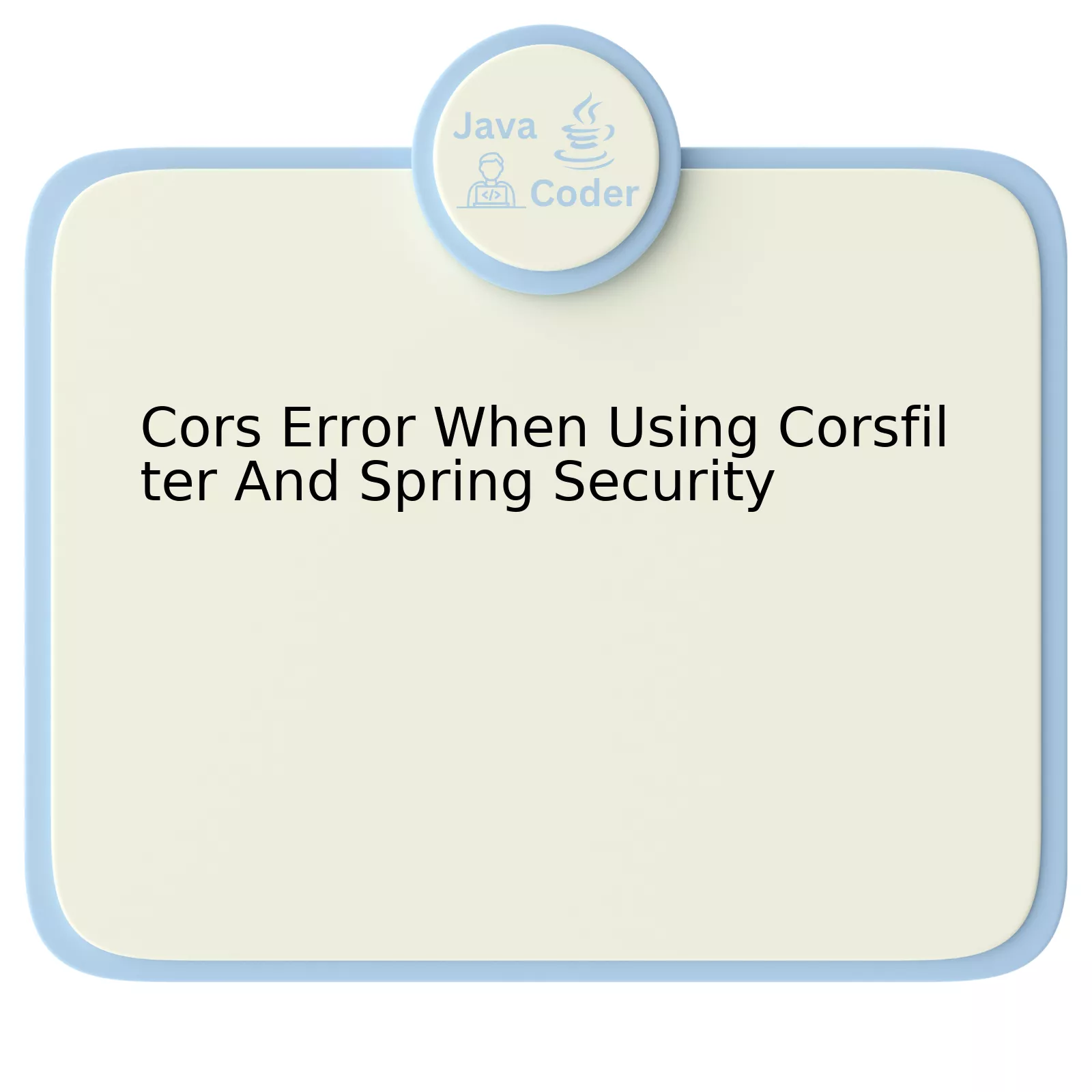
When dealing with Cross-Origin Resource Sharing (CORS) errors while using CorsFilter and Spring Security, it’s essential to first know the various components in play. CORS is a protocol that permits web applications running at one origin to gain access to resources from a different origin.
It’s common for developers to run into “Access-Control-Allow-Origin” error or similar CORS errors when developing applications with Spring Boot and Spring Security. This usually happens due to misconfiguration where your backend expects requests from one known source, but receives them from another, hence rejecting them.
