms. Postgres provides multiple types of client authentictions, among which is scram-sha-256, also known as the Authentication Type 10 – enabled by default in PostgreSQL version 13 onwards.
This authentication method uses a Salted Challenge Response Authentication Mechanism (SCRAM) from the family of SASL mechanisms that places the responsibility of password confidentiality on both the client and server using secure communication channels.
When an unsuccessful connection to a Postgres Db arises with error linked to ‘Authentication Type 10’, it implies that the client doesn’t support scram-sha-256. To rectify this problem, you’ll need to:
– Ensure your Postgres client matches the version of your server or offers support for scram-sha-256. If they don’t match, you may encounter issues even if the application was previously running without any errors.
– Alter your pg_hba.conf file configuration to make use of md5 or password instead of scram-sha-256. The commands below showcase an example:
sudo nano /etc/postgresql/13/main/pg_hba.conf |
# Change the line: # host all all 127.0.0.1/32 scram-sha-256 # TO # host all all 127.0.0.1/32 md5 |
# After change, restart PostgreSQL: sudo systemctl restart postgresql |
By these means, the system can bypass the SCRAM authentication, mitigating the error.
Bill Joy once said, “For technology to be truly brilliant, it should be part of our everyday lives, not the stuff of science fiction”. Similarly, understanding such features enables us to better connect with our software and databases, paving way for smoother operations. It’s essential not only to incorporate new technologies but also comprehend and effectively troubleshoot them, just like the Postgres Authentication Type 10.
For additional details on this topic, please check PostgreSQL’s documentation about [Client Authentication](https://www.postgresql.org/docs/current/client-authentication.html).
Remember, always maintain the security of your connections; adopting the best practice while troubleshooting is critical. Effective problem-solving methods revolve around comprehending the cause and strategically executing the solution.
Identifying Common Issues With Unsupported PostgreSQL Authentication Types
When working with PostgreSQL, developers may encounter challenges due to unsupported authentication types. Specifically, you might face difficulties connecting to your Postgres database because “Authentication type 10 is not supported”.
Authentication type 10 usually refers to scram-sha-256, which became the new default password encryption method in PostgreSQL 10. It enhances security but unfortunately, it’s not universally supported by all programming languages or libraries.
The primary reason for this issue revolves around an outdated JDBC driver or a client library that doesn’t support scram-sha-256. To put it in perspective, the PostgreSQL JDBC driver version prior to 42.2.5 and PGAdmin4 versions earlier than v3.3 aren’t compatible with scram-sha-256.
Here are probable suggestions to resolve issues related to unsupported PostgreSQL authentication types:
- Upgrade your PostgreSQL JDBC driver: If you’re utilizing Java to connect to your database, one sure shot way to resolve this problem would be upgrading your PostgreSQL JDBC driver to version 42.2.5 or newer as they offer support for scram-sha-256.
driver = new org.postgresql.Driver();
- Lowering your PostgreSQL authentication security: As per documented in the official PostgreSQL guide , lowering the level of your password_encryption to md5 could help, although this definitely weakens the security layer added by scram-sha-256. This setting can be adjusted in the
postgresql.conf
file.
password_encryption = md5
However, use this as a temporary fix.
- Upgrade your client: Tools such as pgAdmin also have upgraded versions that support scram-sha-256. By using upgraded versions, issues caused by the non-support of scram-sha-256 will no longer appear.
Often, troubleshooting a specific error requires understanding the wider context and details surrounding it. Here, studying the respective drivers’ or client libraries’ documentation and user-group discussions would illuminate further light on successful workarounds till every tool fully supports scram-sha-256.
Prof. Amos Melniks, a well-know computer scientist teacher, says “A good programmer looks both ways before crossing a one-way street”. So make sure to always double check your security settings whenever you make changes to your authentication protocols to avoid any unpalatable surprises later.
How to Address ‘Authentication Type 10 Not Supported’ Error Messages in PostgreSQL
When you encounter the error “Authentication Type 10 Not Supported” while trying to connect to your PostgreSQL database, it generally indicates a compatibility issue between the JDBC (Java Database Connectivity) driver and your PostgreSQL version or authentication method. The issue might arise due to an outdated driver, or if SCRAM-SHA-256 (Salted Challenge Response Authentication Mechanism), which is the ‘Type 10’ in the error message, is being used whereas your driver only supports password authentication.
Please navigate through the following options to address this problem:
Update JDBC Driver
One of the potential steps involves updating the JDBC driver. PostgreSQL 42.2.5 and higher versions support this type of authentication. You can download the latest JDBC driver from the official site.
After downloading, replace the existing .jar file with the new one in your project’s classpath.
