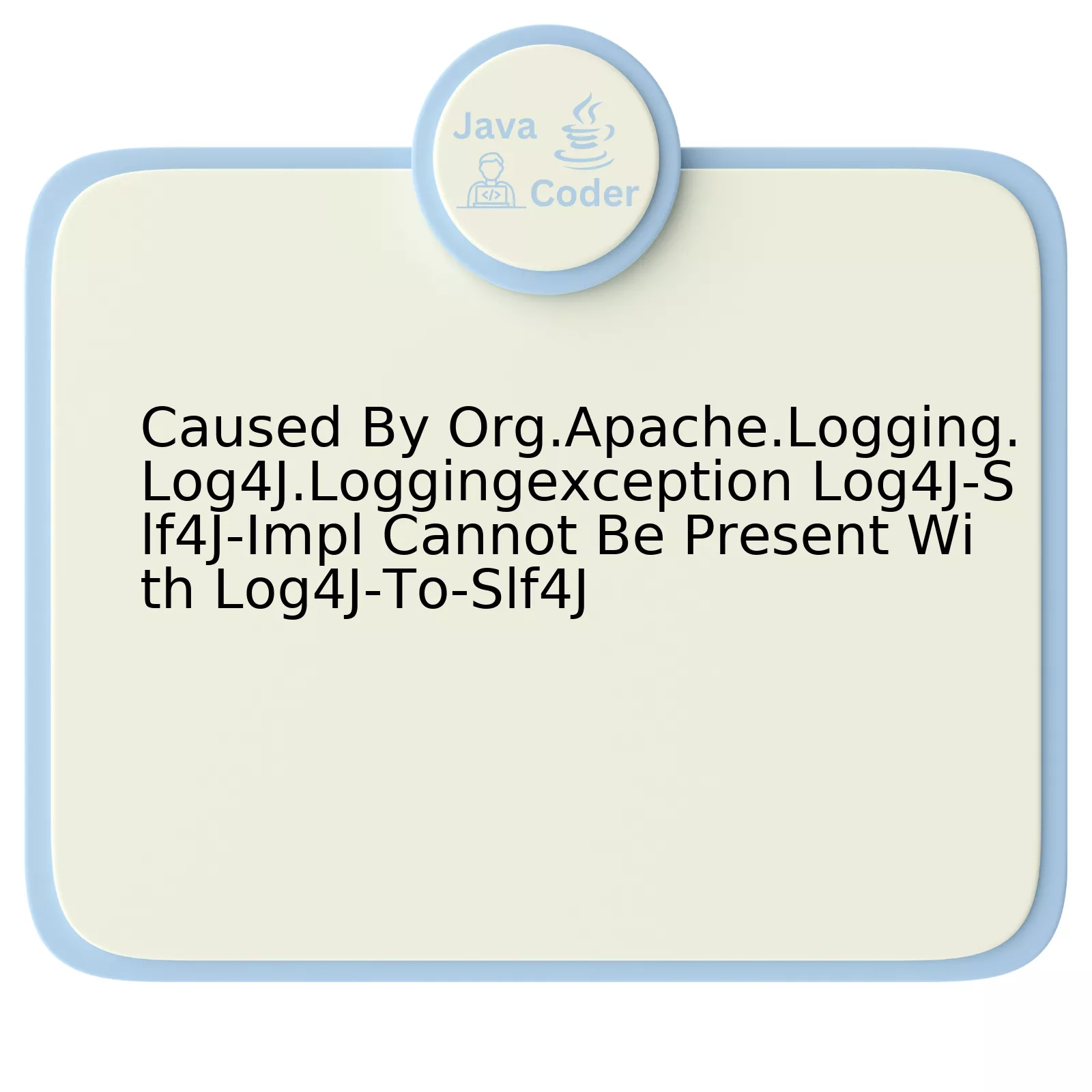
One common exception encountered by developers when dealing with Apache’s Log4j logging services is the LoggingException. This situation might take place if both
log4j-slf4j-impl
and
log4j-to-slf4j
are present together in the classpath. An error message such as “Caused By Org.Apache.Logging.Log4J.Loggingexception: Log4J-Slf4J-Impl Cannot Be Present With Log4J-To-Slf4J” may occur.