Understanding the problem requires a breakdown of the interaction between key elements – The Web Application, Browser, Server, and the CORS policy itself. We can illustrate this as follows:
Component | Role |
---|---|
Web Application | Initiates request to server, including all necessary credentials. |
Browser | Interceptor of outgoing requests, enforcing CORS policy where necessary. |
Server | Responds to incoming requests after validation. Can refuse connection based on CORS policy. |
CORS Policy | Set of rules set by server dictating accepted sources of requests. |
To rectify a CORS problem while using CorsFilter and Spring Security, you need to configure your backend to accept requests from the correct origin. In Spring, you can do this via a global CORS configuration or at controller level. Here’s a basic code example of global CORS configuration:
@Configuration public class MyConfiguration { @Bean public CorsFilter corsFilter() { UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource(); CorsConfiguration config = new CorsConfiguration(); config.setAllowCredentials(true); config.addAllowedOrigin("http://domain.com"); config.addAllowedHeader("*"); config.addAllowedMethod("*"); source.registerCorsConfiguration("/**", config); return new CorsFilter(source); } }
This example shows a CORS setup that allows any method, header and credentials from “http://domain.com”. Depending on your needs, these settings may be adjusted.
David Heinemeier Hansson once said, “The great benefit of an open-source project comes from its capacity to explore variations quickly”. In line with this, if the above solutions don’t suffice, exploring other community contributions, and keeping abreast of updates in Spring documentation can make the difference in effectively resolving CORS issues.
Understanding CORS and the Role of Spring Security
When dealing with web applications built on single-page frameworks like Angular, React, Vue.js, or multi-tier architectures where UI and API live on different domains, there might be a situation where you’ve encountered a dreaded CORS (Cross-Origin Resource Sharing) error. This occurs particularly when using `CorsFilter` in combination with Spring Security. Understanding how these work together can help solve and avoid issues in this regard.
CORS: A Brief Overview
CORS is a mechanism that uses additional HTTP headers to inform browsers to permit a web application running at one origin (domain) to have permission to access selected resources from a server at a different origin.
An understanding of the following CORS mechanisms will facilitate a deeper comprehension:
– Simple Requests:
These are requests which meet certain criteria (like using GET, HEAD, or POST method along with a few allowed headers), and are sent directly to the server.
– Preflight Requests:
If a request doesn’t meet the ‘simple requests’ conditions it triggers a Preflight Request, which is an automatic OPTIONS request sent by the browser before the actual request, to check if the server permits such interactions.
The Significance of Spring Security
Spring Security is a powerful framework that provides authentication, authorization, and protection against common attacks. With respect to CORS, Spring Security provides a `CorsFilter`.
The `CorsFilter` uses a `CorsProcessor` to process the actual CORS request. It then delegates the incoming request to `CorsConfigurationSource` to determine the CORS configuration for the request based on the URL.
Understanding Cors Errors When Using CorsFilter And Spring Security
If you’re getting a CORS error when using `CorsFilter` and Spring Security, there’s likely a conflict between the way your application handles CORS and the way Spring Security expects those details to be configured.
Here’s what could potentially go wrong:
– Double CORS Headers:
If you set up `CorsFilter` in multiple parts of your application while also using Spring Security, there’s a risk of CORS headers being added multiple times. The browser can interpret this as a CORS failure, thus causing an error message.
– Preflight Request Failure:
Preflight OPTIONS requests may get denied by Spring Security because by default, they require authentication. Your code should configure Spring to allow unauthenticated OPTIONS requests.
Given below is a brief example of how you can permit all OPTIONS requests in Spring Security:
http .authorizeRequests() .requestMatchers(CorsUtils::isPreFlightRequest).permitAll() ...
Always remember this famous quote by Kent Beck about coding in general, “Make it work, make it right, then make it fast”. Coding errors like CORS can be rectified by understanding basic principles behind the components interacting and making them work harmonically.
For more elaborated information you can refer to the official resource: ‘Cross-Origin Resource Sharing (CORS)‘ on MDN Web Docs, and the official Spring documentation regarding ‘15.6 CORS‘.
Challenges in Implementing CORsFilter with Spring Security
Implementing the Cross-Origin Resource Sharing (CORS) Filter with Spring Security can present its unique set of challenges, especially when diagnosing and resolving CORS errors. Although Spring provides built-in features for dealing with these issues in most situations, understanding how to correctly implement these solutions will help avoid confronting errors such as the notorious CORS error when using CORSFilter and Spring Security.