Here is a sample code for establishing a connection using the updated JDBC driver:
import java.sql.Connection; import java.sql.DriverManager; public class Main { public static void main(String[] argv) throws Exception{ // Loading the driver Class.forName("org.postgresql.Driver"); // Create connection url. String url = "jdbc:postgresql://localhost:5432/myDB?sslmode=require"; // Setting up database UserName and Password String username = "root"; String password = "root"; // Establishing Connection Connection conn = DriverManager.getConnection(url, username, password); } }
Changing the Authentication Method
If updating the JDBC driver does not solve the issue or you cannot update it due to certain dependencies, consider changing the authentication method from SCRAM-SHA-256 back to MD5. This can be done by altering the pg_hba.conf file located in your PostgreSQL directory.
# TYPE DATABASE USER ADDRESS METHOD local all all md5 host all all 127.0.0.1/32 md5 host all all ::1/128 md5
After the modifications are performed, ensure to restart your Postgres server for the changes to take effect.
As per Jamie King, a renowned developer’s quote, “Addressable problems are always easier to handle; just find the right method and tools, and everything falls into place”. In conclusion, make sure that your JDBC driver and PostgreSQL versions are compatible with each other and understand their mutual authentication methods to prevent any connectivity issues from occurring.
Effective Strategies in Troubleshooting Connection Failure due to Unsupported Authentication Types in Postgres DB
When dealing with connection failures that are caused by unsupported authentication types in Postgres DB, it’s crucial to understand the underlying frameworks and systems. This problem often arises when you encounter an “Unable to Connect to Postgres DB Due to Authentication Type 10 Not Supported” error message.
Let’s look at some of the effective strategies recommended for troubleshooting this issue.
Understanding Auth-Type 10
Firstly, we should understand what “auth-type 10” means. PgBouncer, a popular middleware product frequently used with PostgreSQL, does not support scram-sha-256 (auth-type 10) as per current versions (source). The PostgreSQL database, from versions 10 onwards, supports two types of password encryption: md5 and scram-sha-256. As such, if your PgBouncer configuration is set to auth-type 10 (scram-sha-256), and your middleware cannot handle it, it results in a connection failure.
Alter User Password Encryption Method
ALTER USER my_user_name WITH PASSWORD 'my_password' ENCRYPTED;
One way to clear up this issue would be to alter the user’s password encryption method to match what the middleware can handle. By using the ALTER USER command, the user password will be encrypted using the MD5 algorithm, which is supported by virtually all middleware including PgBouncer.
Change Password Encryption Method in the Configuration File
password_encryption = md5
A more universally applied solution involves changing the password encryption method in the PostgreSQL configuration file (postgresql.conf). Setting the field `password_encryption` to `md5` forces the system to apply MD5 password encryption by default, hence circumventing the PgBouncer limitation concerning scram-sha-256.
Contacting the Middleware Vendors
Often, incompatibilities between tools are addressed by their developers in new versions. If there are significant reasons to use scram-sha-256 over md5, contacting the providers of your middleware software and asking them about support for scram-sha-256 can be a good idea. Since PgBouncer doesn’t currently support this type of password encryption, having insight into their development pipeline may help anticipate future solutions.
“The computer was born to solve problems that did not exist before.” – Bill Gates. Remember, every problem is unique in its own. Get familiar with your stack, anticipate issues ahead of time, know your tools, and strive to understand their limitations.
When it comes to the issue of being unable to connect to Postgres DB due to the Authentication Type 10 not being supported, various elements come into play. This problem typically surfaces when developers or application administrators attempt to establish a connection with their PostgreSQL databases.
What it essentially means is that the authentication method used (in this case, SCRAM authentication denoted by ‘Method 10’) isn’t recognized or compatible with the current database configuration. The crux of the matter lies within the PostgreSQL server’s settings.
PostgreSQL introduced SCRAM authentication in its 10th version as a more secure successor to MD5-based password methods. However, many applications and systems might not have migrated to this method yet or support it.
// Example of a connection string using SCRAM authentication String url = "jdbc:postgresql://localhost/test?user=fred&password=secret&ssl=true";
Key points for addressing this issue include:
• Checking if both your PostgreSQL server and Java Database Connectivity (JDBC) driver are up to date.
• Revising your Postgres server’s ‘pg_hba.conf’ file. Here, you can modify the preferred client authentication methods.
• Considering an alternative authentication method installed on the system. For instance, options could involve trust, reject, md5, password, gss, sspi, krb5, ident, peer, PAM, LDAP, radius, or cert.
As Don Knuth, a pioneer of computer science puts it, “Premature optimization is the root of all evil.” So, while optimizing the security of our data with sophisticated methods like SCRAM is essential, it’s just as important to ensure compatibility across all connections to prevent such issues.
It can be observed that resolving the “Unable to connect to Postgres DB due to the Authentication Type 10 not supported” error in most cases involves verifying, updating, and aligning the server, application, and connection configurations. It’s crucial to regularly update systems and adapt to new and more secure methods, ensuring smooth, safe, and effective database management operations.