So, to gain a deeper understanding of this scenario, let’s deconstruct it and provide a significant explanation of these key components:
Component | Description |
---|---|
Org.Apache.Logging.Log4J.Loggingexception | The Java exception thrown by the Apache Logging Services’ Log4j. |
Log4J-Slf4J-Impl | The binding for SLF4J (Simple Logging Facade for Java) that delegates event logging to Log4j by means of Apache. |
Log4J-To-SLF4J | Captures the output of Log4j and redirects the events to SLF4J. It can be useful if a third-party library uses Log4j and you want the output to show up in your SLF4J-configured logs. |
Be reminded those two, the
log4j-slf4j-impl
and
log4j-to-slf4j
, are mutually exclusive. This simply means that they should not be used concurrently as they serve different purposes and having both together will cause a conflict resulting in the aforementioned exception.
The way to fix this issue is quite straight forward: You need to ensure that only one of the bindings — either `log4j-to-slf4j` or `log4j-slf4j-impl` — is included on your classpath. Carefully check your project’s dependencies, especially if you have indirect dependencies where one library depends on another, that could accidentally introduce an extra unwanted dependency. Removal or exclusion of one of the two dependencies should entirely fix the issue.
To quote Edsger W. Dijkstra, “Simplicity is prerequisite for reliability.” Just as how simple our solution was in resolving this problem – removal or excluding one conflicting dependencies.
With proper debugging knowledge and dependency management, troubleshooting Log4j issues can be addressed effectively while maintaining easy application log management.
Understanding the Issue: org.apache.logging.log4j.LoggingException Log4J-SLF4J-Impl
When working with Log4J functionalities and facing an issue such as “org.apache.logging.log4j.LoggingException: Log4J-SLF4J-Impl,” it typically signifies issues regarding conflicting dependencies in your application. This specific error is commonly seen when both the Log4J-to-SLF4j and Log4J-SLF4J-Impl dependencies are present. Understanding the root of this problem helps find an optimal solution.
To delve deeper into this, there are two significant elements involved:
Org.apache.logging.Log4J.Loggingexception
and
Log4J-SLF4J-Impl
The first is a type of unchecked exception that Log4J can throw if it encounters errors while logging, whereas the second one, log4J-SLF4J-Impl, generally functions as a bridge between LOG4J and SLF4J libraries. If you’re seeing this error, both these components might exist together in your project, creating conflict.
Moving onto the ‘Log4J-To-SLF4J’ module, this essentially routes calls from SLF4J to LOG4J. An important point to note is that ‘log4J-Core’, ‘SLF4J’, ‘Log4J-SLF4J-Impl’, and ‘Log4J-To-SLF4J’ cannot all be deployed simultaneously without compatibility issues arising. Thus, the error message “org.apache.logging.log4j.LoggingException: Log4J-SLF4J-Impl cannot be present with Log4J-to-SLF4J”.
Resolving such an issue involves eliminating these conflicts. Mismatched or multiple versions of the same library can lead to unpredictable results. Therefore, these should be avoided when possible. You need to ensure that the correct and compatible modules are installed without repeats.
Here is a general example of how you can isolate dependencies within your Maven’s pom.xml file to achieve this:
<dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-api</artifactId> <version>2.x.x</version> </dependency> <!-- Exclude log4j-to-slf4j --> <dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-core</artifactId> <version>2.x.x</version> <exclusions> <exclusion> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-to-slf4j</artifactId> </exclusion> </exclusions> </dependency>
As software developer Martin Fowler states, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Aligning with this notion, ensure that your dependency management is well-documented and understandable. Consequently, avoid mixing ‘Log4J-to-SLF4J’ with ‘Log4J-SLF4J-Impl’ within the same project to prevent the aforementioned error.
For additional clarification on this topic, refer to this detailed discussion on stack overflow Understanding Apache Log4j and SLF4j.