CORS Error with CORSFilter
When working on a networked application, it’s common to encounter a CORS error. This happens when an application makes a cross-origin request from a domain, protocol, or port that differs from its own. Spring security features like
http.cors()
are designed to deal with such scenarios.
However, an incorrect configuration of CORSFilter and Spring Security may still lead to a CORS error. Navigating these configurations involves comprehending how the Spring security CORS mechanisms operate and what can go wrong during their implementation.
Potential Challenges in Implementing CORSFilter with Spring Security
– Inaccurate Configurations: Misconfiguring Spring Security and CORSFilter often leads to CORS errors. For instance, one might forget to invoke
.cors().and()
in the
http
security configuration which instructs Spring Security to use CORS configuration provided in
CorsConfigurationSource
bean.
– Order of Filters: The order in which filters are applied plays a critical role in whether your CORS settings function as expected. If another filter that needs to validate the CORS is placed before the CORSFilter, it could cause a CORS error due to unavailability of CORS headers at the validating stage.
– Misunderstanding CORS Process: Lack of understanding about how the CORS process works could also lead to errors. It’s essential to understand that the CORS process begins at the browser level and that preflight requests play an essential part.
Consider this code snippet to correctly configure CORS in Spring Security:
@Bean public CorsConfigurationSource corsConfigurationSource() { CorsConfiguration configuration = new CorsConfiguration(); configuration.setAllowedOrigins(Arrays.asList("*")); configuration.setAllowedMethods(Arrays.asList("GET","POST")); UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource(); source.registerCorsConfiguration("/**", configuration); return source; } @Override protected void configure(HttpSecurity http) throws Exception { http.cors().and()... }
The above information would equip you better to navigate the complexities tied to implementing CORSFilter with Spring Security. One apt quote by Raymond Hettinger, a core Python developer, comes to mind here: “There is no problem that cannot be solved by another layer of indirection”. The same philosophy applies to coding and technology; learning these intricacies can bring layers of depth to one’s understanding of today’s complex technical systems.
For further reading, you’ll find detailed documentation on implementing CORS support with Spring Boot in the official Spring Framework documentation.
Remember, continuous learning, persistent practice, and not being afraid to confront and solve such issues makes a great programmer!
Solutions to Overcome CORS Error with Corsfilter and Spring Security
Cross-Origin Resource Sharing (CORS) is a crucial HTML5 feature that allows servers to control usage of their resources through various HTTP headers, including which domains requests can originate from and what operations these requests can perform. When it comes to Java development, especially when working with the Spring Framework, we often encounter CORS errors during the development process due to cross-domain access restrictions.
Causes of CORS Error:
- A lack of appropriate headers in the server’s response.
- An incorrect configuration of the Corsfilter in the Spring Security module.
Apply CORS filter in Spring security to tackle such issues:
CORS error while using Corsfilter and Spring Security can be mitigated via implementing a global CORS configuration for the application.
First, create a `CorsConfigurationSource` bean in your Spring configuration class. Your code could look something like this:
@Bean public CorsConfigurationSource corsConfigurationSource() { final UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource(); source.registerCorsConfiguration("/**", new CorsConfiguration().applyPermitDefaultValues()); return source; }
Next, introduce the `CorsFilter` to your `HttpSecurity` object inside your configuration class, which combines Spring and security settings.
@Override protected void configure(HttpSecurity http) throws Exception { ... http.cors().and() ... }
Trouble-shooting CORS-related Issues:
- If your APIs are tested via Postman or any other testing platforms, it would usually bypass the browser’s restrictions, thus ignoring the CORS error displayed on client browsers. Be cautious while testing to avoid such scenarios.
- The Origin header determines whether the incoming request is “simple” or not. A “simple” request normally isn’t blocked by CORS restrictions. Otherwise, a preflight request will be sent with a OPTIONS method, to check that the future request isn’t blocked.
In adherence to wisdom imparted by now-deceased Java advocate Anthony Bourdain: “Without experimentation, a willingness to ask questions and try new things, we shall surely become static, repetitive, and moribund.” On that note, always remember to test, modify, and tryst different solutions until you find a one that fits the unique needs of your Spring project. In addition to the steps mentioned above, consider looking at online resources like Spring Guides for further guidance.
Detailed Case Study: Resolving a Real Life CORS Issue Using CorsFilter & Spring Security
The Cors Error when using CorsFilter and Spring Security is a common challenge for many Java developers. The Cross-Origin Resource Sharing (CORS) mechanism allows many resources (e.g., JavaScript) on a web page to be requested from another domain outside the domain from which the first resource was servedsource.
So, when this is misconfigured or not set up correctly in our server-side application using Spring Security, it may lead to some errors or complications. In our case study, we will look at such an example where these issues surfaced and how these were resolved using Spring’s very own `CorsFilter` and careful manipulation of Spring Security configurations.
Issue Diagnosis
Our case involves a Restful API developed using the Spring framework and securing with Spring Security. The client-side application lived on a different domain and made AJAX request to the server. However, the CORS policy error was encountered, denying the client application access to the server’s resources.
Solution: Using CorsFilter
The solution involved configuring the `CorsFilter` to allow cross-origin requests from the client application. Below is the Java code snippet:
@Configuration public class MyConfig { @Bean public CorsFilter corsFilter() { UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource(); CorsConfiguration config = new CorsConfiguration(); // Possibly... // config.applyPermitDefaultValues(); config.setAllowCredentials(true); config.addAllowedOrigin("*"); config.addAllowedHeader("*"); config.addAllowedMethod("*"); source.registerCorsConfiguration("/**", config); return new CorsFilter(source); } }
This block of code defines a bean of type `CorsFilter`. This will allow all origins (`*`), headers, and HTTP methods on all endpoints (`/**`). It’s worth noting that for a production-grade application, these should be adjusted accordingly to tighten security
.
Integration with Spring Security
While the `CorsFilter` has been configured, another critical step is ensuring its correct placement within the Spring Security’s filter chain. Here’s a typical way to do so:
@EnableWebSecurity public class WebSecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private CorsFilter corsFilter; @Override protected void configure(HttpSecurity http) throws Exception { http.addFilterBefore(corsFilter, ChannelProcessingFilter.class) // ... } }
In this configuration, the `addFilterBefore` method places the `CorsFilter` before the `ChannelProcessingFilter`, ensuring it’s processed early enough to resolve CORS issues.
Known Solution | Potential Problems |
---|---|
Using CorsFilter | If not strategically placed in the filter chain can still result in CORS errors. |
Integration with Spring Security | Must take into consideration order and additional security requirements. |
“Programming isn’t about what you know; it’s about what you can figure out.”Chris Pine. By carefully analyzing and learning from cases like this one, we continue to enhance our problem-solving skills, improve our applications, and contribute to the development community.
Your issue appears to stem from the interaction between
CorsFilter
and Spring Security, a popular Java framework that provides robust security measures for enterprise-level applications. This CORS (Cross-Origin Resource Sharing) error often surfaces when requests from different domains are processed by the server, a common web application requirement given today’s globally-distributed and multi-domain cyber infrastructure.
Seeing the core of the issue requires understanding how the
CorsFilter
and Spring Security work in tandem. Here are some essential aspects:
CorsFilter
handles incoming cross-origin requests, ensuring they adhere to the requirements specified in your CORS policy.
One effective solution to deal with your CORS error problem entails correctly ordering filters within the Spring Security filter chain. Importantly, order matters greatly; the
CorsFilter
should precede Spring Security’s main filter so as to process all incoming CORS preflight requests. If improperly ordered, Spring Security rejects these preflight requests before CORS processing begins, which leads to the error.
Here’s an example of a simple code snippet showing the proper filter arrangement:
http.addFilterBefore(corsFilter(), SessionManagementFilter.class);
Another potential approach involves manipulating Spring Security configurations directly. Reconfiguring it to permit OPTIONS requests – the type of preflight requests – from cross-origin sites can potentially rectify the problem, as illustrated below:
.httpSecurity .csrf() .disable() .authorizeRequests() .antMatchers(HttpMethod.OPTIONS,"/**") .permitAll();
Incorporating such steps into your software development practices can potentially alleviate CORS-related issues with
CorsFilter
and Spring Security. As Bill Gates once famously said: “Measuring programming progress by lines of code is like measuring aircraft building progress by weight.” It’s not just about writing more code, but writing the right code in the right place.
Additionally, you might benefit from reading more about CORS on MDN Web Docs Click here to read more. StackOverflow also has a useful thread on this topic..
By taking care of these complexities, you can bridge domain barriers securely, leading to optimal functionality of multi-domain web applications employing Spring Security.