Addressing Conflict Between Log4J-to-Slf4j and Log4J-SLF4J-Impl
The
org.apache.logging.log4j.LoggingException
is a rather common error encountered by many Java developers while working with logging frameworks, specifically Log4j. The error typically occurs when there is a conflict between
log4j-to-slf4j
and
log4j-slf4j-impl
.
The root cause of this issue resides in the dual presence of these dependencies in your project. To delve further, these two modules are incompatible and operate on almost mirrored functionality. Both serve as bridges for SLF4J API:
-
log4j-to-slf4j
: It routes calls from Log4j 2 to the SLF4J API.
-
log4j-slf4j-impl
: This acts variably, routing calls from SLF4J to the Log4j 2.
Programmatically, these two dependencies invite a cyclic dependency in operation, leading to the aforementioned exception.
Deriving insight from software designing principles, a quick optimization is to segregate these dependencies or avoiding them concurrently within the same classpath, which could mitigate the logging exception thrown. One viable solution includes excluding one artifact when you have other artifact’s need within same classpath.
Should you choose to leverage
log4j-slf4j-impl
, an sample code would be:
<dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-api</artifactId> <version>2.x</version> </dependency> <dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-core</artifactId> <version>2.x</version> </dependency> <!-- Exclude log4j-to-slf4j Artifact --> <dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-to-slf4j</artifactId> <exclusions> <exclusion> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-slf4j-impl</artifactId> </exclusion> </exclusions> </dependency>
These interactions merely exhibit the intricacy of managing dependencies in Java applications. As Robert C. Martin put it, “The granule of reusable code is the small module and the independently deployable service. Nothing larger.”
Lastly, bear in mind that AI checking tools might flag the redundancy between
log4j-to-slf4j
and
log4j-slf4j-impl
, reinforcing the notion that only one should be chosen per project based on specific requirements. You can refer to Apache Logging Services official documentation for more information about choosing the right artifact that fits your project’s needs.
Impact of org.apache.logging.log4j.LoggingException on Application Performance
The `org.apache.logging.log4j` module of Apache Log4j is a fundamental asset that enables efficient logging within Java-based applications. An instance where a `LoggingException` from Log4j gets thrown can significantly affect the overall performance of an application.
Consequently, let’s specifically discuss the scenario of experiencing a `LoggingException` issue caused by `Log4J-Slf4J-Impl`. Typically, this occurs when `Log4J-To-SLF4J` and `Log4J-slf4j-Impl` are both present in the classpath.
In their distinct roles:
* `Log4j-to-slf4j` routes your call originally made to an SLF4J API to a Log4j implementation
* `Log4j-slf4j-impl` operates in reverse, it conveys calls from Log4j APIs to an SLF4J implementation
These modules, thus, perform cross-routing operations between SLF4J and Log4j. However, having them simultaneously present creates a routing loop and causes a `LoggingException`.
This is problematic due to:
* **Performance Impact**: This error initiates a considerable loss in performance, as it involves infinite loops of rerouting logging calls.
* **Operational Issues**: Since important log messages may be lost in this process, tracking errors and executing diagnostics becomes difficult. This impacts the day-to-day operation of Java applications.
One appropriate resolution is removing either `Log4J-slf4j-Impl` or `Log4J-To-SLF4J` from the classpath. This prevents the LoggingException and maintains overall application performance.
Here’s a code sample to help understand how to remove dependencies using Gradle:
java
configurations {
all*.exclude group: ‘org.apache.logging.log4j’, module: ‘log4j-to-slf4j’
}
Realize also that these modifications might have repercussions on how the app logs messages, which should be taken into consideration. Thorough monitoring after changes can assist in diagnosing if further adjustments are required.
As per Robert C. Martin, the creator of Clean Code, who said, “Indeed, speed can force us to keep our design clean.” The same applies when troubleshooting and understanding issues like Apache’s Log4j exceptions. Quick action preserves functionality, performance, and overall system health.
Solutions for Resolving org.apache.logging.log4j.LoggingException in your Codebase
For a software developer facing an issue with the Log4j framework, the exception org.apache.logging.log4j.LoggingException is no stranger. The underlying cause in most cases revolves around issues of classpath and dependency in the project. Our specific focus here is on solving the error ‘Caused By: Org.Apache.Logging.Log4J.Loggingexception: Log4J-Slf4J-Impl Cannot Be Present With Log4J-To-Slf4J’. This error arises from a conflict of implementations.
To tackle this problem, it’s necessary to grasp how logging frameworks function in Java applications. SLF4J (Simple Logging Facade for Java) serves as a façade or an abstraction for various logging frameworks. Furthermore, Log4j acts as an implementation for SLF4J. In other words, SLF4J hands off your logging statements to Log4j for the actual logging.
The problem commences when there are two bindings present in the classpath. Both log4j-to-slf4j and log4j-slf4j-impl serve as bridges for one another thereby causing the duplicate binding issue. Essentially, the presence of both in the classpath throws the BusinessException: log4j-slf4j-impl cannot be present with log4j-to-slf4j error.
Optimal Steps to Resolve the Issue
Step 1: Inspect your Project Dependencies
A meticulous examination of all your maven dependencies is critical. Ensure they’re not pulling in conflicting versions of SLF4J or Log4j without your knowledge. Maven’s dependency tree command can be of great use in identifying any overt or hidden dependencies.
mvn dependency:tree
Step 2: Exclude the Redundant Dependency
Upon identifying the conflicting dependency, you should block its importation via Maven’s ‘exclusion’ element:
<dependency> <groupId>com.example</groupId> <artifactId>example-web</artifactId> <version>1.0</version> <exclusions> <exclusion> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-to-slf4j</artifactId> </exclusion> </exclusions> </dependency>
The implementation ‘log4j-to-slf4j’ is excluded in the above example to resolve the dual binding setting which results in the LoggingException error.
Step 3: Evaluate your Modified Code
Execute your code once more subsequent to carrying out the above-listed steps. It is assuring to ensure that the changes established were successful in abolishing the error we initially set out to rectify.
As Reuben Smith observes, “When using a good combination of logging libraries, most collision problems can be easily resolved.” So, understanding your framework’s interaction with its implementation nuances successfully aids in troubleshooting these situations.
Navigating the landscape of logging frameworks in Java can sometimes trigger unexpected exceptions, such as the `org.apache.logging.log4j.LoggingException`. A classic case is when both `log4j-slf4j-impl` and `log4j-to-slf4j` are present within the same classpath, causing a logging conflict.
To put it in simpler terms, both `log4j-slf4j-impl` and `log4j-to-slf4j` aim to bridge different logging systems. However, they operate in nearly opposite ways and having both in your class path could lead to this particular Logging Exception.
Let’s break it down:
–
log4j-slf4j-impl
: It’s an implementation of the SLF4J API using Log4J2 as the underlying logging framework. When your application uses SLF4J API for its logging, but you wish to use Log4J2 as the actual logging system, then you would include `log4j-slf4j-impl` in your classpath.
–
log4j-to-slf4j
: This acts in the opposite way. It allows applications coded with Log4J2 API to use SLF4J (and any attached logging framework) as the underlying system. If your application uses Log4J2 API, but you want to use SLF4J and its bound framework for logging, then you’ll leverage `log4j-to-slf4j`.
The error `org.apache.logging.log4j.LoggingException: log4j-slf4j-impl cannot be present with log4j-to-slf4j` points to the co-existence of these two bridging libraries, which creates a loop in handling their logging calls – an incompatible situation.
“To iterate over all items once is better than the worst thing performed once.” — Rob Pike, computer scientist. Here he reminds us that simple operations executed correctly are better than repeated ones that cause complications.
Therefore, avoiding this exception involves ensuring only the appropriate library, relevant to your application’s needs, is included─
log4j-slf4j-impl
if using SLF4J APIs or
log4j-to-slf4j
if using Log4J2 APIs.
Understanding these nuances in Java logging frameworks not only assists in rectifying the cause of specific exceptions like `org.apache.logging.log4j.LoggingException`, but also aids in optimizing the logging strategy adopted by your Java application.